Microsoft Visual C++ is an integrated development environment (IDE) that provides tools for developing, debugging, and compiling C++ applications on Windows platforms.
Here's a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Microsoft Visual C++?
Definition of Microsoft Visual C++
Microsoft Visual C++ (MSVC) is an integrated development environment (IDE) developed by Microsoft for C and C++ programming. It provides a comprehensive set of tools to create, debug, and optimize applications primarily on the Windows platform. MSVC simplifies the complexity of C++ programming by providing features such as a powerful code editor, integrated debugging, and a rich set of libraries.
Historical Context
The history of MSVC traces back to its early versions in the 1990s, where it served primarily as a compiler and editor. Over the years, Microsoft has released multiple versions, progressively enhancing its capabilities with features like code optimization, CLR integration, and improved debugging tools. By consistently evolving, MSVC has become a premier choice for C++ developers looking to build applications efficiently.
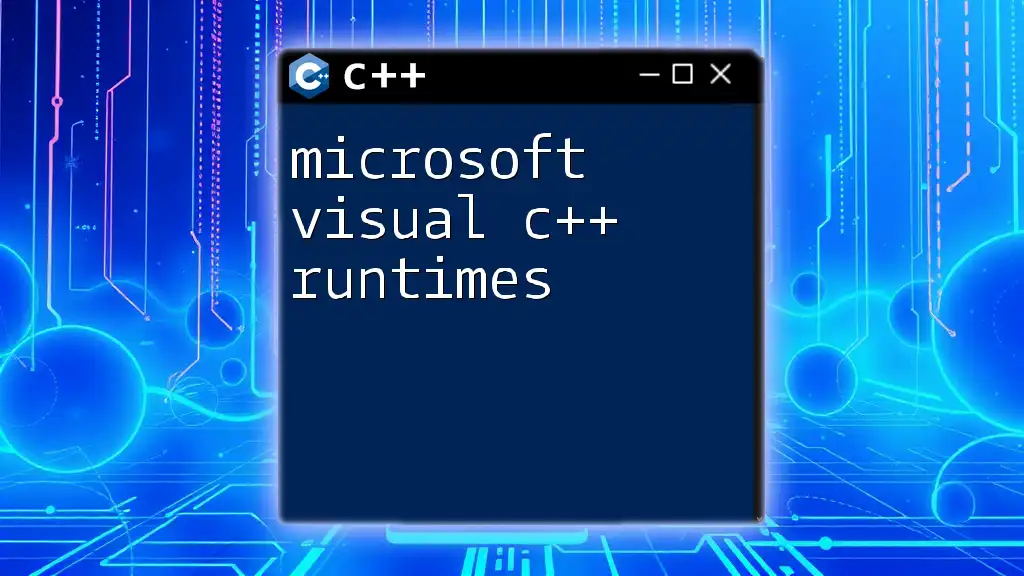
Features of Microsoft Visual C++
Integrated Development Environment (IDE)
The heart of Microsoft Visual C++ is its integrated development environment, which combines essential features into a single user interface.
Code Editor
The code editor in MSVC is designed to enhance productivity. Key features include:
- Syntax Highlighting: This feature colors code elements based on their roles, making it easier to read and understand complex code at a glance.
- IntelliSense: With IntelliSense, developers receive live code suggestions, function parameter info, and quick documentation as they type, reducing errors and speeding up development.
Example of Features
// Using IntelliSense for function suggestions
std::cout << "Hello, World!" << std::endl;
Compilers and Build Tools
MSVC includes a robust compiler that translates C++ source code into executable programs. The compiler supports various C++ standards, ensuring compatibility with modern C++ code.
Code Example
Consider this simple C++ program, which demonstrates the basic usage of the compiler.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code can be compiled and executed seamlessly within MSVC, showcasing its ability to manage basic commands effortlessly.
Debugging Capabilities
Debugging is where MSVC truly shines. The environment comes equipped with sophisticated debugging tools that allow developers to identify and fix issues efficiently.
- Breakpoints: Developers can set breakpoints in their code to pause execution and examine variables and program flow.
- Watches: This feature allows monitoring the values of variables in real-time while the program runs.
- Memory Diagnostics: The IDE provides tools to analyze memory usage, helping to identify leaks or improper usage of resources.
Example
Consider the need to trace a logic error in a complex algorithm. With MSVC, a developer can set a breakpoint right before a critical section of code and step through each line, verifying variable states at each step.
Libraries and Frameworks
MSVC provides access to the standard library and the Standard Template Library (STL), which includes a wide range of pre-built functions and data structures. Additionally, MSVC supports numerous third-party libraries, enhancing its capabilities for specific application needs.
