Creating a simple game engine in C++ involves setting up a basic structure to manage game states, handle rendering, and process input efficiently.
Here's a minimal example of a game engine initialization in C++:
#include <iostream>
class GameEngine {
public:
void init() {
std::cout << "Game Engine Initialized!" << std::endl;
}
void run() {
while (true) {
update();
render();
}
}
private:
void update() {
// Game logic goes here
}
void render() {
// Rendering code goes here
std::cout << "Rendering Frame..." << std::endl;
}
};
int main() {
GameEngine engine;
engine.init();
engine.run();
return 0;
}
Setting Up Your Development Environment
To get started with how to make a game engine with C++, it’s essential to have the right tools at your disposal.
Installation of Compiler and IDE
First, choose a compiler that suits your operating system. Popular choices include GCC for Linux, Clang, and MSVC for Windows. For your Integrated Development Environment (IDE), options like Visual Studio or Code::Blocks offer intuitive interfaces and robust features that are beneficial for game development.
Setting Up a Project Structure
A well-organized project structure helps maintain clarity and manageability. Consider organizing your files in directories for assets (textures, models, sounds), source code (C++ files), and any libraries you may use. Using a build system like CMake or Makefile will streamline the process of compiling your code and managing dependencies.
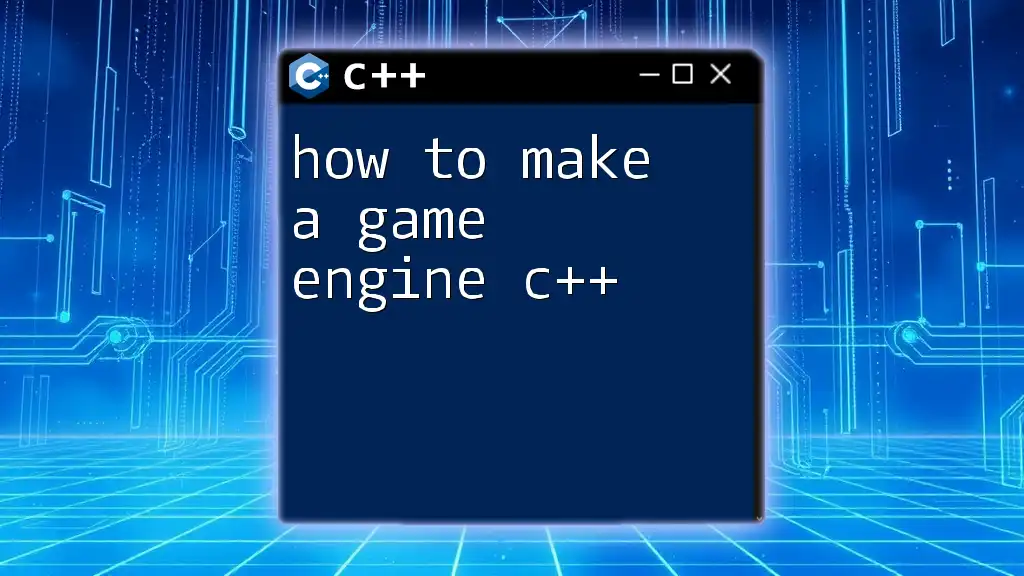
Understanding the Basics of Game Engine Architecture
Before diving into coding, it's crucial to grasp the core components of a game engine.
Main Components of a Game Engine
A typical game engine consists of several key components:
- Graphics Rendering: Responsible for displaying graphics on the screen, typically utilizing APIs like OpenGL or DirectX.
- Physics Engine: Handles the physics behaviors in the game, such as collisions and object movements.
- Audio Handling: Manages sound effects and music playback.
- Input Management: Processes user inputs from various devices (keyboard, mouse, gamepads).
- Scripting: Allows for dynamic runtime behavior and gameplay changes without recompilation.
Game Loop Fundamentals
The game loop is the heart of your engine, responsible for executing the game's logic and rendering frames. It typically follows this structure:
- Initialization: Set up the necessary systems (graphics, audio, physics).
