An if statement in C++ allows you to execute a block of code conditionally, depending on whether a specified boolean expression evaluates to true.
#include <iostream>
int main() {
int number = 10;
if (number > 5) {
std::cout << "The number is greater than 5." << std::endl;
}
return 0;
}
Understanding If Syntax in C++
An if statement is a fundamental control structure in C++, allowing programmers to execute specific code based on whether a given condition evaluates to true or false.
Basic Structure of If Statement
The basic syntax of an if statement is straightforward:
if (condition) {
// code to execute if condition is true
}
In this structure:
- Condition: This is an expression that evaluates to true or false.
- Braces: The braces `{}` denote a block of code that will be executed if the condition is met.
Variations of If Statement
If-Else Statement
An if-else statement provides an alternative route of execution if the condition is false. The structure is as follows:
if (condition) {
// code for true condition
} else {
// code for false condition
}
Example: Suppose you want to check if a user is eligible for a child discount based on their age. Here’s how it can be implemented:
int age;
cout << "Enter your age: ";
cin >> age;
if (age < 18) {
cout << "You are eligible for a child discount.";
} else {
cout << "You are not eligible for a child discount.";
}
In this example, if the age is less than 18, the user is informed about the discount.
Else If Ladder
Sometimes, you might need to evaluate multiple conditions. This is where the else if ladder comes in:
if (condition1) {
// code for condition1 being true
} else if (condition2) {
// code for condition2 being true
} else {
// code if none of the conditions are true
}
Example: Consider a grading system where scores determine the grades:
int score;
cout << "Enter your score: ";
cin >> score;
if (score >= 90) {
cout << "Grade: A";
} else if (score >= 80) {
cout << "Grade: B";
} else if (score >= 70) {
cout << "Grade: C";
} else {
cout << "Grade: F";
}
Here, the program assigns a grade based on the score entered by the user.
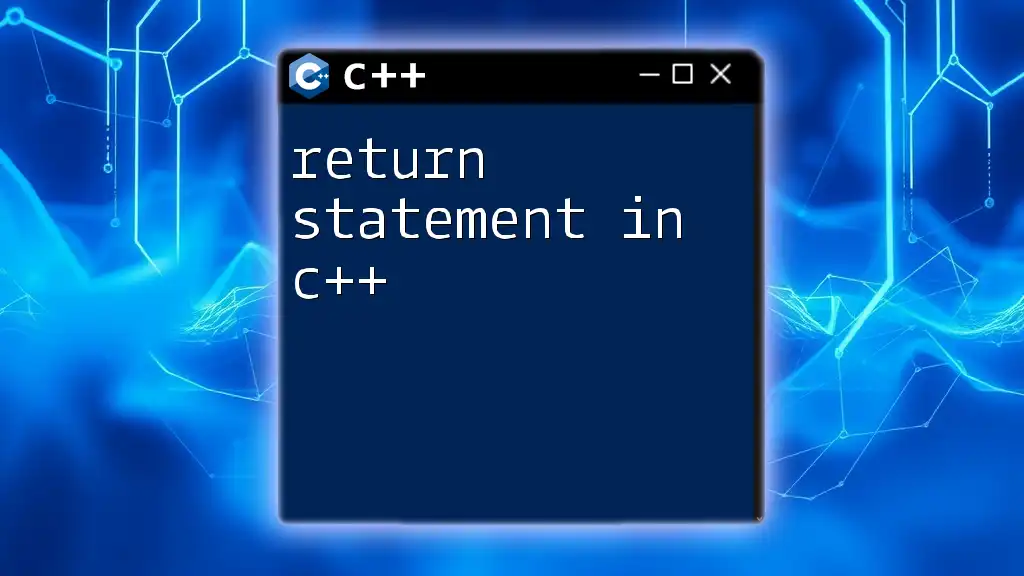
Real-World Examples of If Statements in C++
Example 1: Basic Temperature Check
Let’s put the conditional statement to practical use. Suppose you want to check whether a person should stay indoors or go outside based on temperature:
int temperature;
cout << "Enter the current temperature: ";
cin >> temperature;
if (temperature > 30) {
cout << "It's hot outside, better stay indoors!";
} else {
cout << "The weather is fine, feel free to go out!";
}
In this case, if the temperature exceeds 30 degrees, the suggestion is to stay indoors.
