To initialize a struct in C++, you can create a struct type, define a variable of that type, and assign values to its members either individually or using aggregate initialization.
Here's a code snippet demonstrating both methods:
#include <iostream>
using namespace std;
// Defining a struct
struct Point {
int x;
int y;
};
int main() {
// Method 1: Aggregate initialization
Point p1 = {10, 20};
// Method 2: Individual member assignment
Point p2;
p2.x = 30;
p2.y = 40;
cout << "Point p1: (" << p1.x << ", " << p1.y << ")\n";
cout << "Point p2: (" << p2.x << ", " << p2.y << ")\n";
return 0;
}
Understanding Structs in C++
Structs are fundamental building blocks in C++. They allow you to group related data together. A struct can contain various data types and can be seen as a lightweight class. One of the key differences between structs and classes is that structs default to public access for their members, while classes default to private. This makes structs an excellent choice for simple data representations.
Understanding how to properly initialize a struct in C++ is essential for preventing issues such as undefined behavior, which can lead to hard-to-debug errors.
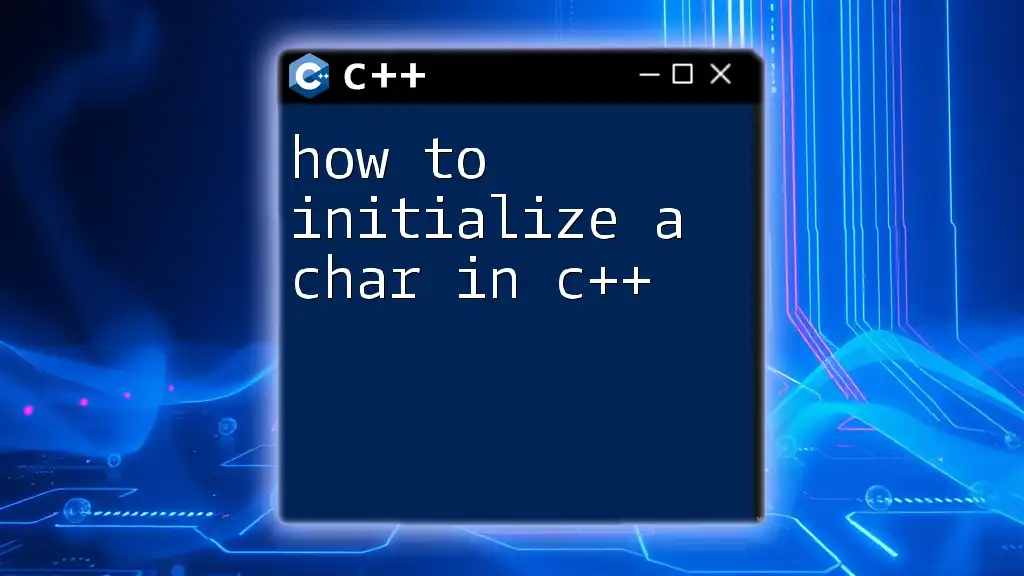
Basic Syntax of Structs
To define a struct, you use the `struct` keyword followed by the name of the struct and its members enclosed in braces. Here's a simple example of a struct that represents a person:
struct Person {
std::string name;
int age;
};
In this example, `Person` is the name of the struct, and it contains two members: `name` of type `std::string` and `age` of type `int`.
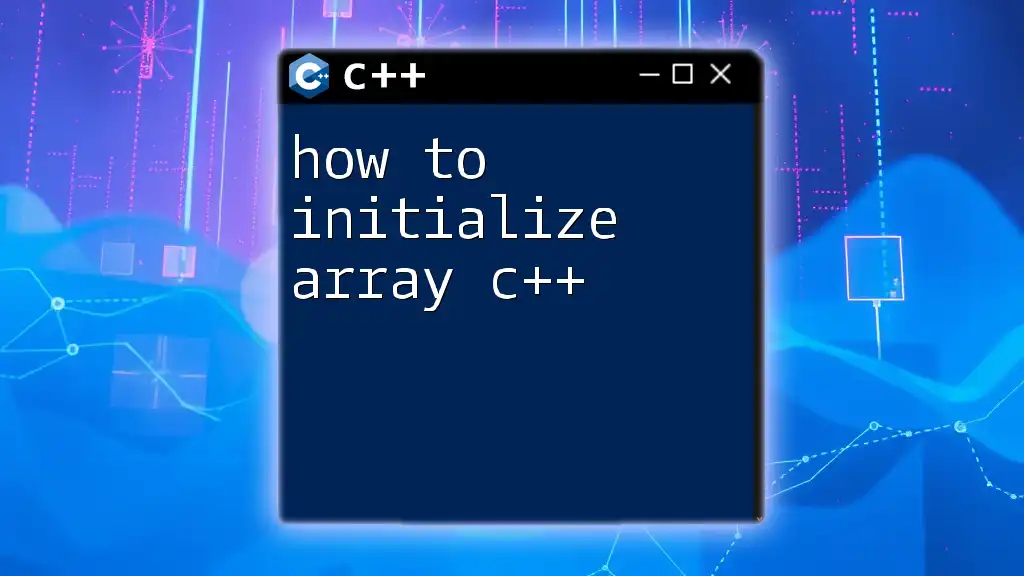
Different Methods to Initialize a Struct in C++
Using Default Constructor
When a struct is defined, you can create an instance of it without initializing its members. This is often referred to as "default initialization." However, it's important to remember that uninitialized members may lead to undefined behavior.
