To find a value in a `std::map` in C++, you can use the `find()` method or the subscript operator `[]` to access the value associated with a specific key.
Here’s an example using both methods:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "apple"}, {2, "banana"}, {3, "cherry"}};
// Using find() method
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Value found using find: " << it->second << std::endl;
} else {
std::cout << "Key not found." << std::endl;
}
// Using subscript operator
std::cout << "Value found using subscript: " << myMap[2] << std::endl;
return 0;
}
Understanding C++ Maps
What is a Map in C++?
In C++, a map is a container that stores elements in key-value pairs. Each key is unique within a map, and it allows for the efficient retrieval of the associated value using the key. Maps are especially useful when you need to look up values based on unique identifiers rather than relying on indices like arrays or vectors.
Types of Maps in C++
C++ provides two primary types of maps:
-
Standard Map (`std::map`): This type of map stores its elements in a sorted order based on the keys. It uses a balanced tree structure (typically a Red-Black Tree) to maintain this order, allowing for logarithmic time complexity for insertion, deletion, and accessing elements.
-
Unordered Map (`std::unordered_map`): Unlike `std::map`, this type does not keep its elements sorted. Instead, it uses a hash table to allow for average constant time complexity when retrieving elements. However, the order of keys is not defined.
Key Features of Maps
-
Automatic sorting: With `std::map`, elements are automatically sorted based on the keys, making it easier to perform range queries.
-
Fast retrieval: `std::unordered_map` offers faster access to values, especially when the number of entries is large and you do not require sorted order.
-
Real-world applications: Maps are widely used in applications like database indexing, counting occurrences of elements, and implementing associative arrays.
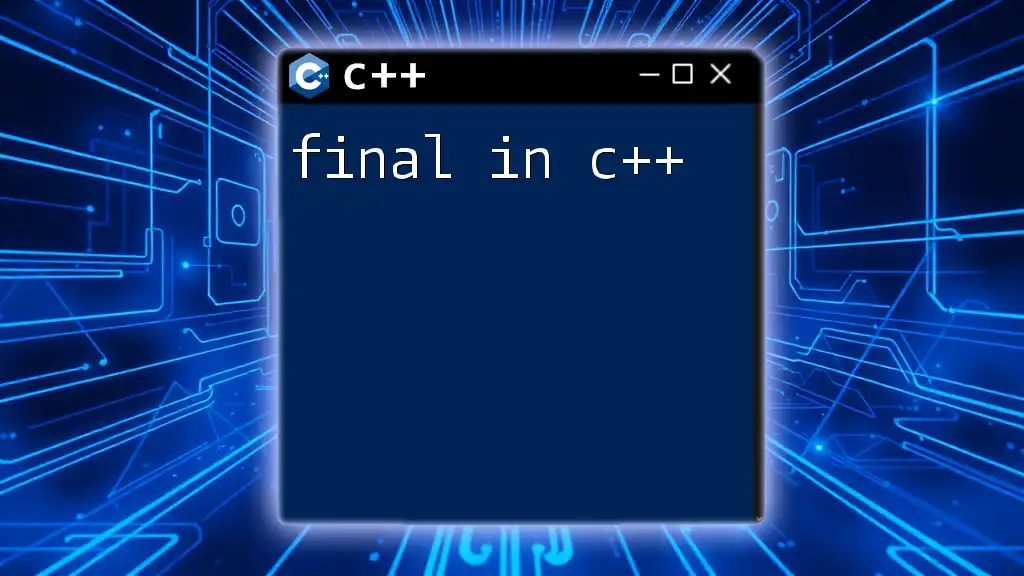
How to Use the Find Function in C++ Maps
What is the Find Function?
The `find` function is a member of the map container that allows you to search for a specific key. If the key exists, it returns an iterator pointing to the corresponding key-value pair. If the key does not exist, it returns an iterator equal to the end of the map, indicating that the search was unsuccessful.
Syntax of the Find Function
The basic syntax of the find function is as follows:
iterator find(const KeyType& key);
- KeyType: Represents the type of the keys stored in the map.
- iterator: An iterator that points to the found element, or `end()` if the key is not found.
