Creating a C++ game engine involves setting up a basic structure for game loops, rendering, and input handling, allowing you to build games by managing various game objects and systems efficiently.
Here’s a simple example of a main game loop in C++:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
bool isRunning = true;
while (isRunning) {
// Update game state
std::cout << "Updating game state..." << std::endl;
// Render game
std::cout << "Rendering game..." << std::endl;
// Delay for a frame (simulate a simple fixed time step)
std::this_thread::sleep_for(std::chrono::milliseconds(16)); // ~60 FPS
}
return 0;
}
What is a Game Engine?
A game engine is a software framework designed to support the creation and development of video games. It provides foundational tools and features that streamline various aspects of game development, enabling developers to focus on gameplay mechanics and content creation rather than underlying technical complexities. Commonly found features in game engines include a rendering engine for graphics, a physics engine for simulating real-world physics, and comprehensive input management systems.
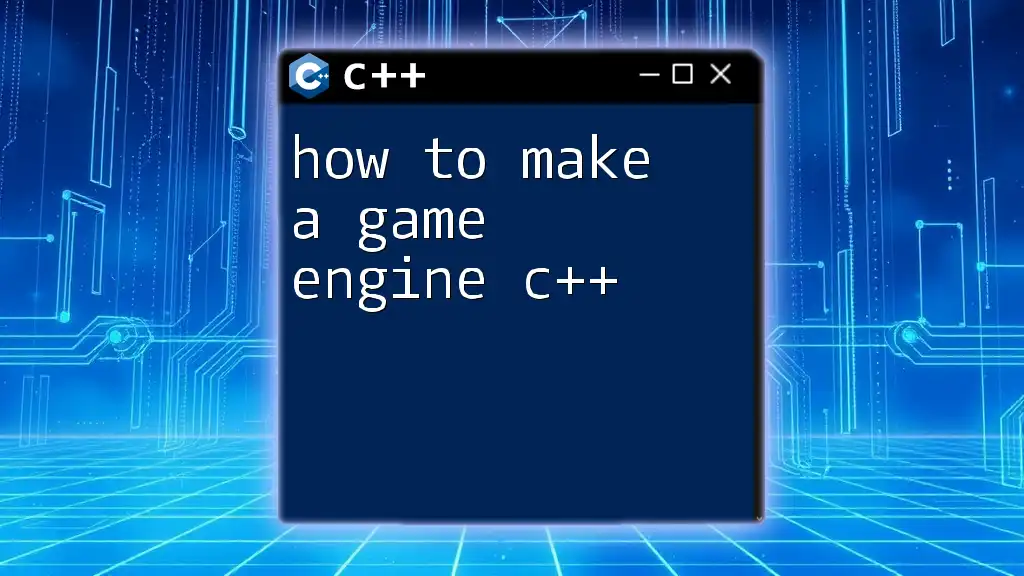
Why C++ for Game Development?
C++ is the preferred language for game development due to its performance capabilities and control over system resources. It allows developers to write low-level code that runs close to the hardware, ensuring efficient performance which is critical in gaming. Moreover, many leading game engines, including Unreal Engine, are built using C++, making it an industry standard and an invaluable skill for aspiring game developers.
