C++ offers more control over system resources and performance, making it a preferred choice for game development, while C# provides a simpler syntax and is commonly used with Unity for rapid development.
Here's a simple code snippet showcasing a basic class structure in both languages:
C++:
class Player {
public:
Player(std::string name) : playerName(name) {}
void display() {
std::cout << "Player Name: " << playerName << std::endl;
}
private:
std::string playerName;
};
C#:
public class Player {
private string playerName;
public Player(string name) {
playerName = name;
}
public void Display() {
Console.WriteLine("Player Name: " + playerName);
}
}
Understanding C++ and C#
What is C++?
C++ is a general-purpose programming language developed in the early 1980s as an extension of the C programming language. It introduced the concept of object-oriented programming (OOP) and has since become a staple in various domains, especially in game development. C++ provides developers with the ability to perform low-level operations and offers unparalleled performance optimizations.
Characteristics of C++ include:
- Low-Level Memory Manipulation: C++ allows direct manipulation of memory through pointers, making it highly efficient for resource-intensive applications.
- Performance: Due to its compiled nature, C++ code executes closer to the machine level, leading to faster runtime performance, which is essential for game development.
What is C#?
C#, pronounced "C-sharp," is a higher-level programming language developed by Microsoft in 2000 as part of its .NET initiative. It is widely known for its simplicity and ease of use, making it a popular choice for game development, particularly with the Unity game engine.
C# characteristics include:
- Managed Code: C# runs on the Common Language Runtime (CLR), which automatically manages memory for you. This feature reduces the risk of memory leaks—a common issue in C++ due to manual memory management.
- Simplicity: The syntax of C# is more user-friendly compared to C++. This lower barrier to entry makes it appealing, especially for beginners in game development.
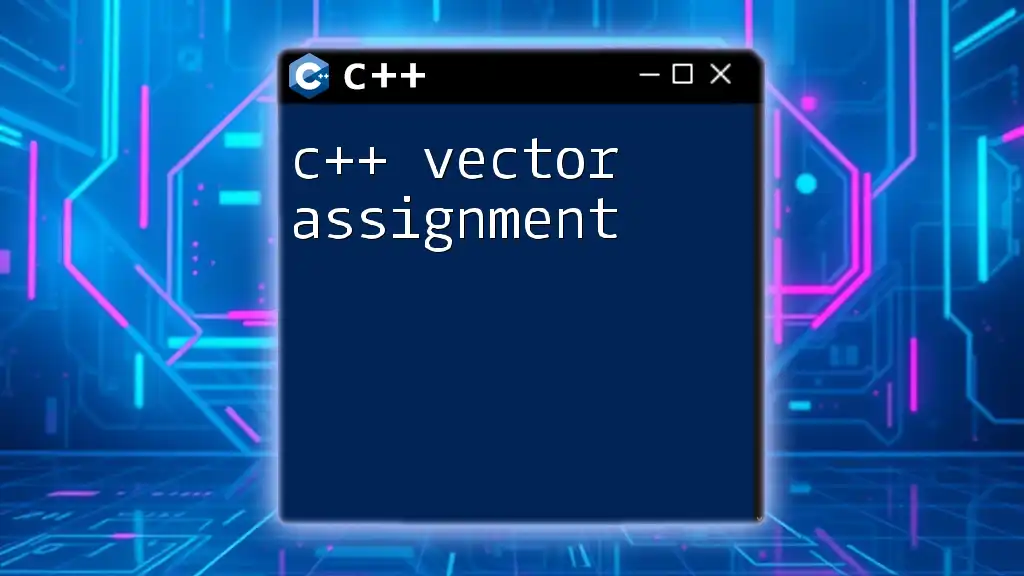
Performance Comparison
Speed and Efficiency
When discussing C++ vs C# for games, one must consider speed and efficiency. C++ typically offers superior performance through its capacity for low-level operations.
In C++, developers can optimize operations for speed. Take a simple example of manipulating an array:
void manipulateMemory(int* arr, int size) {
for (int i = 0; i < size; i++) {
arr[i] += 1;
}
}
The above code directly accesses memory addresses, allowing for rapid execution, which is critical in performance-intensive applications, such as high-frame-rate games.
In contrast, C# prioritizes ease of use, which can sometimes come at the expense of performance. While C# has improved significantly over the years, it still utilizes garbage collection to manage memory, which can lead to unpredictable pauses in execution, especially during critical game moments.
Graphics and Processing Power
C++ has solidified its reputation in graphic-intensive gaming through engines like Unreal Engine, which allows developers to harness high-level graphics capabilities with low-level access. This is particularly important for AAA titles where graphics quality is paramount.
