To create a basic calculator in C++, you can use the following code snippet which allows the user to perform addition, subtraction, multiplication, and division based on their input:
#include <iostream>
using namespace std;
int main() {
char op;
float num1, num2;
cout << "Enter operator (+, -, *, /): ";
cin >> op;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
switch (op) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
if(num2 != 0)
cout << num1 / num2;
else
cout << "Cannot divide by zero!";
break;
default:
cout << "Invalid operator";
}
return 0;
}
Understanding the Basics of C++
What is C++?
C++ is a powerful, high-performance programming language that supports various programming paradigms such as procedural, object-oriented, and generic programming. It has become a preferred language for many applications, including software development, game programming, and system applications due to its efficiency and flexibility.
Basic Constructs in C++
To effectively learn how to make a calculator in C++, it’s crucial to understand its basic constructs:
Variables: Variables are containers for storing data values. In C++, you must first declare the variable before using it.
Data Types: C++ supports several data types, including:
- `int`: for integers,
- `float`: for floating-point numbers,
- `double`: for double-precision floating-point numbers,
- `char`: for characters.
Operators: C++ uses operators to perform operations on variables and values. Familiarize yourself with arithmetic operators such as `+`, `-`, `*`, and `/` which will be vital in your calculator application.
A simple code snippet to illustrate variables and operators is shown below:
int a = 5;
int b = 10;
int sum = a + b;
Control Structures
Understanding control structures helps manage the flow of your calculator. The key constructs here are if-else statements and loops that allow conditional execution and repetitions.
For instance:
if (b != 0) {
double result = a / b; // Division only if b is not zero
} else {
cout << "Cannot divide by zero.";
}
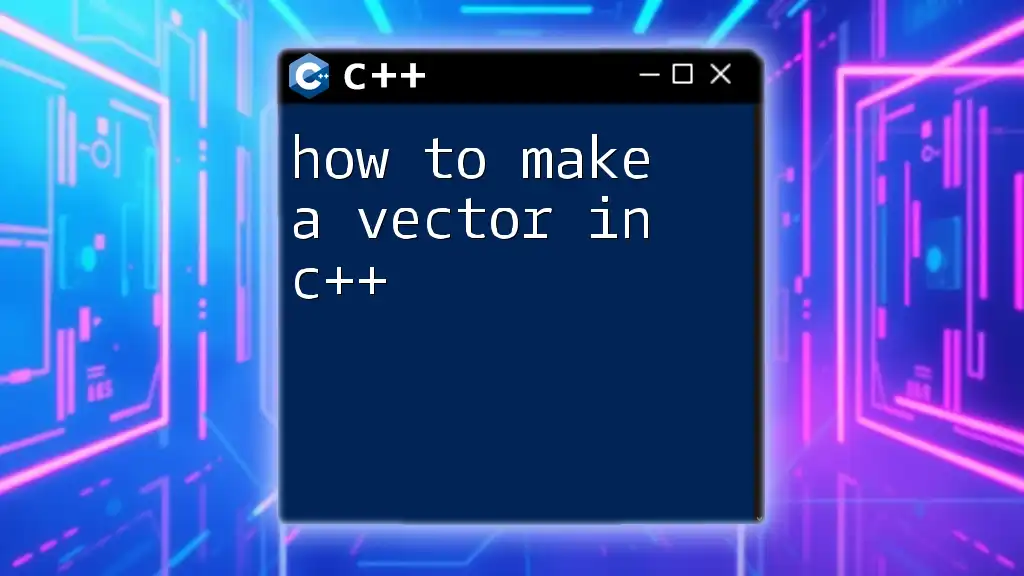
Setting Up Your Development Environment
Choosing an IDE or Text Editor
Before diving into coding, you need to choose an appropriate Integrated Development Environment (IDE) or a text editor. Popular C++ IDEs include Code::Blocks, Eclipse, and Microsoft Visual Studio, which provide user-friendly environments for writing and debugging your code.
Installing a Compiler
You’ll also need a C++ compiler to convert your written code into an executable program. The GNU GCC, MSVC, and Clang are well-known compilers. Ensure you select one that aligns well with your operating system and IDE.