Example of default initialization:
Person person1; // Using default initialization
In this case, while `person1` is created successfully, the `name` and `age` members are not initialized, which could cause issues when you attempt to access them.
List Initialization (C++11 and later)
C++11 introduced the concept of list initialization, which allows for a clearer and safer method of initializing structs. This method uses braces `{}` to initialize members upon creating a new instance.
Here's an example:
Person person2{"Alice", 30}; // Using list initialization
With list initialization, you ensure that both `name` and `age` are initialized directly upon creation, which reduces the risk of undefined behavior. This method is particularly preferred for its syntactic clarity.
Designated Initializers (C++20)
The C++20 standard introduced designated initializers, which allow you to initialize specific members of a struct by name. This leads to more readable code, allowing you to initialize only the members you care about.
Here’s how you could use designated initializers:
Person person3 {.name = "Bob", .age = 25}; // Using designated initializers
This technique is especially useful when dealing with larger structs where you might only want to set certain values while leaving others at their default state.
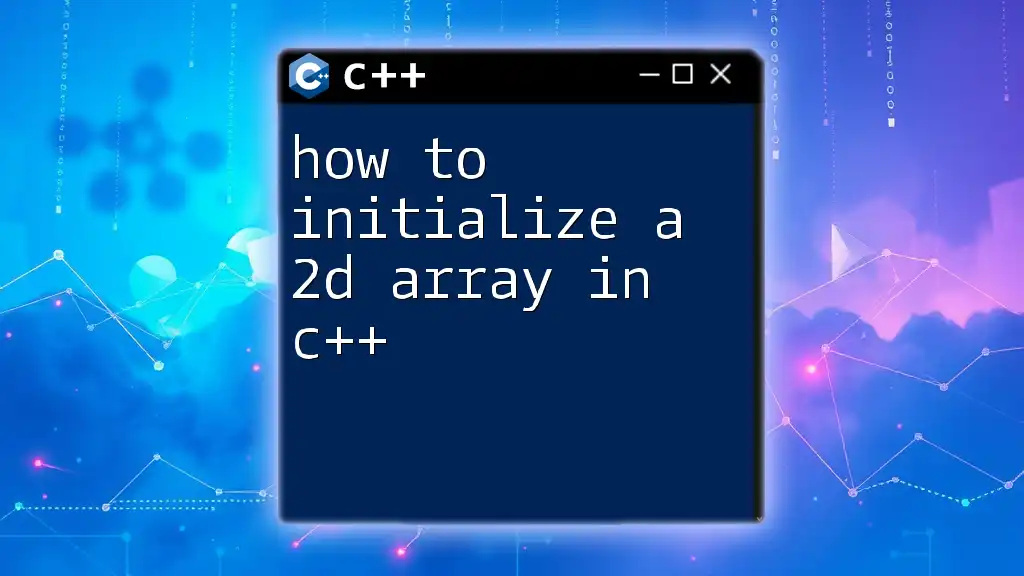
Initializing Structs with Member Functions
Sometimes, it is beneficial to provide a member function specifically for initializing your struct, especially when handling more complex data or needing to perform checks before initialization.
For example:
struct Person {
std::string name;
int age;
void initialize(const std::string& n, int a) {
name = n;
age = a;
}
};
Person person4;
person4.initialize("Charlie", 40);
In this example, we create a `initialize` method within the `Person` struct, which allows for cleaner and more controlled initialization of member variables.