On the other hand, C# shines in game design with Unity, offering a streamlined process for rapid game development. However, for games that require the highest possible graphics fidelity and optimization, C++ may be the more suitable choice.
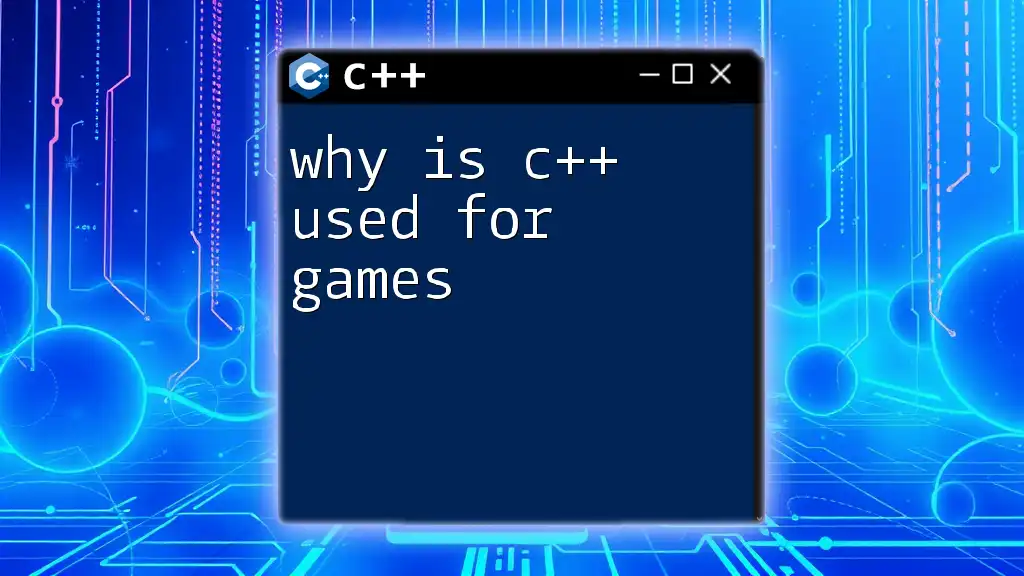
Development Environment and Ecosystem
Tools and Resources
The development tools available for both languages can significantly impact the game development experience.
For C++:
- Visual Studio: Offers a robust IDE with advanced debugging tools and extensive library support.
- Clion: A powerful IDE from JetBrains that comes with smart coding assistance features tailored for C++ developers.
For C#:
- Unity: Arguably the most famous game engine using C#, it provides a large ecosystem of resources, templates, and easy asset imports.
- Visual Studio: Like with C++, Visual Studio also offers a powerful environment for C# development.
Community and Support
Both C++ and C# benefit from strong communities. C++ has long been established in various fields, leading to a wealth of libraries, frameworks, and documentation. C# enjoys the support of a vibrant community within the Unity engine, providing numerous tutorials, forums, and user-generated resources.
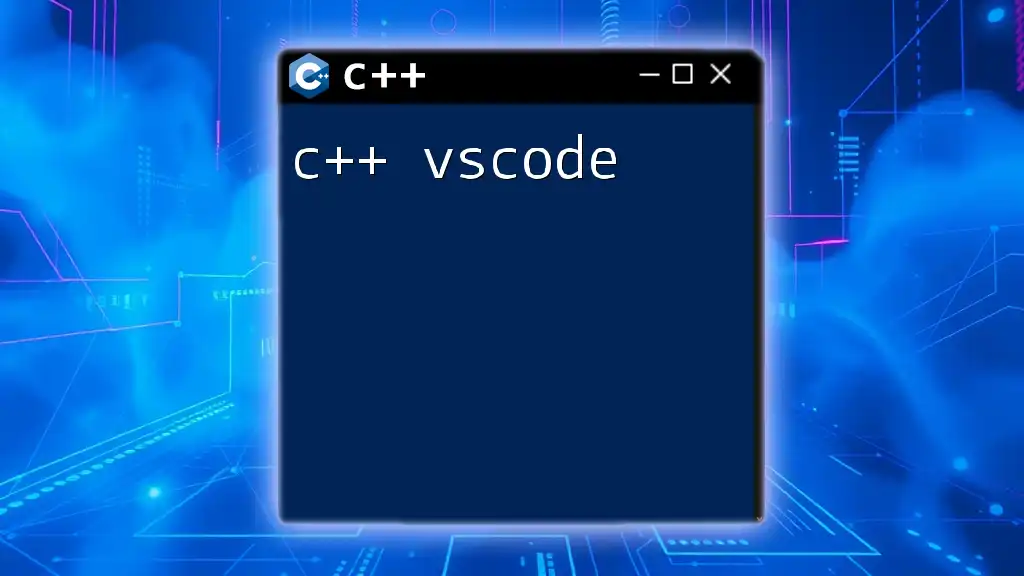
Language Features and Syntax
C++ Language Features
C++ retains complex features that allow for powerful programming but can also create a steeper learning curve:
- Object-Oriented Features: C++ expands on C’s capabilities by introducing classes and objects, allowing for modular code architecture.
