The best way to learn C++ is by practicing basic syntax through hands-on coding, allowing you to grasp concepts effectively and develop problem-solving skills.
Here's a simple code snippet to illustrate a basic C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Fundamentals
What is C++?
C++ is a general-purpose programming language that has stood the test of time due to its versatility and performance. Developed by Bjarne Stroustrup at Bell Labs in the early 1980s, C++ expands upon the features of C while introducing object-oriented programming (OOP) concepts. As a language that supports multiple programming paradigms—including procedural, object-oriented, and generic programming—C++ has found its niche in various fields such as game development, high-performance computing, and systems programming.
Core Concepts of C++
To grasp the best way to learn C++, it's essential to build a solid foundation in the language's core concepts.
Key Terminology
Before diving into coding, familiarize yourself with key C++ terminology:
- Variables: Containers for storing data values.
- Data Types: Define the type of data a variable can hold (e.g., `int`, `float`, `char`).
- Operators: Symbols that perform operations on variables and values (e.g., `+`, `-`, `*`, `/`).
- Expressions: Combinations of variables, constants, and operators that evaluate to a value.
Basic Syntax
C++ syntax is straightforward but requires attention to detail. Understanding the basic structure of a C++ program is crucial for beginners. Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code snippet illustrates the basic structure of a C++ program: it includes a header file, defines the `main` function, and outputs a message to the console.
Object-Oriented Programming in C++
C++ is synonymous with object-oriented programming (OOP), which is a methodology based on objects containing both data and methods.
Characteristics of OOP
The key characteristics of OOP include:
- Encapsulation: Bundling data and methods into a single unit, the object.
- Inheritance: Acquiring properties and behaviors from other classes.
- Polymorphism: Utilizing derived classes to redefine methods in a base class.
Example of a Simple Class
Here's how you can define a class in C++:
class Animal {
public:
void sound() {
std::cout << "Animal sound!" << std::endl;
}
};
This `Animal` class has a single method, `sound()`, showcasing how objects can contain functions and use them.
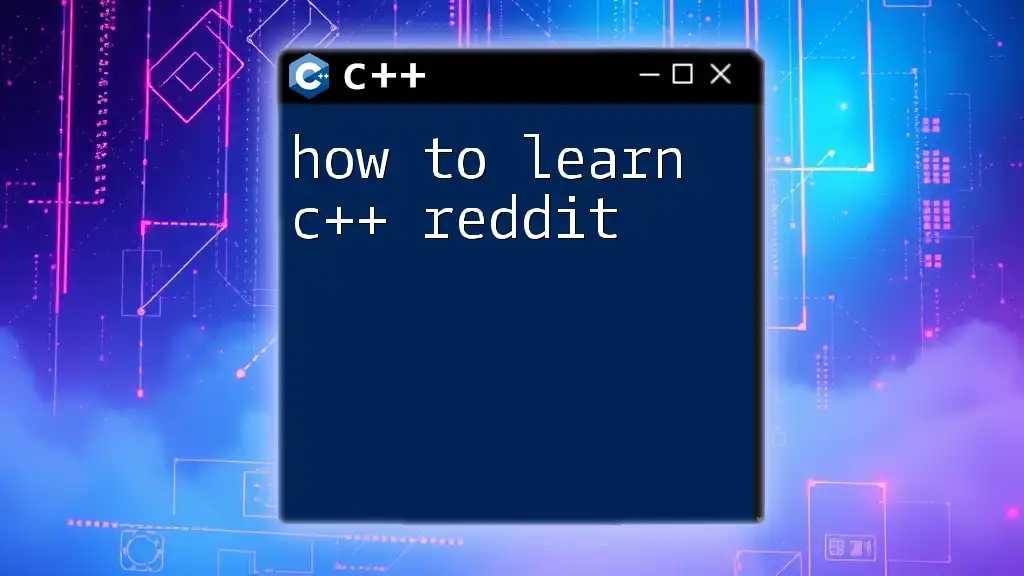
Resources for Learning C++
Books
Books are a foundational resource for learning C++. Here are a couple of recommended titles that provide in-depth knowledge:
- "Effective C++" by Scott Meyers: Offers essential best practices and guidelines for writing robust C++ code.
- "The C++ Programming Language" by Bjarne Stroustrup: Written by the creator of C++, this comprehensive guide covers most aspects of the language.
Online Courses
Online learning platforms provide structured learning paths. Consider exploring:
- Coursera: Offers university-level courses often guided by expert instructors.
- Udemy: Features a variety of courses that allow you to learn at your own pace.
- edX: Provides high-quality classes from institutions like MIT and Harvard.
Interactive Coding Platforms
Hands-on practice is paramount in mastering C++. Websites such as:
- LeetCode: A great place to solve coding problems and improve problem-solving skills.
- HackerRank: Allows you to practice real coding challenges and compete with peers.
- Codecademy: Offers interactive exercises that put theory into practice.
