To find a value in a vector in C++, you can use the `std::find` algorithm from the `<algorithm>` header.
Here’s a code snippet demonstrating how to do this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int value_to_find = 3;
auto it = std::find(vec.begin(), vec.end(), value_to_find);
if (it != vec.end()) {
std::cout << "Value found at index: " << std::distance(vec.begin(), it) << std::endl;
} else {
std::cout << "Value not found" << std::endl;
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector is a part of the Standard Template Library (STL) in C++ that represents a dynamic array. Unlike traditional arrays, which have fixed sizes, vectors can grow or shrink in size as needed, making them a flexible choice for managing collections of data.
Vectors manage memory automatically, meaning you don't need to worry about allocating or deallocating memory blocks manually.
Basic Operations on Vectors
Vectors support several essential operations, such as:
- Creation: You can create a vector using syntax like `std::vector<int> myVector;`.
- Adding Elements: To add an element to a vector, use the `push_back()` function, which appends the new element to the end of the vector.
- Removing Elements: You can remove the last element using `pop_back()`, which reduces the vector's size by one.
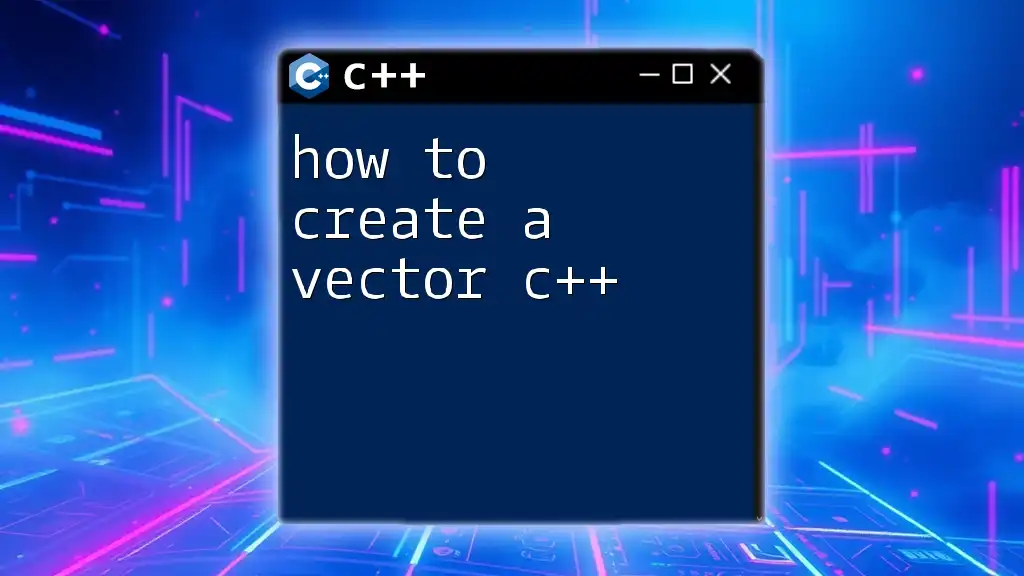
Finding a Value in a Vector
Why Use Vectors?
Vectors are particularly powerful for searching because they offer dynamic sizing and built-in methods that simplify common tasks, such as finding values. Using vectors instead of arrays allows more efficient memory usage and reduces codice complexity.
How to Search in a Vector: An Overview
When looking for a value in a vector, there are primarily two methods you can use: linear search and the `std::find` function. Each method has its own use cases and advantages.
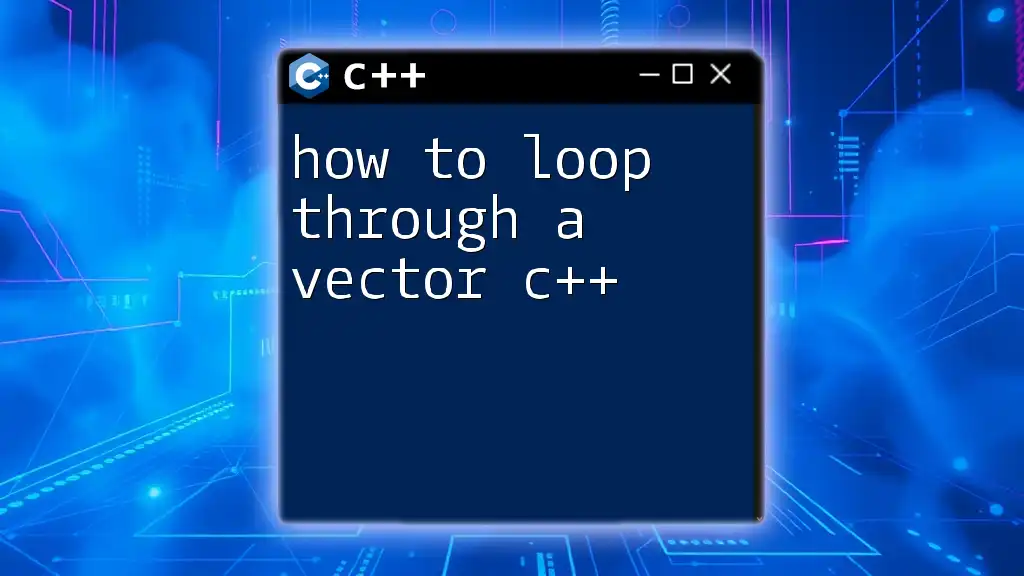
Linear Search in a Vector
What is Linear Search?
