Include guards in C++ are preprocessor directives used to prevent multiple inclusions of a header file, thereby avoiding potential redefinition errors.
Here’s a basic example of include guards in a C++ header file:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Your declarations here
#endif // MY_HEADER_H
What Are Include Guards?
Definition of Include Guards
Include guards in C++ are a programming construct used to prevent multiple inclusions of the same header file in a single translation unit. This is crucial because multiple inclusions can lead to redefinition errors, where the compiler encounters the same class, function, or variable definitions more than once, resulting in compilation failures. By leveraging include guards, developers can ensure that their header files are only included once during the compilation process.
Purpose of Include Guards
The primary purpose of include guards is to stave off redefinition errors that can arise from including the same header file multiple times. This situation can easily occur when several files include a common header. Additionally, include guards enhance compilation time efficiency, as the compiler will not need to reprocess the same header file if it has already been included. This leads to improvements in build speeds, especially in larger projects with many dependencies.
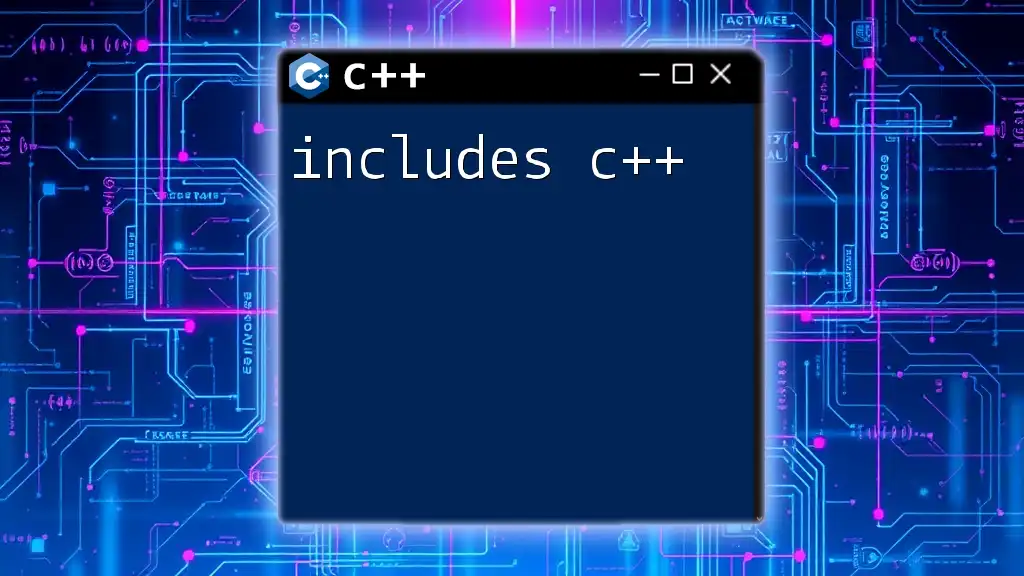
How Include Guards Work
The Mechanism of Include Guards
Include guards are implemented using preprocessor directives that work as conditional compilation checks. The common constructs used in include guards are:
- `#ifndef`: This directive checks whether a given macro (typically associated with the header file) has not been defined yet.
- `#define`: This directive defines the macro when it is found to be undefined.
- `#endif`: This directive marks the end of the conditional checks.
The combination of these directives ensures that the contents of a header file are only included if the macro has not been defined, effectively preventing multiple inclusions.
Example of Include Guards in Action
Consider a simple header file named `my_header.h`:
#ifndef MY_HEADER_H
#define MY_HEADER_H
class MyClass {
public:
void display();
};
#endif // MY_HEADER_H
In this example:
- The preprocessor first checks if `MY_HEADER_H` has been defined.
- If not, it defines `MY_HEADER_H` and includes the class definition.
- If `my_header.h` is included again, the preprocessor finds that `MY_HEADER_H` is already defined and skips the subsequent definitions, preventing any redefinition errors.
