In C++, you can initialize a `char` variable by assigning it a single character enclosed in single quotes, as demonstrated below:
char myChar = 'A';
Understanding Characters in C++
What is a `char`?
In C++, a `char` is a data type specifically designed to store single characters. Each `char` typically occupies one byte of memory, which can represent a variety of characters using different character encoding systems such as ASCII or Unicode. The `char` type is a fundamental building block for text processing in C++ programming.
Differences Between `char` and Other Data Types
Unlike integers that represent whole numbers, or strings which are arrays of `char`, a `char` is restricted to a single character. This distinction is important for memory management: a single `char` consumes less memory than a string. For example, a string 'Hello' takes up more space than a single character 'H'. Understanding these differences is vital for efficient programming in C++.
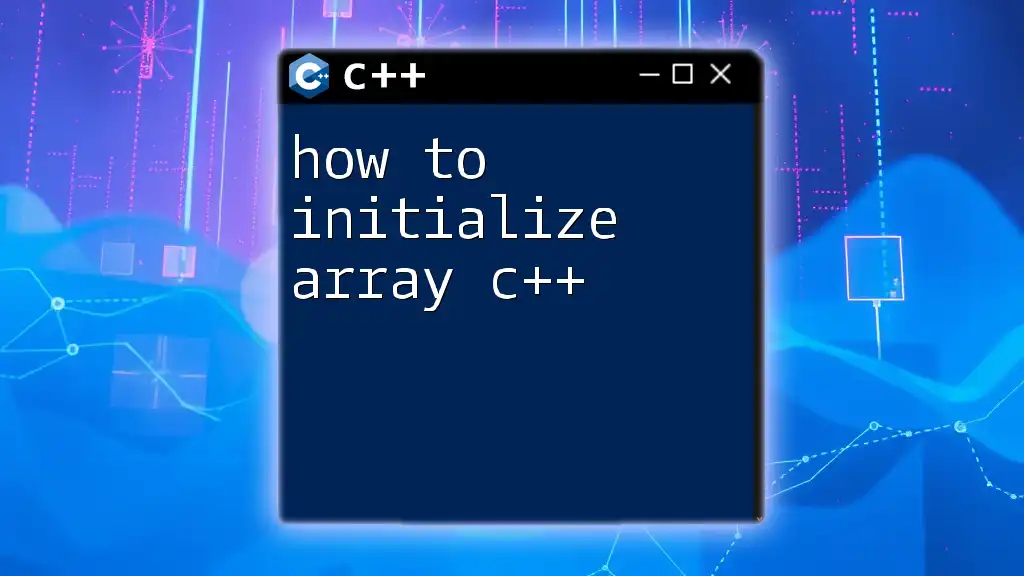
Basic Initialization Techniques
Initialising a Single Character
To declare and initialize a single character, you use single quotes. Here's how it works:
char letter = 'A';
In this example, the variable `letter` is initialized with the character 'A'. The use of single quotes is essential, as double quotes would indicate a string, leading to a compilation error.
Initialising Multiple Characters (Arrays of Char)
When you want to initialize multiple characters, you can create an array of `char`. Here's an example:
char name[5] = {'E', 'l', 'l', 'o', '\0'};
In this snippet, `name` is an array of characters that represents the string "Hello". The null terminator (`'\0'`) is necessary to signify the end of the string in C++. Without it, functions that work with strings may produce unexpected behaviors.