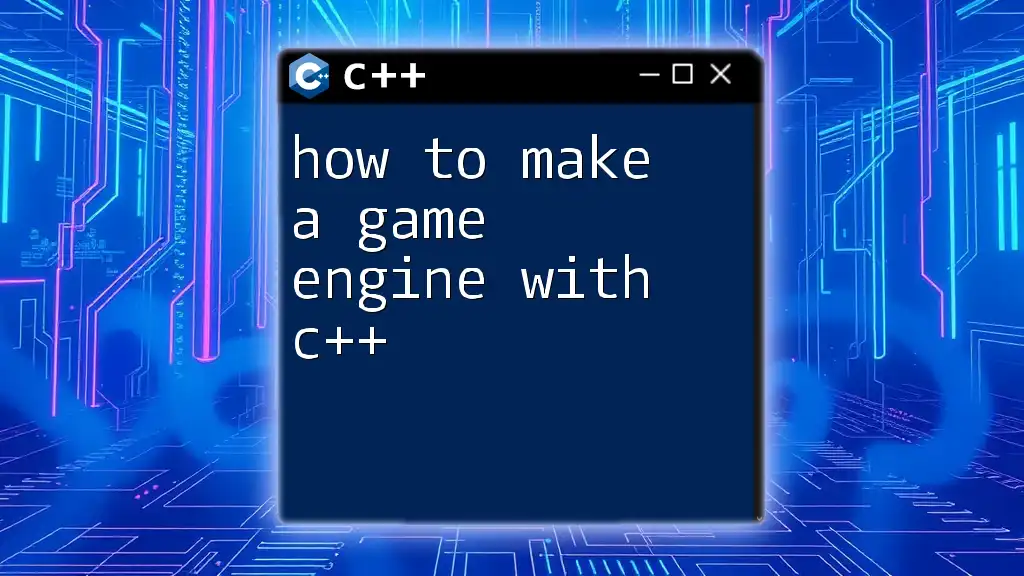
Understanding the Architecture of a Game Engine
Game Engine Components
A game engine typically consists of several core systems that work together seamlessly. These systems include:
- Rendering: Responsible for drawing graphics on the screen, influencing how visuals appear in the game.
- Physics: Manages simulations of real-world physics, like gravity and collision.
- Input: Captures user input from devices like keyboards, mice, and controllers.
The Game Loop
The game loop is a fundamental concept in game development that continually updates the game state and renders frames on the screen. It operates through a repetitive cycle that encompasses game logic processing, input handling, updating physics, and rendering graphics.
Here’s a basic representation of a game loop in C++:
while (gameIsRunning) {
processInput();
updateGameState();
renderGraphics();
}
This loop ensures that your game remains responsive to user actions while continually updating and displaying the game’s content.
The Rendering Engine
What is Rendering?
Rendering is the process of generating a visual representation of a game. It can involve rendering 2D graphics or 3D models and is a crucial aspect of a game engine.
Creating a Basic Rendering Engine
To set up a basic rendering engine, you can use graphics libraries like SDL (Simple DirectMedia Layer) or OpenGL. Here’s a simple initialization example using SDL:
#include <SDL2/SDL.h>
void initSDL() {
SDL_Init(SDL_INIT_VIDEO); // Initialize SDL with video subsystem
SDL_Window* window = SDL_CreateWindow("Game Window",
SDL_WINDOWPOS_CENTERED,
SDL_WINDOWPOS_CENTERED,
800, 600,
SDL_WINDOW_SHOWN);
}
This snippet initializes SDL and creates a window, the starting point for rendering graphics in your game.
The Physics Engine
Introduction to Physics in Games
Physics simulation is vital in game development as it gives a sense of realism and interaction in the gameplay. This involves handling aspects like gravity, momentum, and collision detection.
Creating Simple Physics Models
To create simple physics models, you'll need to implement collision detection and response. Here’s a basic example of collision detection between two circles:
bool checkCollision(Circle a, Circle b) {
float distance = sqrt(pow(b.x - a.x, 2) + pow(b.y - a.y, 2));
return distance < (a.radius + b.radius);
}
This function checks if two circles overlap, returning true if they do. This is essential for implementing game mechanics where interactions occur.
Input Management
Handling User Input
User input is crucial for player interactivity in games. Capturing actions like keyboard presses and mouse movements ensures that players can control their in-game experience effectively.
Creating an Input Manager
To implement an input manager with SDL, you can use the following code snippet:
void processInput() {
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
gameIsRunning = false;
}
if (event.type == SDL_KEYDOWN) {
// Handle key press events
}
}
}
This example captures events like quitting the game and key presses, enabling dynamic user input handling.
