In C++, you can catch all exceptions by using a catch block that specifies the base class `std::exception`, allowing you to handle any exception derived from it.
Here's a simple code snippet demonstrating this:
#include <iostream>
#include <exception>
int main() {
try {
// Code that may throw an exception
throw std::runtime_error("Error occurred");
} catch (const std::exception& e) {
std::cout << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
What Are Exceptions in C++?
In C++, exceptions represent a way to handle errors or unexpected behavior gracefully. Rather than abruptly terminating a program when something goes wrong, exceptions allow developers to define a response to such events. This is particularly crucial in complex applications where user experience and system robustness are paramount.
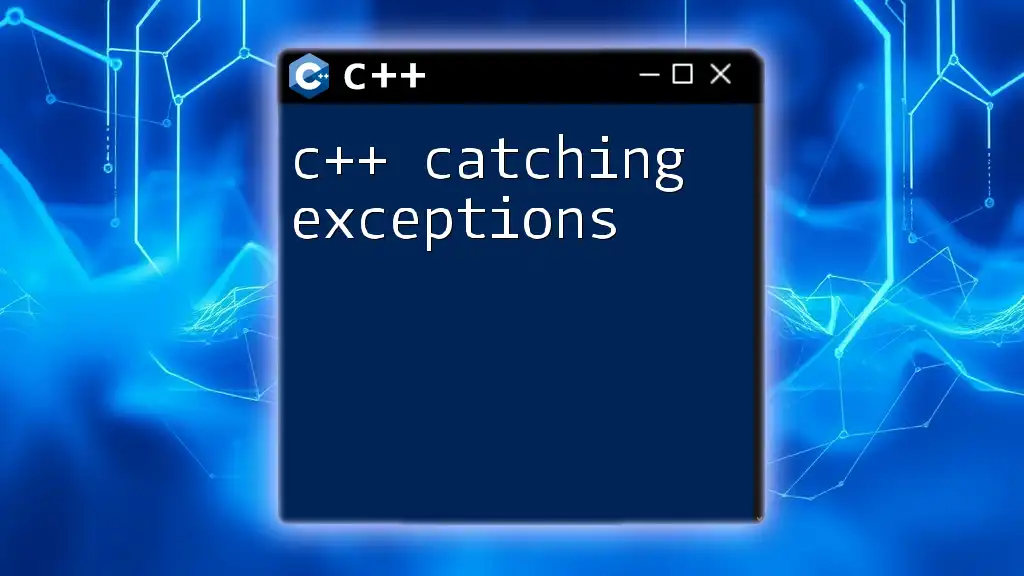
Why Use a Catch-All for Exceptions?
Implementing a catch-all mechanism for exceptions can be beneficial in scenarios where you may not have direct control over all potential error types. It acts as a safety net, ensuring that even unforeseen issues are addressed. This approach can be particularly useful in large systems, where various modules and components may generate different exceptions.
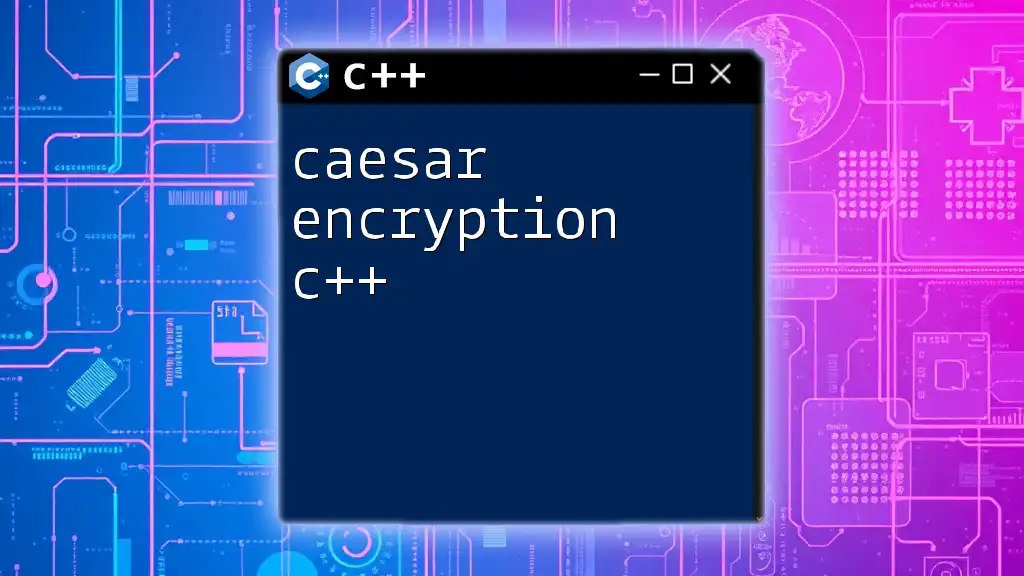
Understanding C++ Exception Handling
Key Components of Exception Handling
The core elements of exception handling in C++ include:
- try-catch blocks: Enclaves where code that might fail is executed (try) and where responses to exceptions are defined (catch).