Example 2: Grading System
Also illustrated previously, grading based on score demonstrates effective use of the if statement in education applications. It checks the score ranges and assigns the corresponding grades.
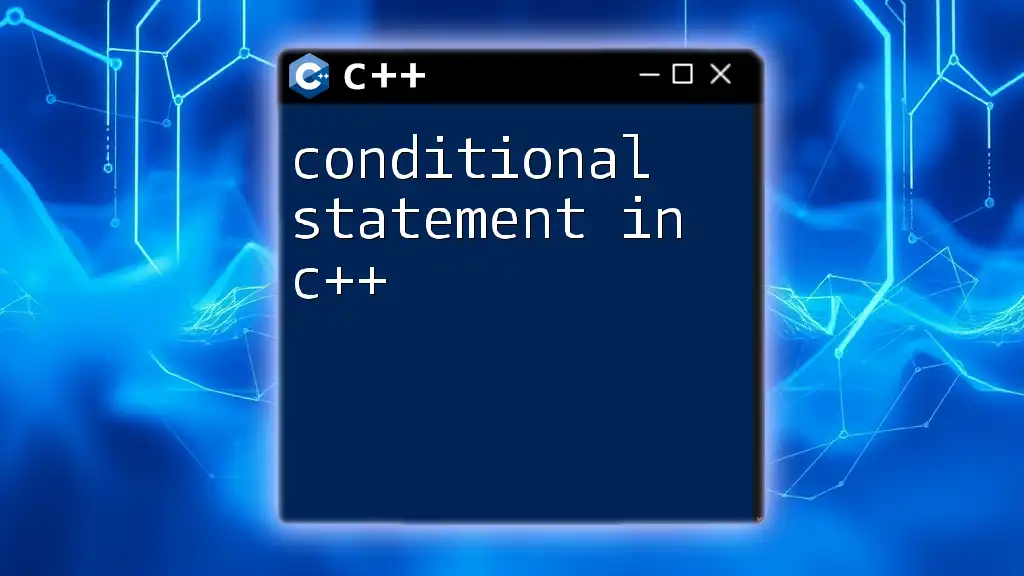
The Importance of Using If Statements
Utilizing if statements significantly enhances code readability and maintainability. By clearly defining conditions for various paths of code execution, programmers can make their intentions clear to anyone who reads the code later.
Additionally, they serve to reduce complexity. A well-structured series of if statements draws a clean, logical pathway through the program, making debugging and modification considerably easier.
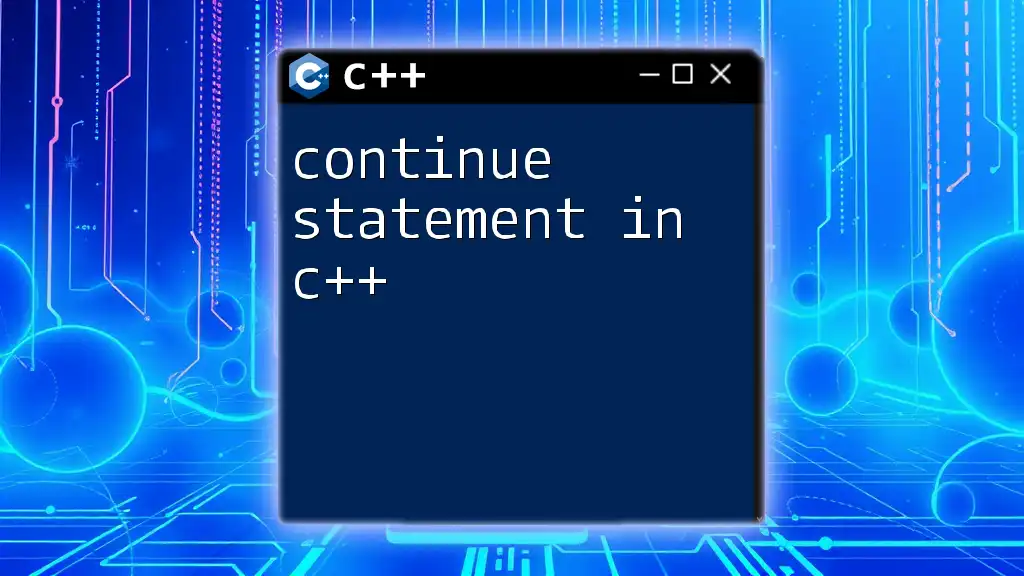
Best Practices for Using If Statements
Keeping Conditions Simple
When writing conditions, strive for clarity and simplicity. Complicated expressions can quickly become hard to understand, even for the original programmer. Simple conditions are more intuitive.