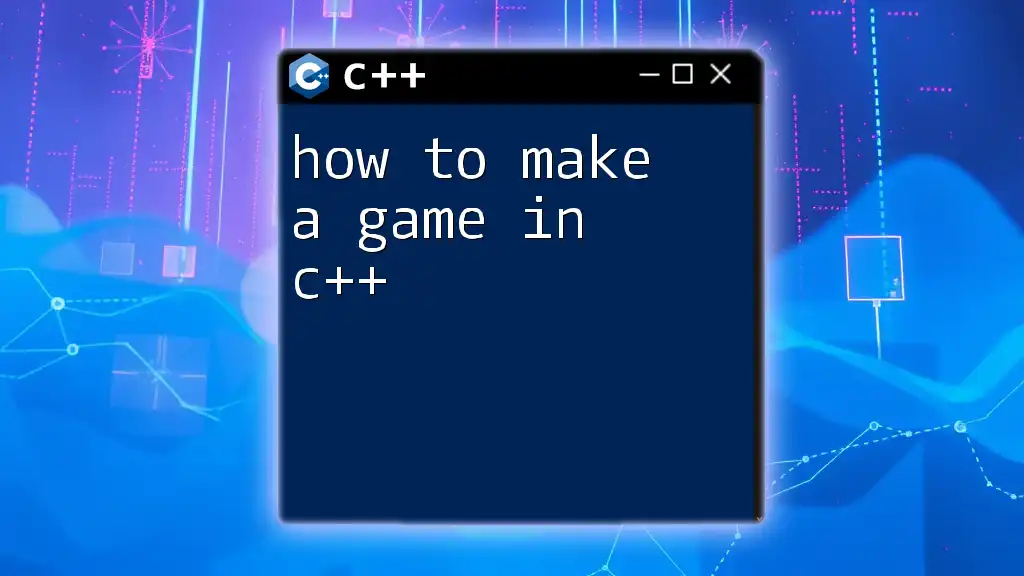
Designing the Game Engine
Engine Architecture Patterns
Entity-Component-System (ECS)
The Entity-Component-System architecture is an effective design pattern embraced in game development. It promotes better organization of game entities, using components to represent various functionalities separately from the entities themselves.
In an ECS design:
- An Entity is a general-purpose object.
- A Component holds data describing properties.
- A System contains logic to process entities that have specific components.
This architecture enhances flexibility and scalability, making it easier to manage complex interactions within your game.
Scripting and Extensibility
Making Your Engine Modular
Allowing changes without recompiling the entire engine is crucial for efficient development. Integrating scripting languages such as Lua or Python can enhance modularity and flexibility.
Integrating Scripting Languages
Setting up Lua within your C++ engine can be performed with the following steps:
- Include the Lua headers and link against the Lua library.
- Initialize the Lua state in your C++ code.
- Load and execute Lua scripts that define game behaviors.
An example initialization might look like this:
extern "C" {
#include "lua.h"
#include "lauxlib.h"
#include "lualib.h"
}
lua_State* L = luaL_newstate();
luaL_openlibs(L); // Load standard libraries
This allows your game logic to be defined in Lua, giving designers the ability to adjust gameplay mechanics without touching the C++ core.