- Input Handling: Collect user inputs.
- Update: Execute game logic and update object states.
- Render: Draw the game state to the screen.
A basic example of a game loop in C++ could look like this:
// Example: Basic Game Loop Structure
#include <iostream>
#include <GLFW/glfw3.h>
void frameBufferSizeCallback(GLFWwindow* window, int width, int height) {
glViewport(0, 0, width, height);
}
int main() {
// Initialize GLFW and create window
glfwInit();
GLFWwindow* window = glfwCreateWindow(800, 600, "Game Engine", NULL, NULL);
glfwMakeContextCurrent(window);
glfwSetFramebufferSizeCallback(window, frameBufferSizeCallback);
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT);
// Update and render logic goes here
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwTerminate();
return 0;
}
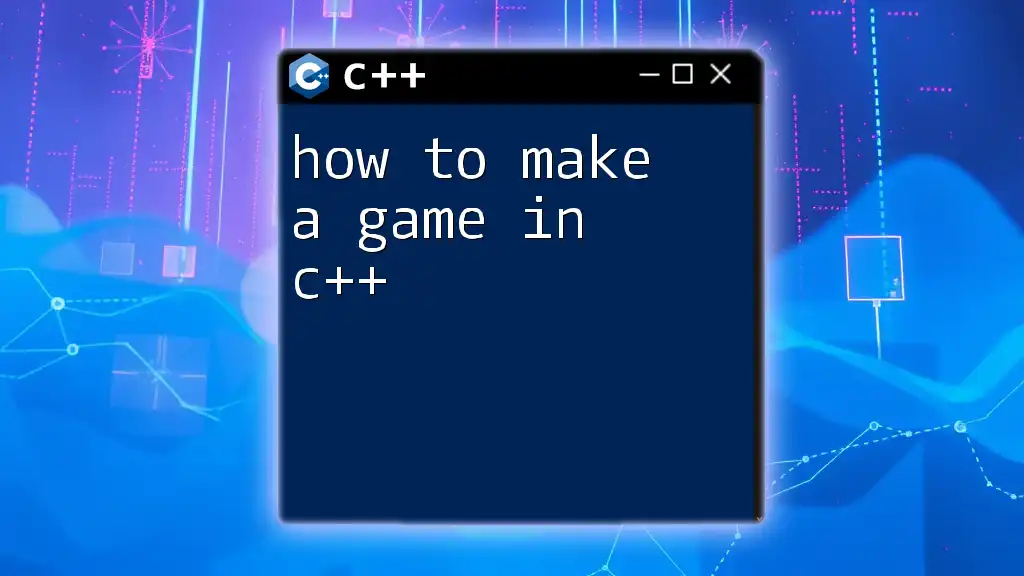
Creating the Core of Your Game Engine
Graphics Rendering
Selecting the right graphics API is pivotal in how to make a game engine with C++. OpenGL is widely used and provides a lot of flexibility. Start by creating a window and rendering context using a library like GLFW. Here’s a basic implementation for setting up a rendering context:
// Example: Setting up a Window using GLFW
#include <GLFW/glfw3.h>
int main() {
glfwInit();
GLFWwindow* window = glfwCreateWindow(800, 600, "Hello Game", NULL, NULL);
if (window == NULL) {
std::cerr << "Failed to create GLFW window" << std::endl;
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// Main loop
}
Physics Engine Integration
Integrating a physics engine like Box2D or Bullet is essential for more realistic gameplay. Implement your physics calculations to handle forces, impacts, and object states efficiently.
