Creating a game engine in C++ involves designing a framework that manages graphics, physics, and input while leveraging libraries like SDL or SFML for rendering; here's a simple example of initializing an SDL window:
#include <SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("My Game Engine", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 800, 600, 0);
SDL_Delay(3000); // Keep window open for 3 seconds
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
Understanding Game Engine Architecture
Definition of Game Engine Components
A game engine is a powerful framework that enables developers to create games without building everything from scratch. The core elements of a game engine typically include:
- Rendering: Responsible for displaying graphics and visual elements.
- Physics: Manages real-world simulations, including movement and collision detection.
- Input Handling: Captures user interactions through keyboard and mouse.
- Audio: Manages sound effects and background music.
- Scripting: Allows designers to create game mechanics without altering the core engine code.
These components work together to bring a game to life, providing essential functionalities that streamline the development process.
Game Loop
The game loop is the heartbeat of a game engine. It continuously updates the game state and renders the visuals, ensuring a fluid gaming experience. The structure of a typical game loop includes:
- Process Input: Checks for user inputs each frame.
- Update: Applies game logic: physics updates, game object states, etc.
- Render: Draws the updated game state onto the screen.
Here’s the basic structure of a game loop in C++:
while (isRunning) {
processInput();
update();
render();
}
This loop will run indefinitely until the game is exited, continually refreshing to provide a real-time experience for the player.
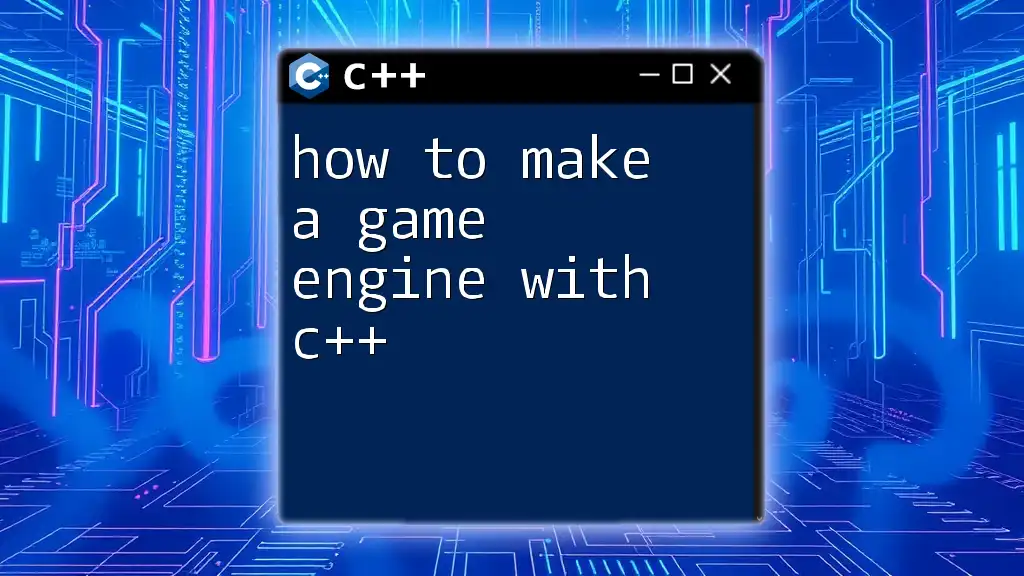
Setting Up Your C++ Environment
Required Software
To build a game engine in C++, you will need certain essential software:
- IDE (Integrated Development Environment): Recommended choices include [Visual Studio](https://visualstudio.microsoft.com/) or [Code::Blocks](http://www.codeblocks.org/).
- Graphics Libraries: Popular options are SDL (Simple DirectMedia Layer) or SFML (Simple and Fast Multimedia Library).
Installation of these tools is straightforward, and you can follow the official documentation provided by each library's website.
Creating a New C++ Project
Once your software is set up, create a new C++ project in your IDE. Follow these steps:
- Open your IDE and select “Create New Project.”
- Choose a C++ application template.
- Name your project (e.g., "MyGameEngine").
- Make sure to properly link necessary libraries (SDL/SFML) in the project settings.
Now you have the foundation for your game engine.