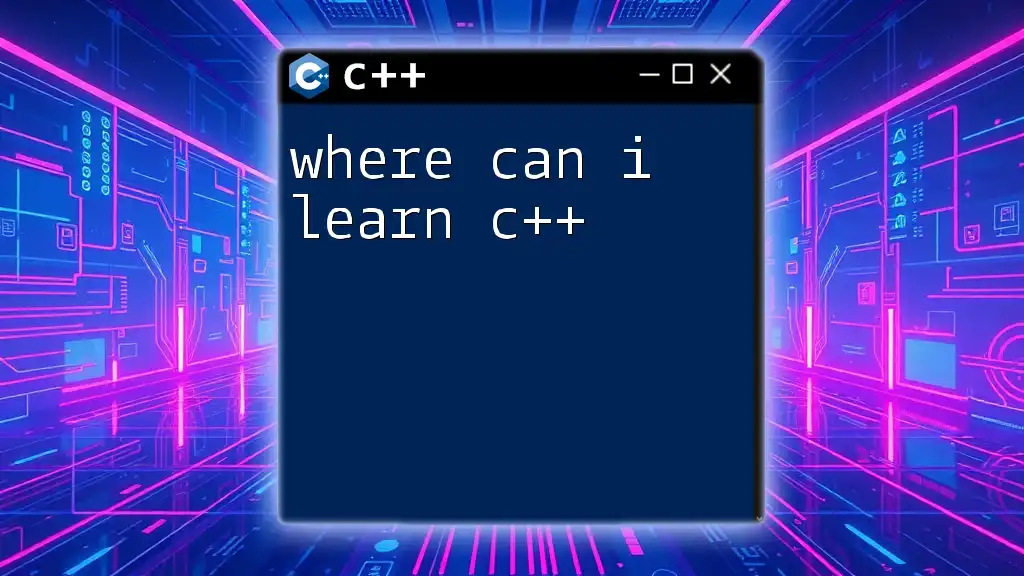
Practical Learning Strategies
Setting Learning Goals
Establishing clear learning objectives can significantly improve your progress. Utilize the SMART criteria—Specific, Measurable, Achievable, Relevant, and Time-bound—to set effective goals. For example, instead of saying "I want to learn C++," aim for "I will complete an online C++ course in four weeks."
Daily Coding Challenges
Consistency is key in programming. Engage in daily coding challenges to solidify your skills. A simple challenge could be:
- Challenge Idea: Write a program that calculates the factorial of a number using recursion.
Here’s a basic implementation:
#include <iostream>
int factorial(int n) {
return (n == 1 || n == 0) ? 1 : factorial(n - 1) * n;
}
int main() {
int number = 5;
std::cout << "Factorial of " << number << " is " << factorial(number) << std::endl;
return 0;
}
Building Projects
One of the most effective ways to learn C++ is to apply your knowledge through hands-on projects. Start with small projects that interest you, such as:
- A personal budget tracker that utilizes file I/O.
- A simple text-based game like Tic-Tac-Toe or Hangman.
These projects will allow you to encounter real-world problems and find solutions, reinforcing your learning.
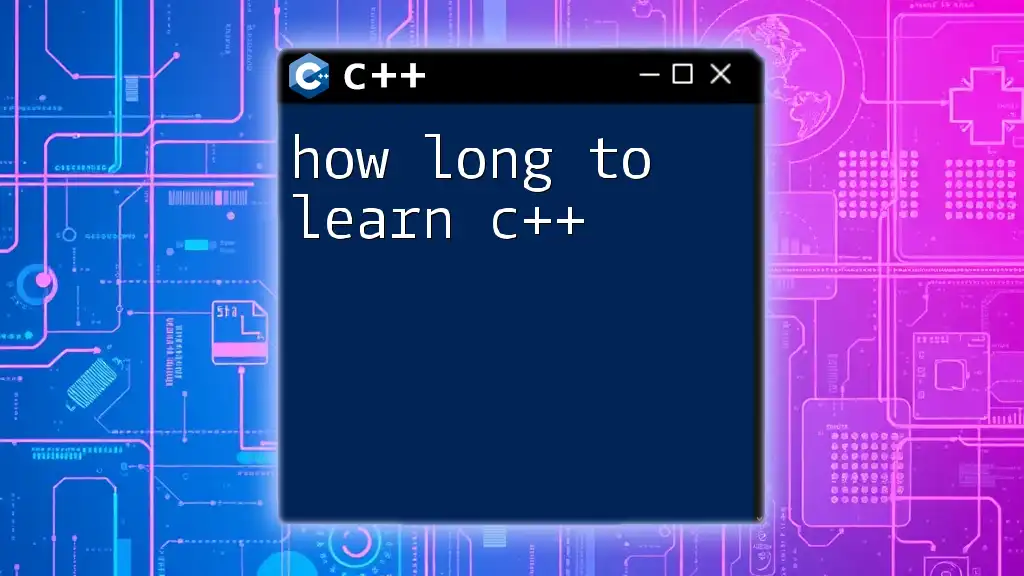
Community and Support
Joining C++ Communities
Finding a community can enhance your learning experience. Online platforms like Stack Overflow and Reddit have dedicated C++ forums where you can ask questions, share knowledge, and connect with fellow learners. In addition, consider joining local meetups or coding clubs to network with like-minded individuals.
