In C++, you can find the length of a static array using the expression `sizeof(array) / sizeof(array[0])`, which calculates the total size of the array in bytes divided by the size of one element.
Here's a code snippet demonstrating this:
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int length = sizeof(arr) / sizeof(arr[0]);
std::cout << "Length of the array: " << length << std::endl;
return 0;
}
What Is Array Length?
In C++, the term array length refers to the number of elements held in an array. Knowing the length of an array is critical for a variety of reasons:
- Memory Management: Understanding array length helps prevent overflow and ensures efficient use of memory.
- Avoiding Errors: Many runtime errors occur due to improper handling of array indices. Knowing the length helps to mitigate this risk.
Common Misconceptions
A common misconception is to confuse array length with the actual size (in bytes) of the array. Always remember that:
- Array Length = Number of Elements
- Array Size = Length of the Array × Size of Each Element
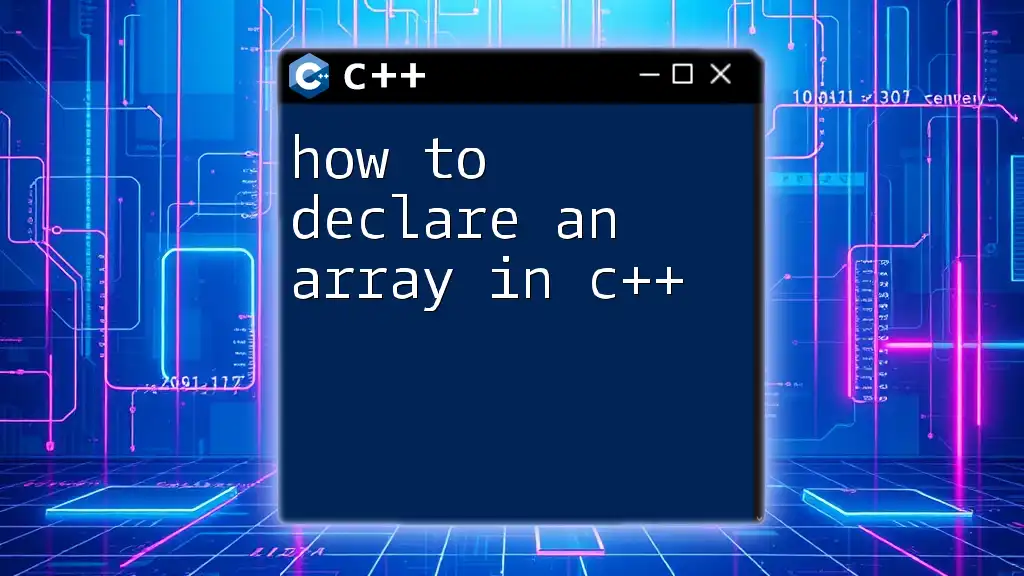
How to Find Length of an Array in C++
Using `sizeof` Operator
One of the easiest and most efficient ways to find the length of an array in C++ is by using the `sizeof` operator. This operator returns the total size of a type or variable in bytes. Here's how you can effectively utilize it:
int arr[10];
int length = sizeof(arr) / sizeof(arr[0]); // length will be 10
Explanation:
- `sizeof(arr)` gives you the total size of the array in bytes.
- `sizeof(arr[0])` returns the size of a single element.
- Dividing the two provides the total number of elements in the array.
This method is efficient but note that it only works for static arrays. If you pass an array to a function, it decays to a pointer, losing this length information.
Manual Counting in Loops
In some cases, you might consider finding the length manually using a loop. This method is especially useful for dynamically sized arrays or arrays where the end condition is not explicitly defined:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int length = 0; // Initialize length
for (int i = 0; arr[i] != '\0'; i++) {
length++; // Increment length for each element
}
cout << "Length: " << length << endl; // Output will depend on manual end condition
return 0;
}
IMPORTANT: This method has downsides. It relies on a specific termination condition (in this case, checking if the element is `'\0'`), which can lead to errors if not correctly defined or if the array is not properly terminated.