- throw statement: The mechanism to signal that an error has occurred, allowing control to be transferred to a matching catch block.
How C++ Handles Exceptions
When an exception is thrown, C++ performs a process known as stack unwinding. This means it will safely clean up resources as it looks for a matching catch block, traversing up the call stack until it finds one.
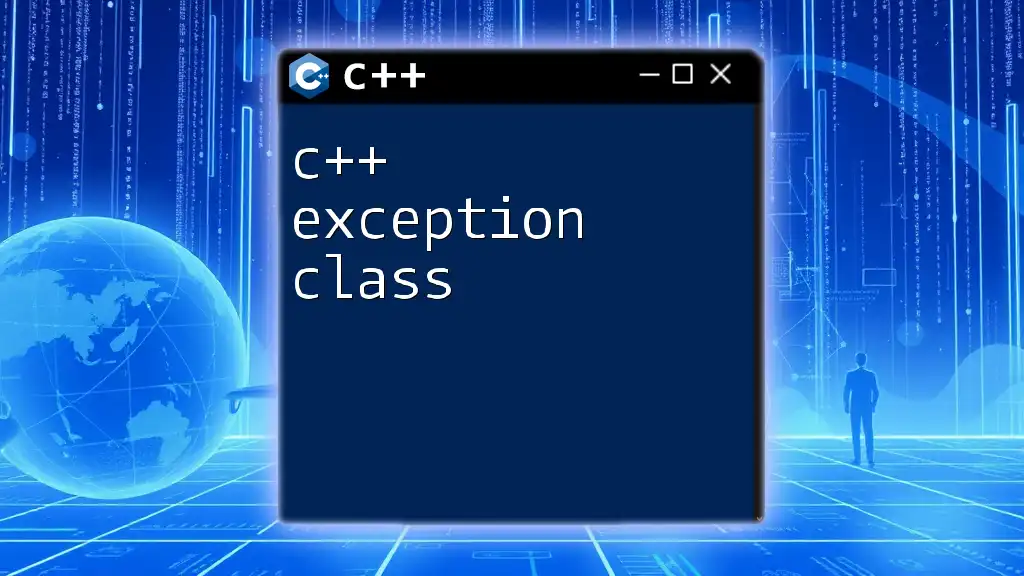
Catch All Exceptions in C++
What Does "Catch All" Mean in C++?
The phrase "catch all" in C++ refers to the ability to handle any exception that may occur without specifying the type. This is typically done using a catch block defined with `catch(...)`. By using this syntax, you can ensure that no exceptions escape your handling logic, which might lead to a program crash.
Using `catch(...)` to Catch All Exceptions
This catch-all block can be easily implemented. Here's an example:
try {
// Some risky code that may throw an exception
int result = riskyOperation();
} catch (...) {
std::cout << "Caught an unknown exception!" << std::endl;
}
In this example, if `riskyOperation()` throws any exception, the catch block will execute, providing a way to handle errors gracefully.