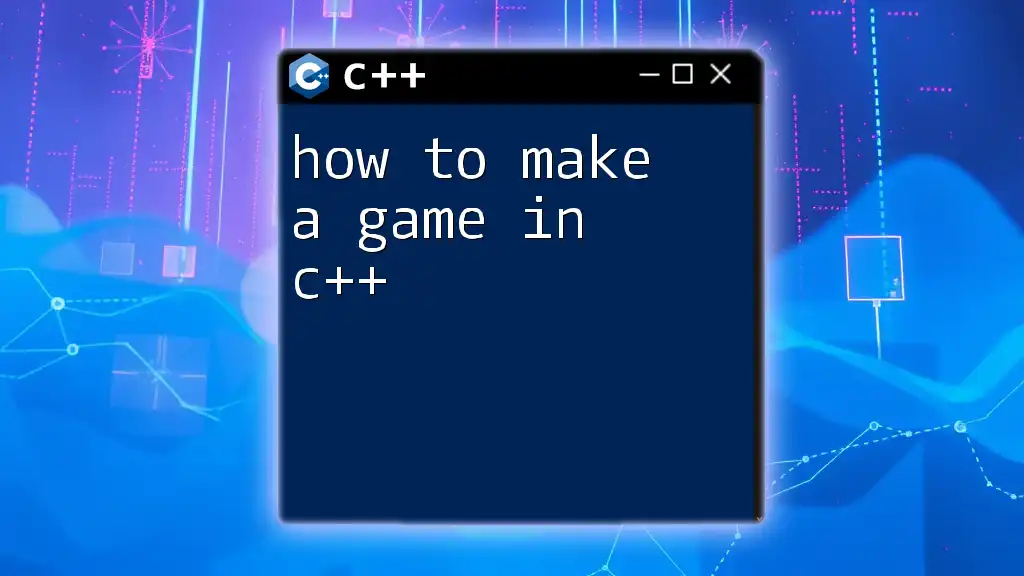
Core Components of a Game Engine
Creating a Window
A critical component of any game engine is the creation of a window that serves as the display area for your game graphics.
Using SDL/SFML to Create a Window
Below is an example of how to create a window using SDL:
#include <SDL.h>
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("Game Engine", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 800, 600, SDL_WINDOW_SHOWN);
This code initializes SDL for video components and creates a window of dimensions 800x600 pixels.
Rendering
Rendering is the process of drawing graphics onto the screen. This is how visual elements—like characters, environments, and effects—become visible to the player.
Basic Rendering with SDL/SFML
You can render a simple rectangle in SDL with the following code snippet:
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED);
SDL_SetRenderDrawColor(renderer, 255, 0, 0, 255);
SDL_RenderClear(renderer);
SDL_Rect rect = { 100, 100, 200, 200 };
SDL_RenderFillRect(renderer, &rect);
SDL_RenderPresent(renderer);
In this code, we initialize a renderer, set the draw color to red, clear the screen, and render a filled rectangle.
Input Handling
Handling user input is essential for engaging gameplay. You need to manage keyboard and mouse inputs to create interactive experiences.
Implementing Input Handling
Here’s a code snippet that demonstrates basic input handling using SDL:
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
isRunning = false;
}
if (event.type == SDL_KEYDOWN) {
// Handle key press logic
if (event.key.keysym.sym == SDLK_ESCAPE) {
isRunning = false; // Exit on escape key press
}
}
}
This example checks for the quit event and processes key presses, allowing players to interact with your game.
Physics Engine
A simple physics engine is essential for simulating realistic movements and collisions between game objects.
Basic Physics Implementation
To implement basic physics, you may use vector mathematics to update object positions. Here’s a simple example:
Vector2D position(0, 0);
Vector2D velocity(1, 1);
float deltaTime = 0.016f; // Simulating ~60 frames per second
position.x += velocity.x * deltaTime;
position.y += velocity.y * deltaTime;
In this code snippet, the position of an object is updated according to its velocity and time elapsed, ensuring smooth movement.
Audio Management
Sound adds another layer of immersion to games, enhancing player experience.
Audio Setup with SDL_mixer
To handle sound, you can integrate SDL_mixer. The following code snippet demonstrates how to initialize and play sounds:
Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048);
Mix_Chunk* sound = Mix_LoadWAV("sound.wav");
Mix_PlayChannel(-1, sound, 0);
This example initializes the audio subsystem, loads a sound effect from a file, and plays it immediately.
Scripting Capabilities
Integrating a scripting system within your engine allows designers to define behaviors without modifying the engine's core code. Popular scripting languages like Lua can be effectively utilized for this purpose.
You can design interfaces through which game designers can define levels, character stats, or even entire game mechanics by writing scripts that the engine interprets.