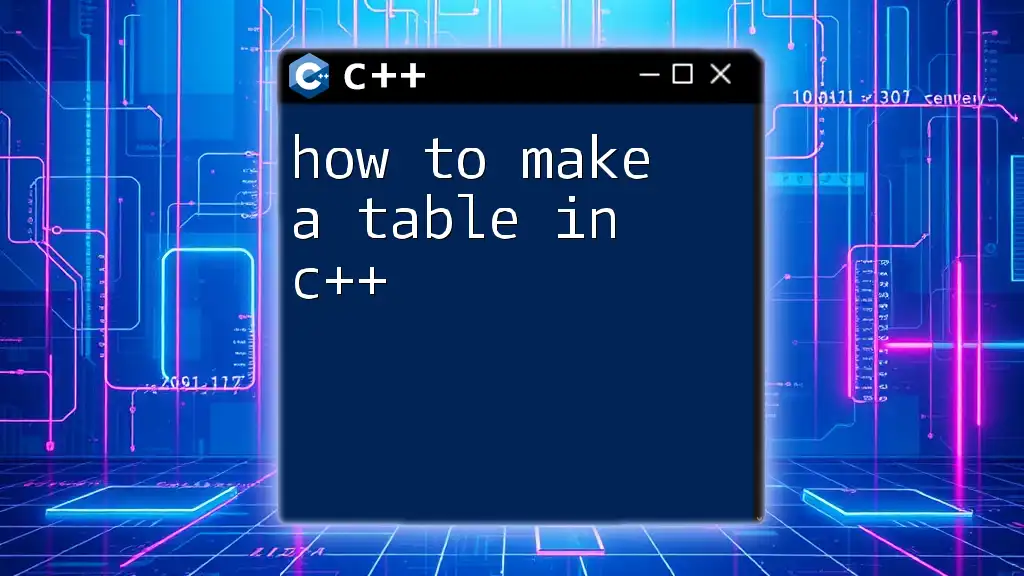
Designing the C++ Calculator
Defining the Requirements
Before coding, clarify the functionalities you want your calculator to perform. These can include basic arithmetic operations such as:
- Addition
- Subtraction
- Multiplication
- Division
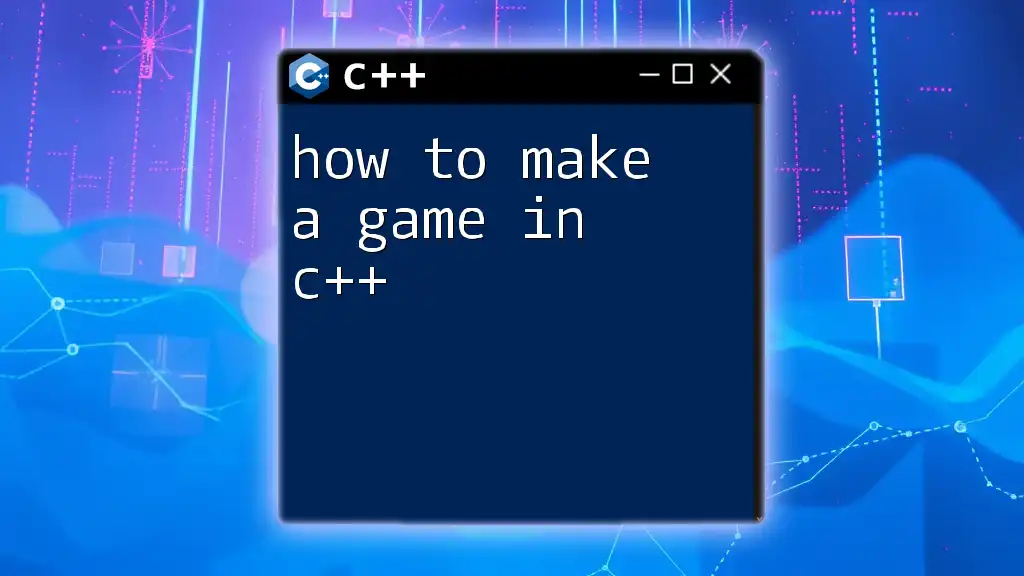
Writing the C++ Calculator Code
Starting with the Main Structure
Begin your calculator application by including the necessary header files. The `<iostream>` header is essential for input and output operations.
#include <iostream>
using namespace std;
Implementing Functions for Operations
Functions encapsulate the logic for specific tasks, making your code modular and easier to manage.
Creating Functions for Each Operation
You can create individual functions for addition, subtraction, multiplication, and division. Below are examples for each operation:
Addition Function
double add(double a, double b) {
return a + b;
}
Subtraction Function
double subtract(double a, double b) {
return a - b;
}
Multiplication Function
double multiply(double a, double b) {
return a * b;
}
Division Function
double divide(double a, double b) {
if (b == 0) {
throw std::invalid_argument("Cannot divide by zero.");
}
return a / b;
}
The Main Calculation Loop
You will create a loop to keep your calculator running until the user decides to exit. This keeps the user experience seamless.