- Multiple Inheritance: C++ allows a class to inherit multiple base classes, a feature not found in C#.
- Operator Overloading: This feature allows developers to define custom behaviors for operators, enhancing class usability.
Example of a simple class hierarchy in C++:
class Base {
public:
virtual void show() { std::cout << "Base class show." << std::endl; }
};
class Derived : public Base {
public:
void show() { std::cout << "Derived class show." << std::endl; }
};
C# Language Features
C# focuses on simplicity and developer productivity:
- Garbage Collection: Significantly reduces the burden of manual memory management, allowing developers to focus on game logic rather than memory allocation and deallocation.
- Built-in High-Level Features: Features like properties, events, and async programming enable a streamlined approach to game development.
C# class example similar to the one provided for C++:
class Base {
public virtual void Show() {
Console.WriteLine("Base class show.");
}
}
class Derived : Base {
public override void Show() {
Console.WriteLine("Derived class show.");
}
}
This example illustrates the straightforward and clean syntax that C# offers, which is appreciated particularly by beginners.
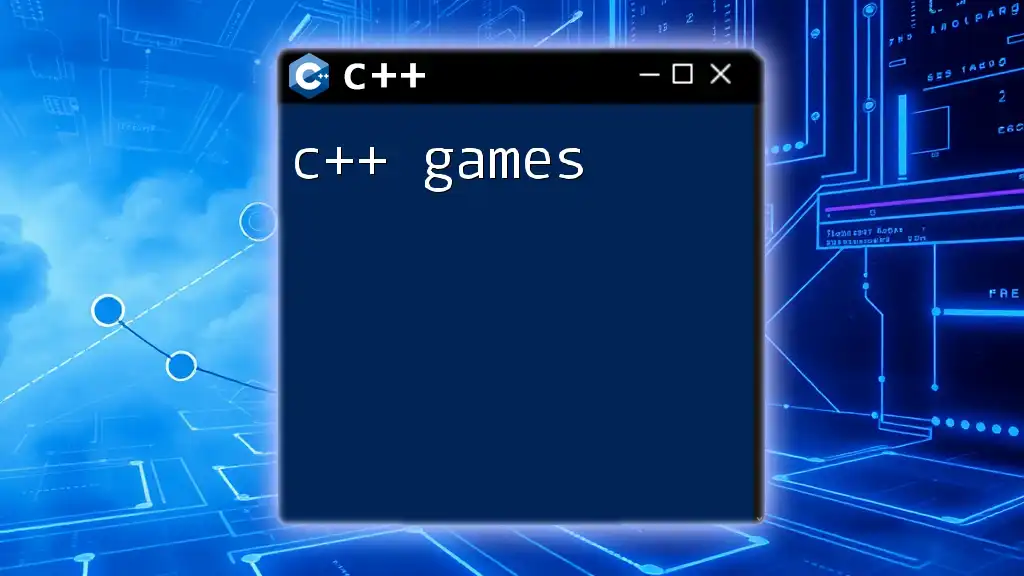
Game Development Use Cases
When to Use C++
C++ is often the preferred choice for large-scale projects where performance is key. Examples include:
- AAA Games: Titles that require extensive resources and optimization often benefit from C++’s performance capabilities.
- Custom Game Engines: Developers working on unique engines for specific artistic visions can leverage C++ to optimize every aspect of the engine.
When to Use C#
C# is ideal for:
- Rapid Development: Small and medium-sized games that require quick iterations and frequent updates.
- Indie Games: Indie developers benefit from the simplicity and speed of development, allowing for greater creativity without extensive programming knowledge.