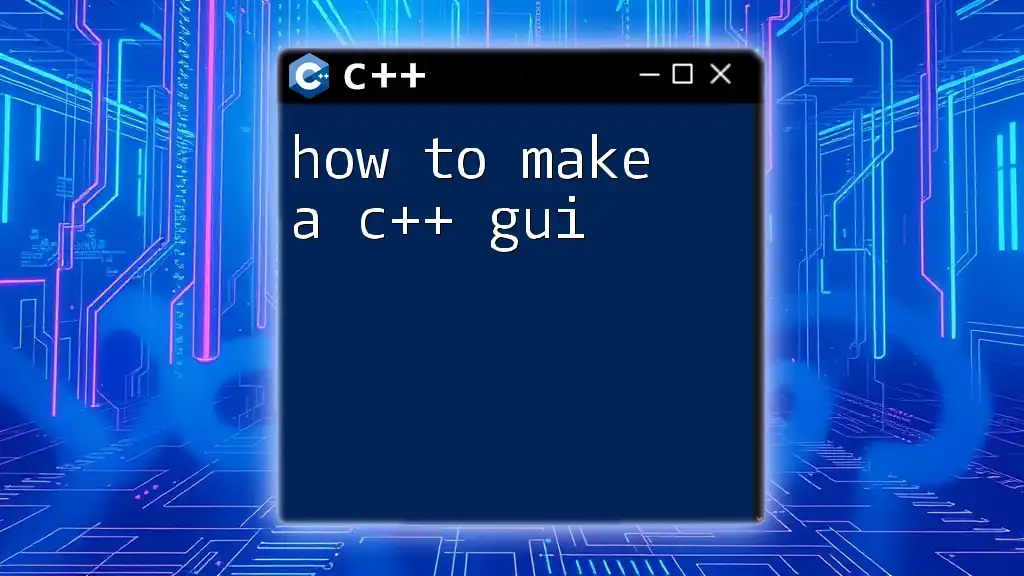
Building Your First Game with the Engine
Game Design Basics
Before diving into coding, understanding core principles of game design is paramount. This includes figuring out the target audience, game mechanics, and overall feel.
Prototyping Your Game Concept
Creating a prototype is essential to validate your game design concepts. Focus on building a small-scale version of your game to test mechanics in a controlled environment.
Implementing a Simple Game
To illustrate your engine's capabilities, let’s build a simple 2D game, such as Pong. The flow involves setting up the game state, rendering paddles, handling ball movement, and detecting collisions.
The following snippet outlines how to manage the game state:
enum GameState { MENU, PLAYING, GAME_OVER };
GameState currentState = MENU;
void updateGame() {
switch (currentState) {
case PLAYING:
// Update game logic for Pong
break;
case GAME_OVER:
// Handle end of game scenario
break;
default:
break;
}
}
This modular approach allows for smooth transitions between different game states while keeping your code organized.
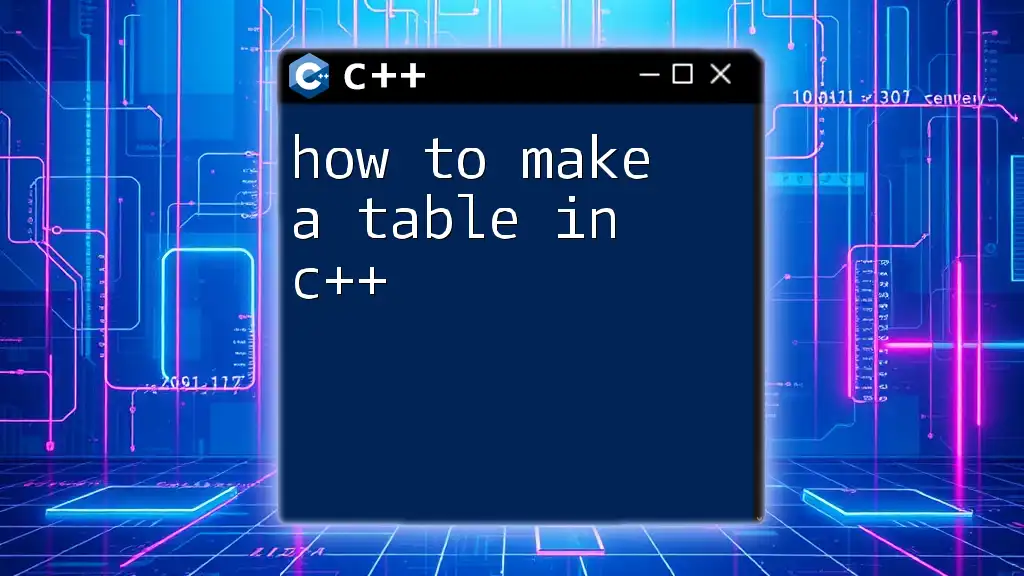
Testing & Debugging Your Engine
Importance of Testing
Testing is crucial to ensure your game engine operates reliably and efficiently. Bugs and errors can have significant impacts on player experience, which is why rigorous testing protocols must be in place.