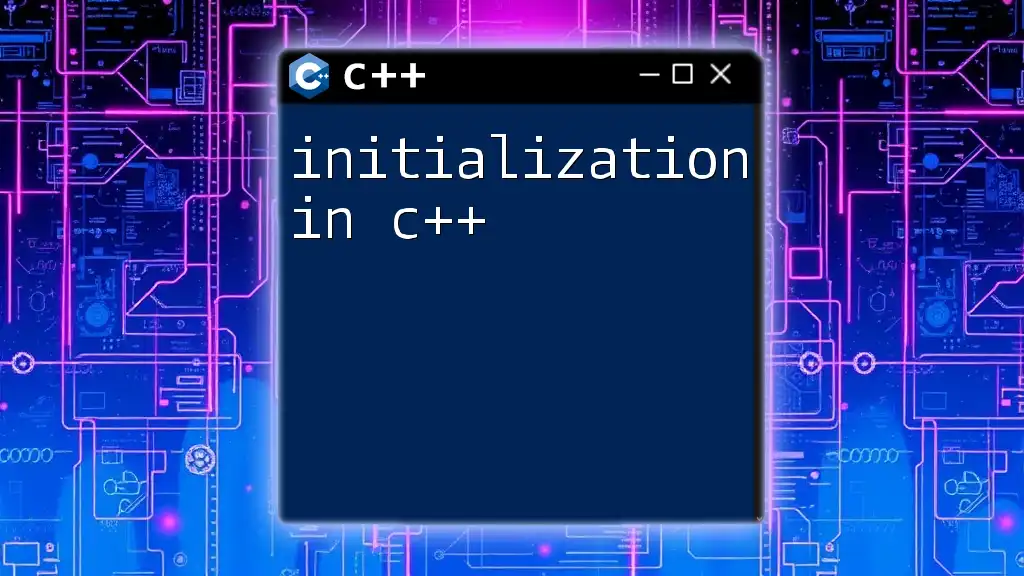
Common Mistakes When Initializing Structs
Common pitfalls often arise from failing to initialize struct members or trying to use uninitialized members. This can lead to undefined behavior or unexpected results in your program.
For instance:
Person personError; // Uninitialized
std::cout << personError.age; // Undefined behavior
To prevent such issues, always ensure that your structs are initialized properly. Here’s an example of a corrected initialization:
Person personCorrect{"Dave", 45}; // Properly initialized
Key Takeaway
Always remember that uninitialized memory can lead to undefined behavior. Use techniques like list initialization or designated initializers to eliminate ambiguities.
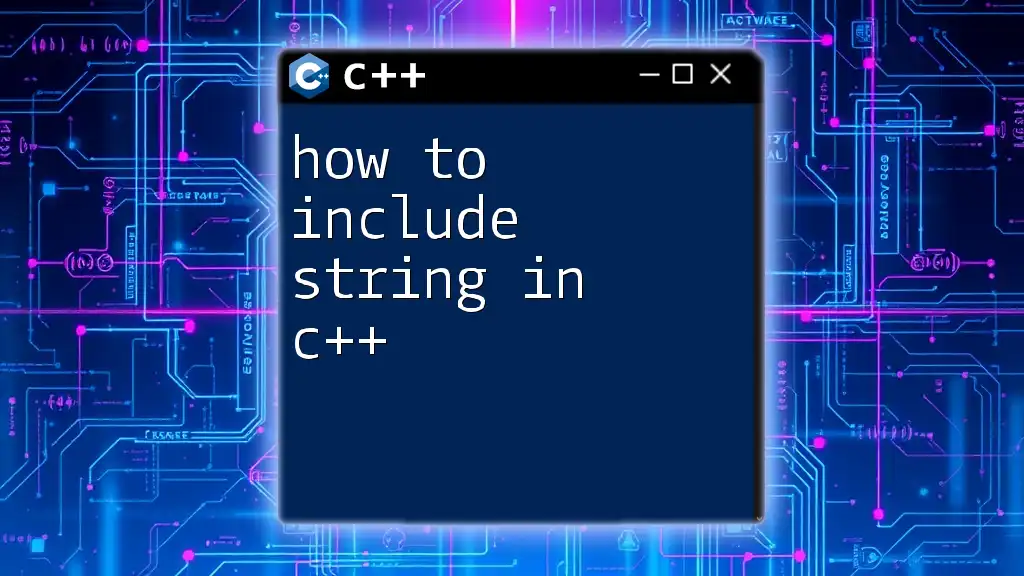
Practical Applications of Structs in C++
Structs are widely used in various applications, from video games to system design. They provide an efficient way to bundle together several related values into one package.
For example, in a game development scenario, you may have a `Vector2` struct to represent coordinates in 2D space:
struct Vector2 {
float x;
float y;
Vector2(float xVal, float yVal) : x(xVal), y(yVal) {}
};
This struct allows for easy manipulation and representation of 2D points in a game environment, solidifying the struct's applicability in real-world contexts.
