To run a C++ program, you need a C++ compiler, a code editor, and the necessary libraries for the specific functionality you intend to implement.
Here's a simple C++ program that demonstrates the required components:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Program Structure
Basic Components of a C++ Program
In C++, all programs follow a specific structure that comprises various components.
Header Files:
Header files are crucial for any C++ program as they contain declarations for functions and variables. Including the right header files at the start of your source code ensures access to essential libraries. The most common header file is `<iostream>`, which is used for input and output operations.
Example of including a header file:
#include <iostream>
Main Function:
Every C++ program must have a `main()` function, where program execution begins. The function typically returns an integer value, representing the success (0) or failure (any non-zero value) of the program's execution.
Example of the structure of a `main()` function:
int main() {
// Code goes here
return 0;
}
Variables and Data Types
Defining Variables:
Variables are containers that hold data values. In C++, every variable must be declared with a specific data type before it can be used. This is essential for memory allocation and type safety within the program.
Example of variable declaration:
int age = 25;
double salary = 65000.50;
Common Data Types:
C++ supports various data types, enabling the programmer to choose the most appropriate one based on the requirement. Common data types include:
- `int`: For integer values.
- `double`: For floating-point numbers.
- `char`: For single character values.
Example of using a character variable:
char initial = 'A';
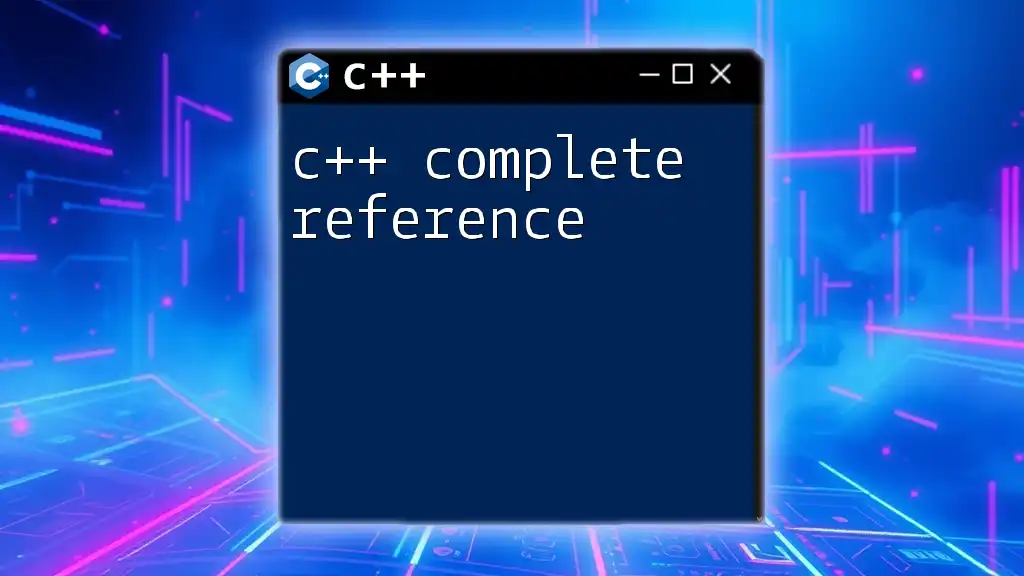
Compiling the Program
What is Compilation?
Compilation is the process of converting the human-readable C++ source code into machine code that the computer can execute. Understanding this process is crucial, as it identifies the various stages involved:
- Preprocessing: The initial stage that processes directives (such as `#include`).
- Compilation: The source code is translated into assembly code.
- Linking: The necessary libraries are linked to create an executable file.
Compilation Tools
Common Compilers Used in C++:
There are several compilers available to compile C++ programs, each with its unique features:
- G++: Part of GNU Compiler Collection, widely used and open-source.
- Clang: Known for its speed and helpful error messages, great for debugging.
- MSVC: A popular choice for Windows development, integrated with Visual Studio.