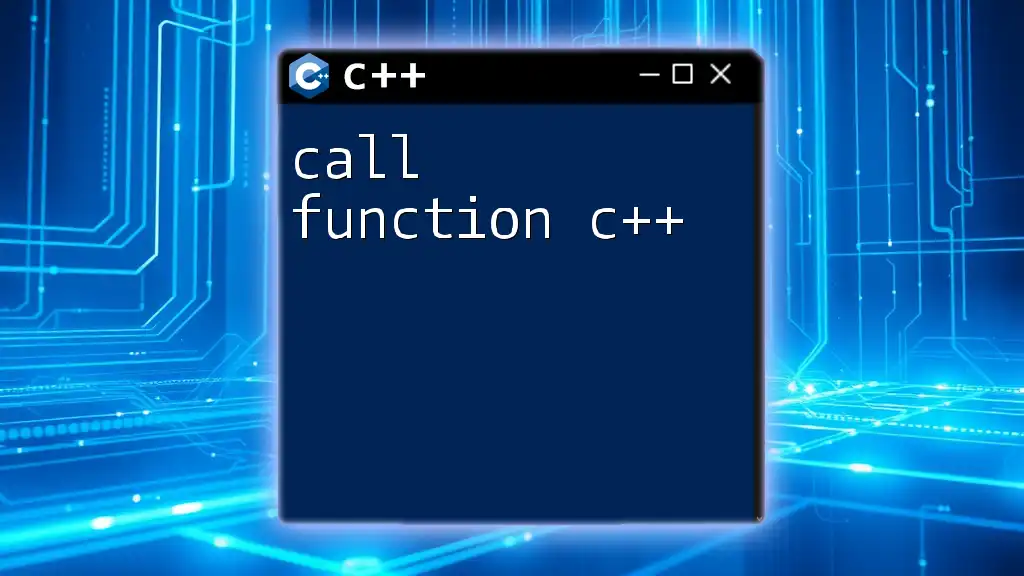
Best Practices for Catching All Exceptions
When to Use a Catch-All
Using a catch-all can be helpful in specific contexts, such as when dealing with third-party libraries where exceptions may not be well documented. Alternatively, it can serve as a final safety measure in a larger try-catch structure.
Avoiding Overuse of Catch-All
However, catch-all blocks should not be overused. Relying solely on catch-all can obscure the specific reasons why an error occurred, leading to poor debugging experiences. Instead, prioritize specific catch blocks that deal with known errors, reserving the catch-all for truly unexpected issues.
Logging and Reporting Exceptions
One crucial aspect of handling exceptions is logging. It's essential to track exceptions to understand failure patterns better. Here’s a quick example:
try {
// Some operation that may fail
} catch (...) {
log_exception("Caught an unknown exception!");
}
In this case, rather than merely notifying users, the program logs the exception message for further analysis.
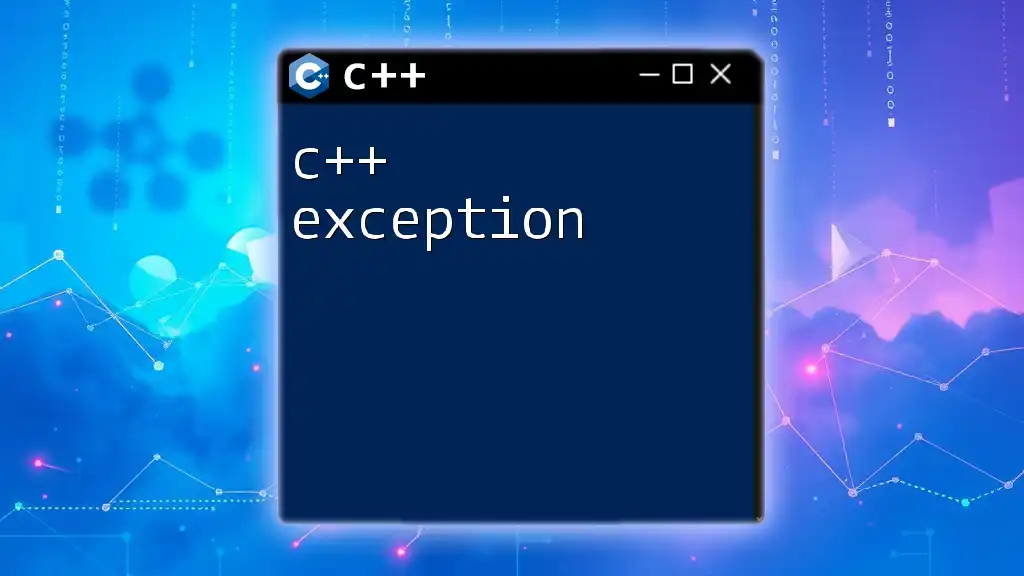
Additional Ways to Catch Exceptions
Using `catch` with Specific Exceptions
Although catch-all is useful, handling specific exceptions provides greater control and clarity. By defining multiple catch blocks, developers can customize responses based on the type of error:
try {
// code that may throw
} catch (const std::runtime_error& e) {
std::cout << "Caught runtime error: " << e.what() << std::endl;
} catch (const std::exception& e) {
std::cout << "Caught standard exception: " << e.what() << std::endl;
} catch (...) {
std::cout << "Caught an unknown exception!" << std::endl;
}
This approach ensures that clear messages are provided for known exception types, while still being able to handle unexpected cases.
