To use mathematical functions in C++, you need to include the `<cmath>` header, which provides essential tools for performing calculations such as square roots and trigonometric functions.
Here’s a simple code snippet demonstrating how to include `<cmath>` and use the `sqrt` function:
#include <iostream>
#include <cmath>
int main() {
double number = 16.0;
double result = sqrt(number);
std::cout << "The square root of " << number << " is " << result << std::endl;
return 0;
}
What is `cmath`?
The `cmath` library is a standard C++ library that provides mathematical functions for performing basic and advanced calculations. It is essential for any C++ program that requires mathematical computations, as it offers a rich set of functionalities. Understanding the role of `cmath` is crucial for effective programming, especially for applications in scientific computing, engineering, and graphics.
One of the main reasons to use `cmath` is its compatibility with C++'s type system. While `<math.h>` is the C version of this library, `cmath` ensures that all function signatures are adapted for C++'s type safety features. This includes the handling of functions such as `abs`, `ceil`, `floor`, and others. Choosing to include cmath in C++ instead of `<math.h>` can help avoid subtle bugs and ensure better alignment with C++ principles.
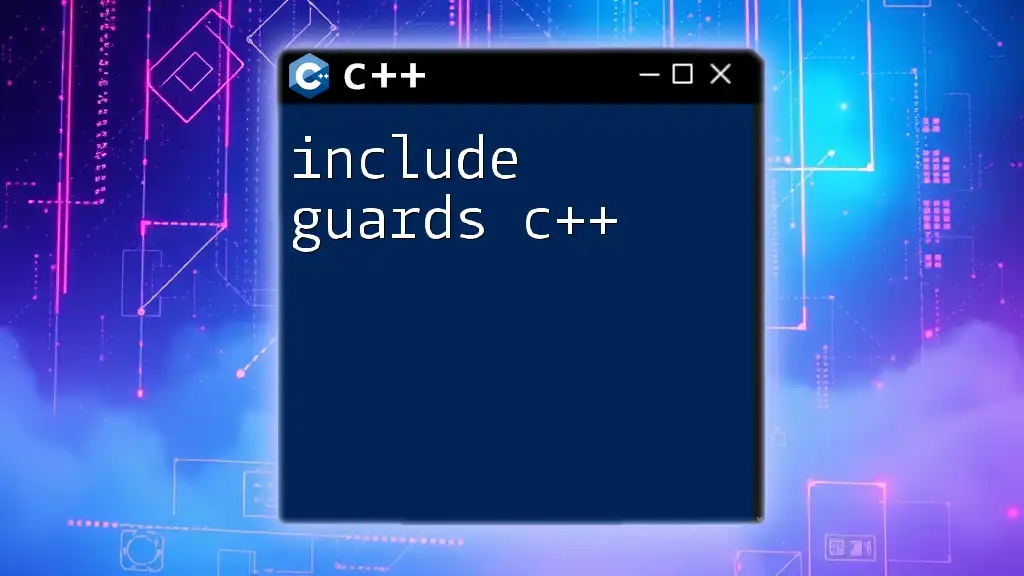
How to Include `cmath` in Your C++ Program
Basic Syntax
To start using the functionalities of the `cmath` library, you first need to include it in your C++ program. This is done using the `#include` directive. The syntax is straightforward:
#include <cmath>
Where to Place the Include Statement
It's common practice to place all your `#include` statements at the top of the file, right after any comments or documentation headers. This approach helps maintain clarity about the dependencies of your source code. Here's an example of proper placement:
#include <iostream>
#include <cmath>
int main() {
// Your code here
return 0;
}
Being consistent about your include placement helps improve code readability for yourself and others.