Example of compiling a C++ program using G++:
g++ -o myprogram myprogram.cpp
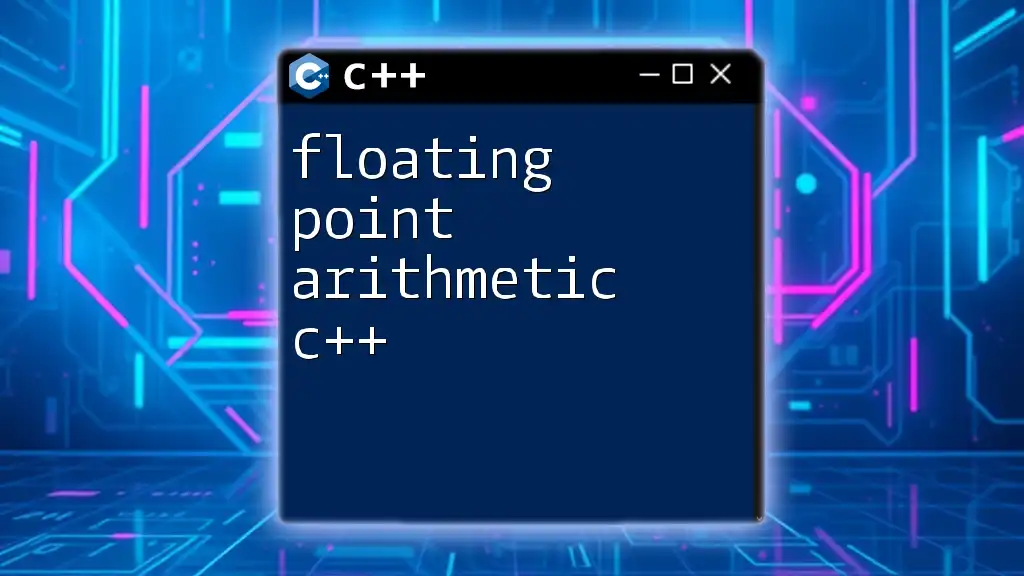
Running the Program
Executable Files
After compilation, the output is usually an executable file. This file is what you run to execute your program. Understanding the difference between source code (your `.cpp` file) and the compiled executable is fundamental for any programmer.
To run the compiled program, use the following command:
./myprogram
Error Handling
While programming, encountering errors is common. Understanding the types of errors and how to fix them is essential:
- Compiler Errors: Issues during the compilation stage, often syntax-related.
- Runtime Errors: Errors that occur while the program is running, such as division by zero or accessing invalid memory.
Example of a compile-time error:
int number = "text"; // Incorrect: inconsistent data types
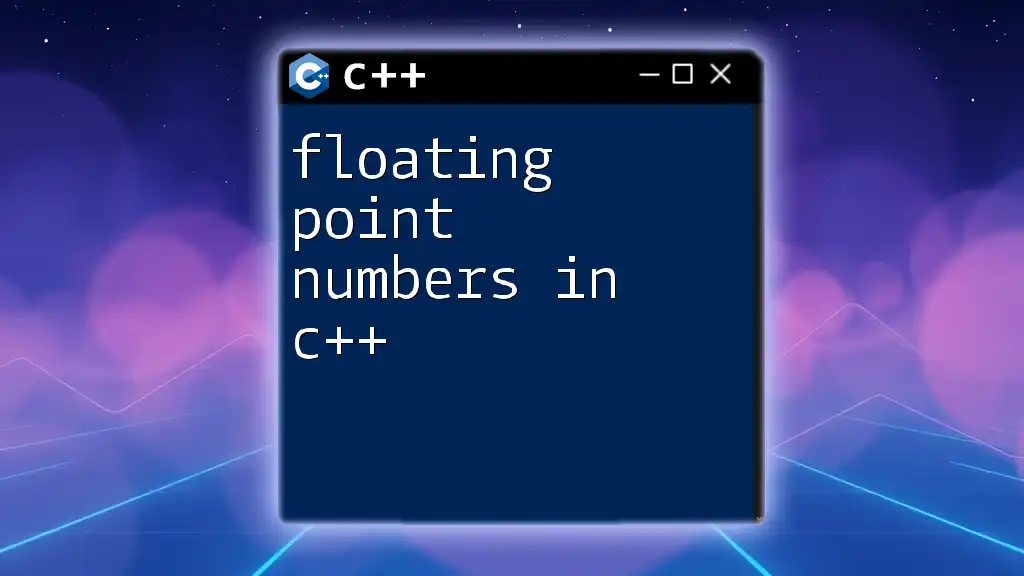
Integrated Development Environments (IDEs)
Popular C++ IDEs and Text Editors
Using an Integrated Development Environment (IDE) simplifies many aspects of C++ programming. IDEs typically come with built-in features such as code highlighting, debugging tools, and compiler integrations.
Overview of IDEs:
- Visual Studio: A comprehensive IDE for Windows with excellent debugging features.
- Code::Blocks: Open-source, lightweight, suitable for beginners.
- CLion: A robust IDE from JetBrains focused on productivity.
Simple Text Editors:
If you prefer a lightweight approach, text editors like Notepad++ and Sublime Text can also be used, although they may require additional setup for compiling and executing code.