Combining Catch Handlers with `catch(...)`
Combining specific and general handlers allows for robust error management. In the following example, specific exceptions are prioritized, ensuring known issues are adequately handled first:
try {
// Some code that may throw
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
} catch (...) {
std::cerr << "Caught an unknown error!" << std::endl;
}
Common Scenarios and Solutions
Using Catch-All in Real-World Applications
A common scenario where a catch-all can be vital is in file handling operations, where various types of errors can arise due to conditions beyond the developer's control:
try {
std::ifstream file("data.txt");
if (!file.is_open()) {
throw std::runtime_error("Unable to open file");
}
// Read file operations
} catch (...) {
std::cerr << "Failed to handle the file operation!" << std::endl;
}
Here, the catch-all block captures any types of failures during file operations, providing a simple way to ensure stability.
Handling Derived Exception Classes
In C++, exceptions can be hierarchical, allowing developers to throw and catch exceptions from a base class. This relationship impacts the way exceptions are handled:
class MyException : public std::exception {
const char* what() const noexcept override { return "My custom exception"; }
}
try {
throw MyException();
} catch (...) {
std::cout << "Caught a custom exception!" << std::endl;
}
In this example, the custom exception can be caught by a catch-all, but specific handling logic should be implemented for better error recognition.
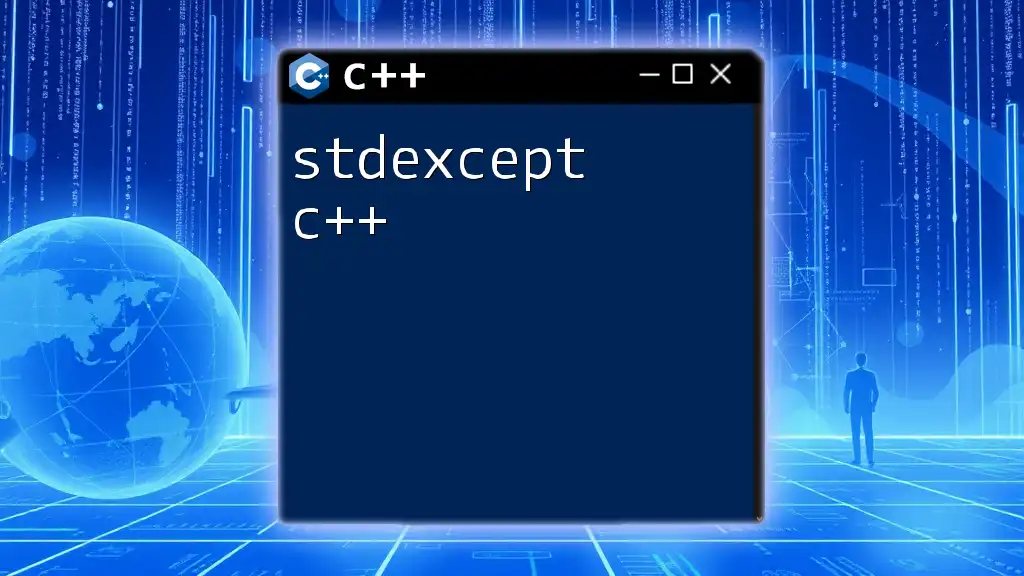
Conclusion
In summary, implementing a mechanism to catch all exceptions in C++ is an important aspect of error management. While the flexibility of `catch(...)` provides a robust way to handle unforeseen issues, it’s crucial to pair this approach with diligent specific exception handling. By adopting best practices such as logging and appropriate use of catch-all blocks, developers can significantly enhance the error handling capabilities of their applications.
