Polymorphism in C++ allows functions or methods to use entities of different types at different times, enabling a single interface to represent different underlying forms (data types).
Here’s a simple example demonstrating polymorphism using base and derived classes:
#include <iostream>
using namespace std;
class Animal {
public:
virtual void sound() { cout << "Animal makes a sound" << endl; }
};
class Dog : public Animal {
public:
void sound() override { cout << "Dog barks" << endl; }
};
class Cat : public Animal {
public:
void sound() override { cout << "Cat meows" << endl; }
};
int main() {
Animal* myAnimal1 = new Dog();
Animal* myAnimal2 = new Cat();
myAnimal1->sound(); // Outputs "Dog barks"
myAnimal2->sound(); // Outputs "Cat meows"
delete myAnimal1;
delete myAnimal2;
return 0;
}
Understanding Polymorphism in C++
What is Polymorphism in C++?
Polymorphism is a core concept in object-oriented programming (OOP) that refers to the ability of different classes to be treated as instances of the same class through a common interface. In simpler terms, it allows methods to do different things based on the object it’s acting upon, even if they share the same name.
There are primarily two types of polymorphism:
- Compile-Time Polymorphism: Achieved through method overloading or operator overloading.
- Run-Time Polymorphism: Achieved through method overriding via virtual functions.
Understanding these two types is crucial for leveraging polymorphism effectively in C++ programming.
Importance of Polymorphism in C++
The importance of polymorphism in C++ cannot be overstated. It brings a slew of benefits:
-
Flexibility and Extensibility: Polymorphism allows for scalable code that can adapt to new requirements without altering existing structures. You can easily add new classes adhering to a common interface without modifying the core logic.
-
Code Reusability: By defining methods and variables at a common level, polymorphism enables developers to write reusable code segments that can work with different data types. This cuts down on redundancy and makes for cleaner code.
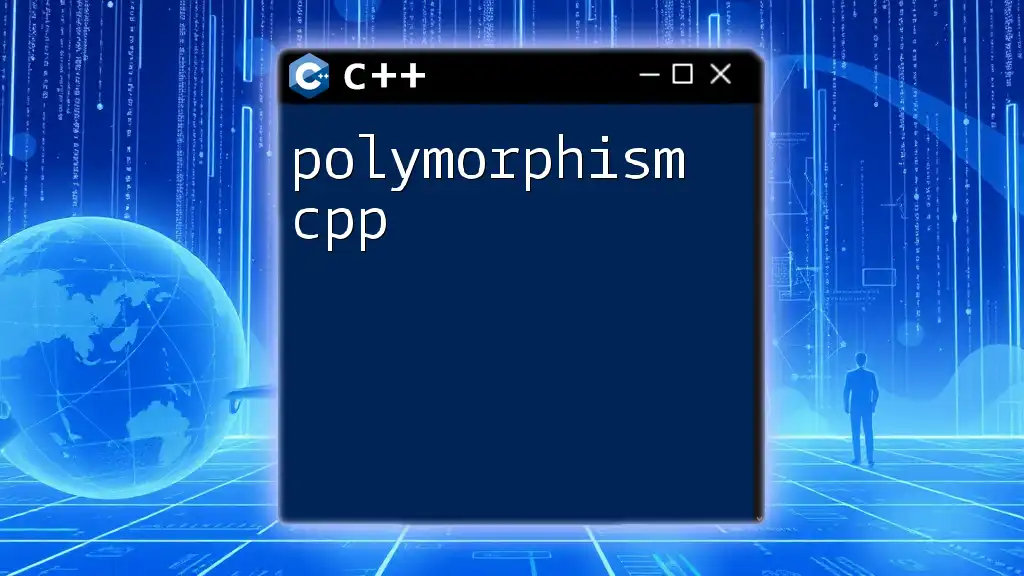
Types of Polymorphism in C++
Compile-Time Polymorphism
Function Overloading
Function overloading allows multiple functions to have the same name but different parameters. This is resolved at compile time, hence the name. For example, you might define a function to compute the area of different shapes:
void area(int radius) {
cout << "Area of Circle: " << 3.14 * radius * radius << endl;
}
void area(int length, int breadth) {
cout << "Area of Rectangle: " << length * breadth << endl;
}
In the code above, the `area` function behaves differently based on whether it’s called with one or two integer parameters.
Operator Overloading
Operator overloading allows developers to define custom behaviors for operators. Here's an example showing how to overload the '+' operator for a `Complex` class:
class Complex {
public:
float real;
float imag;
Complex operator + (Complex const &obj) {
Complex res;
res.real = real + obj.real;
res.imag = imag + obj.imag;
return res;
}
};
In this example, two `Complex` objects can be added together using the `+` operator, enhancing readability and usability.