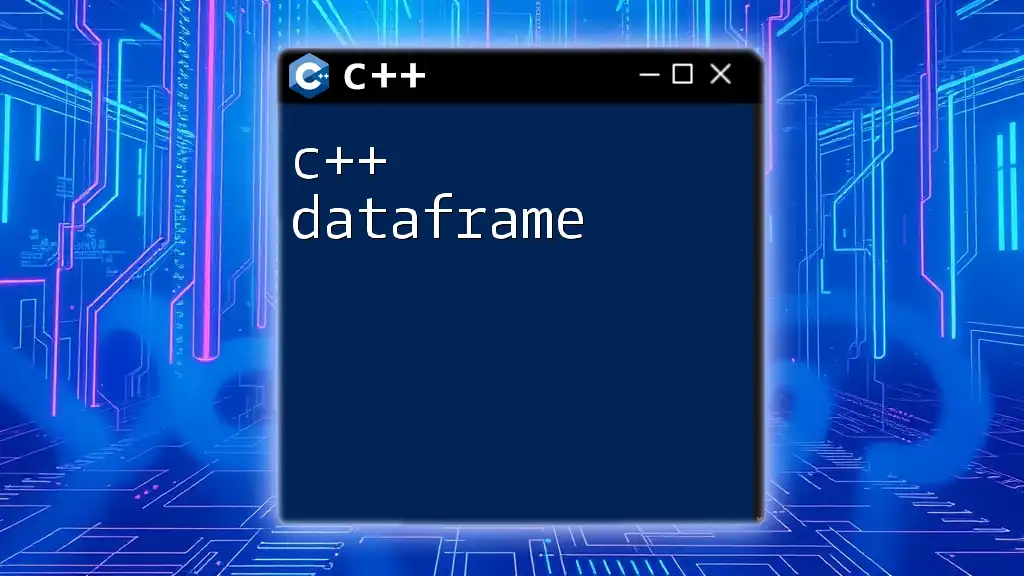
The Learning Curve
C++ Learning Curve
The learning curve for C++ can be steep, especially for newcomers. Key challenges include:
- Pointer Arithmetic: Understanding pointers and memory addresses is crucial yet complex.
- Manual Memory Management: Developers must track their memory allocation and deallocation, which can lead to bugs if not carefully handled.
Despite these challenges, many resources are available to facilitate learning, such as books, online courses, and coding communities focused on C++.
C# Learning Curve
C#, conversely, presents a gentler learning curve. The structure and syntax are straightforward, making it easier to grasp fundamental programming concepts. Developers can quickly begin creating games using Unity, often without extensive programming experience.
Numerous resources are available for beginners, including official documentation, online forums, and vast tutorial libraries.
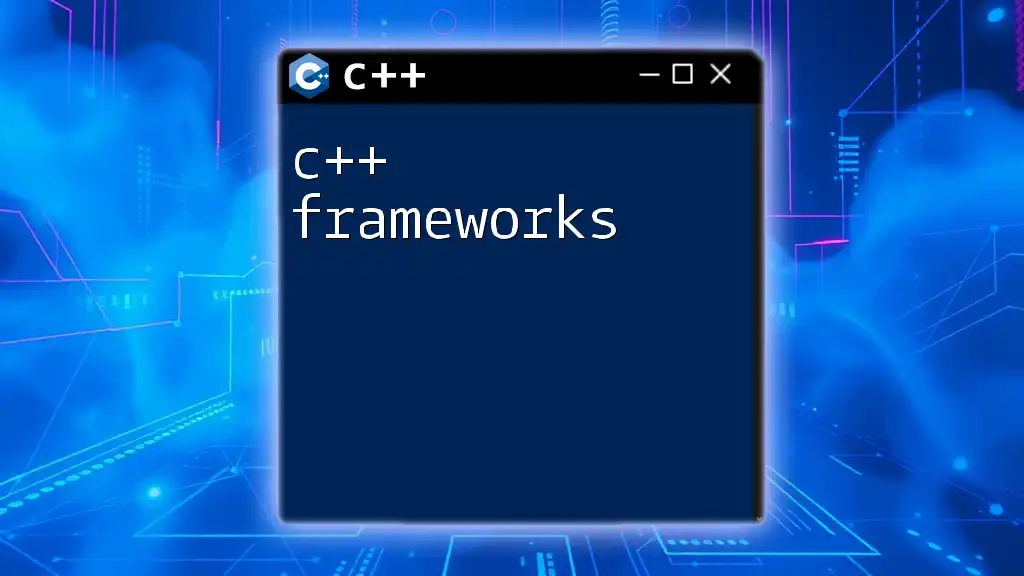
Conclusion
When exploring C++ vs C# for games, it is essential to weigh each language's strengths and weaknesses against your project's specific needs. C++ offers unmatched performance and control, making it ideal for high-demand environments, whereas C# provides accessibility and rapid development, perfect for smaller games or those new to programming.
Each language serves its purpose in the game development spectrum, and understanding these nuances can help you make an informed decision tailored to your project.