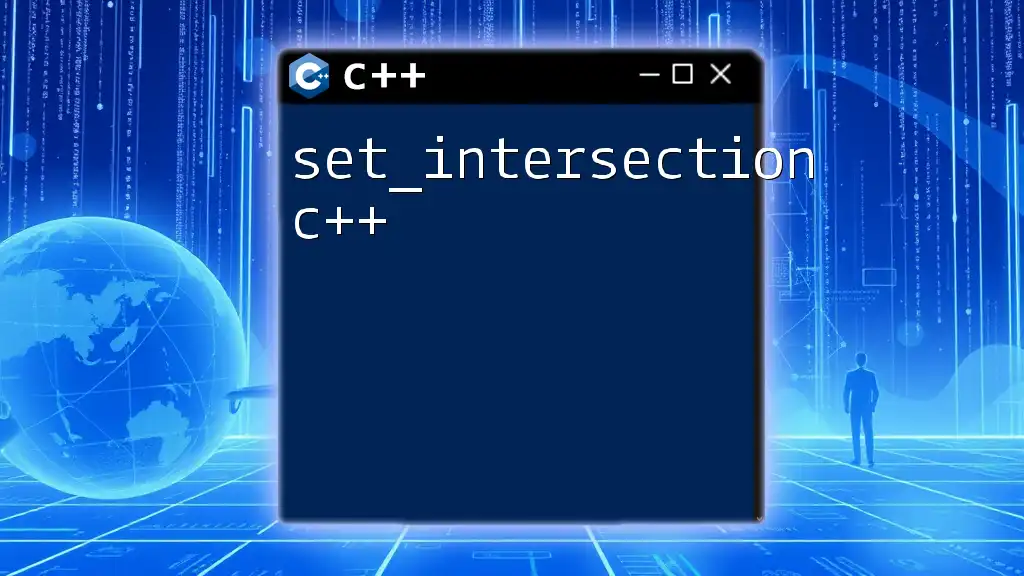
Further Reading and Resources
For those interested in expanding their knowledge, consider exploring additional resources on C++ exception handling, such as reputable books and online courses aimed at deepening your understanding of this critical aspect of programming.
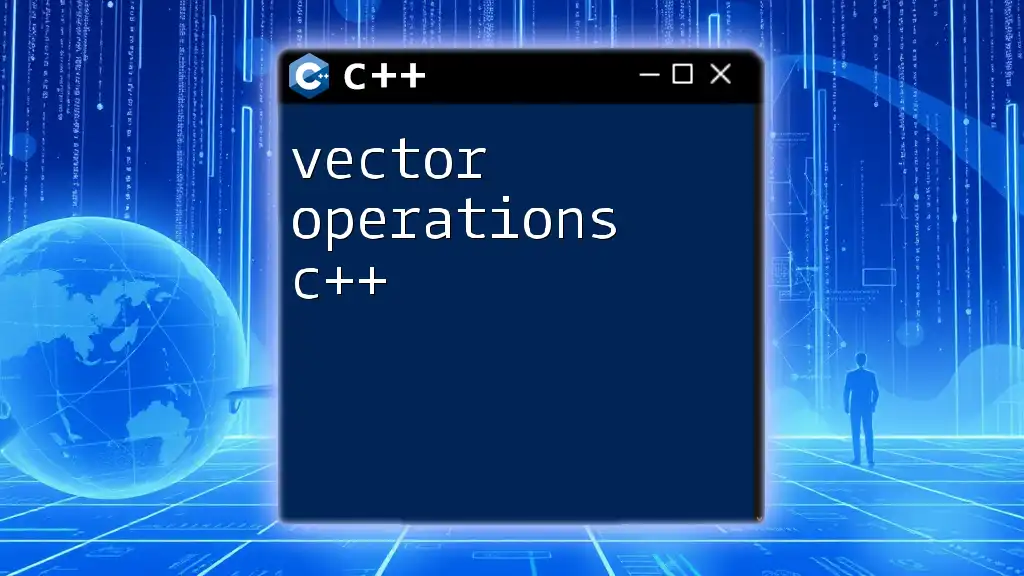
Call to Action
Join our community to stay updated for more quick and concise C++ tutorials! We value your feedback, so feel free to share your thoughts or experiences with exception handling in C++. Your insights are crucial for continuous improvement!