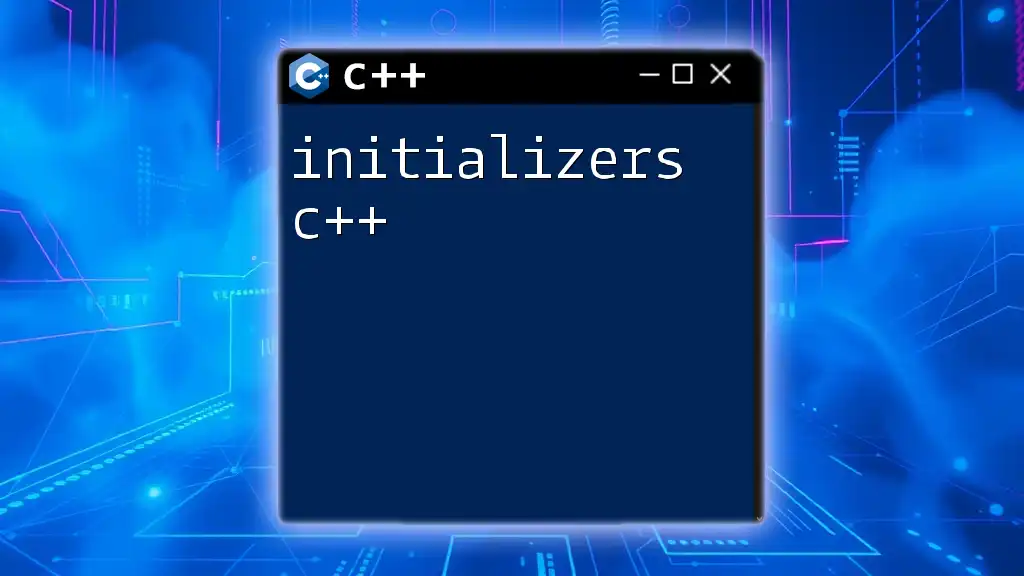
Conclusion
In conclusion, knowing how to initialize a struct in C++ is key to effective programming in the language. Various initialization methods are available, including default initialization, list initialization, and designated initializers. Each of these techniques offers unique benefits that contribute to safer and more readable code.
Practicing these methods will enhance your programming skills and help build robust applications using C++. If you're interested in deepening your understanding of C++ struct initialization and other related topics, consider subscribing for more insightful content.
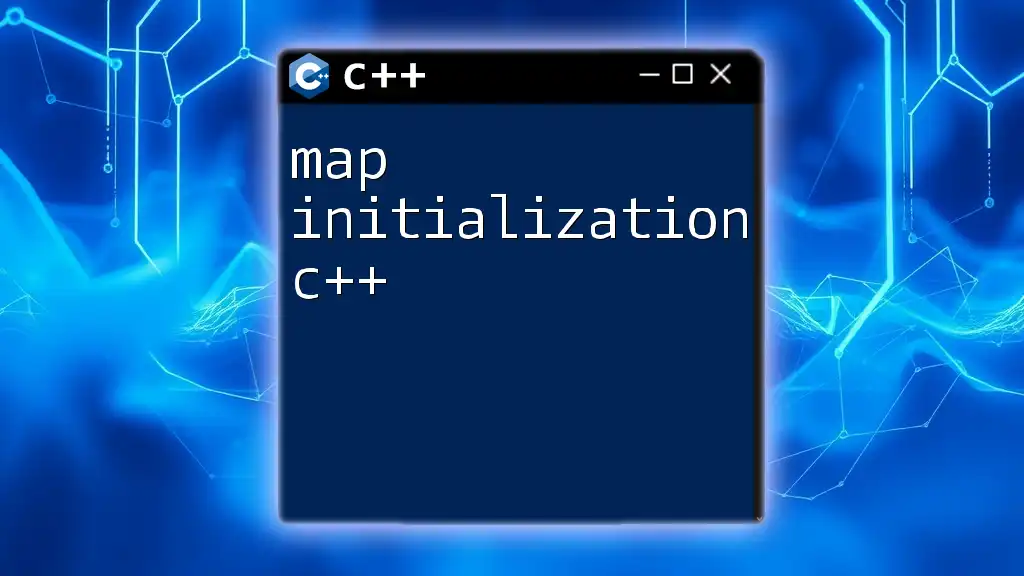
Additional Resources
For those looking to further explore the topic, be sure to check C++ documentation and online courses that provide detailed insights into struct usage and best practices. Happy coding!