To find the size of a string in C++, you can use the `size()` or `length()` method of the `std::string` class.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::cout << "Size of the string: " << str.size() << std::endl; // or str.length()
return 0;
}
Understanding Strings in C++
In C++, a string can be represented in two primary ways: as an `std::string`, which provides a dynamic and more feature-rich representation, or as a C-style string, which is essentially an array of characters terminated by a null character (`'\0'`). Understanding these types and their characteristics is essential when learning how to find the size of a string in C++.
Why is Knowing the Length of a String Vital?
Knowing the length of a string is essential in many programming scenarios, such as:
- Memory Management: Knowing the size helps in allocating appropriate memory for processes involving string manipulation.
- Validation: Checking string lengths is critical in validation scenarios, such as input handling where constraints on string lengths exist.
- Algorithms: Many algorithms for sorting, searching, and manipulating strings depend on knowing the size.
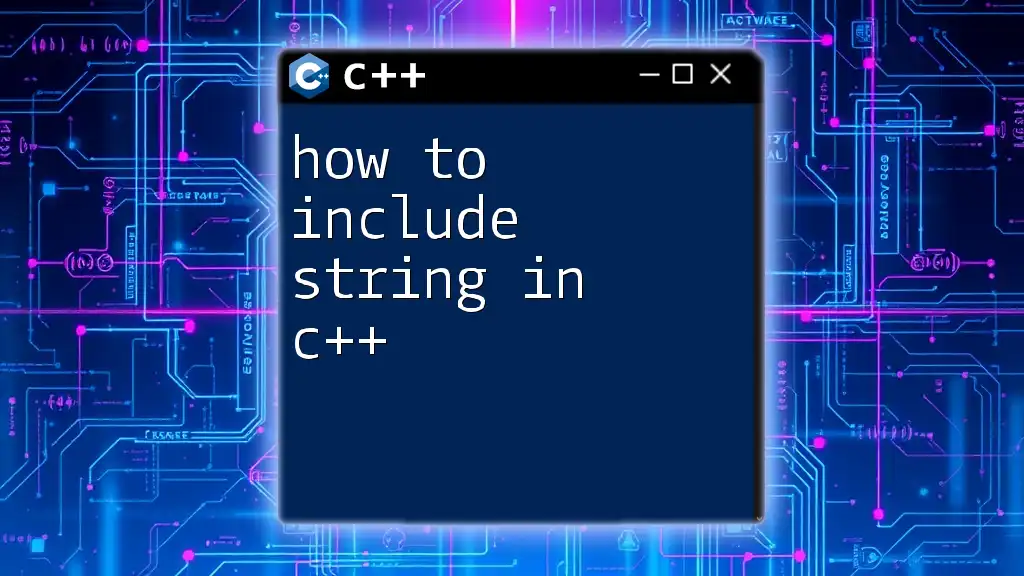
How to Get the Size of a String in C++
When you need to determine the size of a string in C++, two primary methods stand out in the context of `std::string`: `size()` and `length()`. Both methods serve the same purpose and can be used interchangeably.
Using the `size()` Method
The `size()` method returns the number of characters in the string. Here’s how you can use it:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::cout << "Size of the string: " << str.size() << std::endl;
return 0;
}
In this example, the output will be `Size of the string: 13`. The `size()` method calculates the total number of characters, including spaces and punctuation.
Using the `length()` Method
The `length()` method performs identically to `size()`, making it another way to obtain the string's character count. You might prefer `length()` for readability or personal style.
#include <iostream>
#include <string>
int main() {
std::string str = "C++ is great!";
std::cout << "Length of the string: " << str.length() << std::endl;
return 0;
}
This will output `Length of the string: 13`, again counting all characters.