Audio Management
To manage audio, consider libraries such as OpenAL or FMOD. By incorporating these libraries, you can effectively load and play sound effects. For instance:
// Simple Audio Loading Example
#include <AL/al.h>
#include <AL/alc.h>
void playSound(const char* soundFilePath) {
// Implement sound loading and playback
}
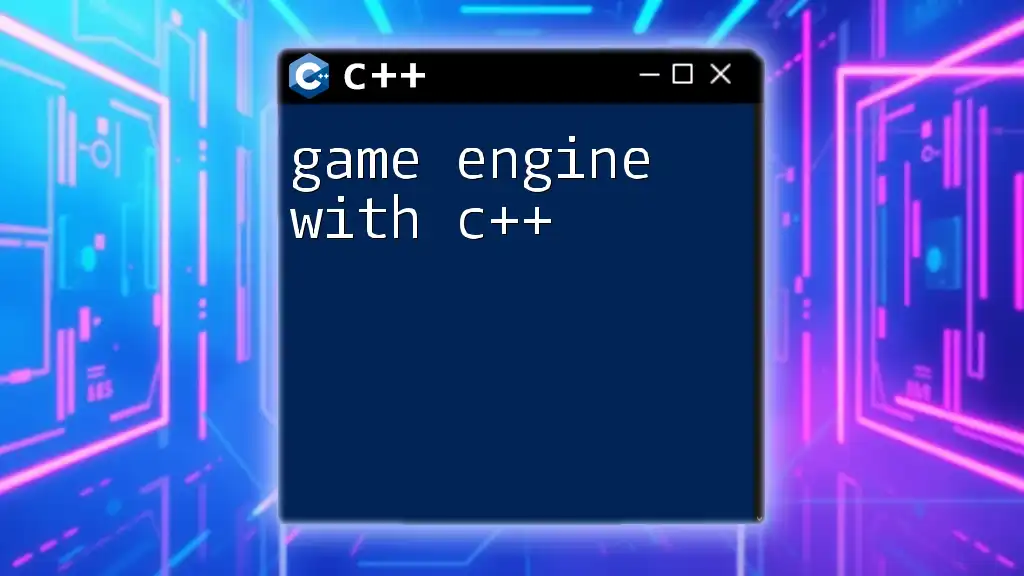
Adding Game Features
Creating a Scene Graph
Scene graphs are crucial for organizing and managing different objects within your game. They help with scene rendering and allow easier transformations (translation, rotation, scaling). Implementing a simple scene graph structure can help you start organizing your game data effectively.
Entity-Component System (ECS) Architecture
ECS is a powerful architectural pattern that facilitates the composition of game objects. Here’s a basic implementation:
// Minimalistic ECS Example
class Entity {
public:
template<typename T> void addComponent(T component) {
// Store component in a map or vector
}
};
class Component {
// Base class for all components
};
Scripting System Integration
Integrating a scripting system can significantly enhance game flexibility. You can choose popular scripting languages like Lua or Python, and embed them into your engine for dynamic gameplay scripting.
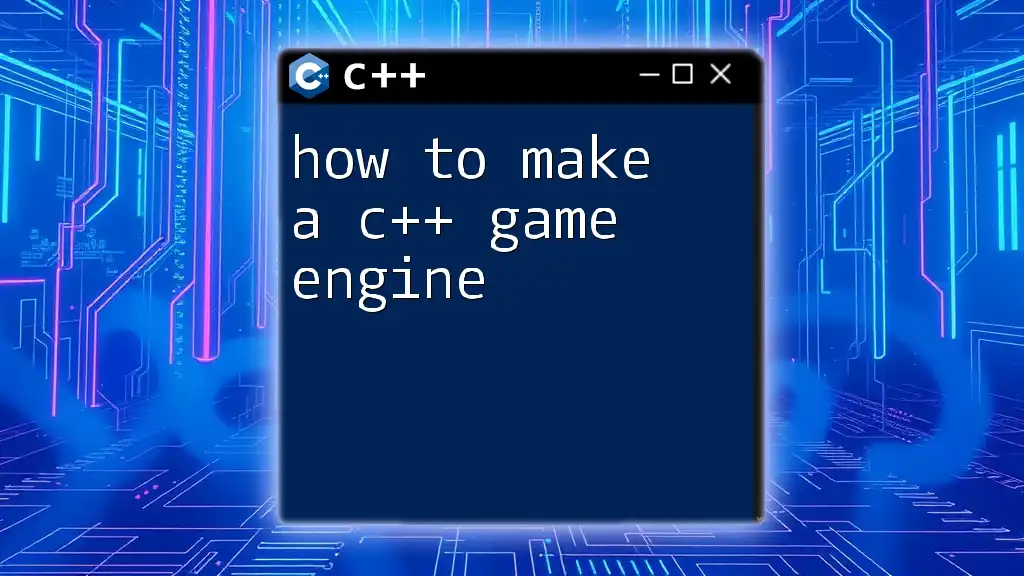
Working with Assets
Loading and Managing Textures/Models
Utilizing third-party libraries like Assimp (for models) and stb_image (for textures) will simplify the loading of various asset types. For example, loading a texture with stb_image may look like this:
// Texture loading with stb_image
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h"
unsigned char* data = stbi_load("path/to/texture.jpg", &width, &height, &nrChannels, 0);
if (data) {
// Create OpenGL texture
stbi_image_free(data);
}
Implementing Scene Management
Implementing a scene management system allows you to transition between different game states smoothly, such as menus and gameplay. Establish a central controller class that manages these transitions to keep your code organized.