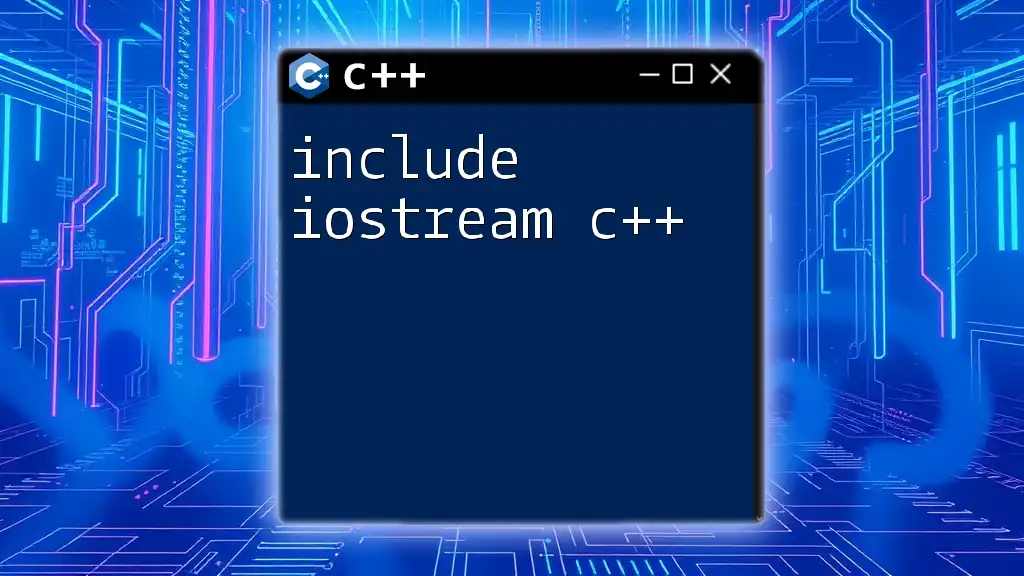
Best Practices for Using Include Guards
Consistent Naming Conventions
When defining include guards, it is essential to use unique macro names to avoid any conflicts with other header files. A popular convention is to use a combination of the project name and the file name, formatted in uppercase. For example, if your project is named "MyProject" and your file is "my_header.h," a recommended guard would be `MYPROJECT_MY_HEADER_H`. This practice minimizes the risk of naming conflicts across different libraries and modules.
Placement of Include Guards
Include guards should be placed at the very top of the header file, before any code that should be conditionally compiled. This ensures that all declarations and definitions are protected from multiple inclusions. Conversely, avoid placing guards inside namespaces or below declarations, as this could lead to unintended behavior.
Utilizing Include Guards with Other Structures
While include guards are the traditional way to prevent multiple inclusions, another method is `#pragma once`. This directive also prevents the compiler from including the same file multiple times but is compiler-specific and not part of the C++ standard. When working on cross-platform projects, it is often safer to rely on include guards for better portability.
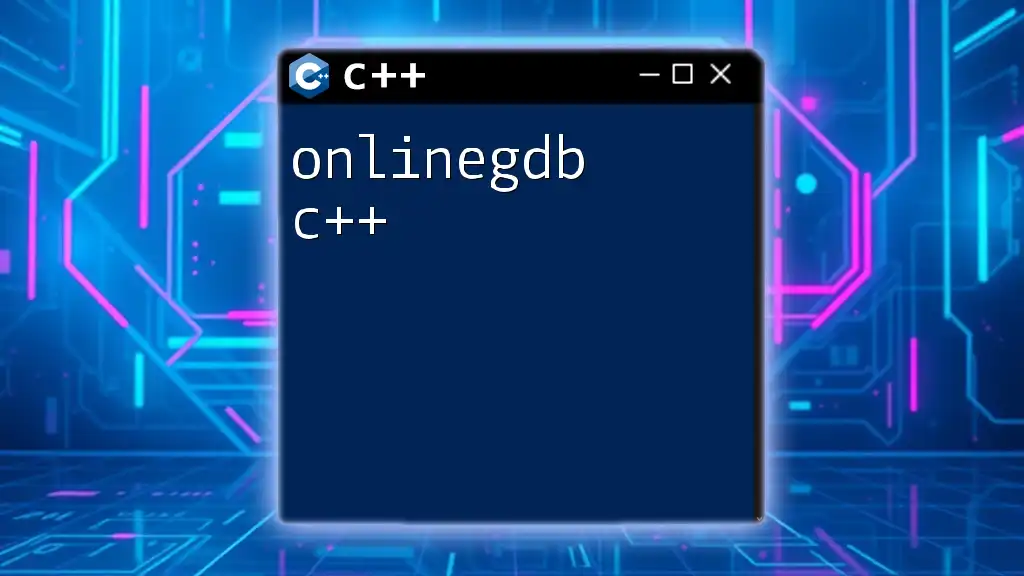
Common Issues with Include Guards
Redefinition Errors
Missing or incorrectly defined include guards can lead to redefinition errors. For instance, consider the following scenario:
// file1.h
class MyClass {};
// file2.h
#include "file1.h" // Correct usage
#include "file1.h" // Error: 'MyClass' redefined
In this case, including `file1.h` twice within a single translation unit will cause the compiler to attempt to redefine `MyClass`, resulting in a compilation error.
Nested Includes
Include guards also manage nested includes efficiently. If `file1.h` includes `file2.h`, and both have their own guards, everything behaves as expected. If `file2.h` is included multiple times through different paths, the guards will ensure it is only processed once.
Debugging Include Guard Problems
When facing issues with include guards, check for:
- Missing guards or erroneous macro checks.
- Ensure that macro names are unique and well-formed.