Tools and Strategies for Effective Testing
Utilizing tools like gdb for debugging C++ code and Valgrind for memory management helps identify issues early. Moreover, unit testing frameworks like Google Test ensure your engine's components function as intended.
Performance Optimization
Optimizing your engine is vital for achieving smooth gameplay. Techniques include:
- Minimizing draw calls: Combine multiple objects into a single draw call when applicable.
- Using spatial partitioning: This can enhance performance by reducing the number of collision checks required.
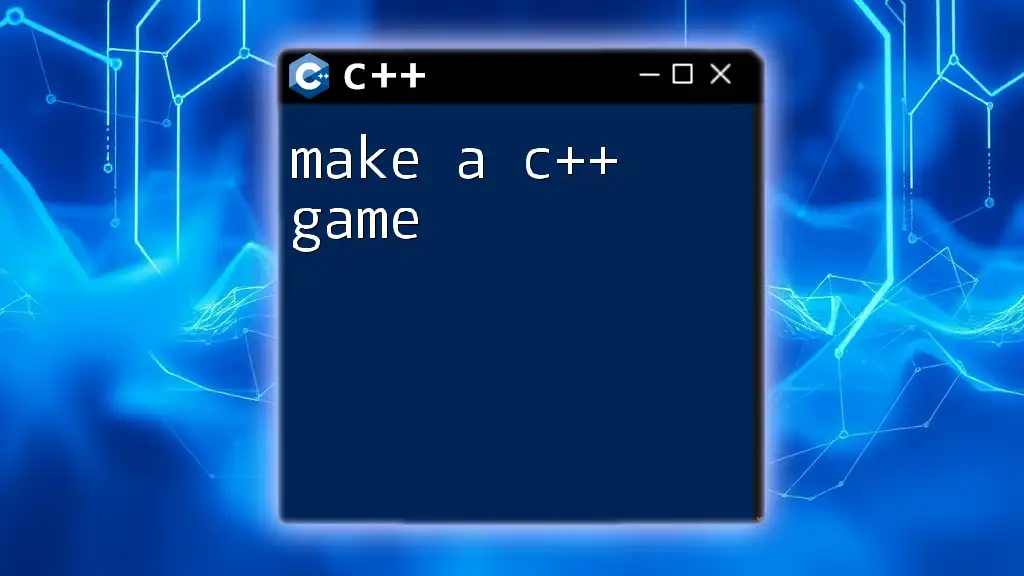
Future Directions and Enhancements
Continuing Development of Your Game Engine
Once you have a foundation, consider adding more advanced features such as artificial intelligence, networking capabilities, and enhanced physics simulations, transforming your engine into a more powerful tool.
Community Contribution and Open Source Considerations
Contributing your engine to the open-source community can accelerate development, draw input from other developers, and improve your codebase through collaboration. Moreover, creating thorough documentation and tutorials is essential to help new users become familiar with your engine’s features.