Linear search is the simplest search algorithm; it involves iterating over each element in the vector until the desired value is found or all elements have been checked. This method has a time complexity of O(n), which may not be efficient for large datasets.
Example of Linear Search
Here’s a basic example of how to implement linear search in C++:
#include <iostream>
#include <vector>
bool linearSearch(const std::vector<int>& vec, int target) {
for (size_t i = 0; i < vec.size(); i++) {
if (vec[i] == target) {
return true; // Found the target
}
}
return false; // Target not found
}
In this code, we define a function linearSearch that takes a vector and a target value as parameters. The function iterates through the vector, checking each element against the target. If a match is found, it returns true; otherwise, it returns false after checking all elements.
When to Use Linear Search
Linear search is best used for small datasets or when the vector is unordered. However, for larger datasets, it is less efficient due to its linear time complexity.
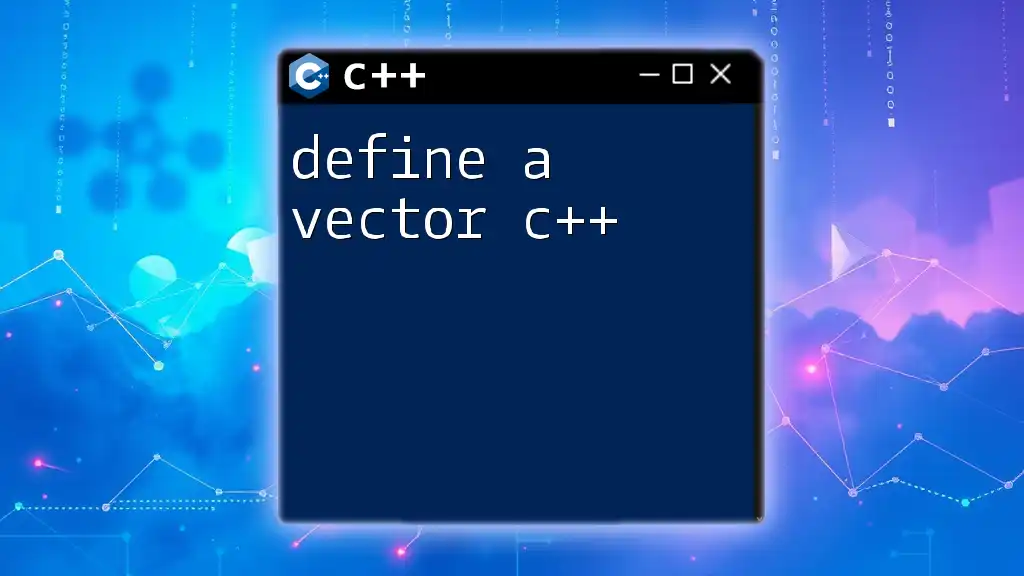
Using the `std::find` Function
What is `std::find`?
The `std::find` function is part of the C++ Standard Library and is found in the `<algorithm>` header. It allows you to search for a value in a vector efficiently, simplifying the code and improving readability.
Example of Using `std::find`
Here’s how to use `std::find`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
In this example, we call `std::find`, passing the beginning and end of the vector and the target value to search. If the element is found, an iterator pointing to that element is returned; if not, it points to `vec.end()`.
Advantages of Using `std::find`
Using `std::find` eliminates the need for manual iteration and comparison, reducing code complexity and potential errors. It is also part of the STL, which means it's optimized for performance compared to a custom search implementation.