Setting Up a Menu-Driven Interface
Prompt the user for input and display a menu of available operations.
int main() {
int choice;
double num1, num2;
do {
cout << "Select operation:\n";
cout << "1. Add\n";
cout << "2. Subtract\n";
cout << "3. Multiply\n";
cout << "4. Divide\n";
cout << "5. Exit\n";
cout << "Enter choice: ";
cin >> choice;
if (choice == 5) break; // Exit option
cout << "Enter two numbers: ";
cin >> num1 >> num2;
// Perform selected operation (to be completed)
} while(choice >= 1 && choice <= 5);
}
Switch Case for Operation Selection
A `switch` statement can simplify the execution of the selected operation. It’s a good practice to use it when you have multiple choices.
switch (choice) {
case 1:
cout << "Result: " << add(num1, num2) << endl;
break;
case 2:
cout << "Result: " << subtract(num1, num2) << endl;
break;
case 3:
cout << "Result: " << multiply(num1, num2) << endl;
break;
case 4:
try {
cout << "Result: " << divide(num1, num2) << endl;
} catch (const std::invalid_argument& e) {
cout << e.what() << endl; // Handle divide by zero error
}
break;
default:
cout << "Invalid choice. Please try again.\n";
break;
}
Displaying Results
Finally, ensure that results are displayed clearly. Provide descriptive output, indicating which operation was performed.
cout << "The sum of " << num1 << " and " << num2 << " is: " << add(num1, num2) << endl;
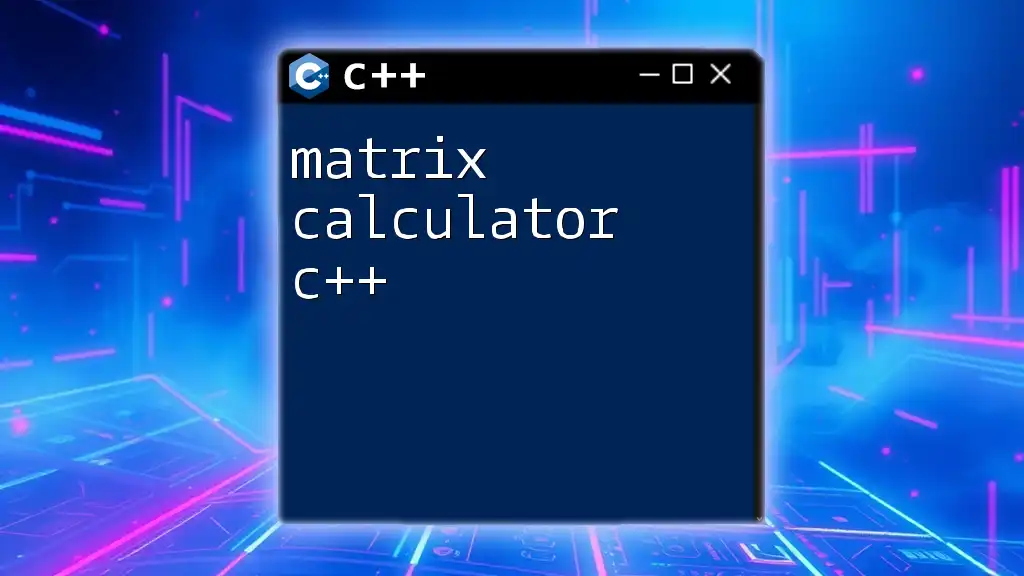
Complete Calculator Code Example
Here's how all parts come together in a cohesive code sample:
#include <iostream>
using namespace std;
double add(double a, double b) { return a + b; }
double subtract(double a, double b) { return a - b; }
double multiply(double a, double b) { return a * b; }
double divide(double a, double b) {
if (b == 0) throw std::invalid_argument("Cannot divide by zero.");
return a / b;
}
int main() {
int choice;
double num1, num2;
do {
cout << "Select operation:\n";
cout << "1. Add\n";
cout << "2. Subtract\n";
cout << "3. Multiply\n";
cout << "4. Divide\n";
cout << "5. Exit\n";
cout << "Enter choice: ";
cin >> choice;
if (choice == 5) break;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
switch (choice) {
case 1:
cout << "Result: " << add(num1, num2) << endl;
break;
case 2:
cout << "Result: " << subtract(num1, num2) << endl;
break;
case 3:
cout << "Result: " << multiply(num1, num2) << endl;
break;
case 4:
try {
cout << "Result: " << divide(num1, num2) << endl;
} catch (const std::invalid_argument& e) {
cout << e.what() << endl;
}
break;
default:
cout << "Invalid choice. Please try again.\n";
break;
}
} while (choice >= 1 && choice <= 5);
return 0;
}
Example Usage
This calculator will loop, prompting users for operation and inputs until they choose to exit. When complete, it displays the results clearly after each operation.