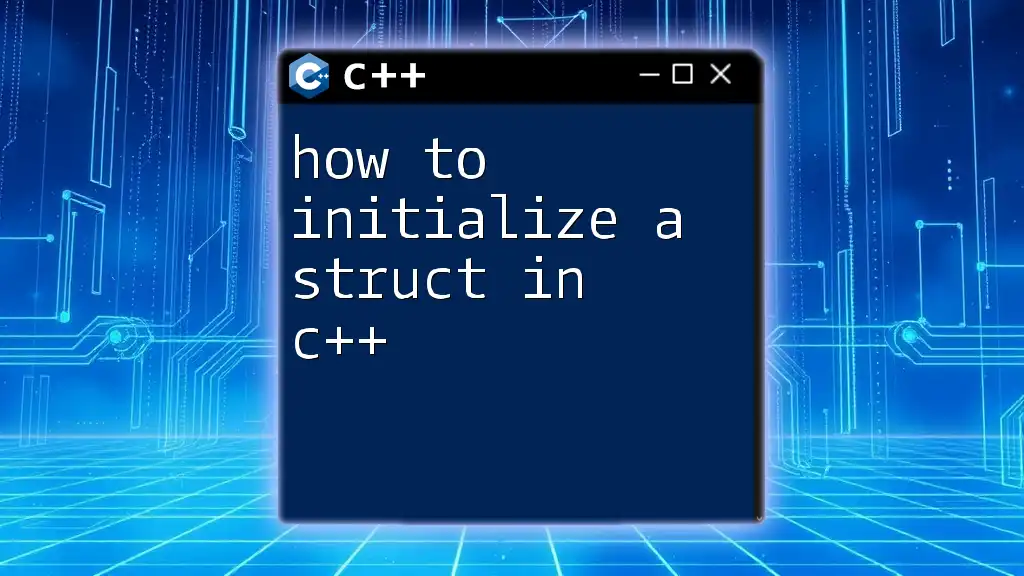
Advanced Initialization Techniques
Using Strings to Initialize Char Arrays
In C++, you can initialize a `char` array from a string easily. Here’s how to do that:
#include <string>
std::string str = "Hello";
const char* cStr = str.c_str();
The method `c_str()` converts the C++ string to a C-style string, which is simply a pointer to the array of characters. Understanding this conversion is important for ensuring proper string handling in older C-style or even some C++ libraries.
Using Initializer Lists
Initializer lists can improve readability when working with `char` arrays. Here’s an example:
char vowels[] = {'a', 'e', 'i', 'o', 'u'};
This technique allows you to easily visualize the data you're storing, making your code more maintainable. It's a great practice to adopt for making character data clear at a glance.
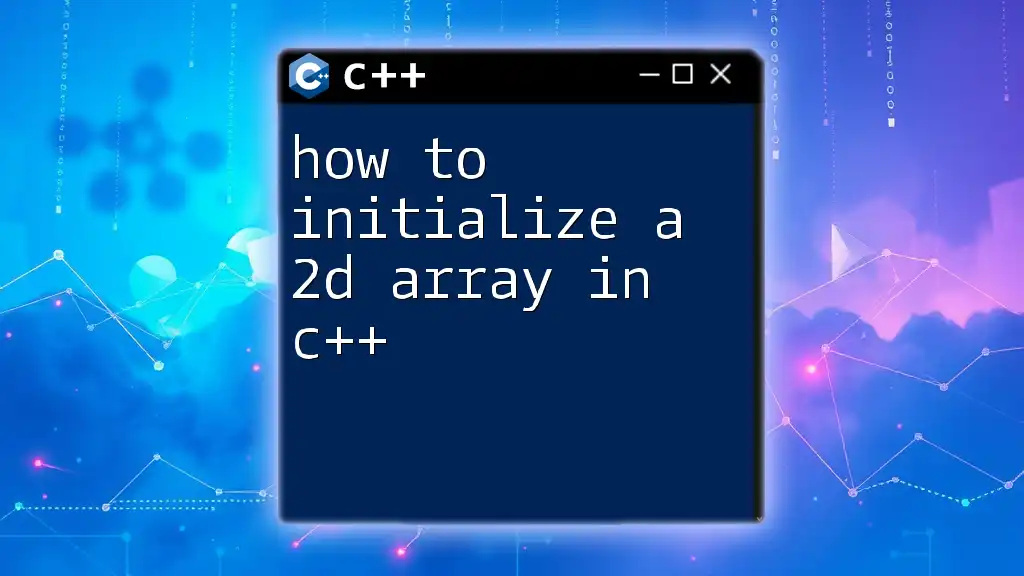
Common Pitfalls
Uninitialized `char` Variables
One of the major risks when working with `char` variables is using uninitialized variables. Consider the following code:
char uninitializedChar;
std::cout << uninitializedChar; // Undefined behavior
Since `uninitializedChar` has not been set to any specific value, printing it will lead to undefined behavior. Always ensure you're initializing your variables properly to avoid bugs that can be difficult to track down.
Using Wrong Quotes
It's crucial to distinguish between single quotes and double quotes. An incorrect usage can lead to compilation errors:
char wrongInitialization = "a"; // Error: should use single quotes
In this case, the compiler expects a string due to the double quotes, resulting in a type mismatch. Always remember to use single quotes for individual `char` values.