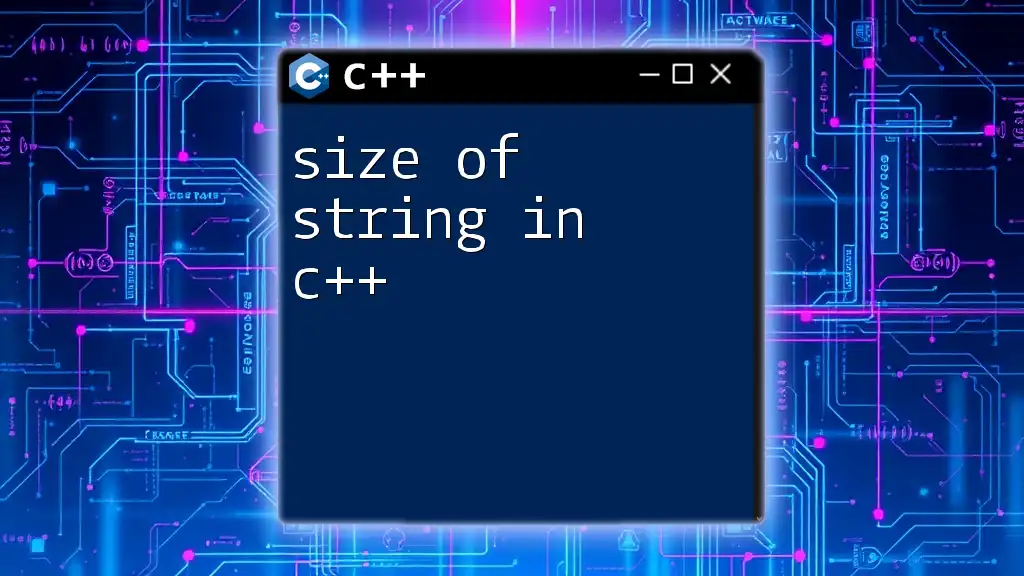
C++ String Length with C-Style Strings
When dealing with C-style strings, finding the length is done through the use of the `strlen()` function, which is part of the `<cstring>` library. Here’s an example of its usage:
#include <iostream>
#include <cstring>
int main() {
const char* cstr = "C-Style String";
std::cout << "Length of the C-Style string: " << strlen(cstr) << std::endl;
return 0;
}
In this case, you’ll get `Length of the C-Style string: 15`. The `strlen()` function traverses the C-style string until it finds the null terminator, counting the characters along the way.
Comparison with `std::string` Methods
Both `size()`/`length()` and `strlen()` return the count of characters, yet `std::string` methods work directly on a string object while `strlen()` requires a pointer to a C-style string. As a result, `std::string` methods are often preferred in modern C++ programming for their safety and ease of use.
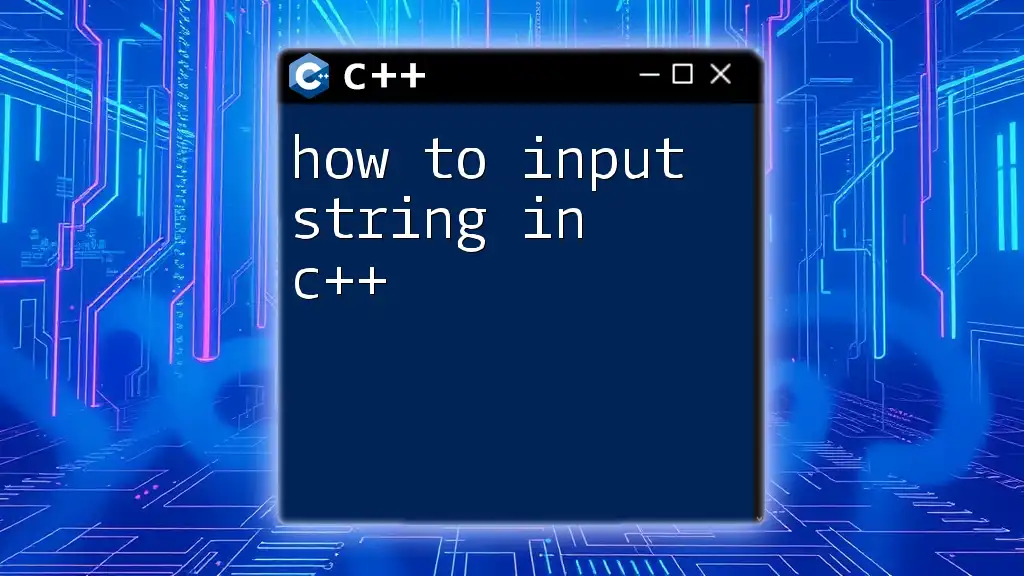
Finding Length of String in C++
Using Iterators
You can also calculate the length of a string using iterators, providing a more manual approach to determine size:
#include <iostream>
#include <string>
int main() {
std::string str = "Iterator Example";
int length = std::distance(str.begin(), str.end());
std::cout << "String length using iterators: " << length << std::endl;
return 0;
}
This example uses the `std::distance()` function, which calculates the number of elements between two iterators, resulting in `String length using iterators: 16`.
Using Range-Based For Loop
Another alternative is to utilize a range-based for loop to count the number of characters explicitly:
#include <iostream>
#include <string>
int main() {
std::string str = "Range Loop";
int length = 0;
for (char c : str) {
length++;
}
std::cout << "Length using range-based for loop: " << length << std::endl;
return 0;
}
In this case, the loop iterates over each character in the string to compute its length, which will give you `Length using range-based for loop: 10`.