Mentorship
Having a mentor can significantly speed up your learning process. Seek guidance from experienced programmers and industry professionals to gain insights and feedback on your progress. Don’t hesitate to reach out to potential mentors or engage in networking events to establish valuable connections.
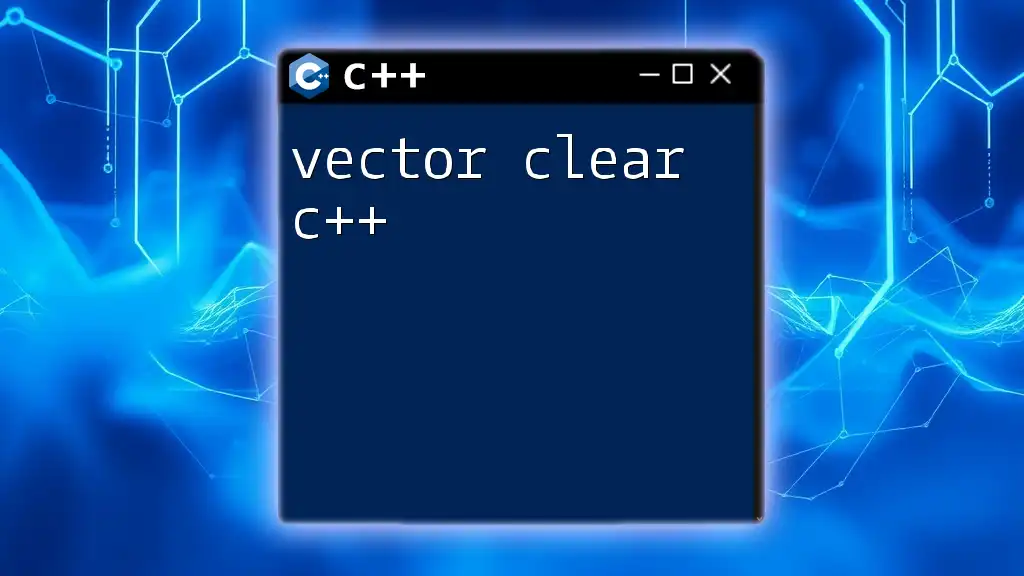
Conclusion
In summary, the best way to learn C++ is a multifaceted approach that combines understanding fundamentals, utilizing quality resources, engaging in practical exercises, and connecting with community support. By setting clear goals, participating in daily challenges, and building projects, you'll pave your way toward mastering this powerful programming language. Embrace the journey, stay consistent, and enjoy the process of discovering the robust world of C++.
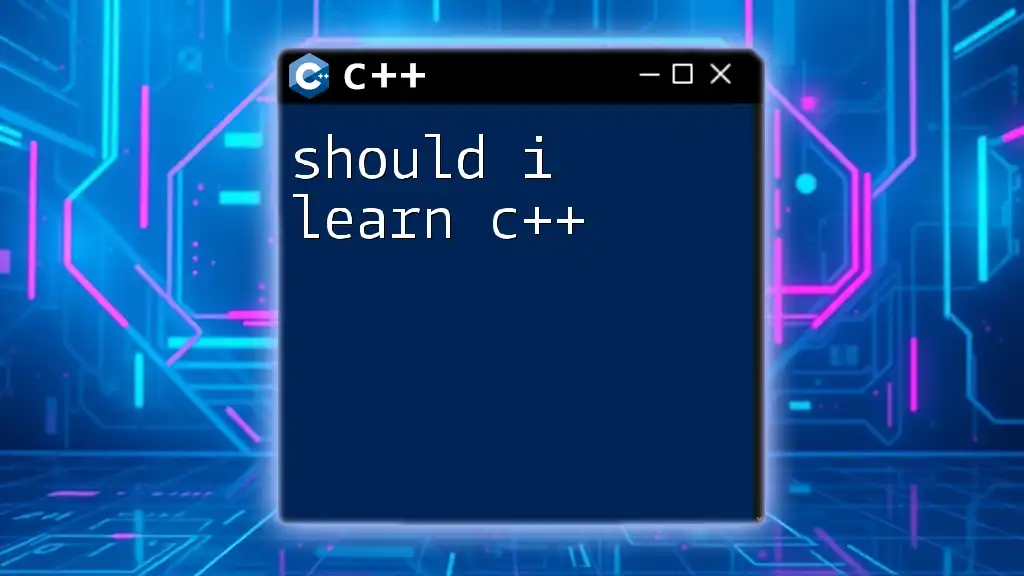
Additional Resources
Furthermore, while learning C++, consider utilizing the following tools and libraries:
- Compilers: Familiarize yourself with GNU GCC or Microsoft Visual C++.
- IDEs: Explore options like Visual Studio, Code::Blocks, or CLion to enhance your coding experience.
- Libraries: Consider learning about Boost and the Standard Template Library (STL) to extend your programming capabilities.
As you continue your journey, seek out additional reading materials and tutorial websites that can provide further insight into C++ programming and its practical applications. Happy coding!