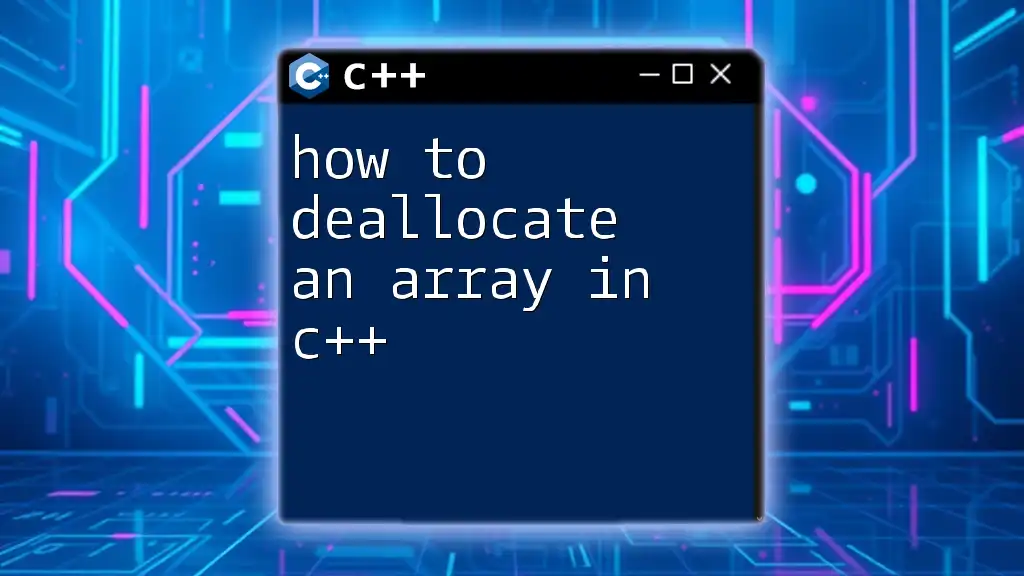
Pros and Cons of Different Methods
Understanding the pros and cons of the various methods for calculating array length can help you choose the best approach based on the context.
-
Using `sizeof` Operator:
- Pros: Fast, no loop is needed, efficient for static arrays.
- Cons: Does not work when arrays are passed to functions (decays to pointers).
-
Manual Counting:
- Pros: Useful in special cases where lengths are not easily determined.
- Cons: Prone to errors and less efficient, especially for large arrays.
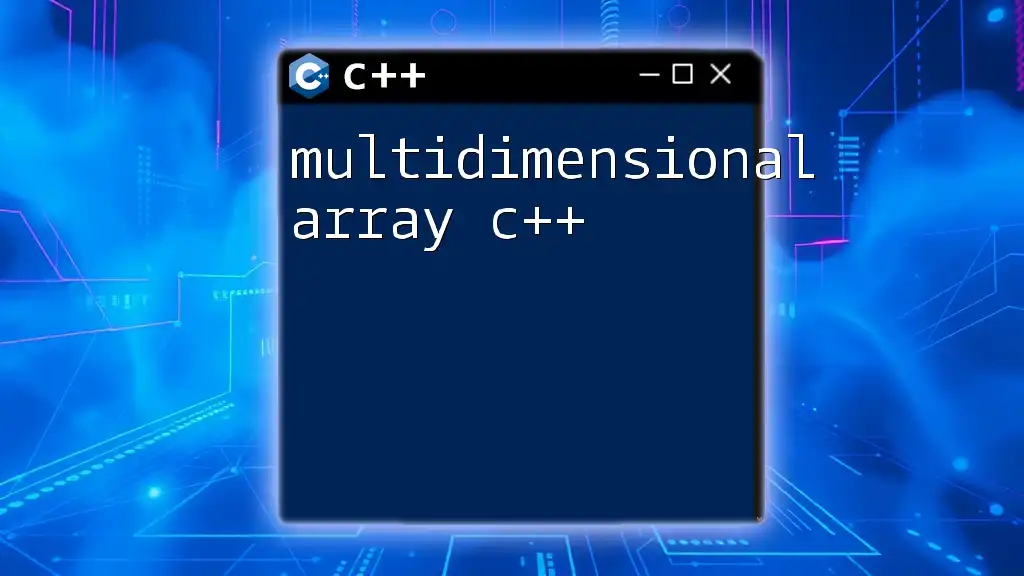
C++ Standard Library Functions
C++ provides various functions that can help manage arrays effectively. While there are no built-in functions to obtain the size of a native array, understanding library alternatives can enhance how you handle data.