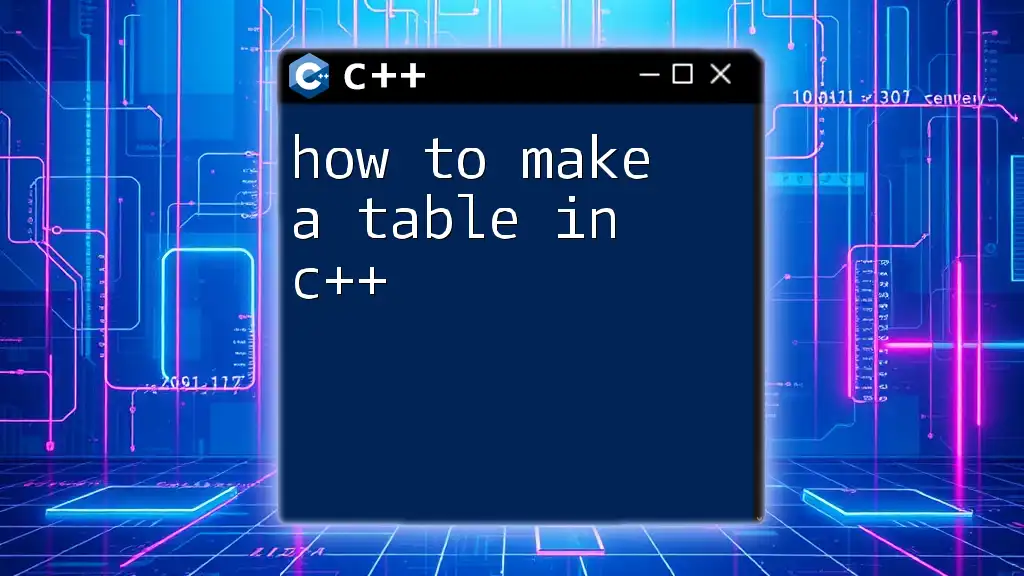
Optimizing Your Game Engine
Performance Considerations
Optimizing your engine is essential for ensuring smooth performance. Consider implementing multi-threading to separate heavy computations from rendering tasks. Additionally, using memory pools can be beneficial to minimize memory fragmentation.
Best Practices for C++ Game Development
It's crucial to adhere to coding standards and practices for maintainable code. Regularly documenting your code and performing code reviews can contribute to a more robust codebase.
