In C++, console logging is typically done using the `std::cout` stream, which outputs text to the standard output (console).
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Console Output in C++
What is Console Output?
Console output refers to the messages displayed in a console window or terminal during program execution. It plays a vital role during development and debugging, allowing developers to observe the program's behavior and output data without needing a graphical interface.
The C++ Standard Library and Console Output
The C++ Standard Library provides essential tools for handling console output, including the `<iostream>` header, which contains the facilities to perform input and output (I/O) operations. Understanding how to leverage this library is crucial for effective console logging.
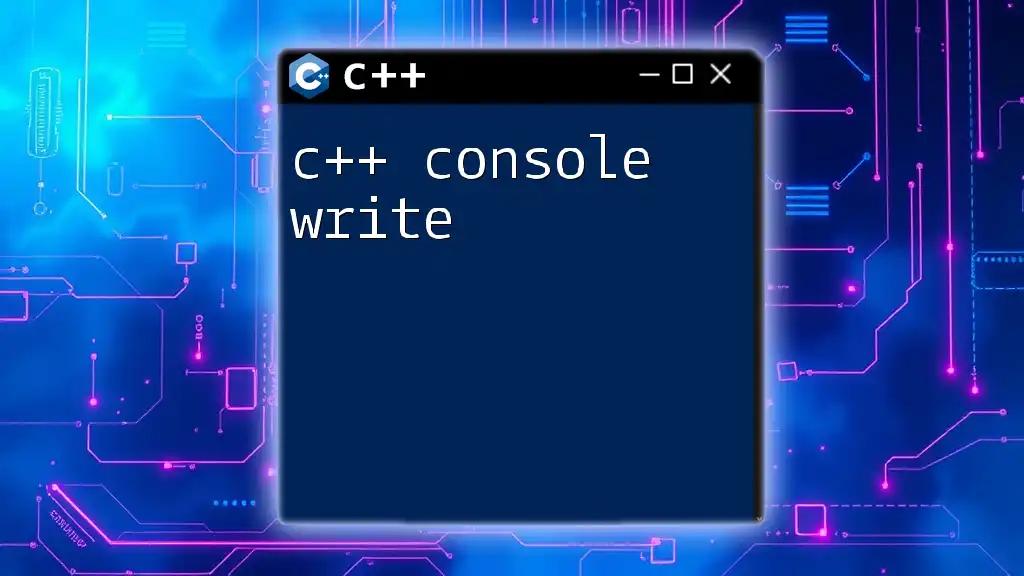
Using `std::cout` to Print to Console
Basic Syntax of `std::cout`
The cornerstone for printing to the console in C++ is the `std::cout` object, used in conjunction with the insertion operator `<<`. The following code snippet demonstrates its basic usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `std::cout` outputs the string "Hello, World!" to the console, followed by `std::endl`, which adds a newline character and flushes the output buffer.
Formatting Output with `std::cout`
For more precise control over output, C++ provides various manipulators that can enhance formatting. Consider the following example that demonstrates the use of `std::setprecision` and `std::fixed`:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << "Pi to 2 decimal places: " << std::setprecision(2) << std::fixed << pi << std::endl;
return 0;
}
In this snippet, `std::setprecision(2)` limits the decimal places displayed, and `std::fixed` ensures that the output is formatted in fixed-point notation. The result is a concise and controlled representation of floating-point data.
Printing Different Data Types
C++ allows for printing various data types using `std::cout`, including integers, floating-point numbers, strings, and characters. The following example illustrates this versatility:
#include <iostream>
int main() {
int age = 25;
double height = 5.9;
std::string name = "John";
std::cout << "Name: " << name << ", Age: " << age << ", Height: " << height << std::endl;
return 0;
}
Here, variables of different types are seamlessly combined into a single output statement, showcasing the power of `std::cout` in handling multiple data types.
