In C++, comments are created using either double forward slashes (`//`) for single-line comments or `/` and `/` for multi-line comments, allowing you to annotate your code without affecting its execution.
Here is an example of both types of comments in C++:
// This is a single-line comment
/*
This is a
multi-line comment
*/
What is a Comment in C++?
In programming, comments are annotations within the source code that are ignored by the compiler. Their primary purpose is to provide context and explanations to those reading the code. Robust comments help maintain the code's clarity and enhance collaboration among developers.
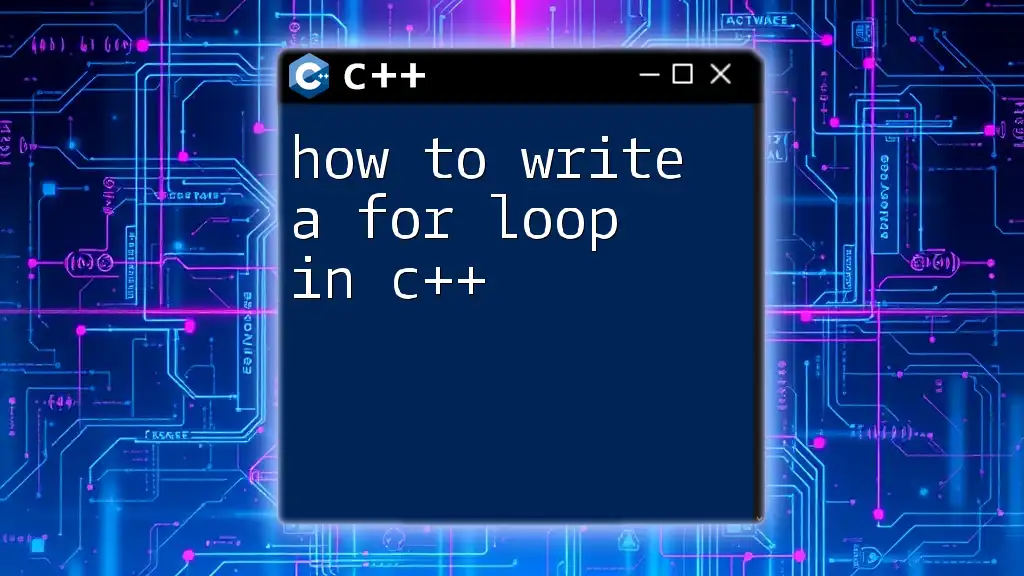
Types of Comments in C++
Single-line Comments
Single-line comments are designed to comment out a single line of code. In C++, they are initiated using `//`. Everything following the `//` on that line is considered a comment.
Example:
// This is a single-line comment in C++
int main() {
return 0; // This returns 0 to signify successful execution
}
In the example above, the comment explains the intent of the code without disrupting its functioning.
Multi-line Comments
Multi-line comments allow you to comment out several lines at once. This is done using the syntax `/* ... */`. Everything between these markers is treated as a comment.
Example:
/* This is a multi-line comment in C++
It can span multiple lines. */
int main() {
return 0; /* This comment ends here */
}
Such comments are particularly useful when you want to provide detailed explanations or temporarily disable blocks of code during testing.
Documentation Comments
Documentation comments are specialized comments that provide detailed information about the functionalities of code, especially for functions, classes, or modules. They often use specific formats that can be processed by documentation generation tools like Doxygen.
Example:
/**
* This function adds two integers.
* @param a First integer
* @param b Second integer
* @return Sum of a and b
*/
int add(int a, int b) {
return a + b;
}
In this example, the documentation comment outlines the purpose of the function, its parameters, and its output. This clarity allows other developers to understand the function's use without diving into its code.
