A conditional statement in C++ allows programs to execute specific blocks of code based on whether a given condition evaluates to true or false.
#include <iostream>
using namespace std;
int main() {
int number = 5;
if (number > 0) {
cout << "The number is positive." << endl;
} else {
cout << "The number is not positive." << endl;
}
return 0;
}
Understanding Conditional Statements
What Are Conditional Statements?
Conditional statements are a crucial part of programming logic, enabling a program to make decisions based on specific conditions or criteria. In C++, these statements control the flow of execution, allowing the program to perform different actions based on different inputs. This aspect of programming is vital for creating dynamic, responsive applications that can adapt to a variety of scenarios.
Key Types of Conditional Statements in C++
C++ provides several types of conditional statements that you can use to control your program's execution:
- `if` statement: Executes a block of code only if a specified condition is true.
- `if-else` statement: Offers an alternative block of code to execute if the condition in the `if` statement is false.
- `else-if ladder`: Enables multiple conditions to be checked in sequence.
- `switch` statement: A more efficient way to evaluate multiple potential values of a single variable.

The `if` Statement
Syntax of the `if` Statement
The `if` statement syntax is straightforward:
if (condition) {
// code block to execute if condition is true
}
Example of Basic `if` Statement
Consider the following code snippet:
int a = 10;
if (a > 5) {
std::cout << "A is greater than 5.";
}
In this example, the program checks if the variable `a` is greater than 5. If this condition evaluates to true, the statement inside the `if` block executes, printing "A is greater than 5." If the condition is false, nothing happens, and the program continues its execution.
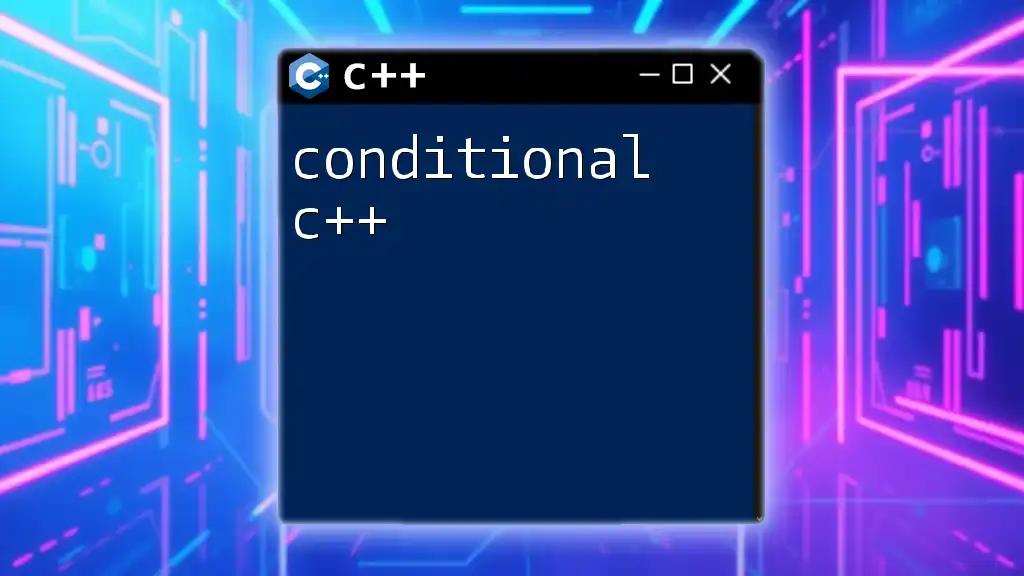
The `if-else` Statement
Syntax of the `if-else` Statement
The `if-else` statement expands upon the `if` statement by providing an alternative course of action:
if (condition) {
// code if true
} else {
// code if false
}
Example with `if-else`
Here's how it looks in a practical scenario:
int b = 5;
if (b < 10) {
std::cout << "B is less than 10.";
} else {
std::cout << "B is 10 or greater.";
}
In this example, the program checks if `b` is less than 10. If true, it prints "B is less than 10." Otherwise, it prints "B is 10 or greater." The `else` clause elegantly handles the opposite condition, ensuring that one of the two outcomes is always presented.
