The time it takes to learn C++ can vary widely depending on prior programming experience and the depth of knowledge desired, but with focused study, one can grasp the basics in about 1 to 3 months.
Here's a simple example of a C++ program that prints "Hello, World!" to illustrate syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Fundamentals
What is C++?
C++ is a general-purpose programming language that has influenced numerous languages and remains one of the most widely used languages today. Initially developed by Bjarne Stroustrup in the early 1980s, C++ introduced object-oriented programming features, which allow developers to create modular and reusable code.
Key Features of C++
- Object-Oriented Programming (OOP): C++ supports OOP principles, including encapsulation, inheritance, and polymorphism. This allows for creating complex software solutions more easily.
- Performance: C++ is a compiled language, which makes it performant and suitable for resource-intensive applications.
- Rich Standard Library: The Standard Template Library (STL) provides a wealth of data structures and algorithms, saving developers significant coding time.
The Learning Curve of C++
The learning curve for C++ can be steep, especially for beginners. Several factors impact how long it may take to learn this language:
- Prior Programming Experience: If you already have a grasp of programming concepts from languages like Python or Java, you might find it easier to pick up C++.
- Complexity of Concepts: C++ introduces complex topics such as pointers, memory management, and object-oriented principles, making the learning process challenging.
- Consistent Practice: The more you practice coding and solving problems, the shorter the learning period will be.
Common challenges faced by learners include understanding basic syntax, mastering pointers and memory allocation, and utilizing the STL effectively. These can create roadblocks, but with determination and patience, you can overcome them.
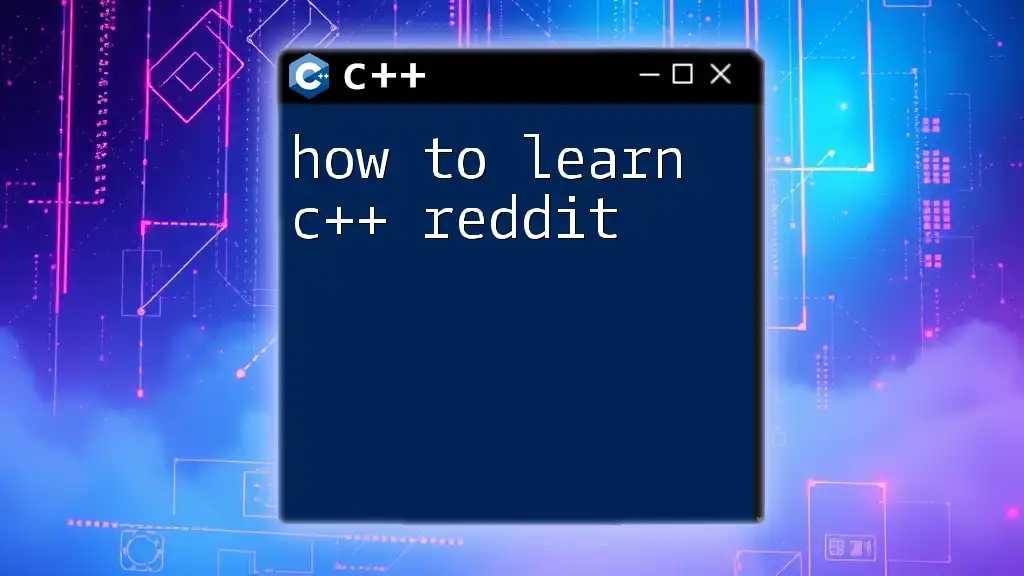
How Long Does It Take to Learn C++?
General Time Estimates
The time it takes to learn C++ varies based on commitment, prior knowledge, and learning methods. Here's a general breakdown:
- Beginner Level: If you dedicate a few hours daily over a few weeks, you can grasp basic syntax and write simple programs. Expect to learn concepts like variables, control structures, and basic I/O operations.
Example of a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Outputs a greeting
return 0; // Indicates that the program ended successfully
}
-
Intermediate Level: Devoting several months between 3 to 6 hours per week can lead you to an intermediate understanding of C++. At this level, you should be familiar with OOP principles, exception handling, and standard libraries.
-
Advanced Level: Achieving advanced proficiency in C++ could take a year or more, depending on the complexity of topics you cover, such as multi-threading, template programming, and memory management.
Learning Styles and Their Impact on Time
Your individual learning style can significantly influence how long it takes to learn C++.
-
Self-paced Learning: If you opt for self-study through tutorials and practice, you can move at your own pace. However, this requires self-discipline and motivation.
-
Structured Learning: Taking formal classes or enrolling in online courses can provide a clearer learning path, potentially accelerating your understanding of C++. A structured program often includes hands-on exercises that reinforce learning.