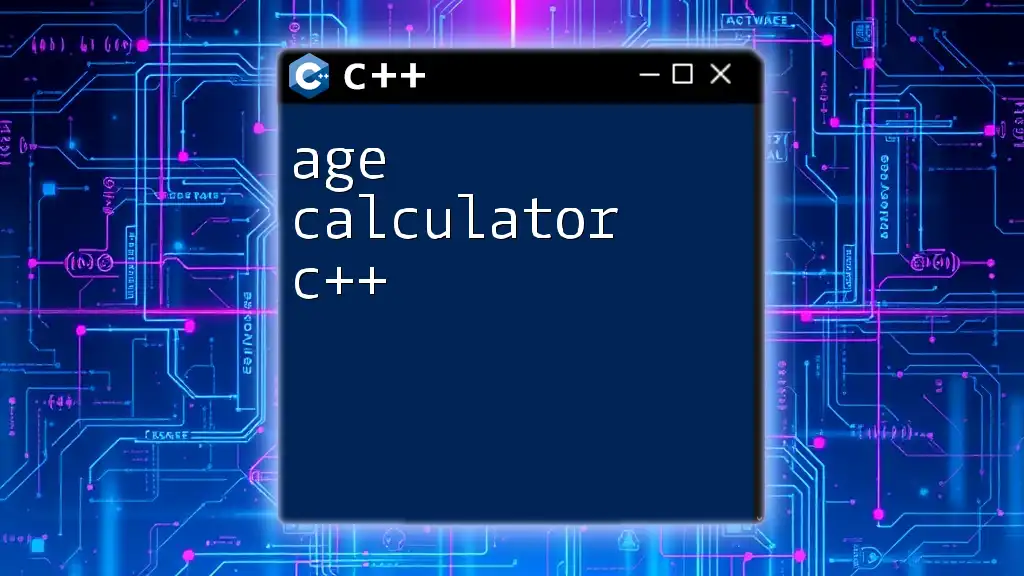
Testing and Debugging the Calculator
Common Issues and Errors
While developing your calculator, be vigilant for common issues like trying to divide by zero, which can crash your program if not handled correctly.
Best Practices for Debugging
To ensure your calculator functions as intended, take advantage of debugging tools in your IDE. Utilize breakpoints and step through your code to inspect variable values at runtime. Writing clean and organized code will also significantly enhance your debugging experience.
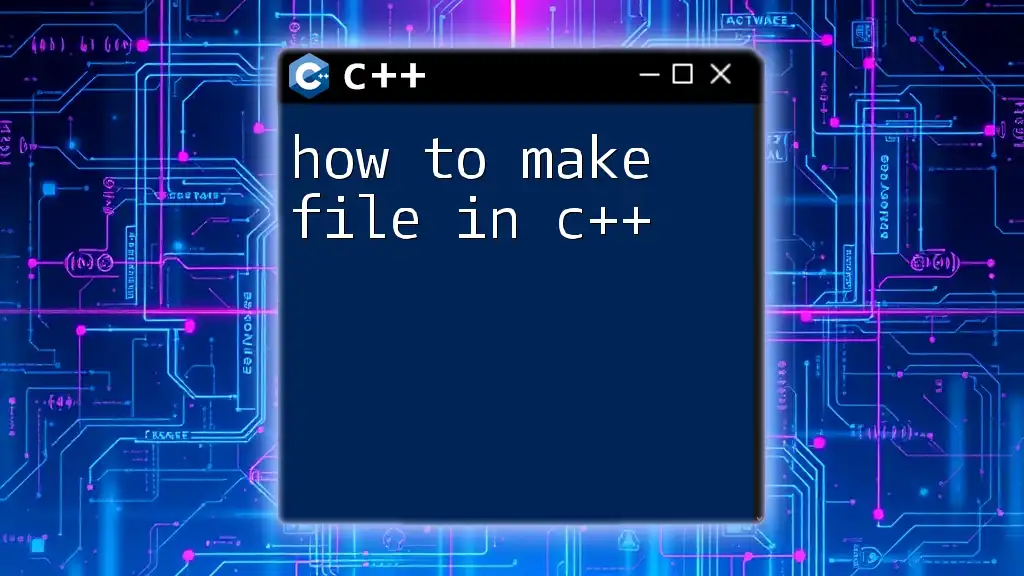
Conclusion
As you’ve learned, creating a basic calculator in C++ involves understanding fundamental programming concepts, designing functional code, and ensuring a user-friendly interface. By employing functions, control structures, and effective error handling, you’ve successfully built a simple yet powerful calculator.
You are encouraged to explore further by adding advanced features such as exponential operations, memory functions, or even a graphical user interface (GUI). Learning and implementing such concepts will enrich your programming toolkit and help you grow as a C++ developer.