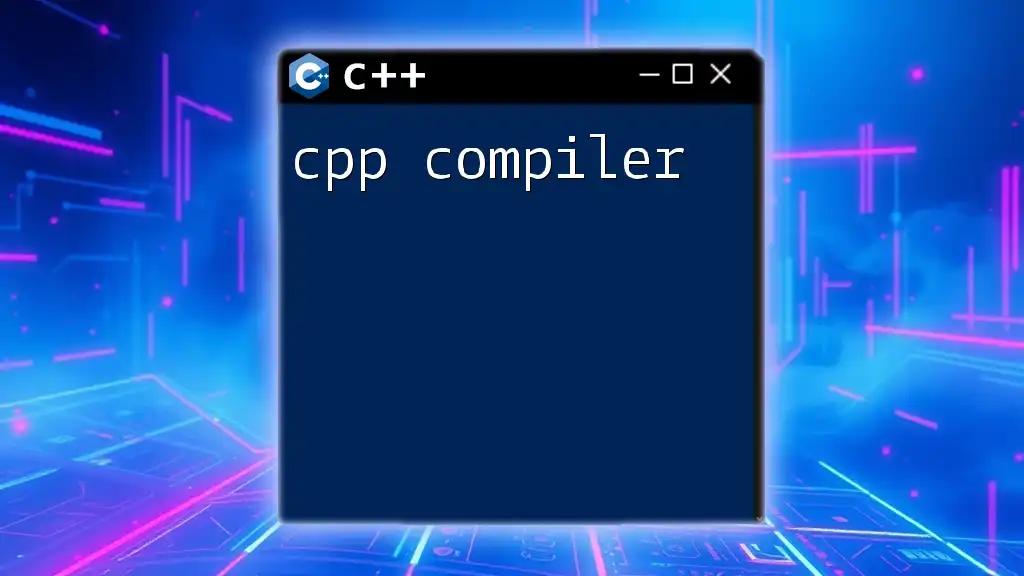
Advanced Console Printing Techniques
Combining Output with Operators
One of the elegant features of `std::cout` is the ability to chain multiple outputs using the `<<` operator. This not only reduces the number of output statements but also makes the code more readable. Here’s an example:
#include <iostream>
int main() {
int age = 25;
double height = 5.9;
std::string name = "John";
std::cout << "Name: " << name << "\nAge: " << age << "\nHeight: " << height << std::endl;
return 0;
}
Error Handling with Output Streams
It is important to differentiate between regular output and error messages in console logging. The `std::cerr` stream is designed for outputting error messages and operates similarly to `std::cout`. Here’s an example of using `std::cerr`:
#include <iostream>
int main() {
int age = -1;
if (age < 0) {
std::cerr << "Error: Age cannot be negative." << std::endl;
}
return 0;
}
Using `std::cerr` allows for instantaneous feedback for debugging issues while maintaining a clean separation from regular output.
Redirecting Output
C++ also enables output redirection, where you can send output to a file instead of displaying it on the console. This is handy for logging purposes. Below is a simple example of redirecting output to a file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("output.txt");
outFile << "This will be written to a file." << std::endl;
outFile.close();
return 0;
}
The above code snippet creates a file named output.txt and writes a message to it. The file can then be opened in a text editor to review the output.
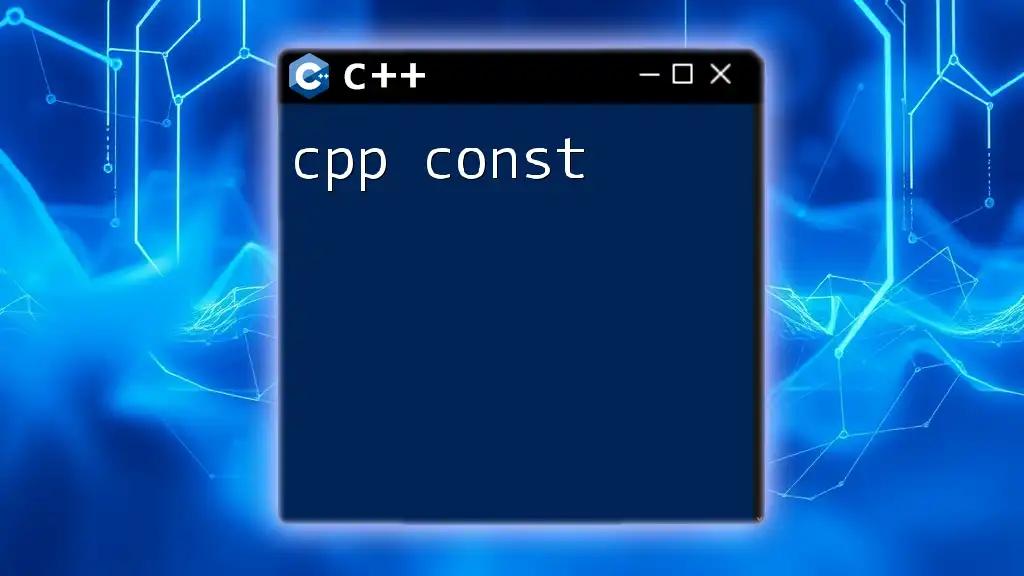
Debugging with Console Logging
Importance of Console Logs in Debugging
Console logging is an invaluable practice that aids developers when identifying bugs or understanding the flow of a program. By strategically placing log statements throughout the code, you can pinpoint where issues arise, leading to more efficient troubleshooting.
Common Use Cases and Scenarios
Effective console logging can include printing variable states at critical points in your code, which allows for better understanding and tracking. Here’s an example where a function logs its entry and exit:
void exampleFunction() {
std::cout << "Entering exampleFunction()" << std::endl;
// function logic ...
std::cout << "Exiting exampleFunction()" << std::endl;
}
By logging entry and exit points, you can monitor the execution flow and identify any discrepancies along the way.