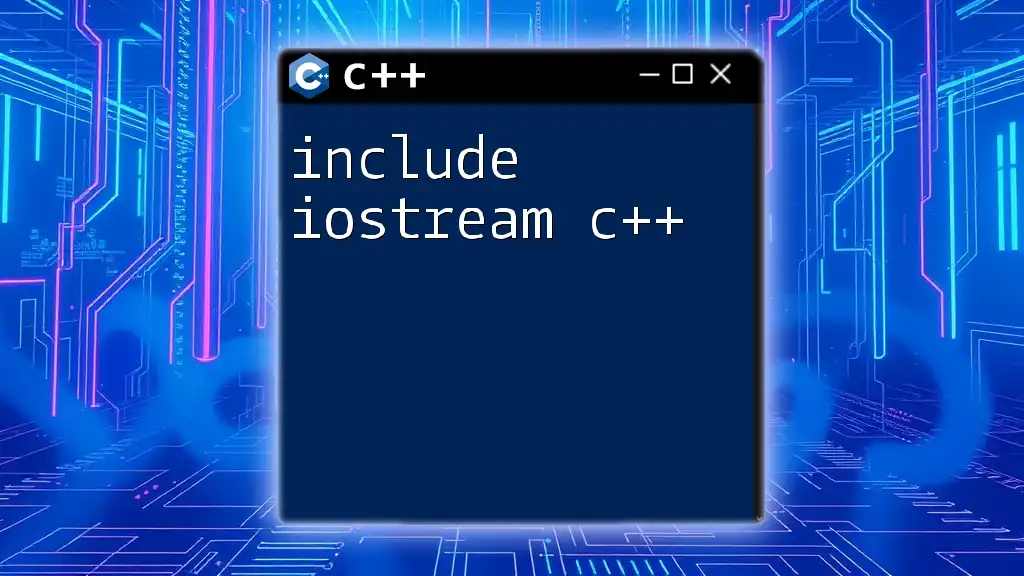
Key Functions in `cmath`
Mathematical Functions
The `cmath` library provides a plethora of functions that cover various aspects of mathematics. Here are some of the core categories of functions you will often use:
Trigonometric Functions
Trigonometric functions are essential when dealing with angles and periodic behavior in your applications. The major functions provided by `cmath` include `sin`, `cos`, `tan`, and their inverses such as `asin`, `acos`, `atan`.
For instance, to calculate the sine of a 90-degree angle:
double angle = 90.0;
double radians = angle * (M_PI / 180.0);
double sine = sin(radians);
In this code snippet, we first convert the angle from degrees to radians since trigonometric functions in `cmath` utilize radians.
Exponential and Logarithmic Functions
The library provides functions such as `exp`, `log`, and `log10` to help with exponential calculations and logarithmic conversions.
Consider this example demonstrating the calculation of the exponential and natural logarithm:
double value = 2.0;
double expValue = exp(value); // e^2
double logValue = log(value); // Natural log of 2
These functions are crucial for algorithms that require growth calculations, such as in finance, data science, or modeling phenomena.
Power and Rounding Functions
The library also includes power functions like `pow`, which allows you to raise numbers to specific powers, and rounding functions like `ceil`, `floor`, and `round`.
Here’s an example of using the power function:
double base = 3.0, exponent = 4.0;
double powerValue = pow(base, exponent); // 3 raised to the power of 4
Additionally, rounding functions are useful in situations where precision is required, such as graphical applications or iterative algorithms.
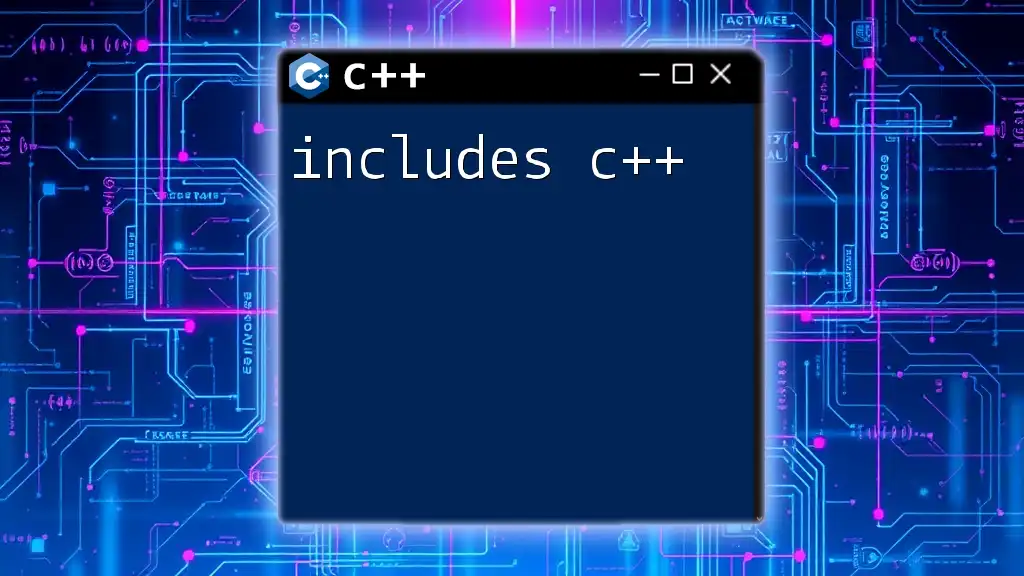
Performance Considerations
When to Use `cmath`
Understanding when to use `cmath` is important to optimize both performance and resource management in your applications. You should include cmath in C++ whenever you need to perform mathematical operations that are not inherently supported by basic operators. This is particularly true in data processing, simulations, or graphics operations.