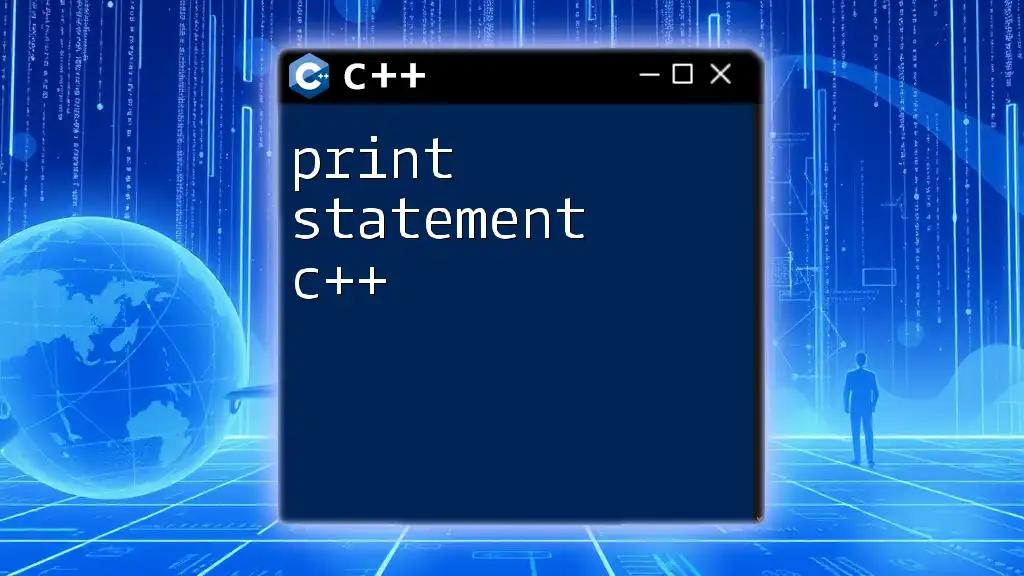
The `else if` Ladder
Syntax of the `else if` Ladder
When multiple conditions need to be evaluated, the `else-if` ladder comes into play:
if (condition1) {
// code if condition1 is true
} else if (condition2) {
// code if condition2 is true
} else {
// code if both conditions are false
}
Example of Using `else if`
Consider this example to illustrate the use of `else if`:
int score = 85;
if (score >= 90) {
std::cout << "Grade: A";
} else if (score >= 80) {
std::cout << "Grade: B";
} else {
std::cout << "Grade: C or lower";
}
In this case, the program evaluates the `score` variable and checks multiple conditions sequentially. If `score` is 90 or higher, it prints "Grade: A." If not, but it is 80 or higher, it prints "Grade: B." If neither condition is met, it defaults to printing "Grade: C or lower." This sequential checking allows for a clear evaluation of multiple potential scenarios.