Run-Time Polymorphism
Overview of Run-Time Polymorphism
Run-time polymorphism allows method calls to be resolved during program execution, making it more dynamic. This is primarily achieved through the use of virtual functions.
Virtual Functions
Virtual functions enable derived classes to override base class methods. When you call a virtual function via a base class pointer/reference that points to a derived class object, the derived class version of the method is invoked, illustrating run-time polymorphism.
Here's an example:
class Base {
public:
virtual void show() {
cout << "Base show" << endl;
}
};
class Derived : public Base {
public:
void show() override {
cout << "Derived show" << endl;
}
};
In this case, if you create an instance of `Derived` but refer to it through a pointer of type `Base`, calling the `show` method will trigger the `Derived` implementation.
Pure Virtual Functions and Abstract Classes
Sometimes, you may want to create an interface without providing any implementation — this is where pure virtual functions come in. They define a contract for derived classes.
class Abstract {
public:
virtual void show() = 0; // Pure virtual function, making it an abstract class
};
In this scenario, any class that inherits from `Abstract` must implement the `show` method or also be declared abstract.
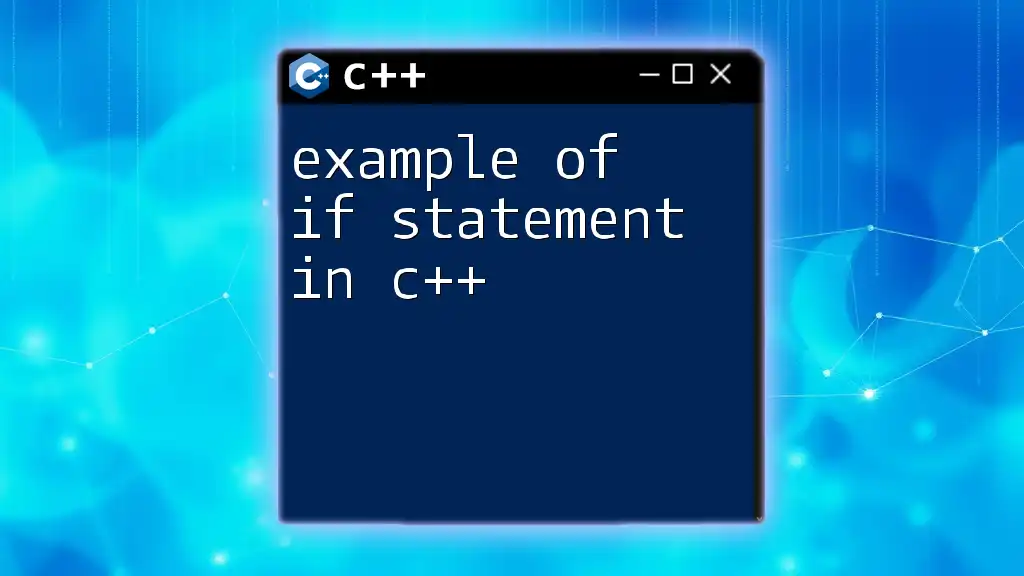
Polymorphism Example in C++
Building a Simple Polymorphic Application
Designing a Base Class and Derived Classes
Let’s create a simple hierarchy of classes that illustrate the concept of polymorphism. We can design a base class `Animal` which has a pure virtual function `sound()` and then derive `Dog` and `Cat` classes from it:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() {
cout << "Woof!" << endl;
}
};
class Cat : public Animal {
public:
void sound() {
cout << "Meow!" << endl;
}
};
Here, `Dog` and `Cat` both implement the `sound` method, providing specific behavior.
Demonstrating Polymorphism in Action
Now we can create a function which takes a pointer of type `Animal` and calls its `sound` method. This is where the magic of polymorphism takes place.
void makeSound(Animal* animal) {
animal->sound();
}
int main() {
Dog dog;
Cat cat;
makeSound(&dog); // Outputs: Woof!
makeSound(&cat); // Outputs: Meow!
}
In the `main` function, when we call `makeSound` with `Dog` and `Cat` objects, the appropriated `sound` method is invoked based on the actual object being referred to, not the type of the pointer.
