The Tower of Hanoi is a classic recursive problem where the goal is to move a stack of disks from one peg to another while following specific rules, and can be implemented in C++ as shown below:
#include <iostream>
void hanoi(int n, char source, char target, char auxiliary) {
if (n == 1) {
std::cout << "Move disk 1 from " << source << " to " << target << std::endl;
} else {
hanoi(n - 1, source, auxiliary, target);
std::cout << "Move disk " << n << " from " << source << " to " << target << std::endl;
hanoi(n - 1, auxiliary, target, source);
}
}
int main() {
int n = 3; // Number of disks
hanoi(n, 'A', 'C', 'B'); // A, B and C are names of rods
return 0;
}
What is the Tower of Hanoi?
The Tower of Hanoi is a classic mathematical puzzle composed of three rods and a number of disks of different sizes, which can slide onto any rod. The challenge is to move the entire stack from the source rod to the target rod while following these strict rules:
- Only one disk can be moved at a time.
- Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack or an empty rod.
- No disk may be placed on top of a smaller disk.
This puzzle not only offers an intriguing challenge but also serves as an excellent teaching tool in computer science, primarily for illustrating recursive algorithms.
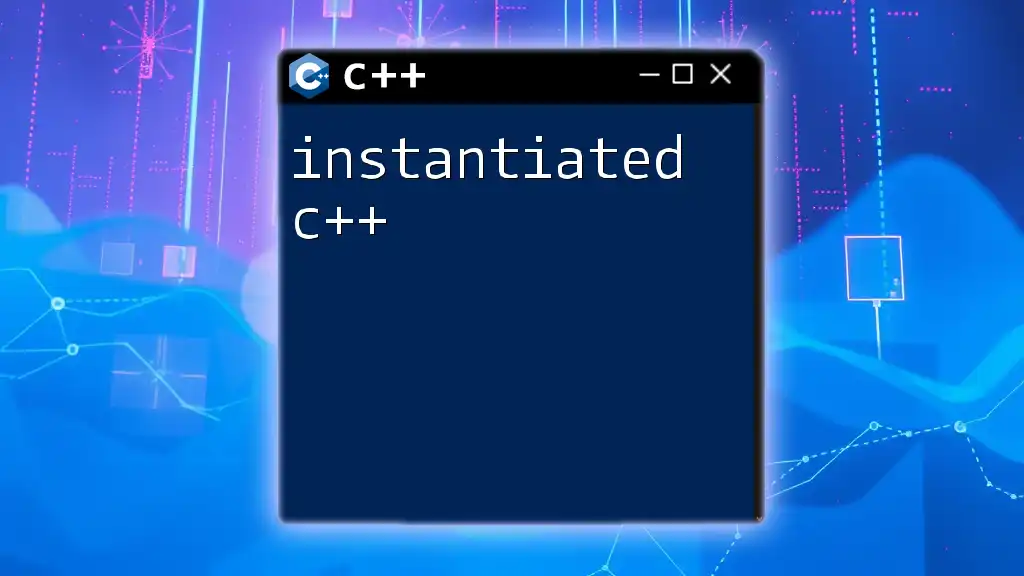
Why Learn the Tower of Hanoi in C++?
Learning to solve the Tower of Hanoi in C++ is essential for understanding recursion and algorithm design. The problem offers insights into how to approach problems through breaking them down into smaller subproblems, a fundamental concept in programming.
Additionally, mastering this puzzle can help develop skills applicable in multiple fields, such as optimization scenarios and game development. Understanding its solution enhances problem-solving capabilities and solidifies your grasp of recursive function implementation.