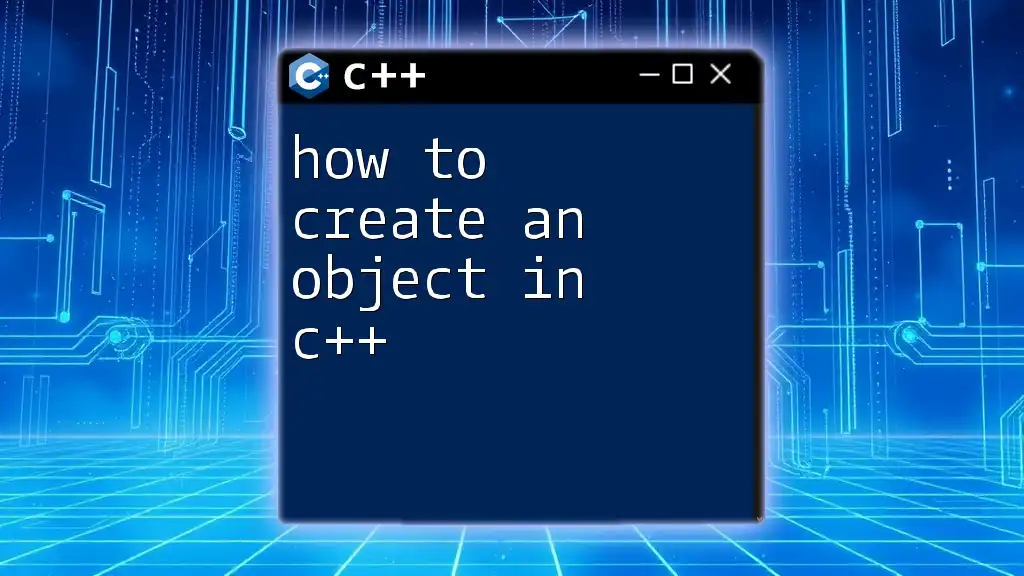
Best Practices for Commenting in C++
Write Meaningful Comments
Comments should serve a clear purpose and add value. They need to succinctly explain the logic behind your code or highlight the purpose of specific sections.
Example:
// Adding numbers (Poor)
int add(int a, int b) {
return a + b;
}
// Adds two integers and returns the result (Better)
The second comment is more informative and gives context, which helps readers quickly grasp what the function does.
Avoid Redundant Comments
Comments that reiterate what the code already makes clear can be detrimental, cluttering the code and obscuring functionality. Redundant comments can mislead readers into thinking they are necessary, while they may not be.
Example:
// Set x to 5 (Redundant)
int x = 5;
Here, the comment adds no value, as the code is self-explanatory.
Keep Comments Up-to-Date
Maintaining code often brings changes, which can render existing comments outdated. Regularly updating comments ensures that they accurately reflect the code's current intention and behavior, avoiding confusion.
Use Comments for Explanation, Not Just for Notation
Comments are especially crucial for complex algorithms. They provide necessary insights into your thought process and reasoning, guiding readers through intricate logic.
Example:
// Calculate the factorial of n using recursion
int factorial(int n) {
if(n <= 1) return 1; // Base case
return n * factorial(n - 1); // Recursive call
}
This example illustrates how comments elucidate the process, making it easier for readers to grasp the function's intent.
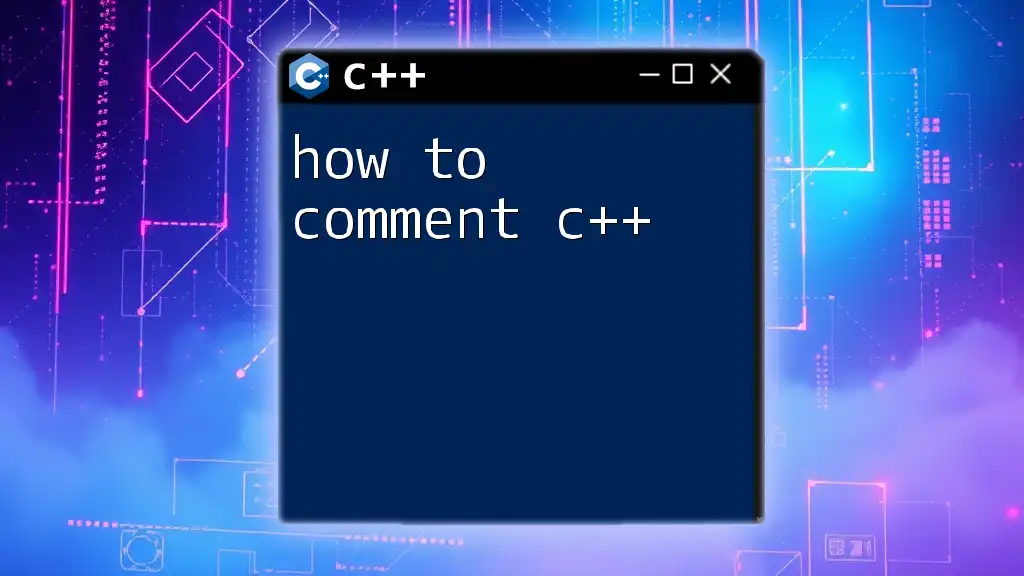
Common Mistakes in Commenting
Over-Commenting
While comments are beneficial, excessive commenting can make code cumbersome and harder to read. It's essential to strike a balance and ensure comments add clarity rather than redundancy.