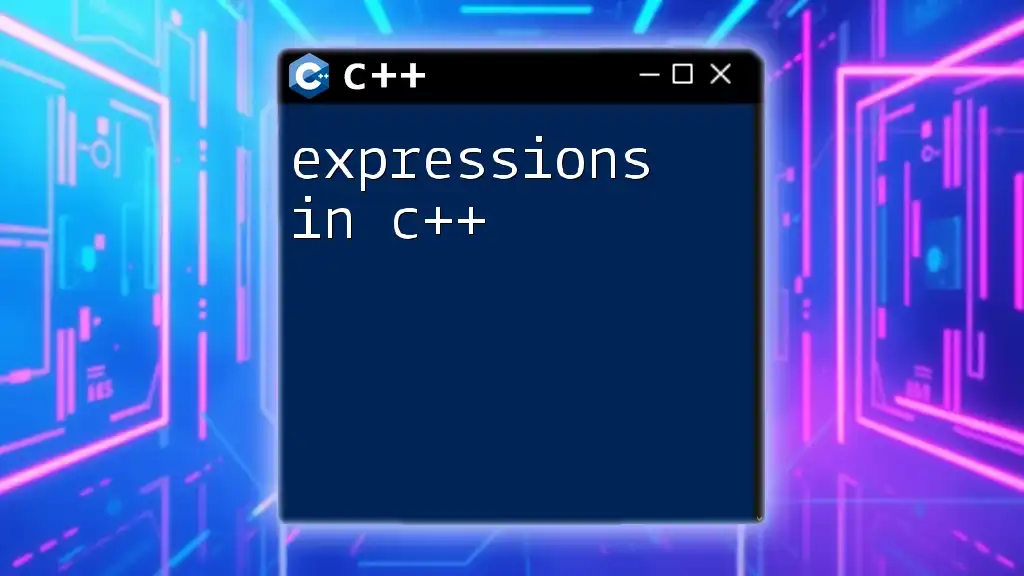
Practical Applications of Polymorphism in OOP C++
Real-World Examples
Polymorphism is highly applicable in various domains:
-
User Interface Elements: In GUI frameworks, user interface components like buttons and text boxes often share a common base class, allowing for generic handling of all components in the same manner.
-
Game Development: In games, entities like characters, enemies, and items can utilize polymorphism for managing different behaviors such as collision detection or actions like jumping and running.
Benefits for Developers
Polymorphism streamlines code management and fosters better collaboration among developers. Here’s how:
-
Streamlined Code Management: As classes evolve or new functionalities are introduced, polymorphism allows the codebase to handle changes with minimal disruption.
-
Enhanced Collaboration: By adhering to interfaces and shared contracts, multiple developers can work on different components of the same project without stepping on each other’s toes.
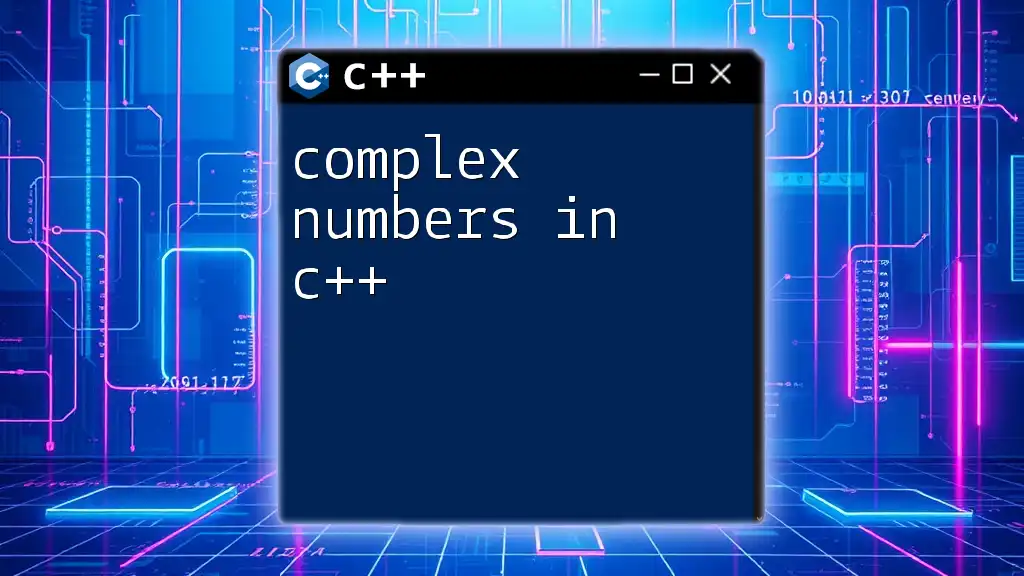
Conclusion
Summary of Key Points
Polymorphism enriches C++ programming by promoting flexibility, reusability, and easier maintenance of code. Understanding its mechanisms—both compile-time and run-time—is essential for any aspiring developer.
Next Steps for Learners
To deepen your understanding, explore further applications of polymorphism in real-world projects. Engage with open-source projects that leverage these concepts and experiment by building your own applications.
Call to Action
Feel free to share your experiences or questions about implementing polymorphism in your projects. Engaging in discussions will enhance your learning and help others in the community as well!