A Closer Look at STL Containers
Utilizing `std::vector` from the Standard Template Library (STL) is a great alternative for managing dynamic arrays:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << "Length of vector: " << vec.size() << std::endl;
return 0;
}
Advantages of using vectors:
- Vectors automatically manage their size.
- They provide useful member functions like `.size()` to retrieve the number of elements easily.
- Unlike static arrays, vectors can grow and shrink dynamically, which adds flexibility to your programs.
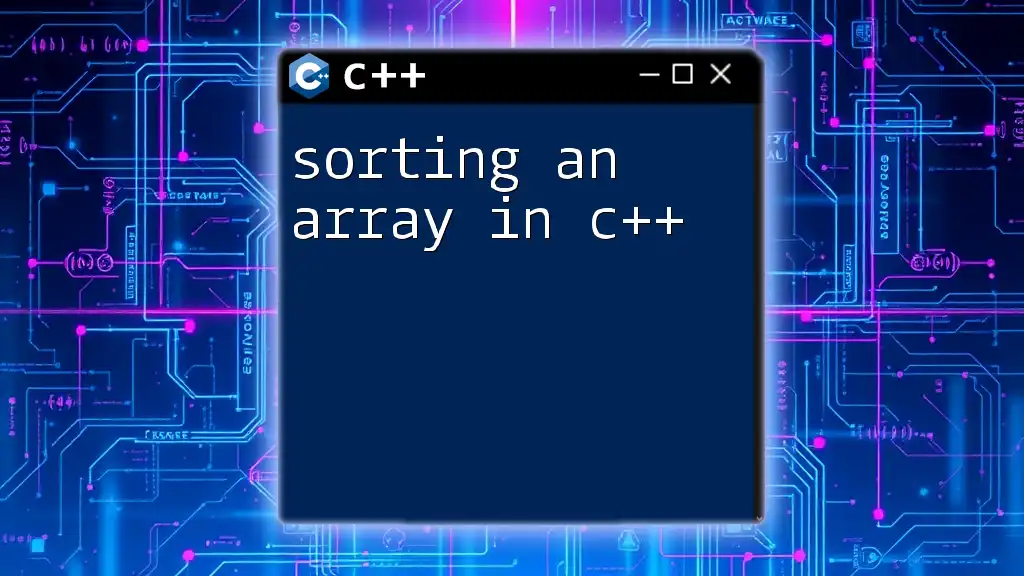
Conclusion
In summary, knowing how to find the length of an array in C++ is crucial for maintaining memory safety and efficient coding practices. Depending on your use case—whether you're working with static arrays or dynamic data—there are multiple methods available.
Using the `sizeof` operator is a quick and efficient way for static arrays, while manual counting can be applied in more tailored situations. However, for modern C++ programming, leveraging STL containers like `std::vector` simplifies many challenges associated with managing array lengths and increases your code's maintainability.
