A valid C++ statement must follow the syntactical rules of the language and typically involves a complete instruction, such as declaring a variable or executing a function. Here’s an example of a valid C++ statement:
int x = 10;
Understanding C++ Statements
Definition of a C++ Statement
In C++, a statement is a logical instruction that the compiler can execute. Statements are the building blocks of a C++ program, controlling the flow of execution. The key characteristics of a valid statement include:
- It must be well-structured and adhere to syntax rules.
- It must end with a semicolon (`;`), which tells the compiler that it has reached the end of the instruction.
Types of C++ Statements
C++ statements can be categorized into several types, each serving a unique purpose in programming.
Expression Statements
These include variable declarations, assignments, function calls, or any expression that performs an action.
Example:
int x = 5; // Valid expression statement
x++; // Valid: increments x
Compound Statements
Also known as blocks, compound statements group multiple statements into one, typically enclosed in curly braces `{}`.
Example:
if (x > 0) {
// Valid compound statement
x++;
}
Selection Statements
These statements allow for decision-making in code through constructs such as `if`, `else if`, and `switch`.
Example:
if (x > 0) { // Valid selection statement
// Do something
} else {
// Do something else
}
Iteration Statements
These statements repeat a block of code while a condition is true, or a specific number of times using loops like `for`, `while`, and `do-while`.
Example:
for (int i = 0; i < 10; ++i) { // Valid iteration statement
// Code to be executed
}
Jump Statements
Jump statements, such as `break`, `continue`, and `return`, alter the control flow of a program by jumping to a different part of the code.
Example:
return 0; // Valid jump statement in a function

The Importance of Syntax
Why Valid Syntax Matters
Understanding and using valid syntax is crucial for the following reasons:
- Impact on Code Execution: An improperly structured statement can lead the program to crash or produce unintended results.
- Common Errors: Errors due to invalid syntax can lead to frustrating debugging sessions. Tracking down these errors can consume a significant amount of development time.
- Debugging Challenges: Many programming environments highlight syntax errors, but they may not always catch logical errors stemming from incorrect syntax.
Common Syntax Rules in C++
There are a few essential syntax rules to adhere to in C++:
- Case Sensitivity: C++ is case-sensitive, meaning that `Variable` and `variable` are treated as different identifiers.
- Semicolon Termination: Every complete statement must end with a semicolon (`;`) to signify the end of an instruction.
- Parentheses and Brackets Use: Properly matching braces `{}` and parentheses `()` is vital for maintaining proper function blocks while performing operations and control flow.

Analyzing Statements: Validity Check
Factors that Determine Valid C++ Statements
To determine if a statement is valid, consider the following factors:
Data Types
Using the correct data types is essential for the validity of statements. For example:
int a = 5; // Valid
float b = 2.5; // Valid
int c = "Hello"; // Invalid
Keywords
Using C++ reserved words inappropriately can lead to invalid statements since keywords serve special functions in C++. Always ensure that keywords like `if`, `return`, and `int` are used correctly.
Operations
Operators must be used correctly within a valid statement. For example:
int d = a + b; // Valid
int e = a + ; // Invalid: Missing operand
Examples of Valid and Invalid Statements
To foster clarity, let’s explore some common examples, distinguishing between valid and invalid statements.
Example 1: Variable Declaration
int number = 10; // Valid
int number 10; // Invalid: Missing '='
Example 2: Function Definition
void myFunction() { // Valid
// function code
}
void myFunction() { // Invalid: Missing function code
}
Example 3: Conditional Statements
if (number > 5) { // Valid
// execute something
} number > 5 { // Invalid: Wrong syntax
}