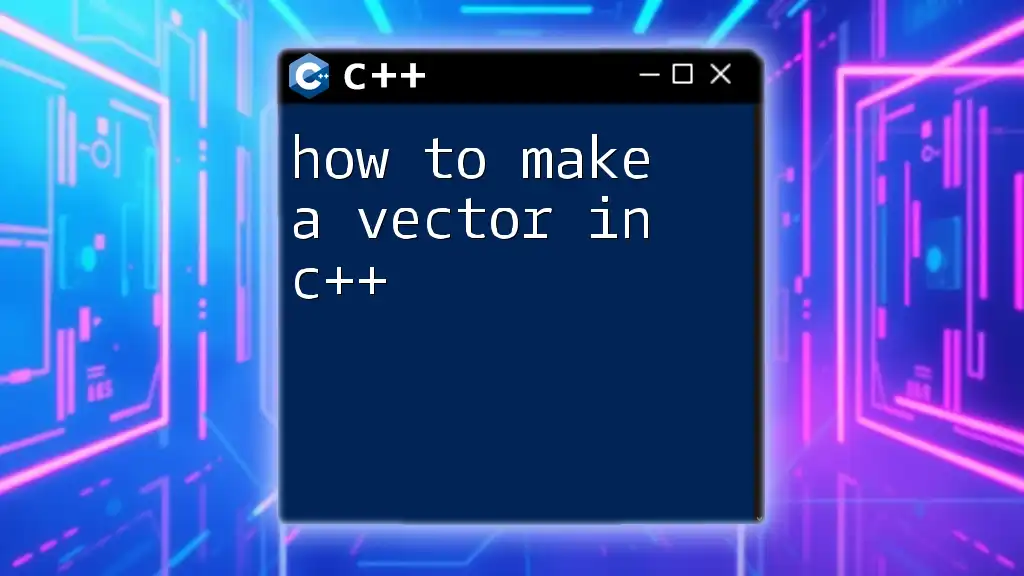
Conclusion
Building a C++ game engine is a challenging yet rewarding endeavor that encompasses multiple disciplines, from physics simulation to graphics rendering. With the right architecture, persistent testing, and community engagement, you can develop a powerful tool for game creation. Experimentation and exploration are key; as you understand how to make a C++ game engine, remember that each step of the journey offers invaluable learning opportunities and the potential for creating compelling gaming experiences.
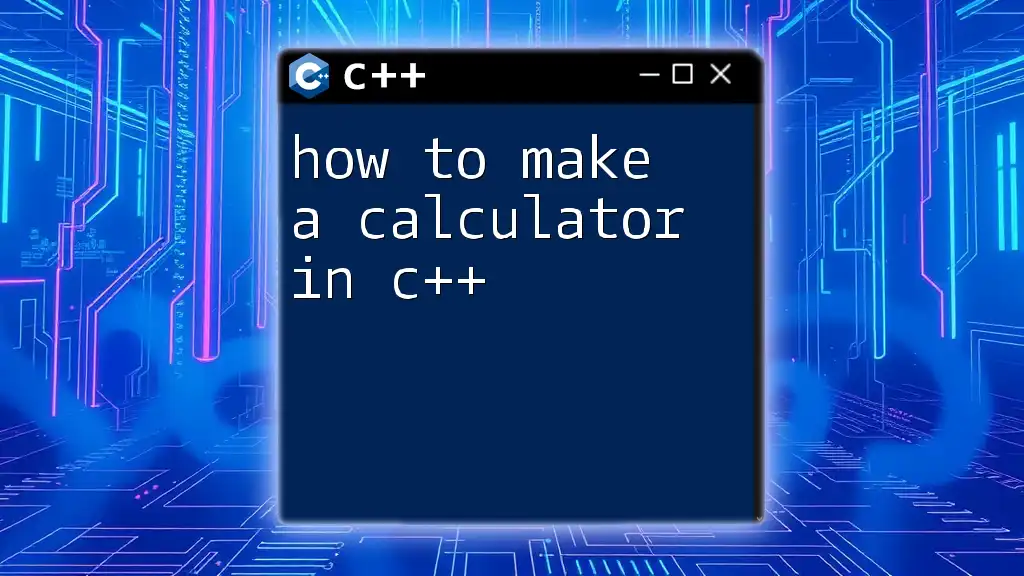
References
To further broaden your knowledge and skills, consider these resources:
- "Game Programming Patterns" by Robert Nystrom
- "Real-Time Rendering" by Tomas Akenine-Möller et al.
- SDL Documentation
- OpenGL Tutorial Series