In C++, to throw an `invalid_argument` exception, you can use the `throw` keyword along with the `std::invalid_argument` class, which typically is included from the `<stdexcept>` header.
Here’s a code snippet demonstrating this:
#include <stdexcept>
void checkValue(int value) {
if (value < 0) {
throw std::invalid_argument("Value must be non-negative");
}
}
Understanding Exceptions in C++
What are Exceptions?
Exceptions in C++ provide a way to react to exceptional circumstances (like runtime errors) in programs by using throwing and catching mechanisms. They allow developers to manage errors and maintain the flow of execution without unpredictable crashes.
The Standard Library and Exception Types
C++ standard library supports various exception types, each serving specific needs. `std::invalid_argument` is one of these exceptions, designed to signal that an argument passed to a function is outside of the expected range or otherwise invalid. This type of exception is particularly useful when you want to ensure that the values your functions deal with are valid and to provide meaningful error messages when issues arise.
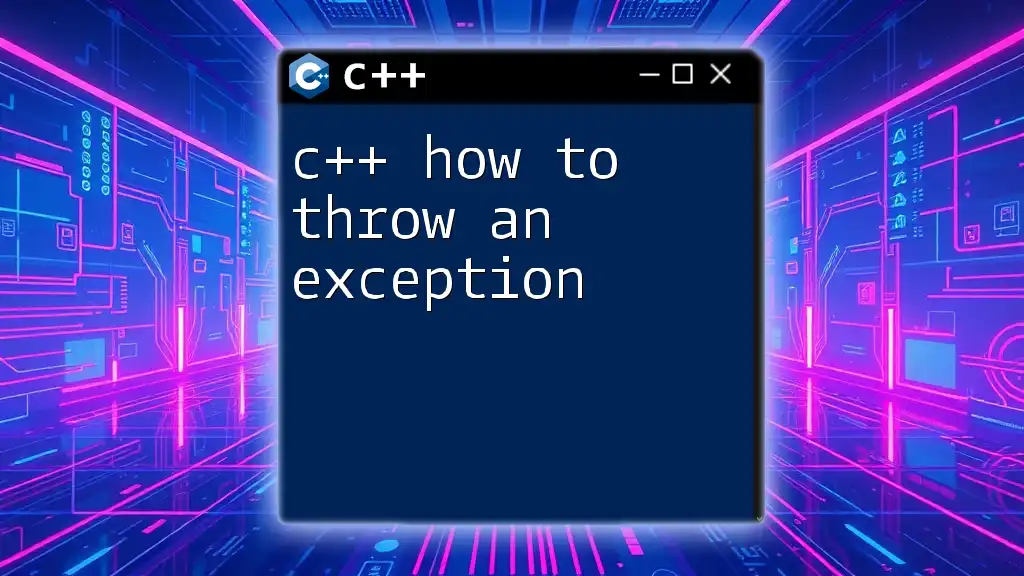
Throwing Invalid Argument Exceptions
When to Throw an Invalid Argument Exception
Throwing an `std::invalid_argument` exception is appropriate in various scenarios, especially when you have functions that require parameters that need to adhere to specific constraints. For instance, a function expecting a positive integer as input should throw an exception if it receives a negative integer. Validating inputs is crucial for preventing unexpected behavior within the program.
Consider a function that processes user data where an invalid age (e.g., negative numbers) could lead to logical errors downstream. In such cases, throwing an exception helps identify problems early in the execution flow.
Syntax for Throwing an Invalid Argument Exception
Throwing an `std::invalid_argument` exception is straightforward. The basic syntax for throwing this exception consists of using the `throw` keyword followed by the exception type and the error message description.
throw std::invalid_argument("Error message");
In this syntax:
- `throw` indicates that an exception is being raised.
- `std::invalid_argument` is the type of exception, indicating the nature of the error.
- The string provides a detailed error message, helping the developer understand the context of the problem.
Implementing a Function that Throws std::invalid_argument
To demonstrate how to throw an `std::invalid_argument` exception, consider the following function that validates an age input:
#include <stdexcept>
#include <string>
void validateAge(int age) {
if (age < 0) {
throw std::invalid_argument("Age cannot be negative.");
}
// Further processing...
}
In this snippet:
- The `validateAge` function takes an integer parameter `age`.
- If `age` is negative, the function throws an `std::invalid_argument` exception with a relevant error message.
- This ensures that any external calls to `validateAge` will handle the invalid input appropriately.