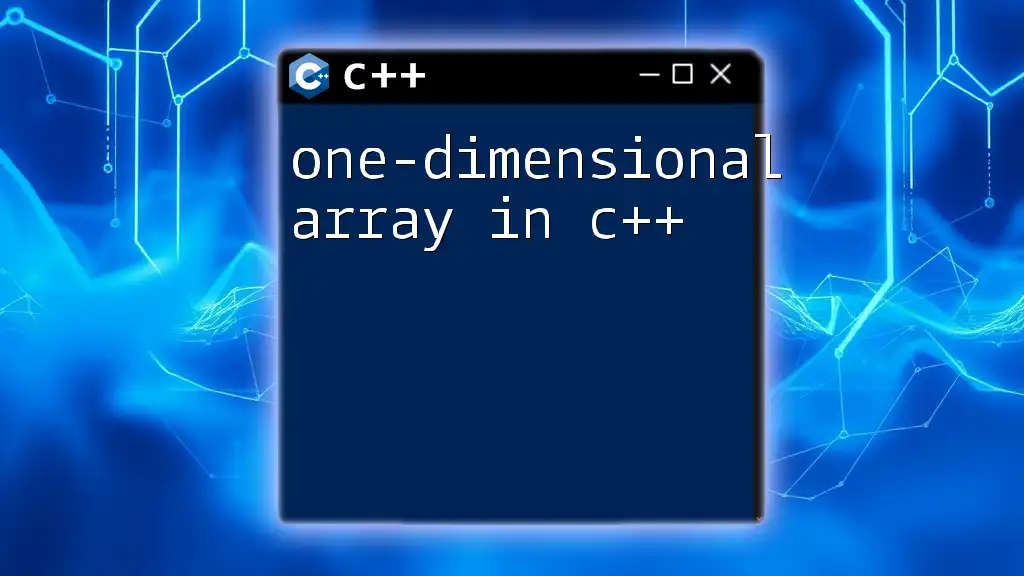
Frequently Asked Questions (FAQ)
How to Get Length of Array in C++?
To quickly find the length of an array, you can use the `sizeof` operator or switch to using `std::vector` for more dynamic use cases.
What Is the Best Way to Find Size of Array in C++?
For static arrays, the `sizeof` operator is preferred. In contrast, for arrays that may vary in size, consider using STL containers like `std::vector` for better flexibility and safety.
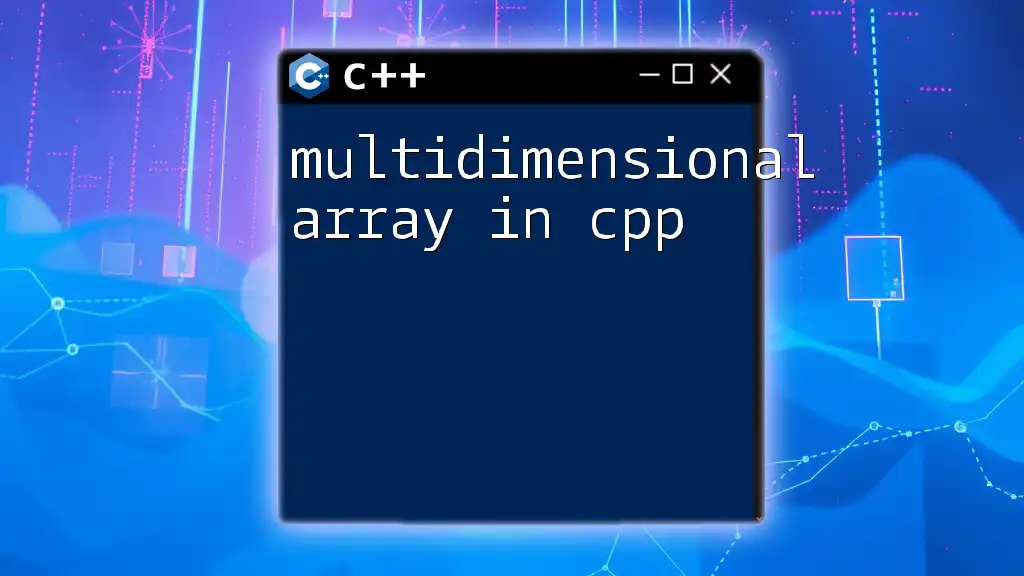
Additional Resources
For those looking to deepen their understanding of arrays and C++ in general, it is highly recommended to explore books and online tutorials dedicated to C++ programming. These resources can guide you through more advanced topics and techniques related to arrays and data structures.