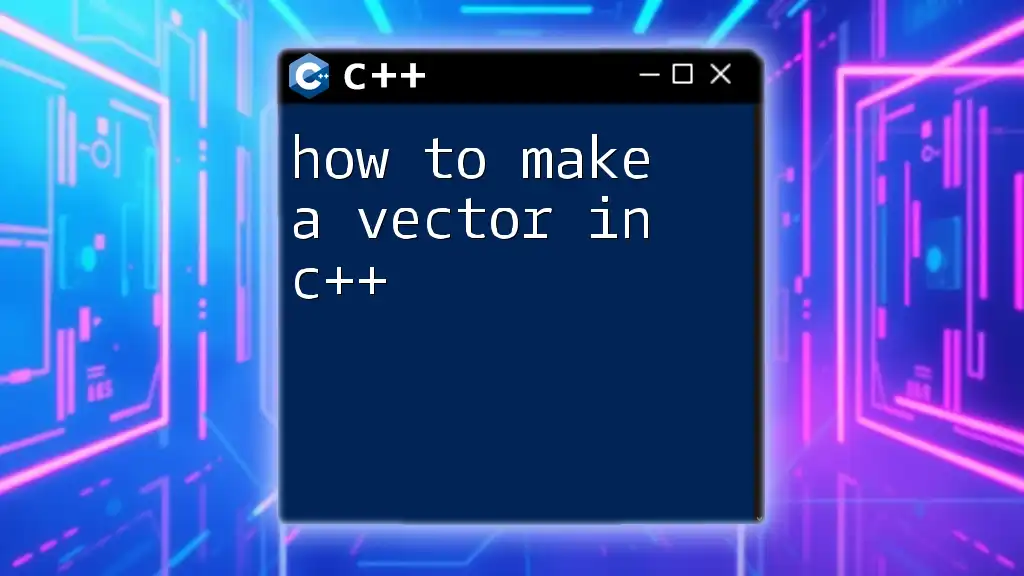
Testing and Debugging
Unit Testing and Debugging Strategies
Implementing unit tests using frameworks like Google Test helps catch bugs early in the development process. Additionally, tools like Valgrind can assist in analyzing memory usage, helping you optimize and stabilize your engine.
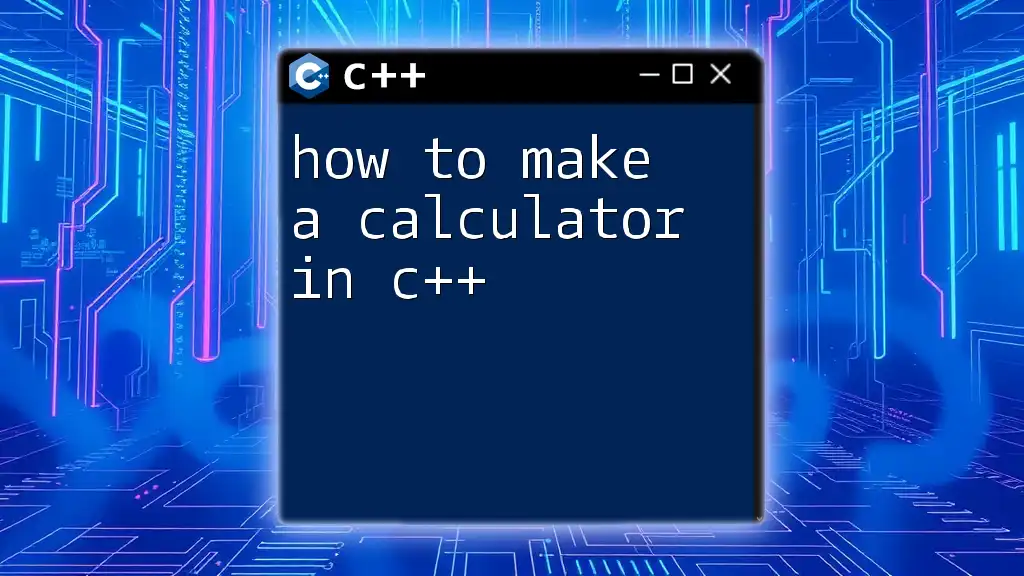
Conclusion
In summary, building a game engine with C++ demands an understanding of several systems, from graphics and physics to asset management. The journey is demanding but incredibly rewarding, offering a solid foundation to create games. As you continue to develop your skills, explore resources like books, online courses, and community forums for additional insights and support. Embrace the challenges, share your projects, and continue learning in the ever-evolving realm of game development!