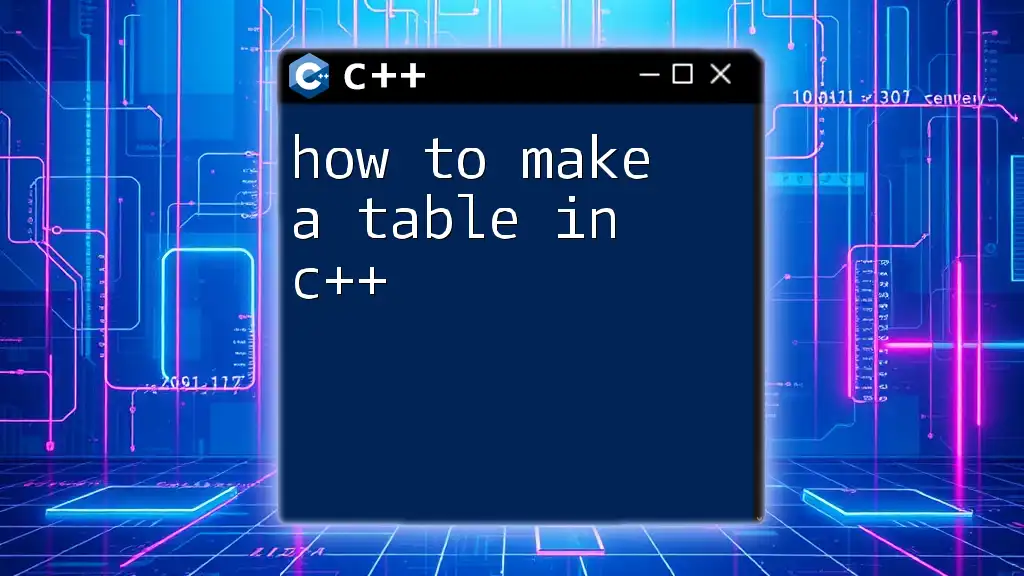
Example Game: Creating a Simple Game with Your Engine
Concept Overview
For this article, we will create a very basic game—a simple platformer or a Pong clone. The mechanics will serve as a practical demonstration of the components we've set up in the engine.
Implementing the Game
You'll need to manage Game States effectively, such as Main Menu, Play, and Game Over. Use states to control transitions within your basic game.
Game objects must be handled dynamically. You can define a simple `GameObject` class to represent these objects:
class GameObject {
public:
Vector2D position;
Vector2D velocity;
void update(float deltaTime) {
position.x += velocity.x * deltaTime;
position.y += velocity.y * deltaTime;
}
void render(SDL_Renderer* renderer) {
// Render logic here
}
};
Finalizing Your Engine
After implementing the core components, focus on the optimization techniques that enhance performance. Consider memory management, efficient rendering techniques, and reducing CPU load. Implementing techniques like object pooling can significantly improve performance in games with numerous entities.
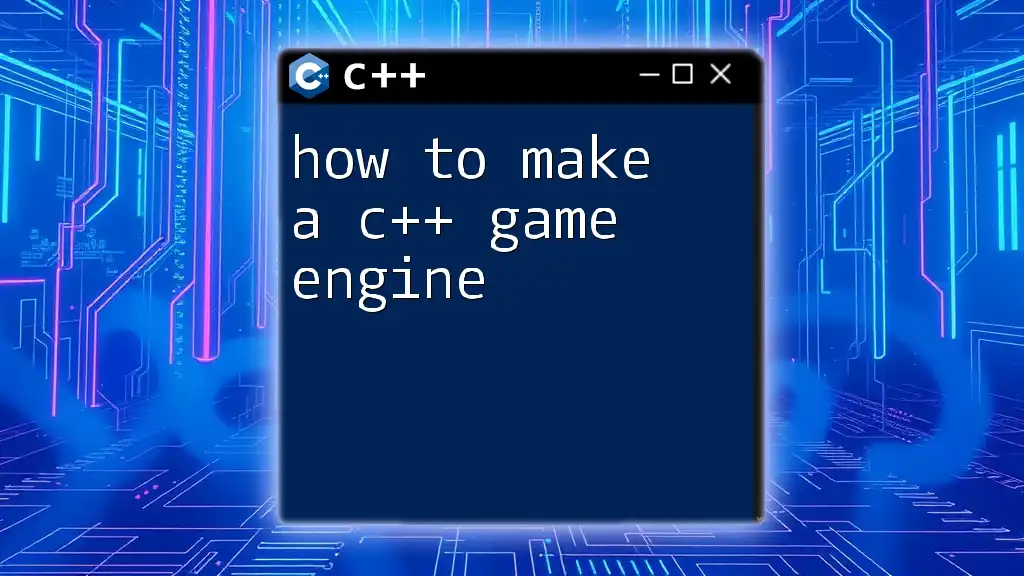
Conclusion
In this guide, we explored how to make a game engine in C++ by covering the essential components, from creating a window to rendering graphics, handling input, incorporating sound, and even adding physics. Each of these elements plays a crucial role in game development, allowing developers to create engaging experiences.