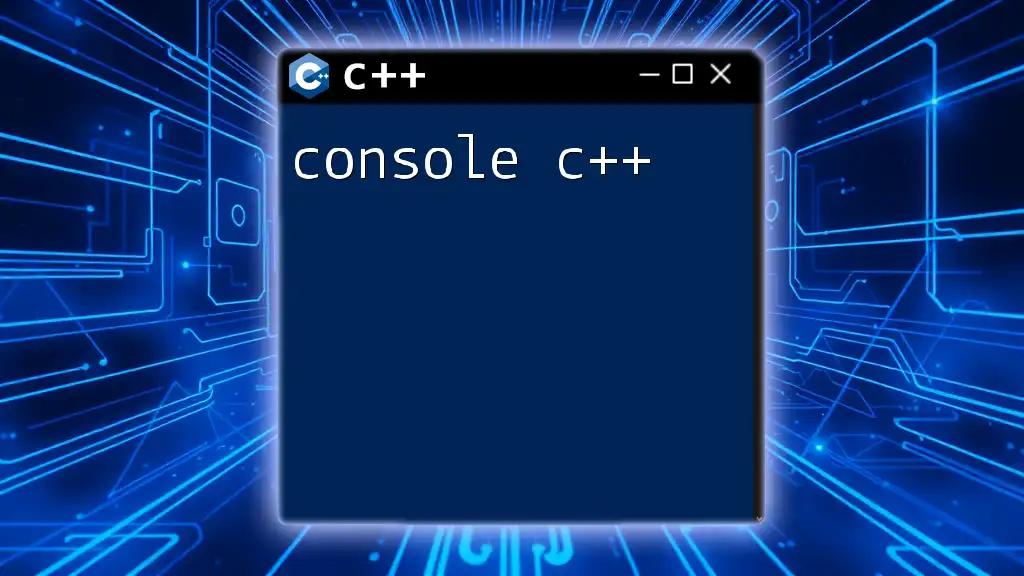
Conclusion
In this article, we have explored the essential tools and techniques for implementing console logging in C++. The ability to output data to the console using `std::cout`, format messages, handle errors with `std::cerr`, and even redirect outputs to files is foundational for developing robust C++ applications. Practice these techniques, and you will enhance your debugging capabilities, leading to improved program quality and performance.
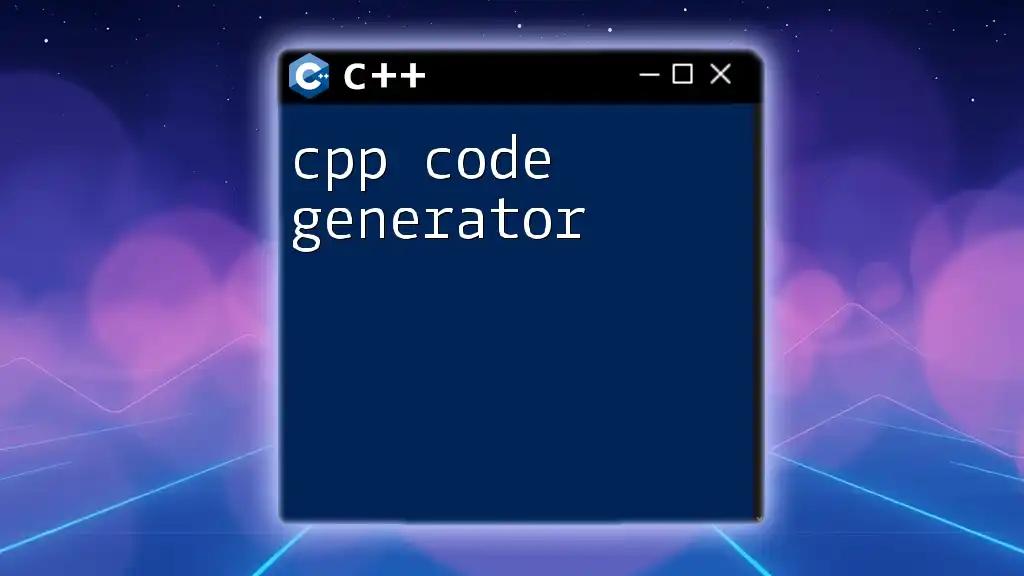
Additional Resources
Recommended Tools and Libraries
For advanced logging capabilities, consider exploring libraries such as spdlog or log4cpp, which provide additional features for managing console and file logging.
Next Steps
Engage in hands-on practice with the examples provided, and don’t hesitate to explore further resources to deepen your understanding of C++. Subscribe to newsletters or follow discussions in developer communities for ongoing learning opportunities.