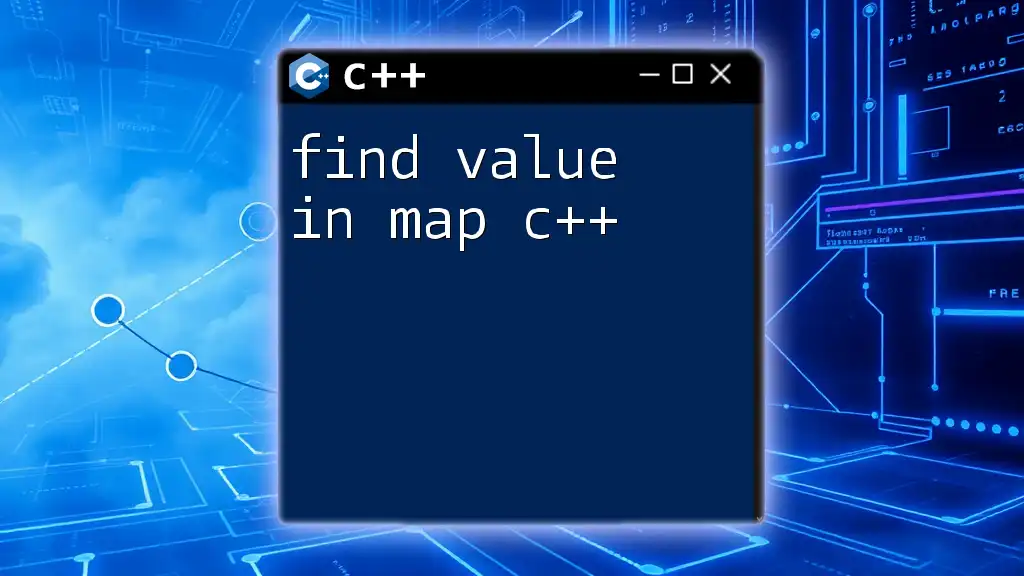
Searching for an Element and Edge Cases
Finding Elements with Custom Conditions
Sometimes you might need to search for elements based on specific criteria instead of a direct match. You can achieve this using lambda functions.
Example with Lambda Function
Here’s how to search using a lambda function:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
auto it = std::find_if(vec.begin(), vec.end(), [](int value) { return value > 25; });
if (it != vec.end()) {
std::cout << "Found first element greater than 25: " << *it << std::endl;
}
return 0;
}
In this case, `std::find_if` allows for more complex conditions by using a lambda function that specifies criteria—in this case, finding the first element greater than 25.
Handling Unsuitable Inputs
Always be cautious about edge cases when dealing with vectors, such as empty vectors, which will return `vec.end()` upon searching. Implement checks to avoid unnecessary searches on empty datasets, ensuring a smooth user experience.
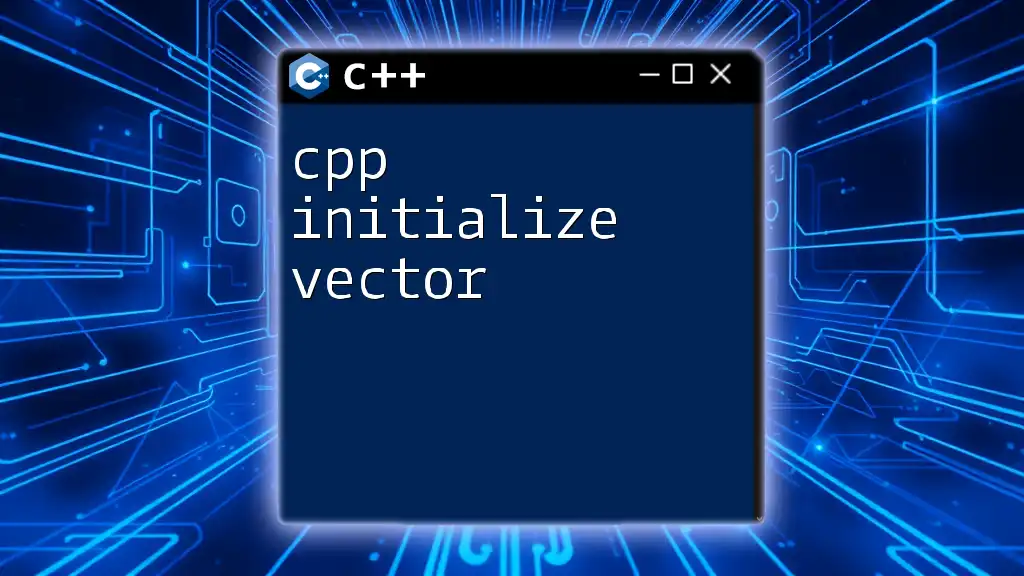
Additional Sorting and Searching Techniques
Binary Search in Sorted Vectors
Binary search offers another powerful way to find a value in a vector, but it has a crucial requirement: the vector must be sorted. Binary search operates in O(log n) time complexity, making it much more efficient for large collections of data.
Example of Binary Search with `std::binary_search`
Here's how to perform binary search using `std::binary_search`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
bool found = std::binary_search(vec.begin(), vec.end(), 3);
std::cout << "Element found: " << (found ? "Yes" : "No") << std::endl;
return 0;
}
In this example, `std::binary_search` determines whether the specified value exists in the vector. Note that this call assumes the vector is sorted.