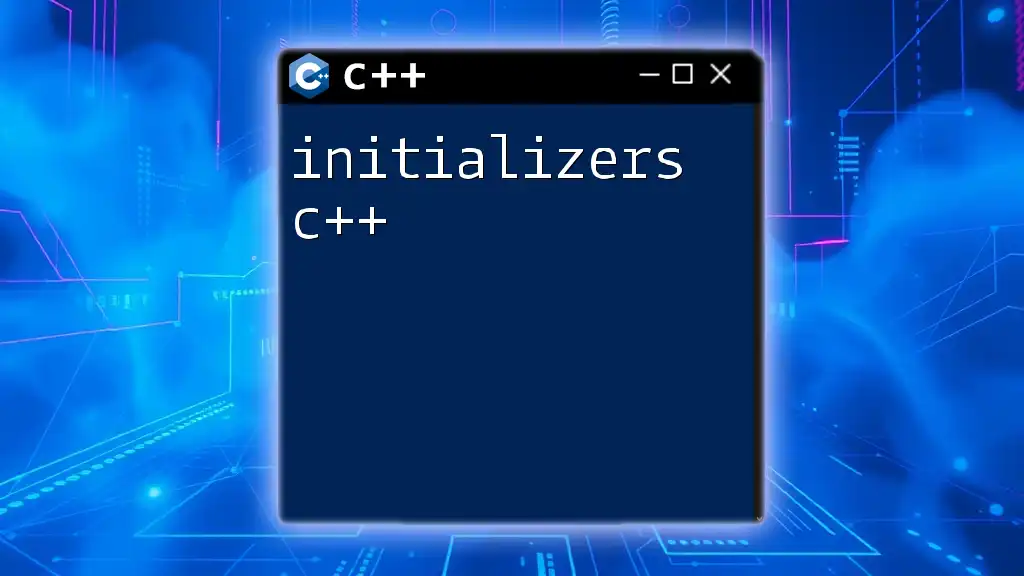
Special Use Cases
Initializing `char` with Escape Sequences
In C++, you can use escape sequences to initialize `char` variables as well. Here are examples:
char newline = '\n';
char tab = '\t';
Here, `newline` is an escape sequence that denotes a line break, while `tab` represents a horizontal tab. These can be quite useful when formatting output.
Initializing Multi-dimensional Char Arrays
C++ also allows you to create multi-dimensional `char` arrays. For example:
char grid[2][3] = {{'A', 'B', 'C'}, {'D', 'E', 'F'}};
This `grid` is a 2D array that can be used for various applications, such as creating matrices. Understanding how to initialize these will enable complex data structures in your programs.
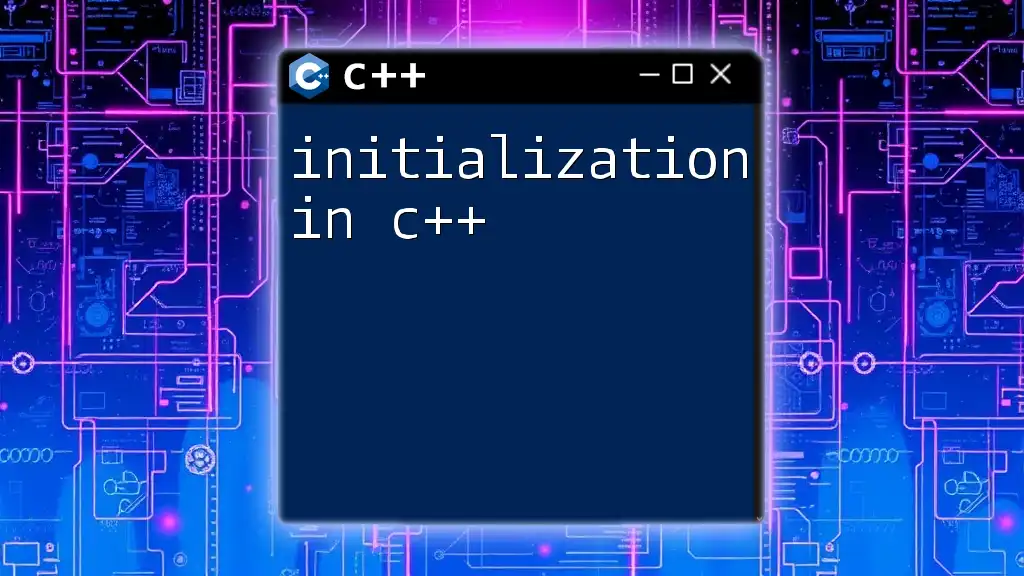
Conclusion
Knowing how to initialize a char in C++ is a fundamental skill that forms the foundation of many programming tasks. By mastering both basic and advanced techniques, as well as understanding common pitfalls, you can ensure that your character handling in C++ is robust and efficient. Practice is essential, so take the time to experiment with these various initialization methods and see how they can improve your coding proficiency.