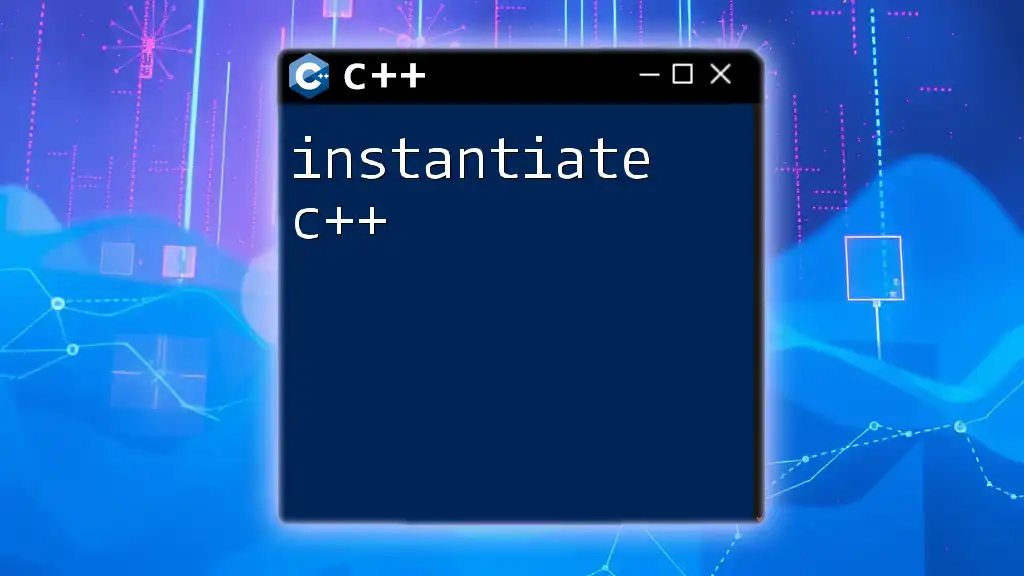
Understanding the Tower of Hanoi Concepts
Structure of the Puzzle
At its core, the Tower of Hanoi consists of:
- Rods: Three vertical sticks where disks can be placed.
- Disks: A finite set of circular objects, each varying in size. They must be stacked in decreasing size from bottom to top.
The visual arrangement of these components is crucial to understand the puzzle's complexity. The disks begin stacked on one rod and must be moved to another, ensuring that larger disks do not sit atop smaller ones.
The Recursive Nature of the Solution
Recursion is a technique in computer science where a function calls itself to solve smaller instances of the same problem. The Tower of Hanoi provides a perfect illustration of this concept. To move `n` disks from one rod to another, the solution can be broken down into simpler parts:
- Move the top `n-1` disks to the auxiliary rod.
- Move the largest disk to the target rod.
- Move the `n-1` disks from the auxiliary rod to the target rod.
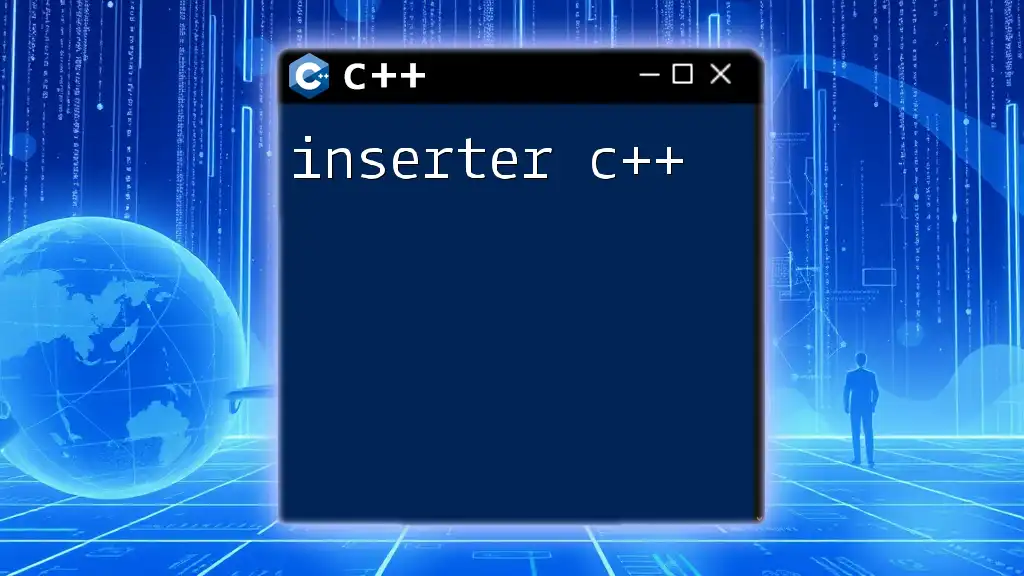
Implementing the Tower of Hanoi in C++
Setting Up the C++ Environment
Before writing the code for the hanoi tower c++, it's essential to set up the C++ environment. This can be done by installing an Integrated Development Environment (IDE) like Code::Blocks, Visual Studio, or even using online compilers like Replit. Once you're equipped, create a new C++ project to house your implementation.
Base Case and Recursive Case
A recursive function typically consists of two critical components:
- Base Case: The condition under which the recursion will stop. For the Tower of Hanoi, this occurs when there is only one disk to move.
- Recursive Case: The process that reduces the problem size, which in this puzzle involves dealing with `n-1` disks recursively.