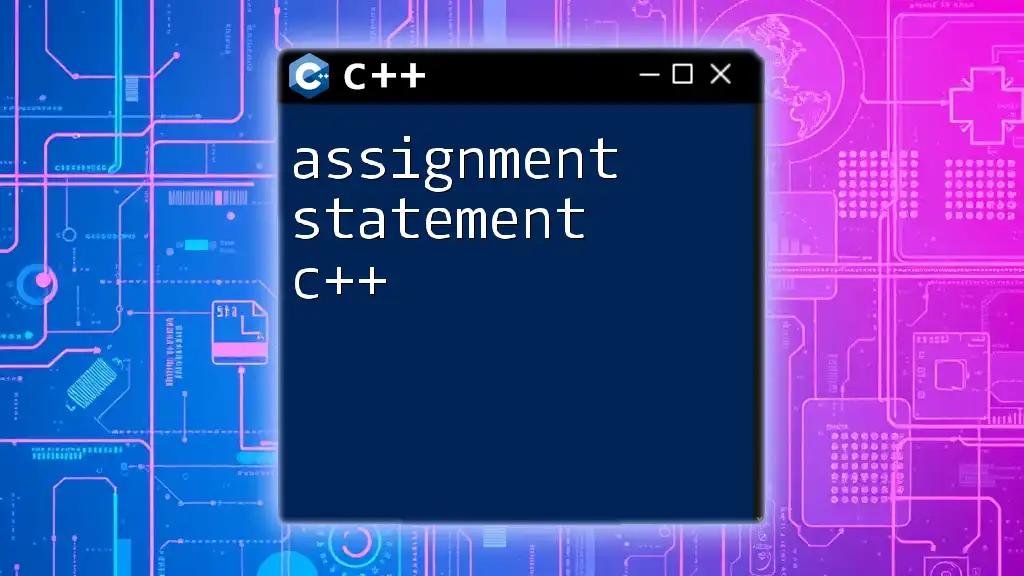
The `switch` Statement
Syntax of the `switch` Statement
The `switch` statement is a control statement that allows you to execute different blocks of code based on the value of a variable:
switch (expression) {
case value1:
// code block for value1
break;
case value2:
// code block for value2
break;
default:
// default code block
}
When to Use `switch` Statements
The `switch` statement is particularly useful when you have a single variable that can take on several values. It's more efficient than multiple `if-else` statements in such cases.
Example of `switch` Statement
Here’s a straightforward implementation of the `switch` statement:
char grade = 'B';
switch (grade) {
case 'A':
std::cout << "Excellent!";
break;
case 'B':
std::cout << "Well done.";
break;
case 'C':
std::cout << "Good job.";
break;
default:
std::cout << "Grade not recognized.";
}
In this example, the variable `grade` is evaluated. Depending on its value, a specific message is printed. The `break` statement is crucial as it prevents the execution from falling through to subsequent case blocks. The `default` case acts as a fallback, ensuring that even an unexpected value is gracefully handled.
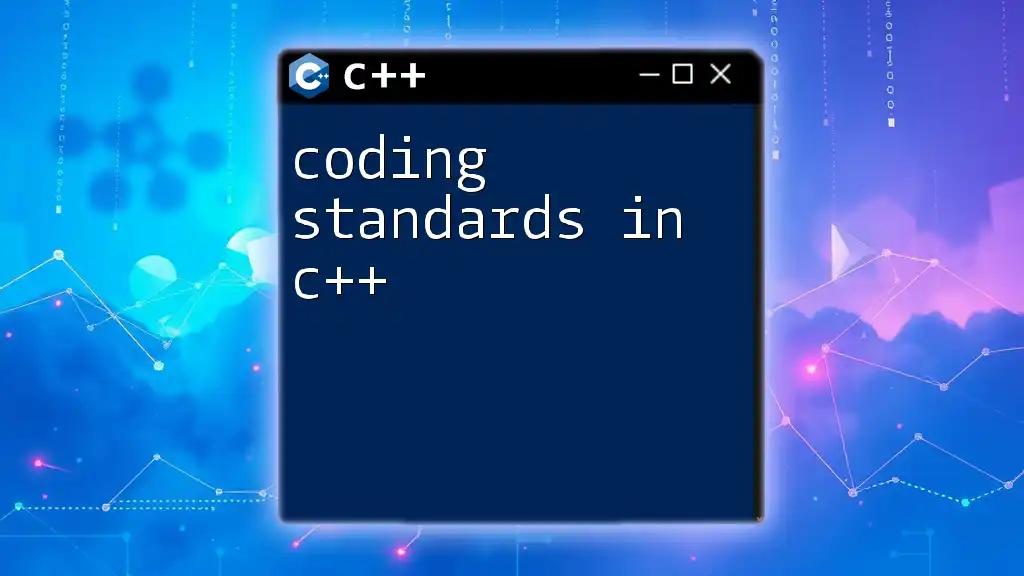
Nested Conditional Statements
What Are Nested Conditionals?