Overhead and Efficiency
Although `cmath` provides a wide range of functionalities, it does come with a certain level of overhead. The actual performance implications depend on the specific nature of the calculations. In cases requiring extensive numerical methods—like in machine learning or complex simulations—using the optimized algorithms from numerical libraries can be preferable.
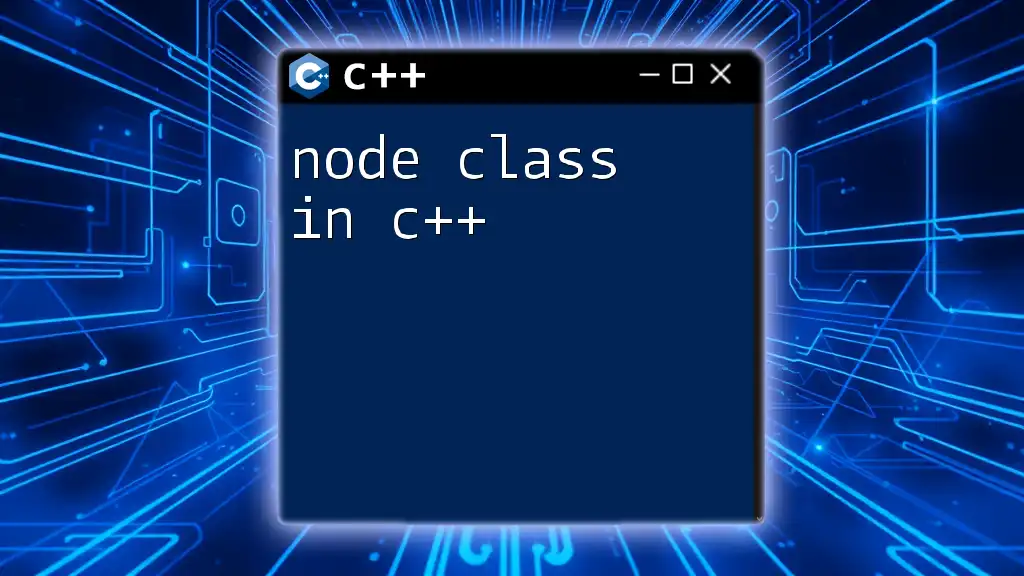
Common Errors and Debugging Tips
Common Pitfalls When Using `cmath`
One of the primary areas to be cautious about when using `cmath` is the domain of functions. For example, passing a negative number to the square root function will lead to a runtime error:
double result = sqrt(-1); // Error: domain error
This would typically result in a runtime exception, so proper error handling is necessary. Ensuring your values lie within defined domains is crucial for robust programming.
Debugging Techniques
If you encounter issues when using `cmath`, here are some helpful techniques:
- Check Compiler Warnings: Compilers typically issue warnings for misuse of functions, which can assist in pinpointing errors.
- Use Assertions: Add assertions before performing calculations to check for valid input ranges.
- Unit Tests: Implement unit testing to verify that your mathematical functions behave as expected, especially for edge cases.