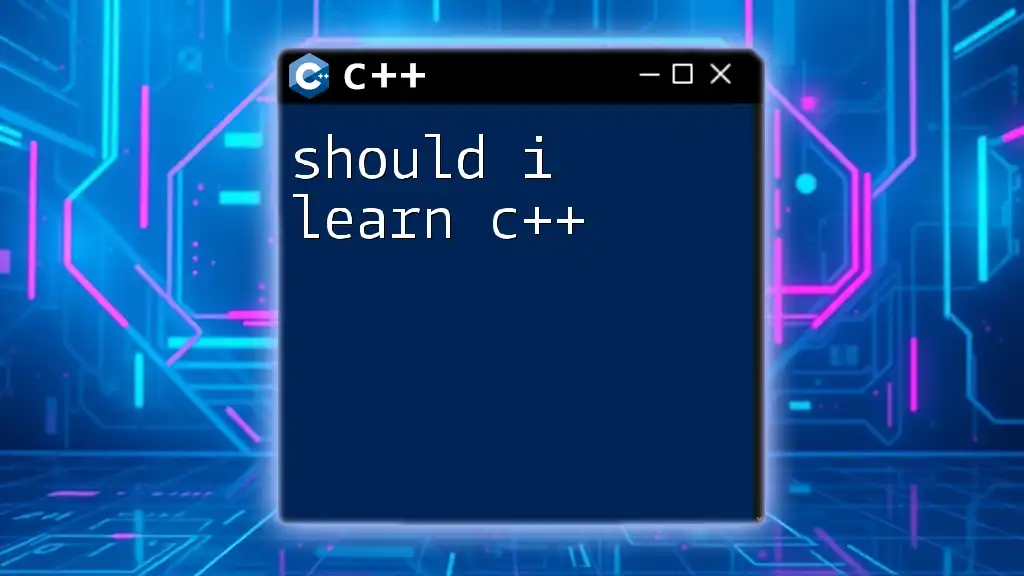
Effective Learning Strategies
Recommended Resources
To successfully navigate the learning landscape of C++, it's crucial to rely on quality resources:
-
Books: Several outstanding books can guide you through C++:
- "C++ Primer" by Stanley B. Lippman: A comprehensive introduction to C++ for beginners and intermediates.
- "Effective C++" by Scott Meyers: Offers valuable insights into writing more efficient C++ code.
-
Online Courses: Enrolling in reputable online platforms like Coursera, Udacity, or Codecademy can also help you grasp the nuances of C++ effectively, providing structured content and exercises.
Practical Exercises
To solidify your learning, engage in practical exercises that challenge your understanding:
-
Coding Challenges: Utilize platforms like LeetCode, Codecademy, or HackerRank to practice solving problems in C++. These exercises are designed to push your understanding and improve your coding skills.
-
Projects: Working on real-world projects can help you apply your skills. Begin with simpler projects like a:
- Basic Calculator: Implementing mathematical operations and user input handling.
- To-Do List Application: A console-based app that allows users to add, delete, and manage tasks.
Utilizing Code Snippets
Learning C++ can be significantly enhanced by examining and understanding various code snippets. A snippet library will aid in quick learning, showcasing practical applications of C++ concepts.
Example Snippet: Here's a brief example demonstrating the concept of classes and objects:
#include <iostream>
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Calls the bark method
return 0;
}
In this snippet, we create a class `Dog` with a method `bark()`. The `main` function creates an object of the `Dog` class and calls the method, showcasing how OOP works in C++.
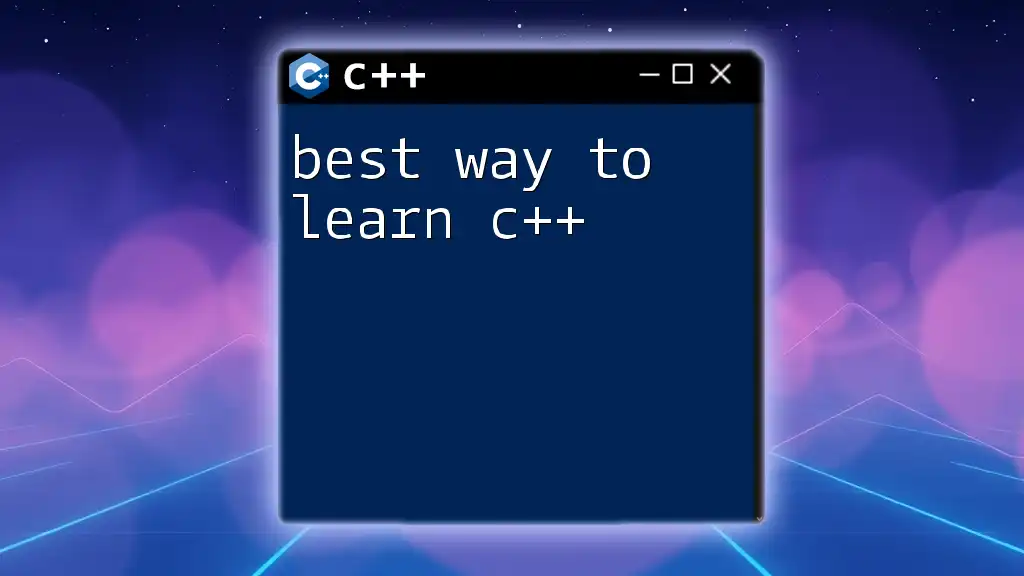
Tips for Accelerating Your Learning Journey
Consistent Practice
To master C++, consistent practice is key. Aim to code daily, experimenting with different concepts and refining your skills. Short, focused practice sessions can be more beneficial than infrequent, longer study periods.