- Use compiler output to identify specific instances of redefinition errors, as they often provide clear pointers on where the issues lie.
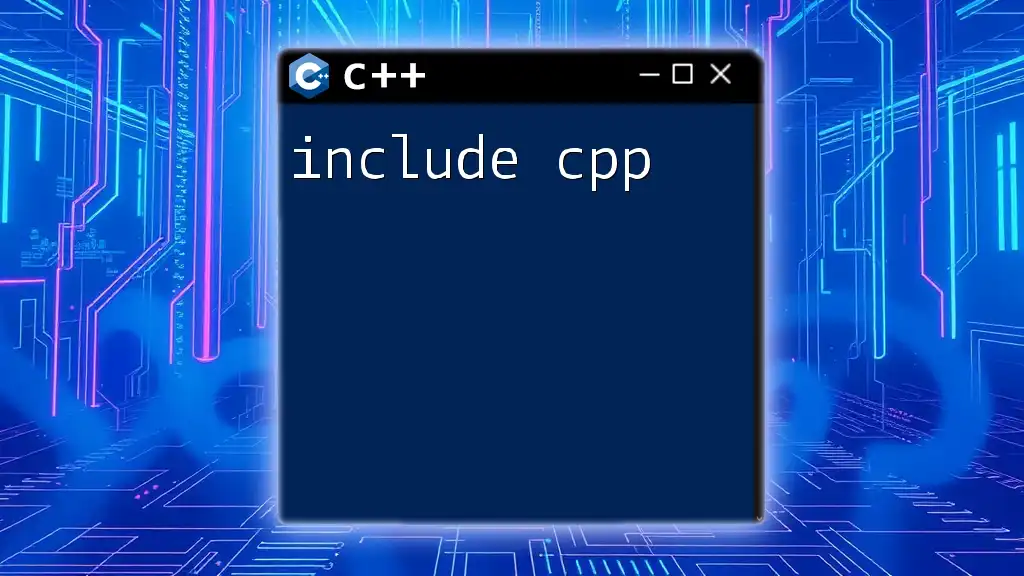
Testing Include Guards
Writing Test Cases
Testing header files with include guards is essential for verifying their functionality. A simple test could involve creating two separate source files that include the same header file and compiling them together. If no redefinition errors occur, the include guards are functioning correctly.
Using Compiler Output
Compiler messages can be invaluable for debugging include guard problems. When a redefinition error occurs, the line numbers in the output can lead you directly to the conflicting declarations. Understanding these messages is critical in resolving issues swiftly.
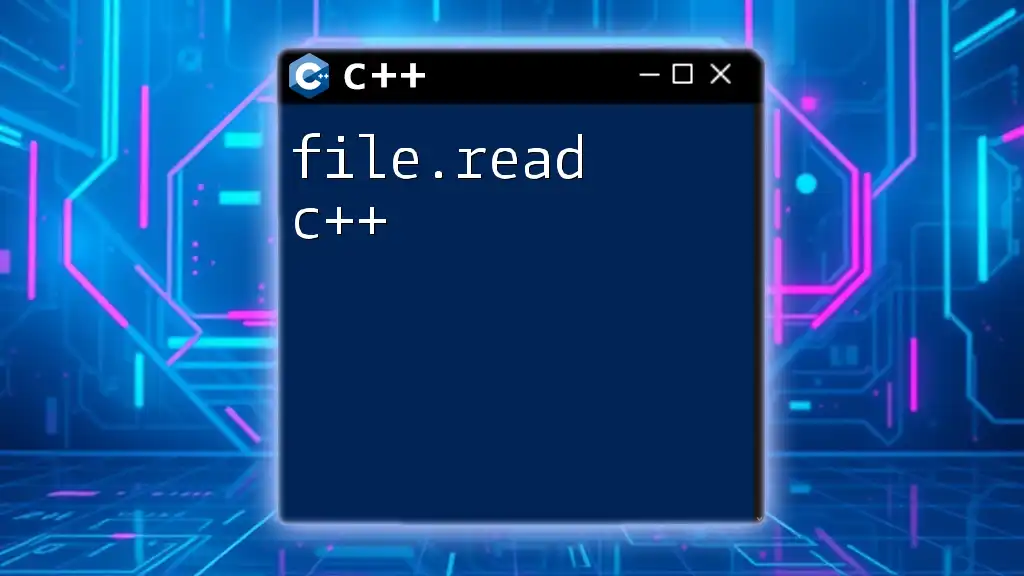
Conclusion
Include guards are an important aspect of C++ programming that helps prevent multiple inclusions of header files, saving time and avoiding potential compilation errors. By following best practices, such as using consistent naming conventions and correctly placing the directives, developers can create robust and efficient code. Implementing include guards is a practical skill that will enhance your C++ coding proficiency and contribute to cleaner, more maintainable projects.