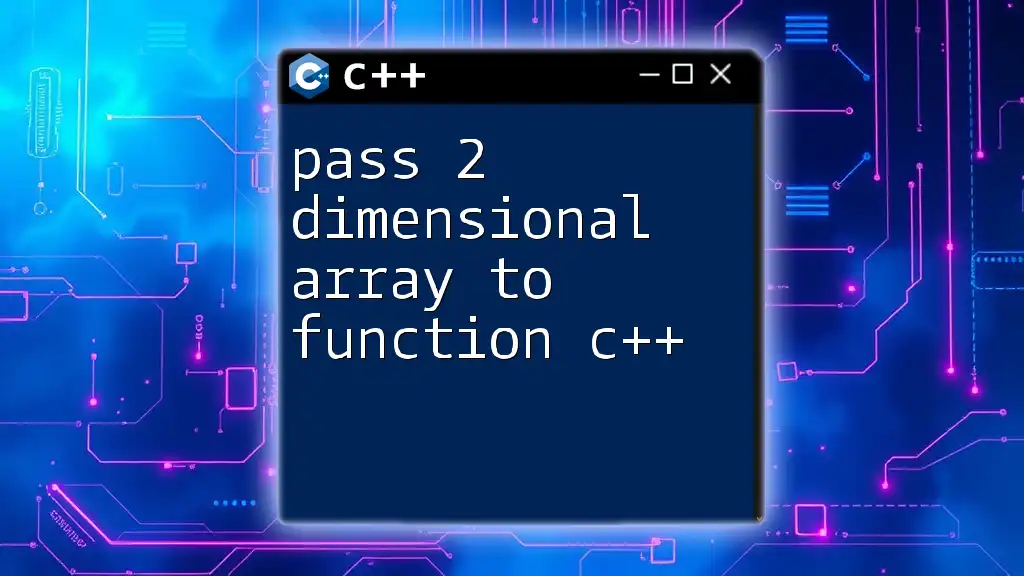
Additional Components for Advanced Programs
Libraries and Frameworks
As you delve deeper into C++, utilizing libraries and frameworks can greatly enhance functionality while reducing the time you would spend coding from scratch. The Standard Template Library (STL) is one of the most important libraries, providing a rich set of algorithms and data structures.
Example of using STL in your program:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4};
Debugging Tools
Debugging is an integral part of the development process. Utilizing debugging tools can save a significant amount of time and frustration.
Debugging a C++ Program:
Tools such as GDB (GNU Debugger) are quintessential for identifying runtime errors. They allow you to step through your code, inspect variable values, and diagnose issues effectively.
Basic commands to initiate debugging with GDB:
gdb myprogram
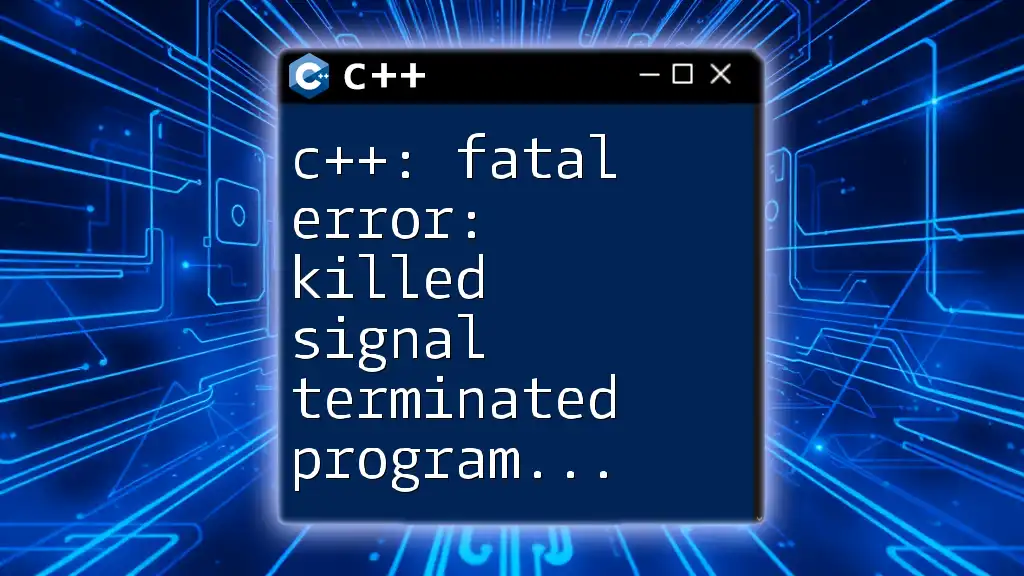
Conclusion
In summary, the following components are required to run this program c++ include understanding the program structure, compiling, execution, and awareness of errors. Engaging with IDEs, libraries, and debugging tools further enhances your C++ programming experience. By mastering these components, you'll be well-equipped to write efficient C++ programs and tackle more complex projects in the future. Happy coding!