Join a Community
Becoming part of a programming community can profoundly impact your learning journey. Engage with other learners and experts on forums like Stack Overflow or Reddit. These communities can provide invaluable insights, answers to queries, and support that enhances your learning experience.
Seek Feedback
Feedback is an essential part of learning. Consider finding a mentor or joining a study group where you can discuss problems and solutions. Constructive criticism can help you identify areas for improvement and accelerate your learning process.
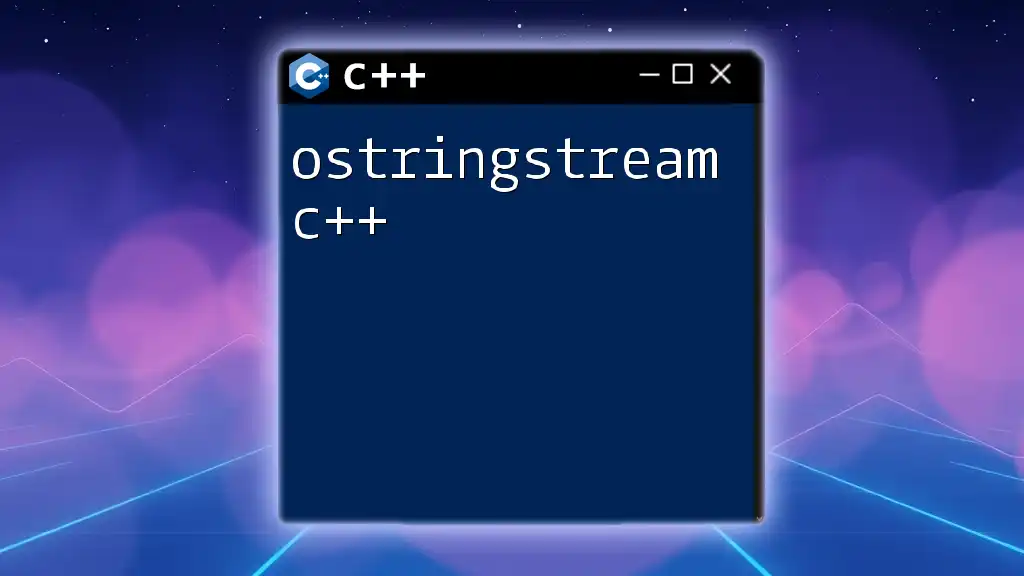
Conclusion
In summary, how long to learn C++ depends on various factors, including individual dedication, learning styles, and prior programming experience. While beginners may grasp the basics in a few weeks, achieving mastery in C++ requires consistent practice and engagement with more advanced concepts over months or even years. The journey may seem daunting, but with the right resources and a commitment to learning, anyone can become proficient in C++.
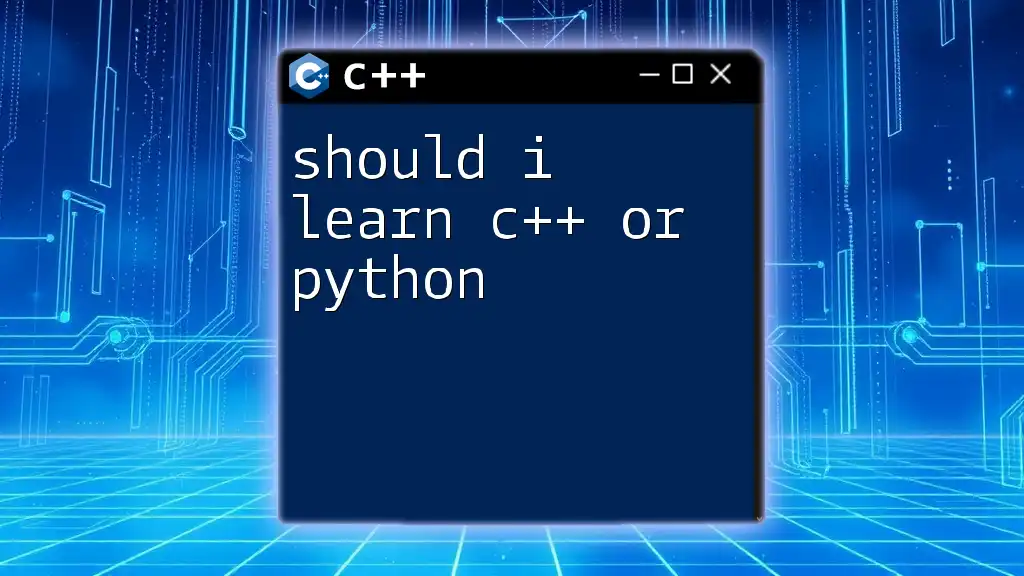
Call to Action
Join our C++ workshop to learn concise and effective command usage, enhancing your programming capabilities in a supportive environment. Share your learning story and experiences with us in the comments, and let’s grow together in this exciting programming journey!