Common Mistakes and Misconceptions
Misunderstandings About C++ Syntax
Common misconceptions that can lead to invalid statements include:
-
Confusing Semicolons with Commas: While semicolons (`;`) denote the end of a statement, commas (`,`) are used in lists such as in function calls.
-
Using Incorrect Data Types: Many beginners mistakenly mix types, such as trying to assign a string to an integer variable.
-
Omitting Parentheses in Control Flow Statements: Leaving out the required parentheses in conditional statements leads to errors in program logic.
Tips to Avoid Common Errors
To avoid common pitfalls:
- Consistent Formatting: Adhering to a regular and consistent code style significantly enhances readability and helps detect errors.
- Using Comments: Adding comments to code snippets assists in understanding their purpose and eases the debugging process.
- Using an IDE: Leveraging an Integrated Development Environment (IDE) can provide real-time syntax checking, highlighting errors before compilation.
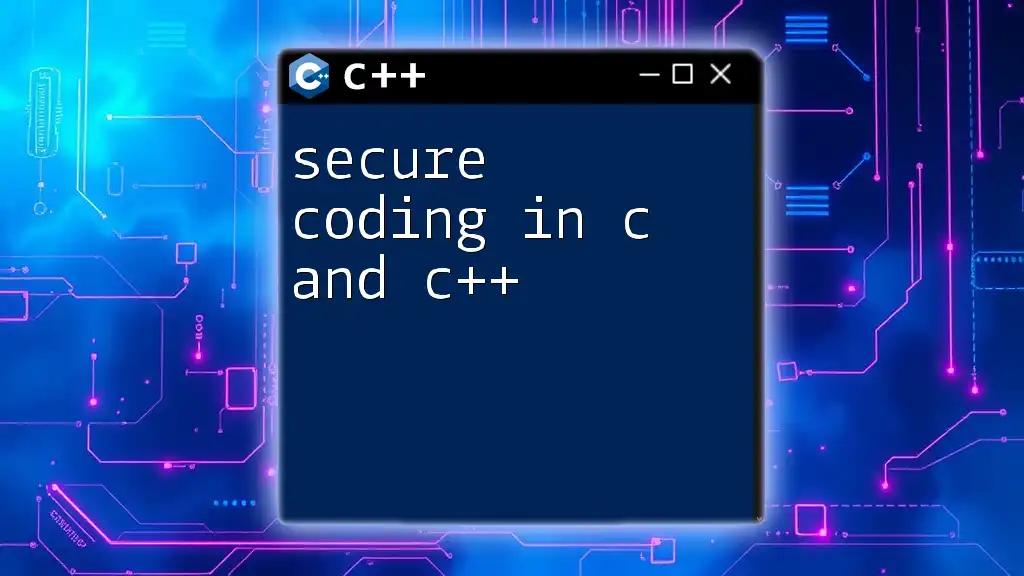
Practical Exercise: Identify Valid Statements
Exercise Instructions
To apply what you’ve learned, review the following statements, identifying which are valid and why they may or may not be correct.
- `int validVar = 1;`
- `float invalidVar = 1.0 // Missing semicolon`
- `if (true) { cout << "Hello"; }`
- `println("Hello World!"); // Invalid: 'println' is not a valid C++ function`
Answer Key and Explanations
-
`int validVar = 1;`: Valid because it correctly declares an integer variable and terminates with a semicolon.
-
`float invalidVar = 1.0`: Invalid due to the lack of a semicolon at the end.
-
`if (true) { cout << "Hello"; }`: Valid since it properly follows the conditional structure and ends with a semicolon in the cout command.
-
`println("Hello World!");`: Invalid because `println` is not a recognized function in C++. The correct function should be `cout << "Hello World!";`.

Conclusion
Understanding which of the following is a valid C++ statement is crucial for any programmer, from beginners to experienced developers. By recognizing the types of statements, adhering to syntax rules, and analyzing common mistakes, you can ensure that your C++ programming is efficient and error-free. Continuous practice and engagement with C++ will further solidify your grasp on valid syntax and enhance your programming skills.

Additional Resources
To enhance your knowledge further, consider exploring additional resources:
- Comprehensive C++ programming books
- Online tutorials and courses
- C++ documentation from credible sources (like cppreference.com)
- Community forums and support groups aiding in C++ learning
Embrace the journey and continue exploring the fascinating world of C++ programming!