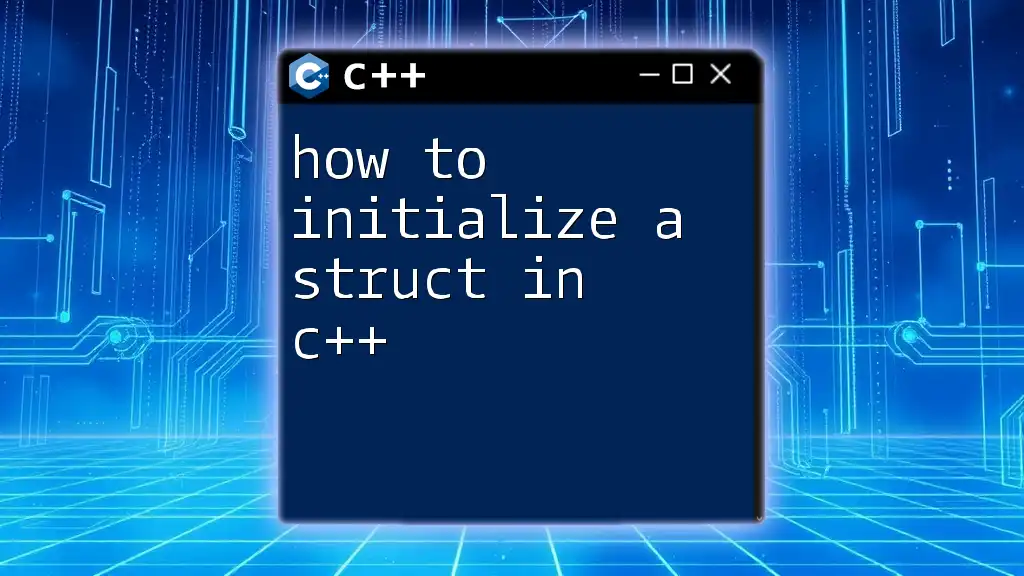
Important Considerations
Performance Implications
Although `size()` and `length()` are very efficient in terms of performance, it is crucial to recognize that `strlen()` will always traverse the entire C-style string, making it significantly less efficient for larger strings. Using `std::string` is generally recommended due to its optimized methods.
Safe Usage Practices
When working with strings in C++, keep the following in mind:
- Always prefer `std::string` over C-style strings to prevent buffer overflow and memory leak issues.
- When passing strings, be cautious with the possibility of empty strings and null pointers.
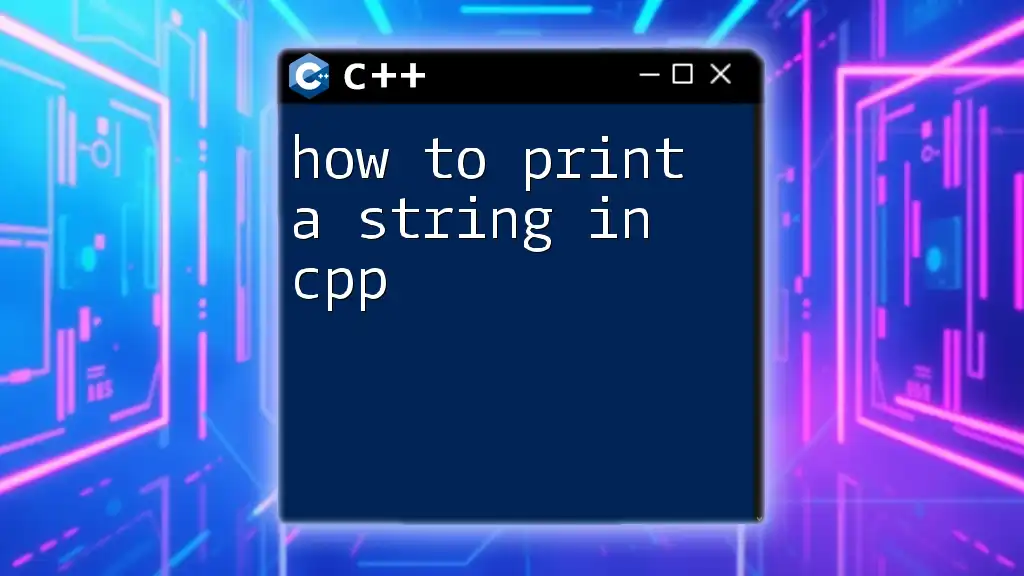
Conclusion
In summary, knowing how to find size of a string in C++ is fundamental for effective string manipulation. By utilizing `size()` and `length()` methods on `std::string`, or `strlen()` on C-style strings, developers can confidently manage string lengths. Whether working with modern or older code bases, understanding these functions will enhance your string handling capabilities in C++.