Nested conditionals occur when one conditional statement is placed inside another. This allows for complex decision-making processes.
Example of Nested Conditionals
Below is an example showing nested conditionals in action:
int age = 20;
if (age >= 18) {
std::cout << "Adult";
if (age >= 65) {
std::cout << " and senior citizen.";
}
} else {
std::cout << "Minor";
}
In this code snippet, the program first checks if `age` is 18 or older. If true, it prints "Adult." Then, it checks if the age is 65 or older, adding " and senior citizen" if applicable. If the age is less than 18, it prints "Minor." This structure allows for nuanced control over program flow based on multiple criteria.
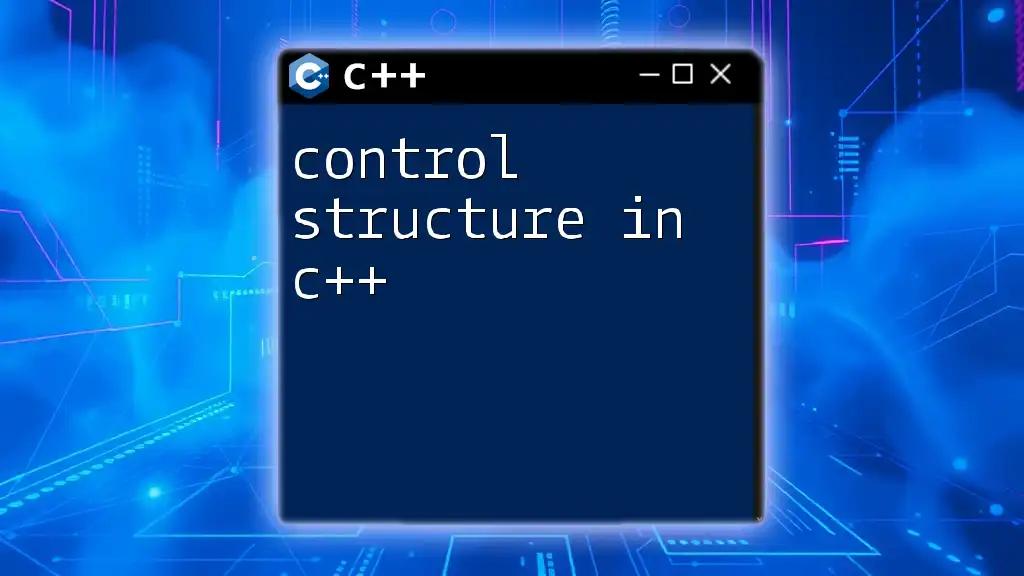
Common Mistakes and Best Practices
Pitfalls to Avoid
While writing conditional statements, it's essential to avoid common mistakes such as:
- Forgetting to include `break` in a `switch`: Without `break`, the program continues executing the subsequent case blocks, which can lead to unexpected behavior.
- Misplacing brackets in `if` statements: Properly using brackets ensures that the intended blocks of code are executed under the correct conditions.
Best Practices
To enhance clarity and maintainability in your code, consider these best practices:
- Keep conditions simple: Clarity in your conditions helps others (and future you) understand the program logic easily.
- Use meaningful variable names: Descriptive variable names reduce confusion and improve the readability of your conditions.