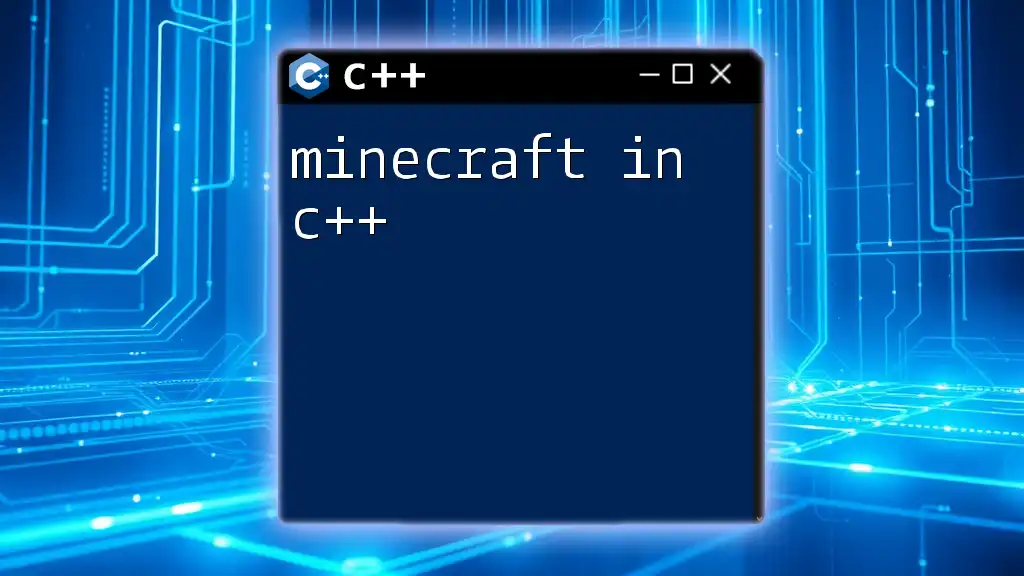
Conclusion
The `cmath` library is a powerful tool in your C++ programming toolkit, allowing for efficient mathematical computations through its diverse range of functions. By understanding how to include cmath in C++ and leveraging its functionalities, you can significantly enhance the capabilities of your applications.
As you dive deeper into C++ programming, exploring the extended features of `cmath` will undoubtedly empower you to tackle more complex problems in areas like scientific research, graphics programming, and data analysis. Don’t hesitate to experiment further and discover all that `cmath` has to offer!
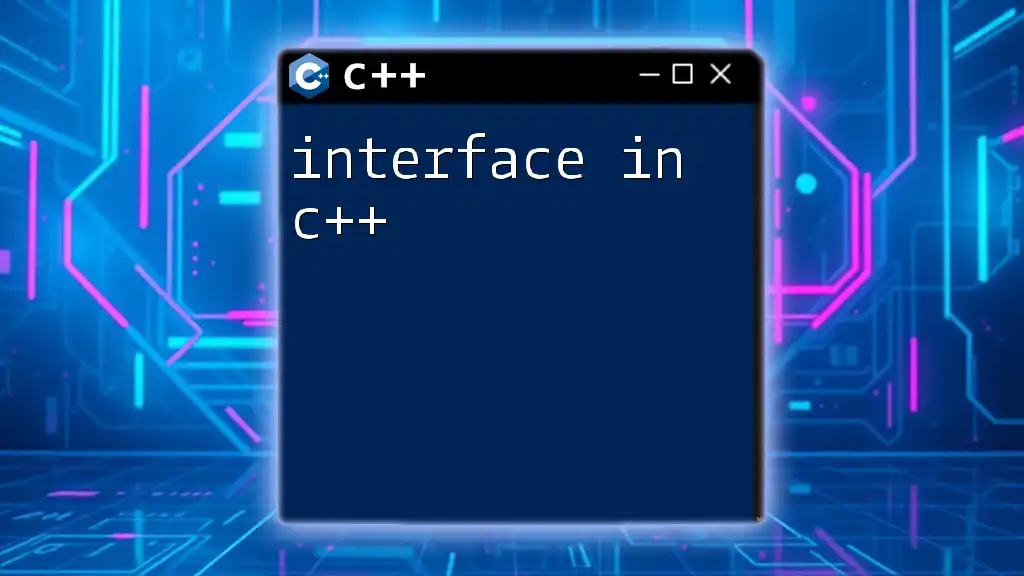
Additional Resources and References
For more in-depth learning, consider:
- Official C++ documentation on `cmath`
- Recommended textbooks and resources that cover advanced mathematical techniques and algorithms
- Community forums like Stack Overflow or GitHub for practical examples and discussions with fellow programmers.
With these tools at your disposal, you'll be well-equipped to tackle mathematical challenges in C++ programming. Happy coding!