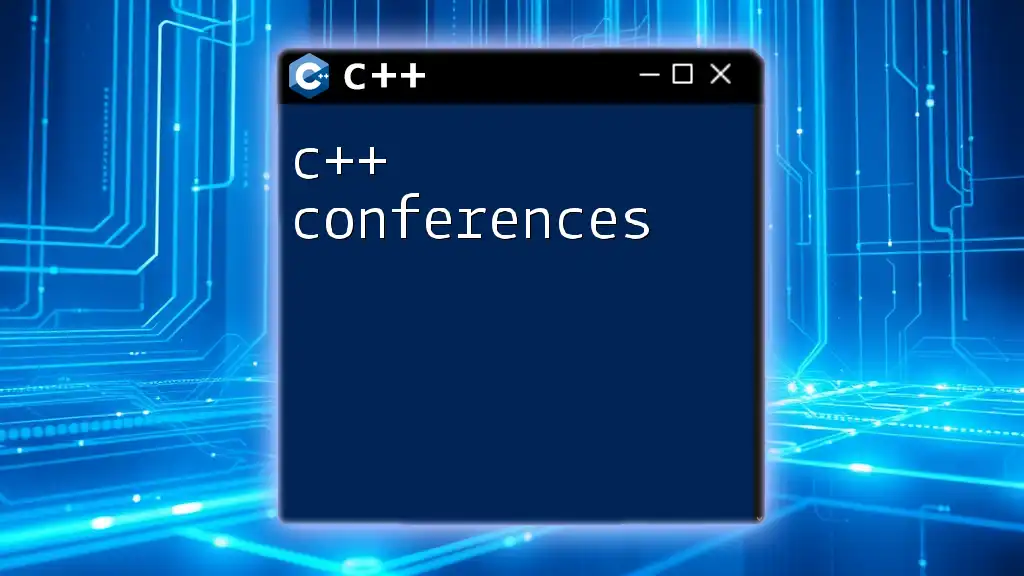
FAQs
Common Questions About C++ and C#
Is C++ better for game performance? Yes, in most cases, C++ outperforms C# due to its low-level capabilities and optimization potentials.
Can you use both languages in a single project? Yes, it is possible to use both languages in a project, especially with engines like Unity, where C++ can be used for performance-critical components and C# for game logic.
Which language is more suitable for VR development? Both languages have their place in VR development. C++ is often used in high-performance applications, while C# with Unity allows for rapid development and prototyping, which is also crucial in VR.
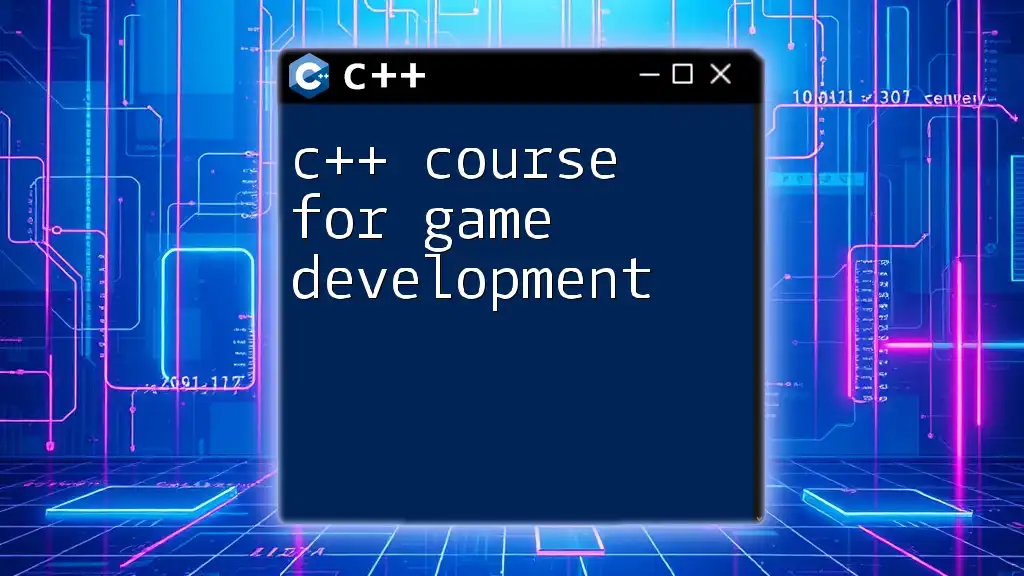
Call to Action
For those eager to dive deeper into game development, consider subscribing to our newsletter for insightful tutorials and guides on mastering C++ and C# commands. Additionally, take advantage of our free eBook, designed to help you get started on your game development journey using either language.