Step-by-Step Code Walkthrough
Structure of the C++ Program
Start by including necessary headers and structuring the basic framework of the program. Here’s a simple setup for our Tower of Hanoi implementation:
#include <iostream>
using namespace std;
void towerOfHanoi(int n, char from_rod, char to_rod, char aux_rod) {
// Recursive function body will go here
}
int main() {
int n = 3; // Number of disks
towerOfHanoi(n, 'A', 'C', 'B'); // A, B and C are the names of rods
return 0;
}
Recursive Function Implementation
Now, delve into the heart of the program through the recursive function itself. The function should check if there is only one disk to move; if so, it executes the move and returns. If more disks are present, it will perform a sequence of moves.
void towerOfHanoi(int n, char from_rod, char to_rod, char aux_rod) {
if (n == 1) {
cout << "Move disk 1 from rod " << from_rod << " to rod " << to_rod << endl;
return;
}
towerOfHanoi(n - 1, from_rod, aux_rod, to_rod);
cout << "Move disk " << n << " from rod " << from_rod << " to rod " << to_rod << endl;
towerOfHanoi(n - 1, aux_rod, to_rod, from_rod);
}
This code achieves the objective by breaking the problem into manageable moves and ensures disks are arranged according to the rules.
Testing the Code
Once everything is coded, testing it is crucial. Running the program will yield a series of outputs detailing each move required to solve the Tower of Hanoi for three disks, as defined in the `main` function.
Sample Output
If `n` is set to 3, the expected output may look like this:
Move disk 1 from rod A to rod C
Move disk 2 from rod A to rod B
Move disk 1 from rod C to rod B
Move disk 3 from rod A to rod C
Move disk 1 from rod B to rod A
Move disk 2 from rod B to rod C
Move disk 1 from rod A to rod C
This output succinctly conveys the steps taken to solve the puzzle.
Optimizations and Considerations
Understanding Time Complexity
The time complexity of the Tower of Hanoi algorithm is O(2^n), which signifies an exponential growth in the number of moves required as the number of disks increases. This is a significant characteristic to consider when dealing with larger values for `n`.
Alternative Approaches
While recursion is the most intuitive approach for this puzzle, it’s worth noting that non-recursive methods also exist. For instance, an iterative method using a stack data structure may be employed, though it can be more complex.
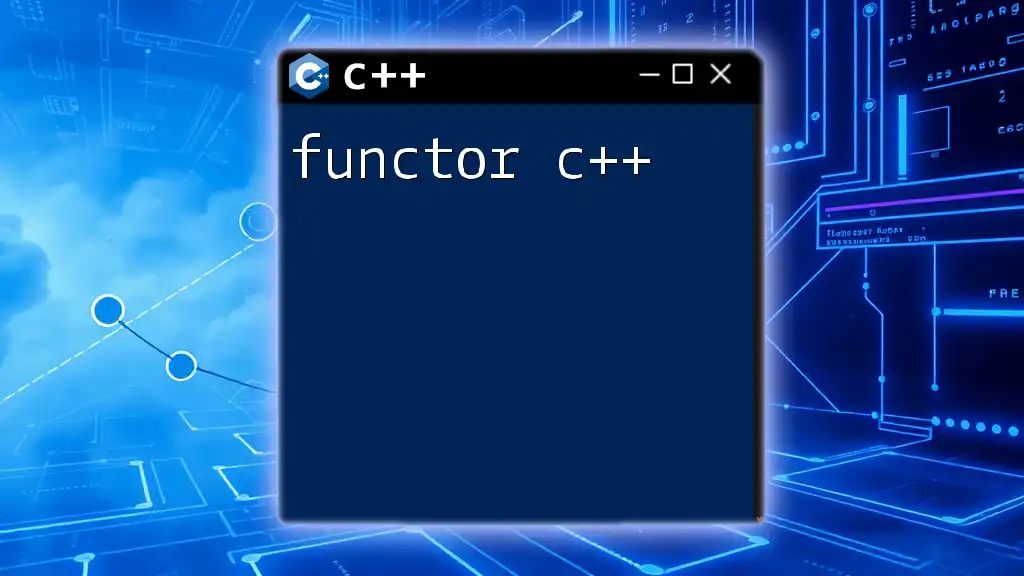
Conclusion
In summary, this exploration of the Tower of Hanoi in C++ has shown its intricate relationship with recursion and algorithmic problem-solving. Understanding this puzzle solidifies your grasp of recursion while improving your ability to tackle more complex programming challenges.