Under-Commenting
Conversely, under-commenting can lead to misunderstandings. Without adequate explanations, readers may struggle to comprehend the code's purpose or logic. It's crucial to understand the right amount needed to maintain clarity.
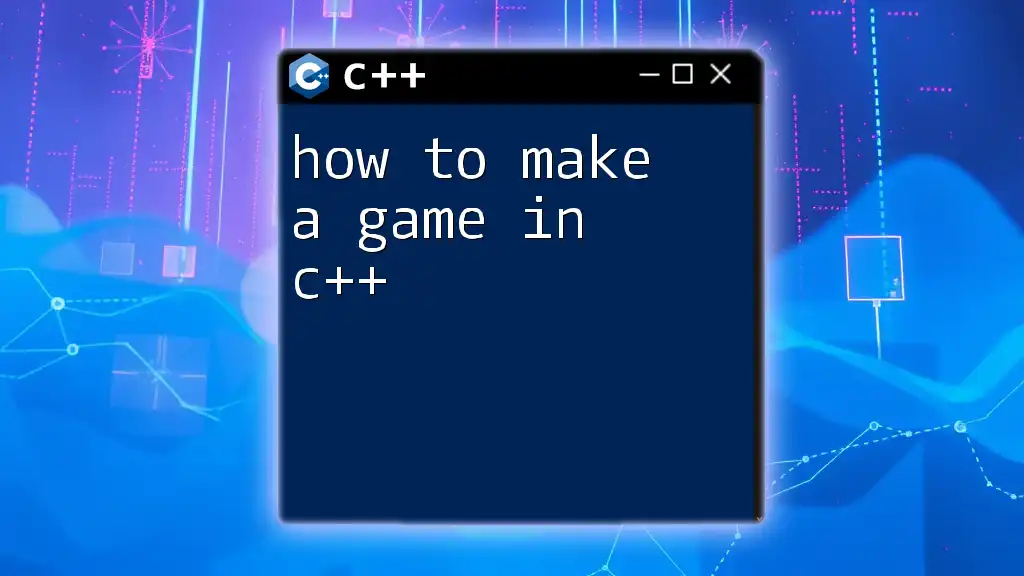
When Not to Comment
There are situations where adding comments can be unnecessary. If code is straightforward and self-explanatory, additional comments may clutter it.
This emphasizes the importance of writing clean, understandable code alongside comments.
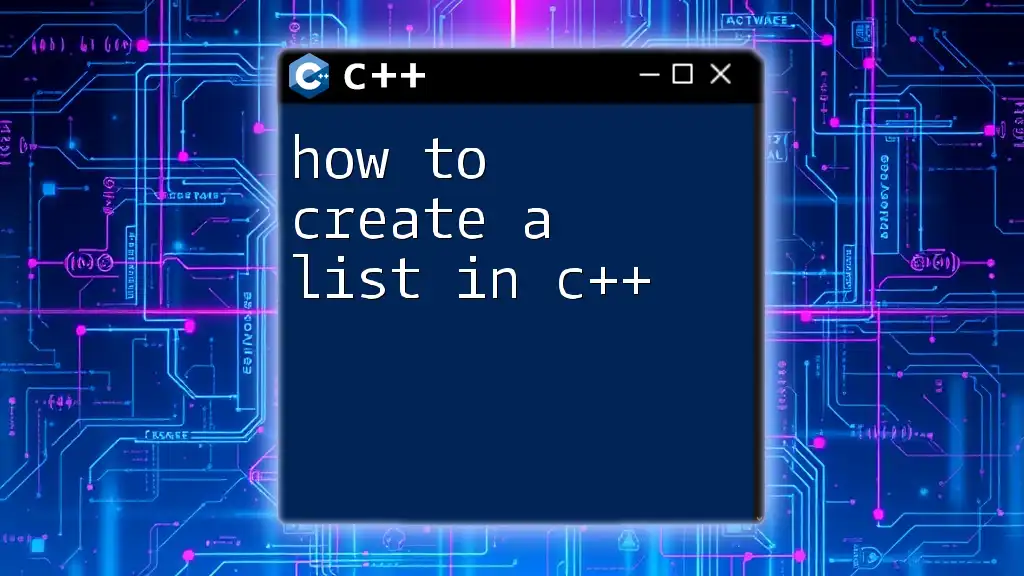
Tools to Aid in Commenting
Code Editors with Commenting Features
Modern integrated development environments (IDEs) and code editors offer various features that facilitate writing comments effectively. Some editors provide auto-completion for documentation comments and color-coding to set them apart from the code, helping developers make their commentary clearer.