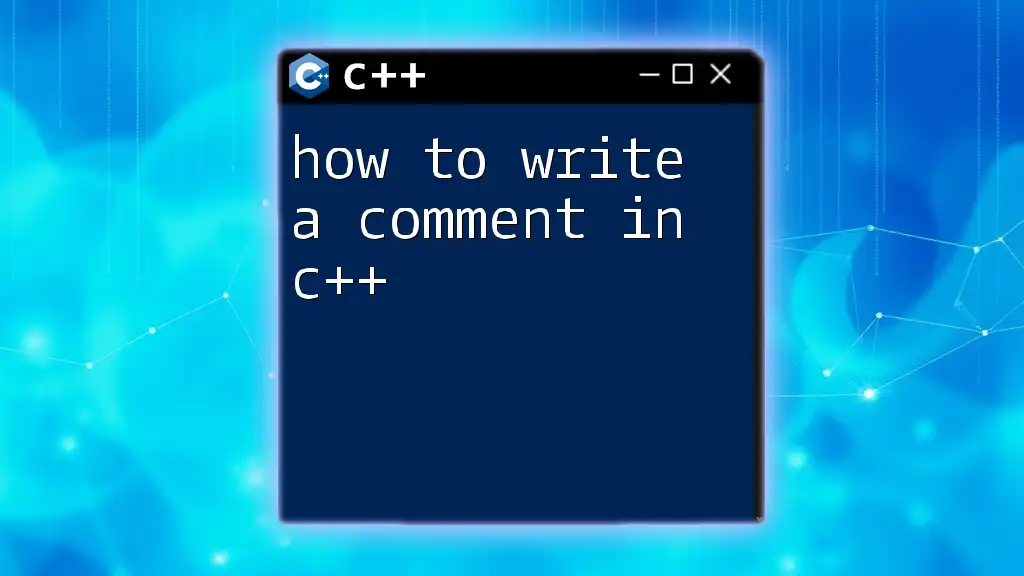
Catching Invalid Argument Exceptions
Basics of Exception Handling with Try-Catch Blocks
In C++, you can handle exceptions gracefully using try-catch blocks. This structure allows you to attempt executing code that might throw an exception. If an exception occurs, you can catch it and respond accordingly, preventing your application from terminating unexpectedly.
Example of Catching an Invalid Argument Exception
Here is an example of how to catch an `std::invalid_argument` exception:
#include <iostream>
int main() {
try {
validateAge(-5);
} catch (const std::invalid_argument& e) {
std::cerr << "Caught an invalid argument exception: " << e.what() << std::endl;
}
return 0;
}
In this example:
- The `validateAge` function is called within a `try` block.
- If the function throws an `std::invalid_argument`, the catch block executes.
- The error message produced by `e.what()` is displayed using `std::cerr`, providing useful feedback for debugging.
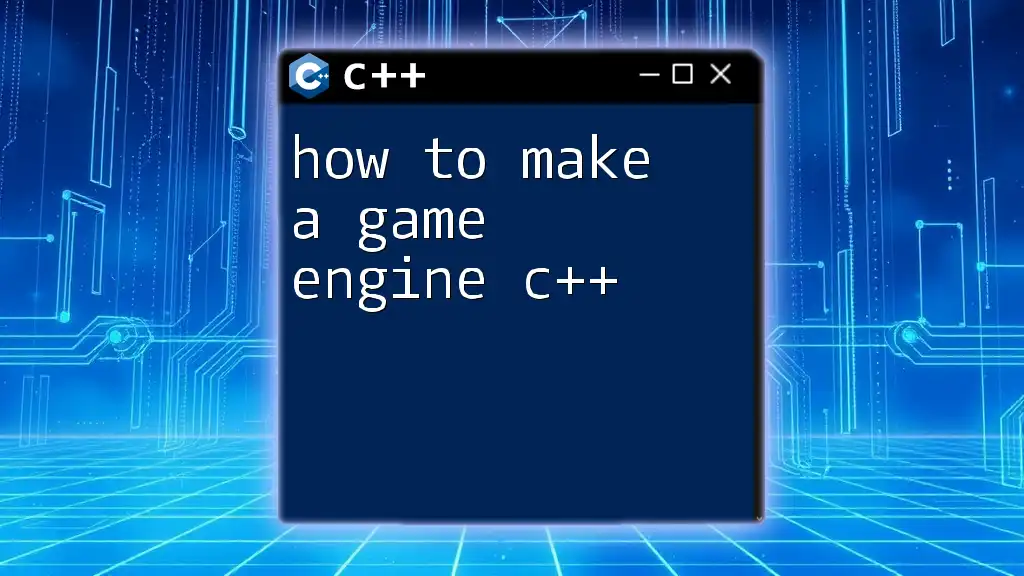
Best Practices for Using Invalid Argument Exceptions
Validation Before Throwing Exceptions
Before throwing an exception, ensure that necessary validations are performed rigorously. This could involve using assertions, checking parameters against valid ranges, or even conducting more complex validation if needed. A strong emphasis on input validation leads to cleaner and more reliable code.
Documenting Exceptions in Code
Always document the exceptions that a function might throw within its comments or documentation. This practice enhances the maintainability of your code, enabling future developers (and yourself) to understand the expected behavior. Using comments like the following can help:
/**
* Validates the age.