Continuing beyond this basic framework, you can explore more advanced concepts such as integrating AI, optimizing performance, and creating more complex rendering systems. Resources for learning and further exploration abound, providing avenues for you to deepen your knowledge.
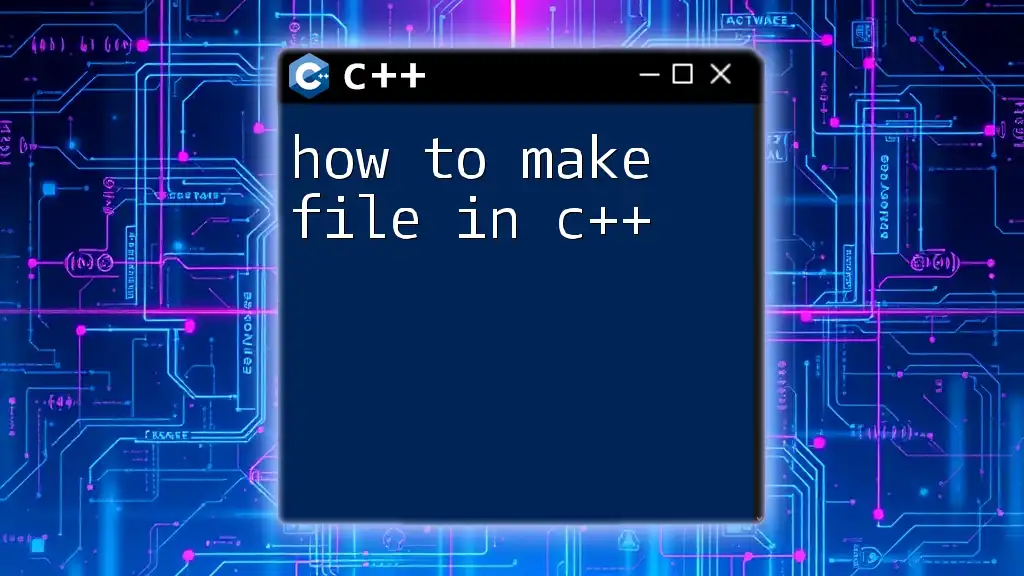
Additional Resources
To further your understanding, consider checking out various online resources, books, and communities dedicated to game development and C++. Websites like Stack Overflow, GamerGods, and GameDev.net offer invaluable advice and insight.
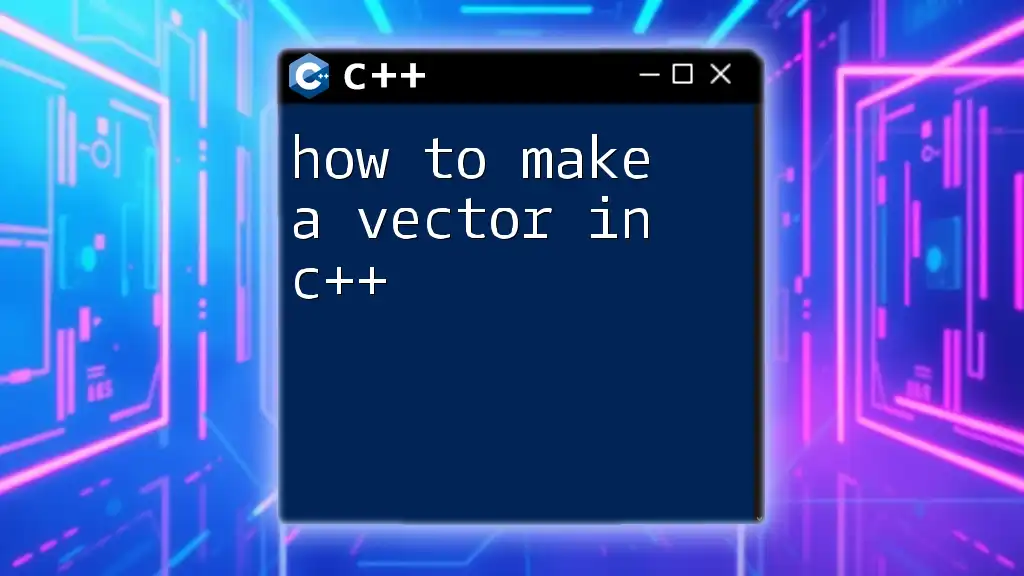
Call to Action
We invite you to share your experiences as you explore the world of game engine development. What games have you created using your initial engine? Join our community for updates and more concise tutorials on C++. Happy coding!