Code Review Practices that Include Comments
In collaborative coding environments, emphasizing comment quality during code reviews can bolster best practices. Establishing standards for commenting can lead to consistent and informative comments across the codebase.
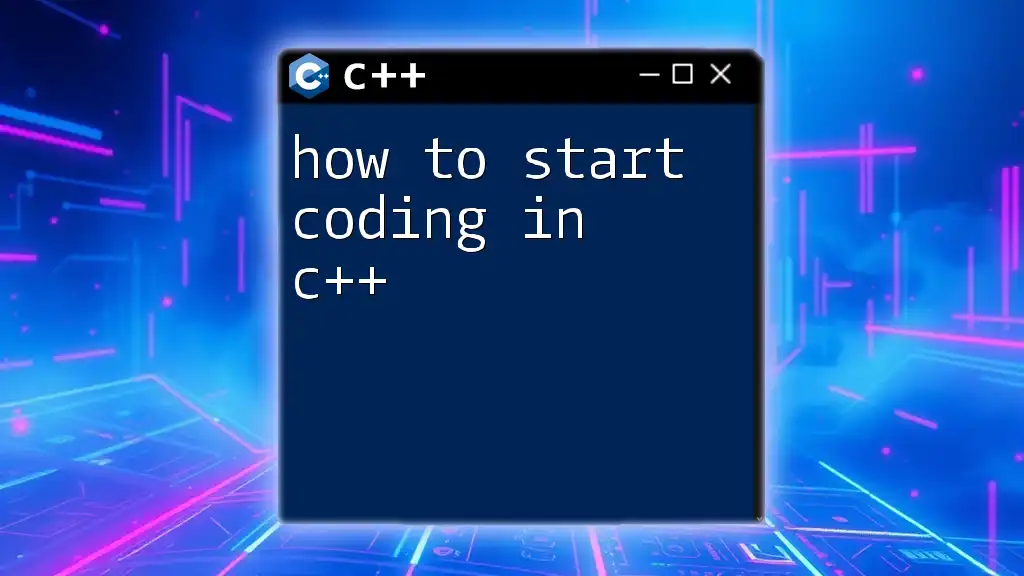
Conclusion
In summary, knowing how to write a comment in C++ is a vital skill for any developer seeking to create readable, maintainable code. Comments not only enhance clarity but also foster effective collaboration. By adopting good commenting practices, you can help yourself and others understand the code more efficiently, making future development and maintenance smoother.
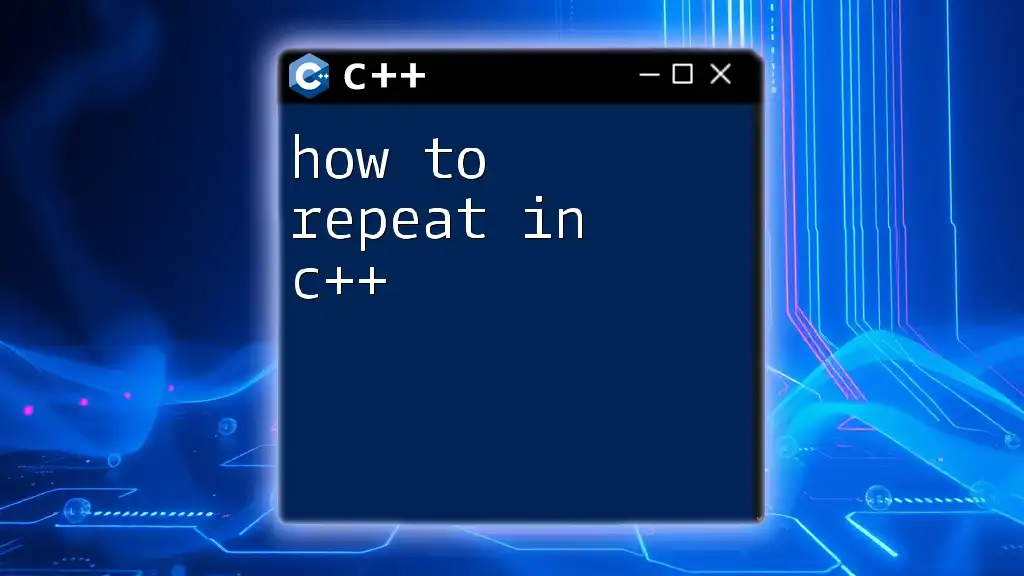
Call to Action
We encourage you to reflect on your commenting practices. Have you encountered challenges with comments in your code? Share your experiences and inspiration for future topics in our community!