A valid C++ array definition specifies a data type followed by an identifier and an optional size, like this example:
int numbers[5]; // defines an array of 5 integers
Understanding C++ Array Syntax
Basic Definition of a C++ Array
A C++ array is a collection of elements of the same type, stored in contiguous memory locations. The basic syntax to define an array in C++ is:
type arrayName[arraySize];
Here, `type` represents the data type of the array, `arrayName` is the identifier we use to refer to the array, and `arraySize` specifies how many elements the array can hold. For instance:
int numbers[5]; // This defines an array of 5 integers.
This definition allocates memory for five integers in consecutive locations.
Types of Arrays
One-Dimensional Arrays
One-dimensional arrays are the most basic type of arrays where elements are accessed using a single index. The syntax for defining a one-dimensional array is straightforward:
dataType arrayName[size];
An example of a one-dimensional array could be:
double scores[10]; // This creates an array capable of holding 10 double values.
Multi-Dimensional Arrays
Multi-dimensional arrays, such as two-dimensional arrays (think of them as matrices), allow you to define arrays where elements are accessed using multiple indices. The syntax for a two-dimensional array is:
dataType arrayName[rowSize][columnSize];
For example:
int matrix[3][4]; // This creates a 3x4 matrix of integers.
To access an element in a two-dimensional array, you would use:
matrix[row][column];

Identifying Valid Array Definitions
Key Components of Array Declaration
When defining an array, three critical components must be correctly included:
-
Data Type: Each array must specify a valid data type such as `int`, `float`, or `char`.
-
Array Name: The name must adhere to C++ naming conventions. It should start with a letter or underscore and can contain letters, numbers, or underscores.
-
Size Specifier: This indicates how many elements the array is designed to hold. It's crucial to provide a non-negative integer for the array size.
Common Mistakes in Array Definitions
Several common mistakes can lead to invalid array definitions. For example:
-
Missing Size: Declaring an array without specifying its size is invalid.
int arr[]; // Invalid
-
Negative Size: Specifying negative sizes in array definitions is also invalid.
int arr[-5]; // Invalid
-
Non-Type Defined: Defining an array without specifying the data type will lead to a compilation error.
array; // Invalid
Understanding these mistakes is essential, as they can lead to runtime errors and complications in code execution.

Practical Examples of Valid Array Definitions
Valid One-Dimensional Arrays
Here are several examples of valid one-dimensional array definitions:
int arr[3] = {1, 2, 3}; // Initializes an array of integers with three elements.
float prices[5] = {10.99, 5.49, 2.25, 12.50, 3.00}; // Initializes an array of floating-point numbers.
Valid Multi-Dimensional Arrays
A multi-dimensional array can be defined as follows:
char grid[2][3] = {{'a', 'b', 'c'}, {'d', 'e', 'f'}}; // A 2D array of characters.
To access an element from this array, you would write:
char letter = grid[1][2]; // This will access 'f', which is located in the second row and third column.