* Throws std::invalid_argument if age is negative.
*/
void validateAge(int age);
Performance Considerations
While exceptions are powerful, keep in mind that they can incur performance costs. If a function produces exceptions frequently in a performance-sensitive context, consider alternatives such as returning error codes or using optional types. Use exceptions judiciously, primarily for truly exceptional conditions that cannot be prevented through regular validation.
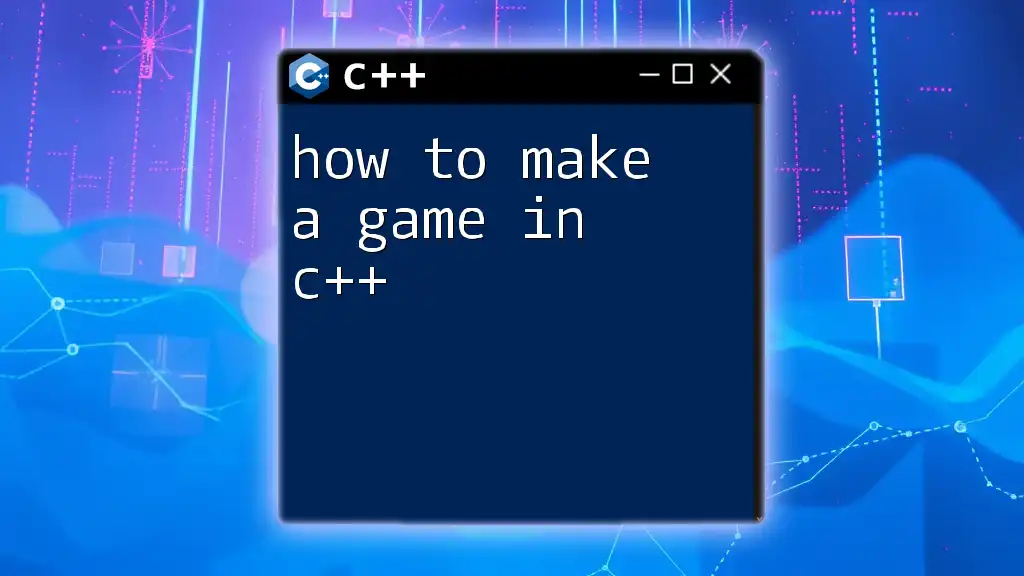
Conclusion
Learning how to throw an invalid argument exception in C++ is a fundamental skill that enhances your error handling capabilities. By throwing `std::invalid_argument`, you communicate issues clearly and maintain control over program execution—a crucial aspect of good software design. Always remember to validate your inputs meticulously and utilize exception handling constructs effectively to ensure the robustness of your code.
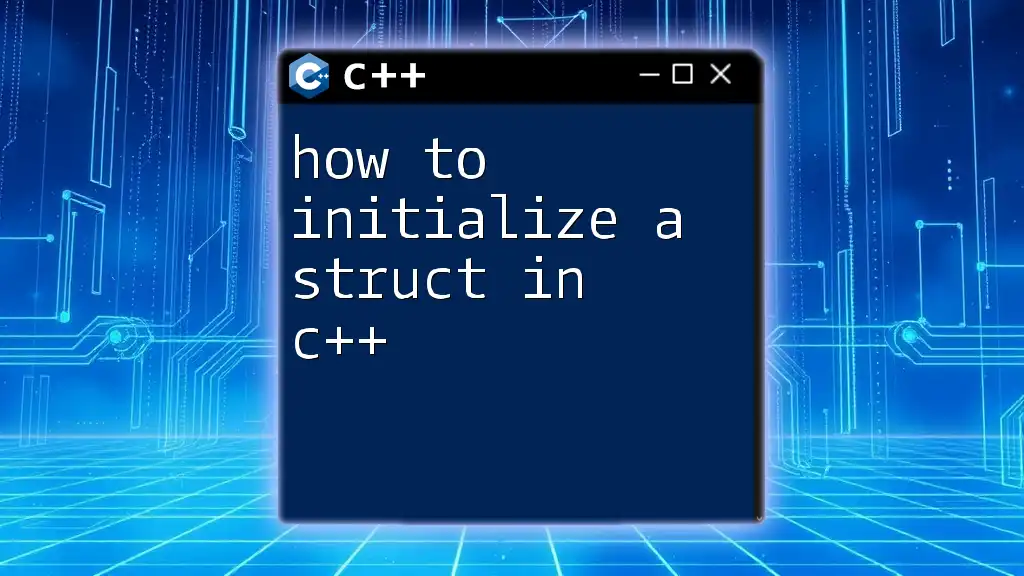
Additional Resources
For further reading, explore topics related to C++ exception handling in the standard library, deeper dives into the types of exceptions, and best practices for effective error management in larger applications.