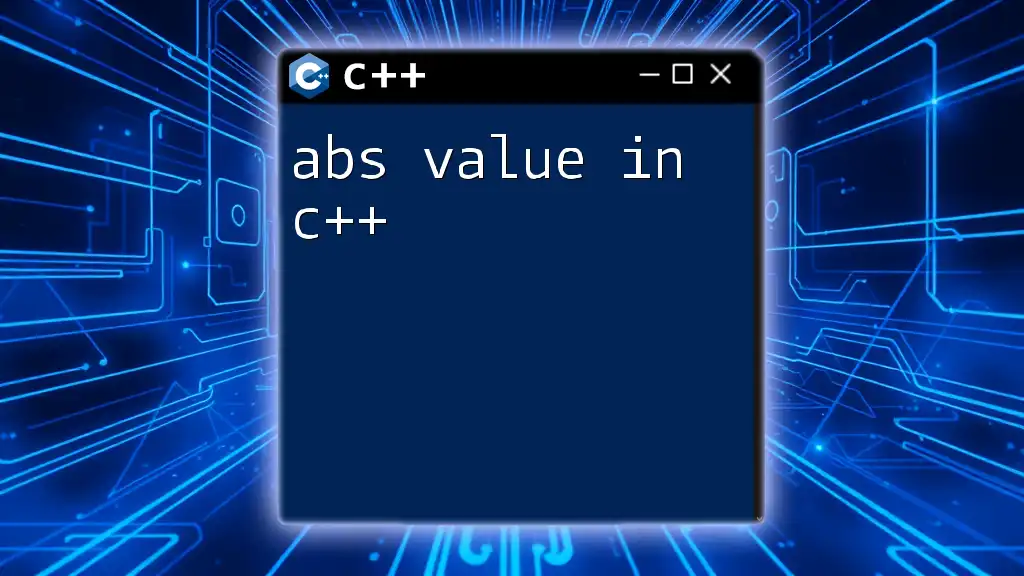
Examples of Finding Values in C++ Maps
Basic Example with `std::map`
Here’s a simple example showing how to use `find` with a `std::map`:
#include <iostream>
#include <map>
using namespace std;
int main() {
map<int, string> myMap;
myMap[1] = "Apple";
myMap[2] = "Banana";
auto it = myMap.find(1);
if (it != myMap.end()) {
cout << "Found: " << it->second << endl;
} else {
cout << "Key not found!" << endl;
}
return 0;
}
In this code, we initialize a map with integer keys and string values. We use the `find` function to look for the key `1`. The check `if (it != myMap.end())` ensures that the key was found before accessing the associated value with `it->second`. If the key were absent, we would see a message stating that the key is not found.
Using `std::unordered_map`
Finding a value using an `std::unordered_map` operates similarly but can offer better performance for large datasets:
#include <iostream>
#include <unordered_map>
using namespace std;
int main() {
unordered_map<string, int> myMap;
myMap["apple"] = 5;
myMap["banana"] = 3;
auto it = myMap.find("apple");
if (it != myMap.end()) {
cout << "Found: " << it->second << endl;
} else {
cout << "Key not found!" << endl;
}
return 0;
}
In this case, we utilize a map of strings to integers. The process remains the same, as we check for the presence of the key "apple" and retrieve its value if found.
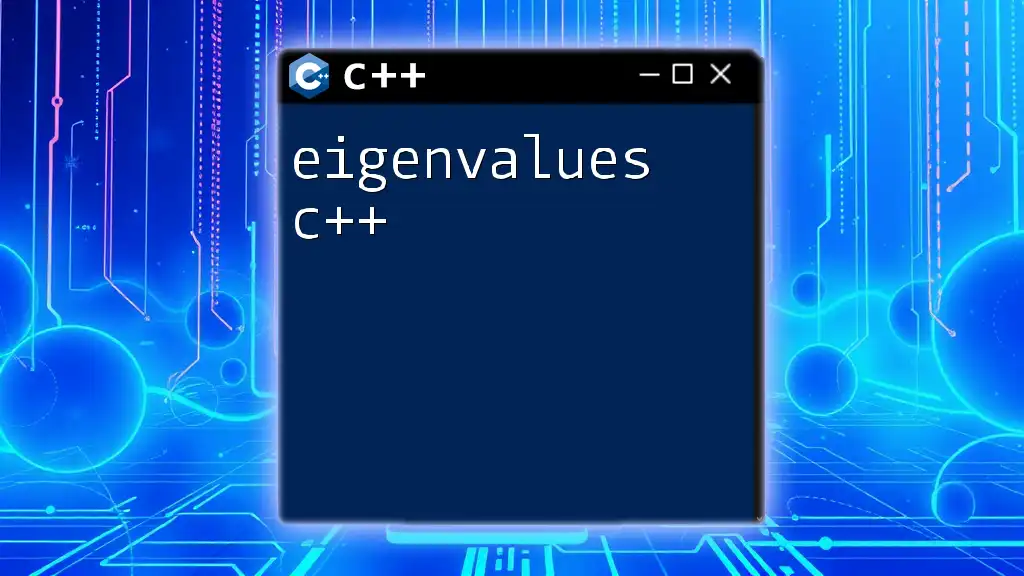
Handling Cases When Key is Not Found
Checking if Key Exists
When using the `find` function, it is critical to handle cases where the key might not exist in the map. The check `if (myMap.find(key) == myMap.end())` allows you to determine whether the key is present. Here's how:
if (myMap.find(key) == myMap.end()) {
cout << "Key does not exist!" << endl;
}
This conditional check is crucial for avoiding access to non-existent elements, which would lead to undefined behavior.
Alternative Methods to Check for Keys
Another method to check if a key is present is to use the `count` function. This function returns the number of elements with a specified key. Since keys in a map are unique, it will return either `0` (key does not exist) or `1` (key exists):
if (myMap.count(key)) {
cout << "Key exists!" << endl;
}
Using `count` can sometimes be more intuitive, especially for readers who may not be familiar with iterators and the `find` function's behavior.