Avoiding Redundant Condition Checks
Optimizing your if statements can lead to better performance. If the same condition is checked multiple times, consider restructuring your code to avoid redundancy.
Utilizing Boolean Logic
Sometimes, you might need to check multiple conditions. Utilizing logical operators like AND (`&&`) and OR (`||`) allows you to combine conditions efficiently. Here’s an example:
int age;
bool hasLicense;
cout << "Enter your age: ";
cin >> age;
cout << "Do you have a license? (1 for Yes, 0 for No): ";
cin >> hasLicense;
if (age > 18 && hasLicense) {
cout << "You are allowed to drive.";
} else {
cout << "You cannot drive.";
}
In this case, both conditions must be true for the user to be allowed to drive.
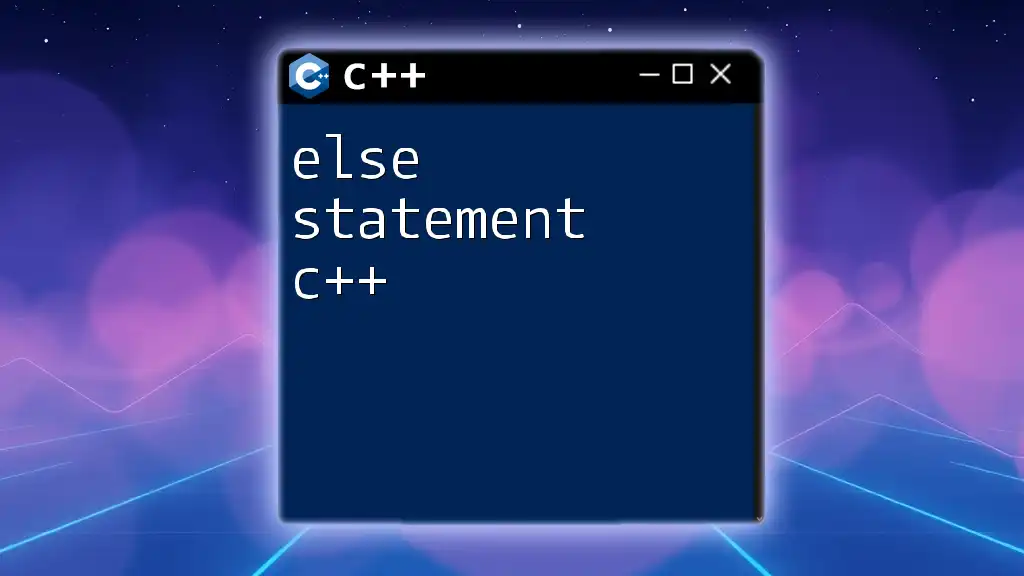
Conclusion
Understanding the example of an if statement in C++ is fundamental for any programmer aiming to grasp the logic of conditional execution. By applying this control structure, you can make decisions in your programs, enhancing their interactivity and functionality. Remember to practice with your own examples to cement your understanding.