Summary of Searching Techniques
- Linear Search: Direct iteration; efficient for small or unordered datasets.
- `std::find`: Simplifies searching with automatic iteration.
- `std::find_if`: Offers flexibility for custom conditions.
- Binary Search: Highly efficient but requires sorted vectors.
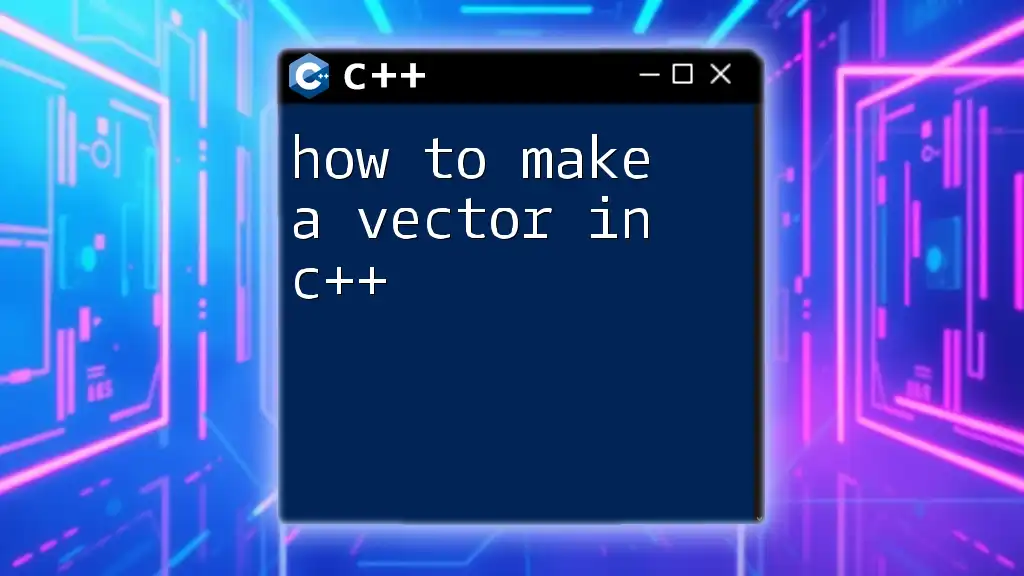
Conclusion
Knowing how to find a value in a vector in C++ is essential for efficient data management and usage of STL features. Through linear search, `std::find`, and lambda functions, developers can optimize queries and enhance performance.
Feel free to practice these techniques and explore how they can be applied in real-world applications. Engage with the content, ask questions, and discuss your experiences in the comments!
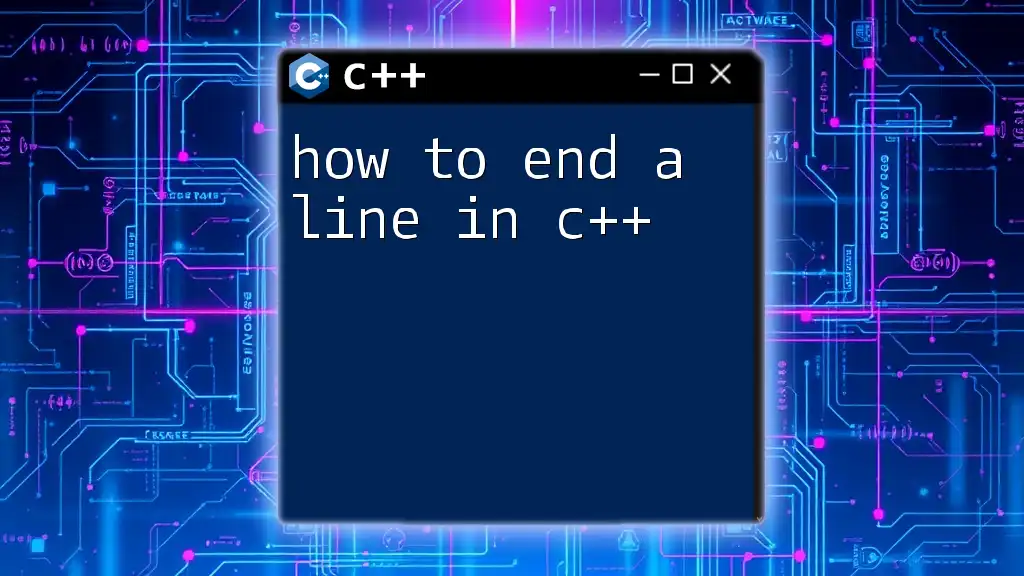
Additional Resources
For further reading on C++ vectors and algorithms, check out books and online courses that delve deeper into the nuances of C++ programming. The STL documentation is also an excellent resource for exploring more functions and capabilities.