Examples and Error Analysis
Compare Valid and Invalid Definitions
Let’s consider a few examples to determine which of the following is a valid C++ array definition.
- Example A:
int validArray[10]; // Valid
- Example B:
float invalidArray[5.5]; // Invalid, size must be an integer.
- Example C:
double anotherValid[10] = {1.0, 2.0}; // Valid, but extra indices will be initialized to zero.
Discussion on Errors
Elaborating on the errors, it’s essential to recognize why some definitions are invalid. For instance, in Example B, a non-integer type for the array size leads to a compilation error. These issues can significantly affect how programs execute and result in hard-to-track bugs.
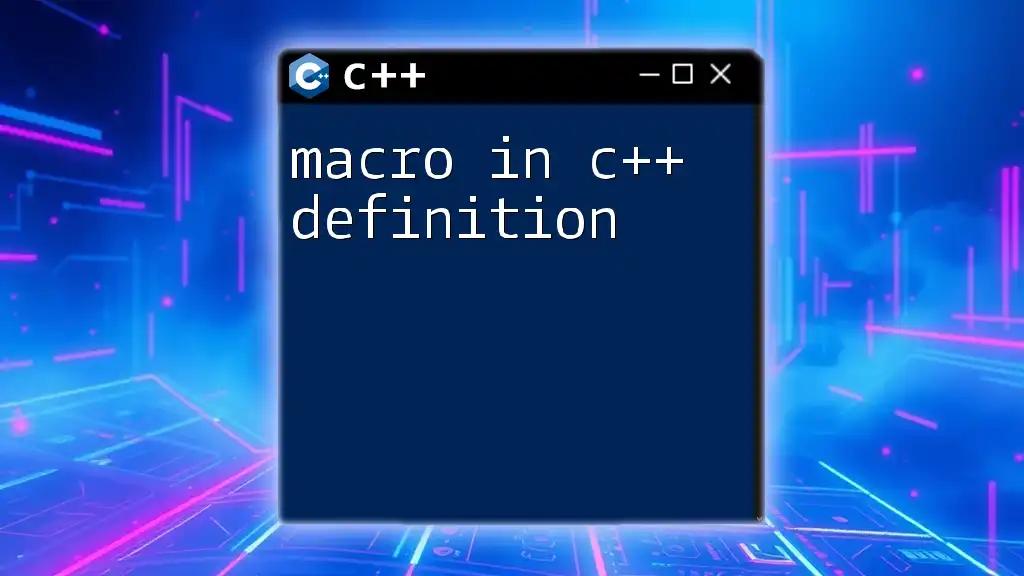
Best Practices for Defining Arrays
Array Initialization Techniques
When defining arrays, it's good practice to initialize them properly. For example, initializing a string array can be done as follows:
std::string names[3] = {"Alice", "Bob", "Charlie"}; // Valid initialization of a string array.
Another best practice is to initialize arrays with default values. For instance:
int sequence[10] = {}; // Initializes all elements to zero.
Dynamic Arrays with std::vector
In modern C++ development, using `std::vector` is often preferred over traditional arrays as it provides more flexibility. `std::vector` allows dynamic memory allocation and can resize during runtime.
Here’s an example of how to define a dynamic array:
#include <vector>
std::vector<int> dynamicArray = {1, 2, 3, 4, 5}; // Creates a dynamic array of integers.
Using `std::vector` not only simplifies memory management but also avoids several pitfalls associated with raw arrays, such as memory overflow and underflow.
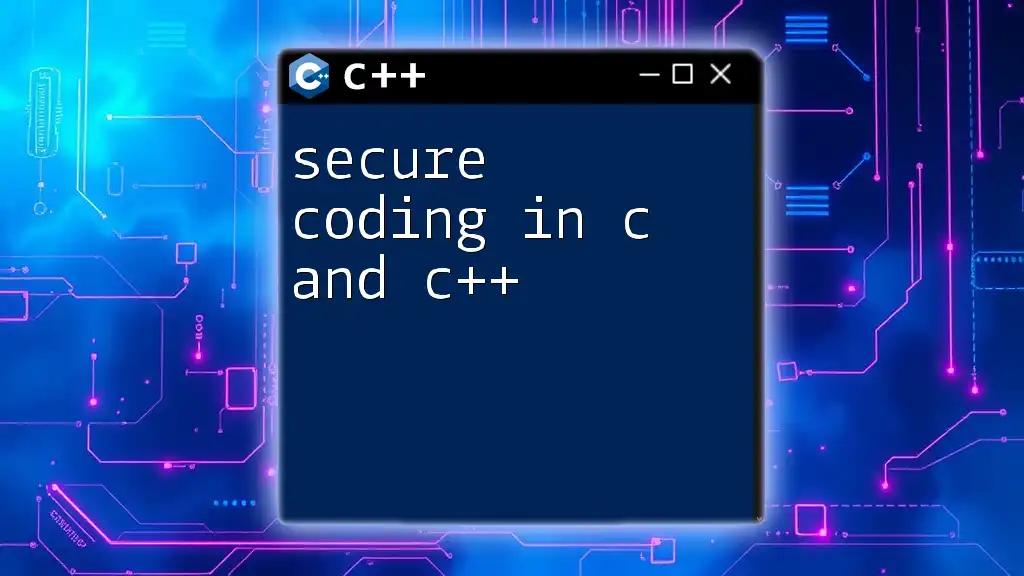
Conclusion
In summary, understanding which of the following is a valid C++ array definition is crucial for effective programming in C++. Making sure your array definitions are accurate will save you time and headaches in the long run. Engage in practice and explore different array definitions to solidify your understanding.

Additional Resources
For further reading, please explore the official C++ documentation on arrays and various online platforms that provide coding tutorials. Engaging with quizzes or exercises designed to reinforce your learning can also be immensely beneficial.
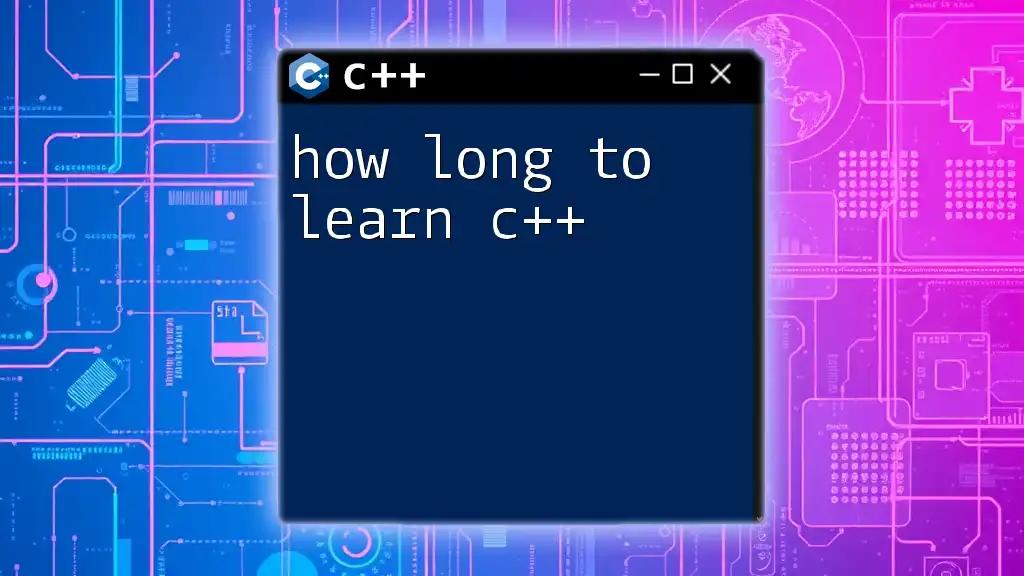
Call to Action
We encourage you to share your own experiences or challenges with defining arrays in C++ in the comments below! Additionally, don’t hesitate to sign up for our upcoming classes or workshops to dive deeper into the world of C++ programming.