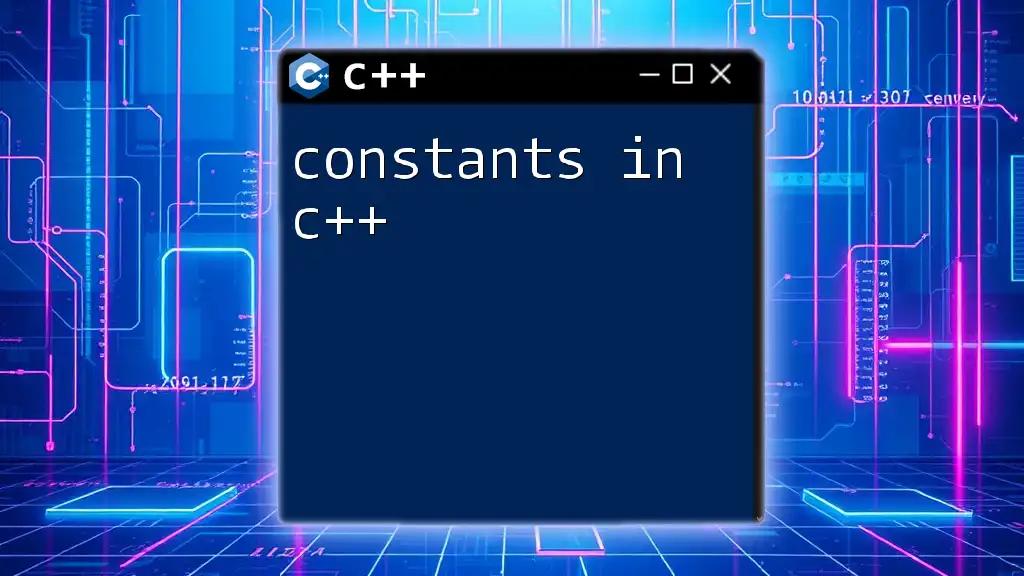
Conclusion
Conditional statements in C++ are fundamental for making decisions in programming. Their diverse forms allow developers to write flexible and powerful code that responds intelligently to user input and other variables. Mastering these statements is essential for anyone looking to grow their programming skills in C++ and enhance their ability to develop complex, responsive applications.
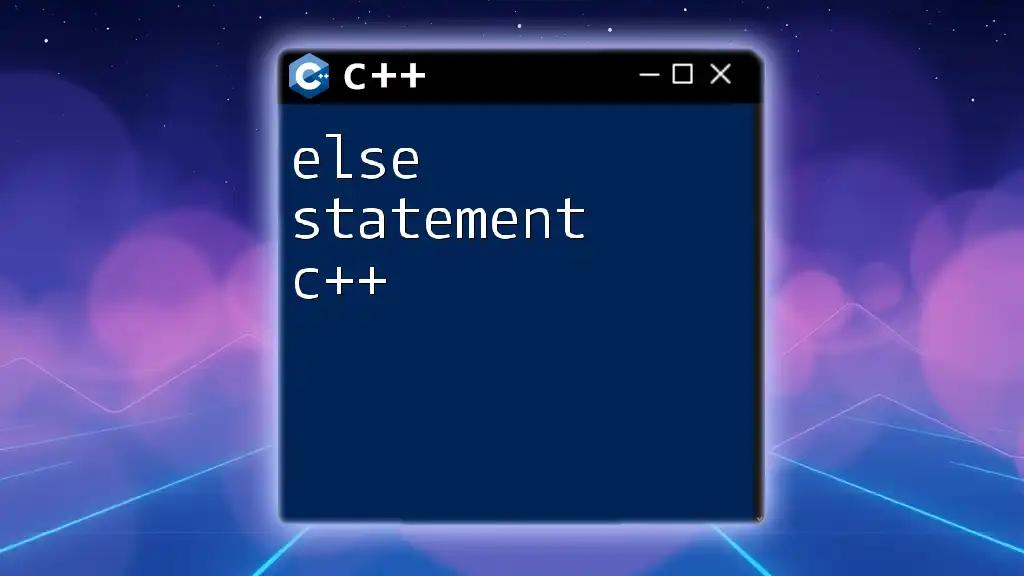
Additional Resources
For further exploration of conditional statements in C++, consider diving into official documentation, online coding tutorials, and reference materials available widely across the programming community.