Encouragement to Explore Further
As a final thought, I encourage you to build on this knowledge. Consider variations of the Tower of Hanoi, such as exploring different algorithms, creating a graphical user interface, or applying the concepts learned to other recursive problems.
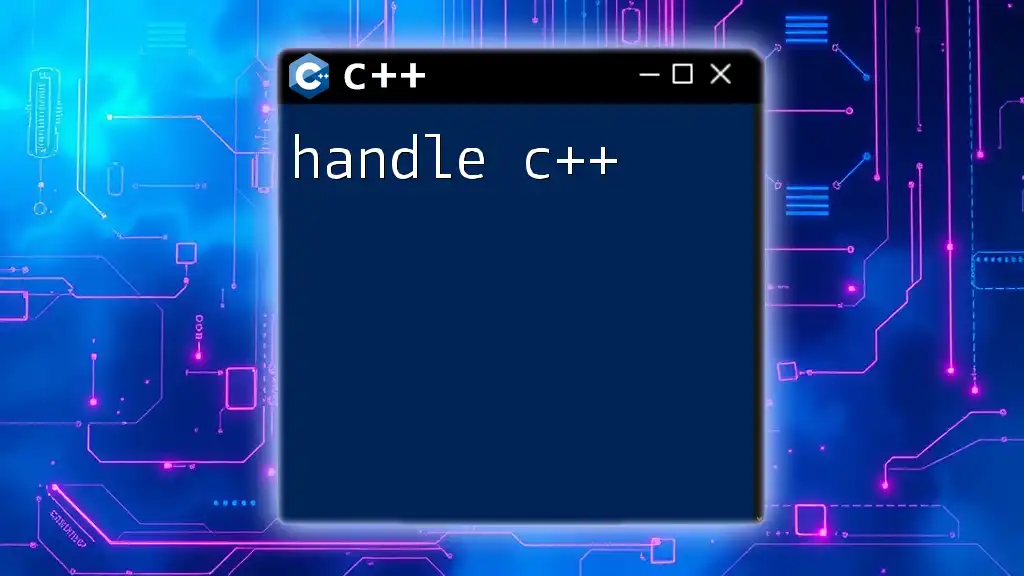
Additional Resources
Online Platforms for Learning C++
For those looking to advance their skills in C++, consider utilizing platforms such as:
- Codecademy
- Udacity
- Coursera
Recommended Books
Some recommended literature for deeper insights include:
- "C++ Primer" by Stanley B. Lippman
- "Effective Modern C++" by Scott Meyers
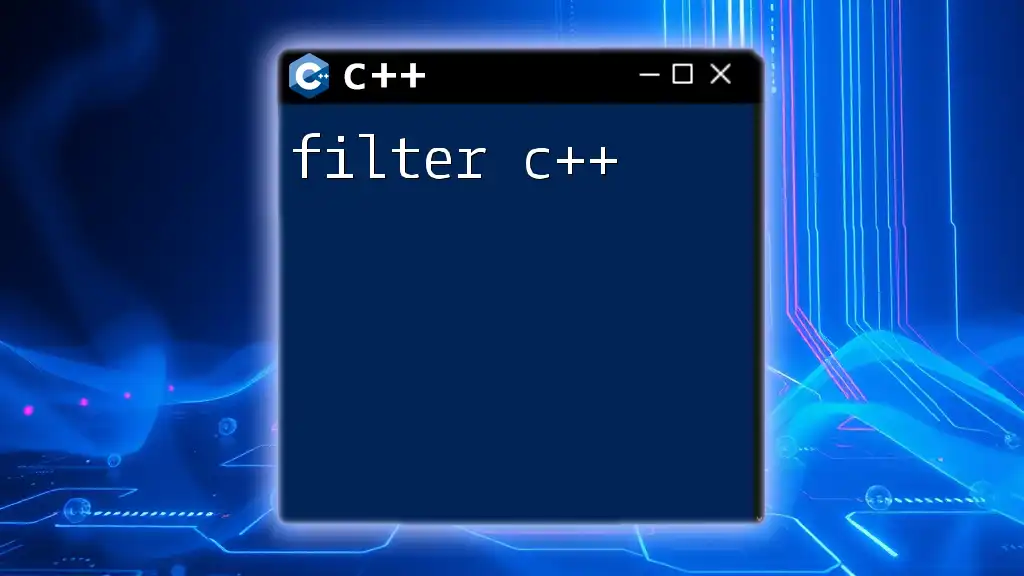
Call to Action
Join our community today to deepen your understanding of C++ concepts like the hanoi tower c++. Together, we can explore more tutorials and offer personalized assistance as you master this elegant language.