To clear the console in C++, you can use system commands that vary by operating system; for example, on Windows, you can use `system("cls");`, and on Unix-based systems, you can use `system("clear");`.
Here’s a code snippet demonstrating both:
#include <cstdlib> // Required for system()
int main() {
#ifdef _WIN32
system("cls"); // Clear console for Windows
#else
system("clear"); // Clear console for Unix-based systems
#endif
return 0;
}
Understanding the Need to Clear the Console
In C++, effectively managing console output is crucial for providing a streamlined user experience. During the development process, especially for interactive applications or command-line tools, output clutter can reduce readability and confuse users. This is where understanding how to clear the console in C++ comes into play.
You might want to clear the console for several reasons:
-
Enhancing User Experience: A cleaner console can make it easier for users to focus on the current task without distractions from previous outputs.
-
Managing Output for Complex Applications: In applications that display ongoing results or metrics, regularly clearing the console can help maintain a manageable output.
-
Maintaining Readability During Iterative Processes: For loops or repeated user inputs, clearing the screen every iteration can ensure that the updated information is presented clearly.
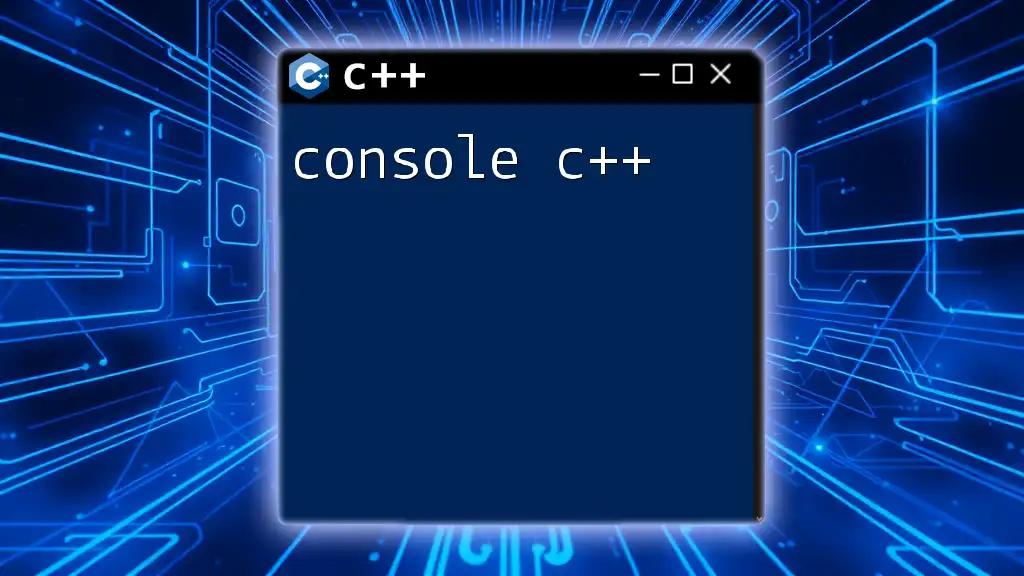
Methods to Clear Console in C++
Using System Commands
What is System Command?
In C++, system commands allow you to execute operating system-level commands directly from your code. This capability also provides an avenue to manipulate console behavior, including clearing the screen.
How to Clear Screen in C++ using `system("cls")`
On Windows systems, you can use the following command to clear the console:
#include <iostream>
#include <cstdlib>
int main() {
system("cls"); // clears the console screen on Windows
std::cout << "Console Cleared!" << std::endl;
return 0;
}
Explanation: The `system("cls")` command instructs the operating system to carry out the "cls" command, which is predefined in Windows to clear the console. This method is simple but comes with its drawbacks, especially in terms of portability and performance.
Using `system("clear")` for Unix/Linux
For Unix-based systems (Linux, MacOS), the command differs slightly:
#include <iostream>
#include <cstdlib>
int main() {
system("clear"); // clears the console screen on Unix/Linux
std::cout << "Console Cleared!" << std::endl;
return 0;
}
Explanation: Here, `system("clear")` functions similarly to clear the screen but is tailored for Unix-like environments.
Creating a Cross-Platform Clear Screen Function
Why Create a Custom Function?
Using system commands, while straightforward, can be risky as they depend heavily on the underlying operating system. Instead, it's a good practice to write a custom function that adapts to different environments.
Code Snippet: Custom Clear Function
#include <iostream>
void clearConsole() {
#ifdef _WIN32
system("cls");
#else
system("clear");
#endif
}
int main() {
clearConsole();
std::cout << "Console Cleared!" << std::endl;
return 0;
}
Explanation: This function checks the operating system using the preprocessor directive `#ifdef _WIN32`. It executes the appropriate command based on whether it's running on Windows or a Unix-based system, thus providing cross-platform compatibility.

Alternative Methods to Clear Console without System Calls
Using ANSI Escape Codes
What are ANSI Escape Codes?
ANSI Escape Codes are sequences of characters that allow you to control text formatting, colors, and cursor position in a terminal. They can also be used to clear the console.
Code Snippet for Clearing the Screen
#include <iostream>
void clearConsole() {
std::cout << "\033[2J\033[1;1H"; // ANSI escape codes to clear screen and reset cursor
}
int main() {
clearConsole();
std::cout << "Console Cleared!" << std::endl;
return 0;
}
Explanation: The code snippet utilizes `\033[2J` to clear the entire screen and `\033[1;1H` to reposition the cursor at the beginning of the console. This method works on most Unix-like systems and terminals that support ANSI escape codes.
Simple Character Spamming
Alternative Approach Without Clearing
Sometimes, instead of actually clearing the console, you might just want to push the previous output "off-screen." This can be achieved by printing a series of new lines.
Code Snippet: Spamming New Lines
#include <iostream>
void pseudoClear() {
for (int i = 0; i < 100; i++) {
std::cout << std::endl; // effectively pushes old content out of view
}
}
int main() {
pseudoClear();
std::cout << "Pseudo Console Cleared!" << std::endl;
return 0;
}
Explanation: This method may not truly clear the console, but it visually removes old content by scrolling it out of view. It’s simple but not the most efficient or effective solution.

Performance Considerations
When it comes to clearing the console, performance should be a consideration, especially in applications where this operation may happen frequently.
-
Using System Calls: Though convenient, employing `system` calls is generally inefficient. Each call spawns a new process, which can slow down an application, particularly if it’s being called repeatedly in a loop.
-
Using ANSI Codes: This method is typically faster since it determines screen manipulation directly without the overhead of launching new processes. However, not all terminals support these codes, which may limit their usability.
To ensure optimal performance, consider the specific needs of your application and decide on an appropriate method based on frequency and compatibility.

Conclusion
Overall, understanding how to clear the console in C++ opens a variety of avenues for enhancing the user experience and managing output effectively. Whether you choose to utilize system commands, ANSI escape codes, or a custom-built function, the key is to find a method that resonates with your coding style and the requirements of your project.
Experiment with the different methods and adapt them according to your needs. Regardless of your chosen approach, mastering console management in C++ will undoubtedly improve your programming toolkit.

Frequently Asked Questions
Is clearing the console necessary in every C++ program?
While not always necessary, clearing the console can improve readability and user interaction, especially in command-line applications.
What are the risks of using system calls?
Using system calls can expose your application to security risks, reduce performance, and limit portability across different OS platforms.
Can I use these methods in a GUI application?
In GUI applications, these methods are not applicable since they target console output. Instead, consider using built-in GUI libraries for managing visual output.