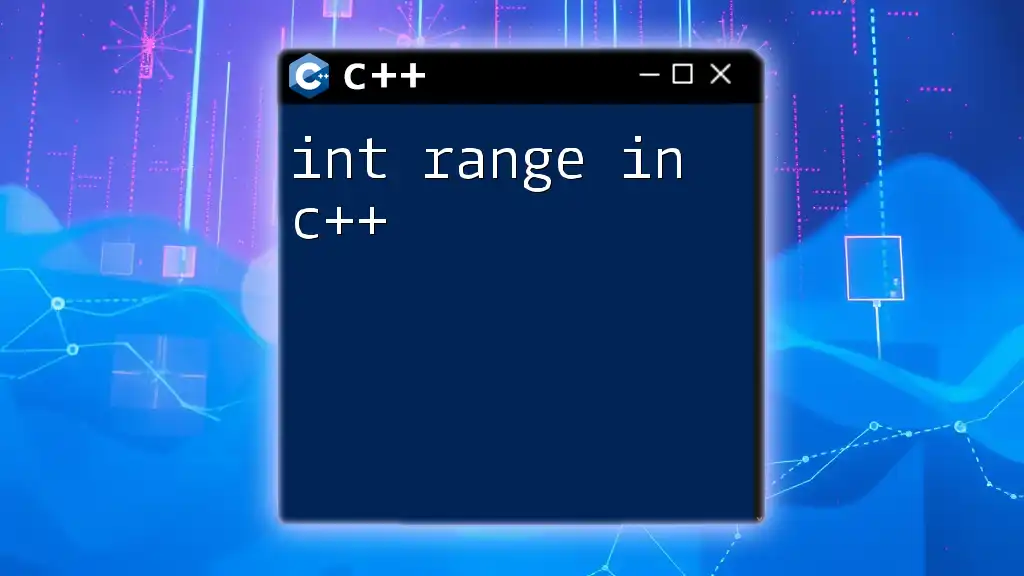
Performance Considerations
Time Complexity of Find Operations
The time complexity for the find operation varies between the two types of maps:
- For `std::map`, the average and worst-case complexities are O(log n) due to the nature of the underlying balanced tree structure.
- For `std::unordered_map`, the average case is O(1), allowing for very quick lookups, but this can degrade to O(n) in the worst case if there are many hash collisions.
When to Use Which Map Type
Choosing between `std::map` and `std::unordered_map` depends on your specific use case:
- Use `std::map` when you need to maintain the order of elements or when performing range queries.
- Use `std::unordered_map` when you require faster retrieval times and the order of keys is not important.
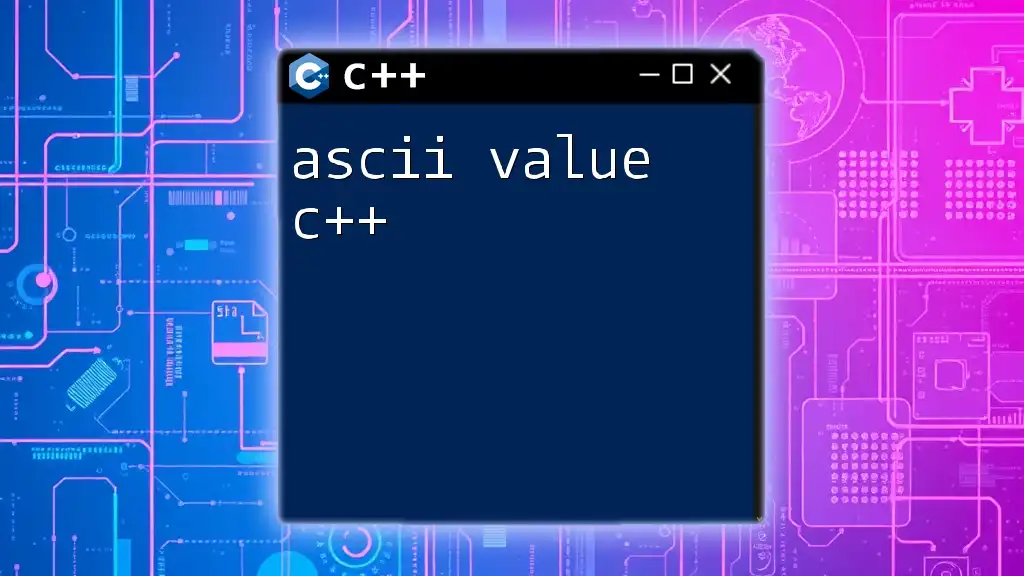
Conclusion
In this article, we've explored how to find values in map C++ using the `find` function. By understanding the basic structure and operation of maps, you can effectively implement them into your coding practices. Remember to handle cases where a key may not be present and choose the appropriate type of map to suit your needs. Experimenting and practicing with these concepts will deepen your understanding and boost your proficiency in C++ programming.