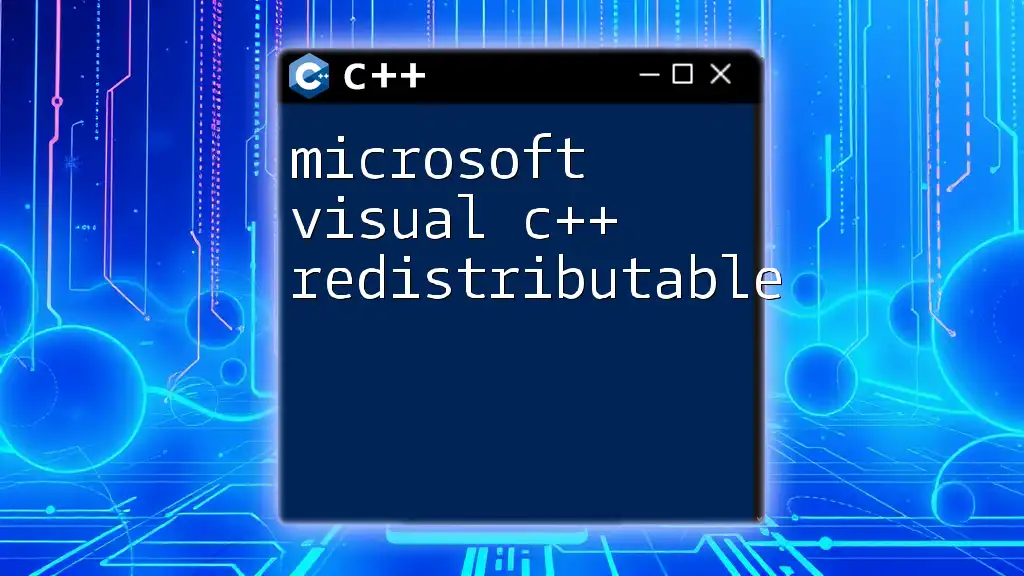
Using Microsoft Visual C++ for Development
Setting Up Microsoft Visual C++
The first step to using Microsoft Visual C++ is installing the IDE. Users can download Visual Studio, which integrates MSVC, and follow the installation prompts.
Quick configuration tips include selecting C++ development workloads during setup, ensuring that all necessary components for C++ programming are installed efficiently.
Creating Your First C++ Project
After installation, creating your first C++ project is an exciting venture. Upon launching MSVC, navigate to File > New > Project, and select a Console Application.
Example Project
Set the project name and location. Once created, the IDE will generate a basic structure, and you can adjust project properties such as include directories, output paths, and more to suit your needs.
Writing Code in MSVC
Best Practices for Code Structure
Writing clean, maintainable code is crucial in any programming language, including C++. It's essential to follow best practices such as consistent naming conventions and thorough documentation.
Code Snippet Example
Here’s a well-structured code example demonstrating these principles:
#include <iostream>
// Function to add two numbers
int add(int a, int b) {
return a + b; // Return the sum of a and b
}
int main() {
int result = add(5, 10); // Adding two integers
std::cout << "The result is: " << result << std::endl; // Displaying the output
return 0;
}
This code illustrates clarity and maintainability — a good practice for any developer looking to create understandable programs.
Compilation and Execution
Compiling and executing code in MSVC is straightforward. Users can build their project using the Build menu and select either Debug or Release configuration, depending on their needs.
Step-by-Step Example
This process allows for optimized performance in the Release mode while providing detailed debugging information in Debug mode. Simply press F5 to run the application, and the output will be displayed in the integrated terminal.
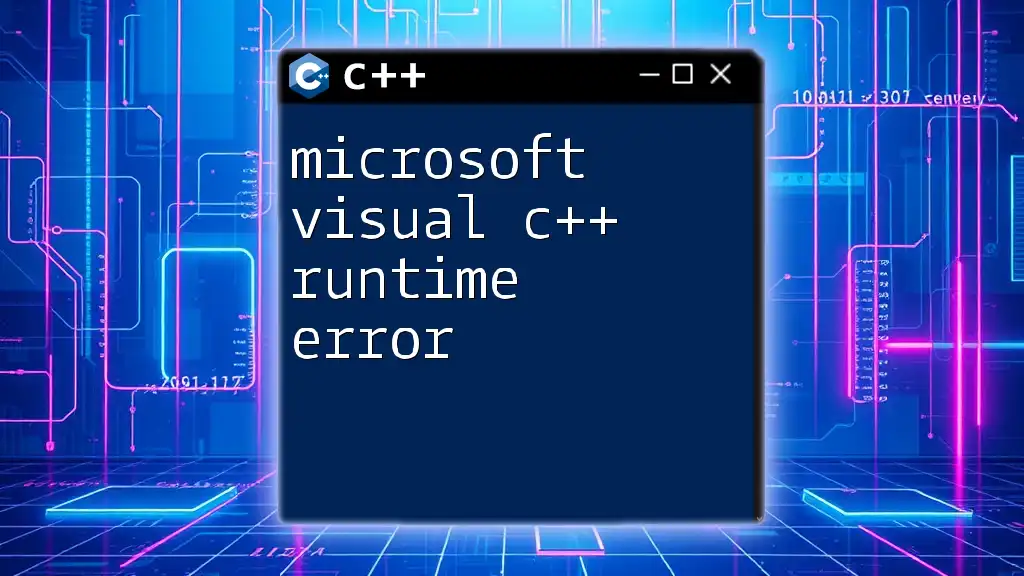
Advanced Features of Microsoft Visual C++
Performance Profiling
MSVC includes tools for performance profiling, enabling developers to analyze and improve application performance. By examining CPU usage and memory consumption, programmers can make informed adjustments to enhance efficiency.
Static Code Analysis
Static code analysis is an essential part of the development cycle. MSVC integrates static analysis tools to identify code quality issues before running the program, ensuring that potential bugs and vulnerabilities are addressed early.
Cross-Platform Development
In today's environment, cross-platform capabilities are increasingly important. MSVC supports cross-platform development through tools like CMake, enabling users to build applications for various operating systems while maintaining a single codebase. This flexibility ensures that developers can target multiple platforms without significant overhead.
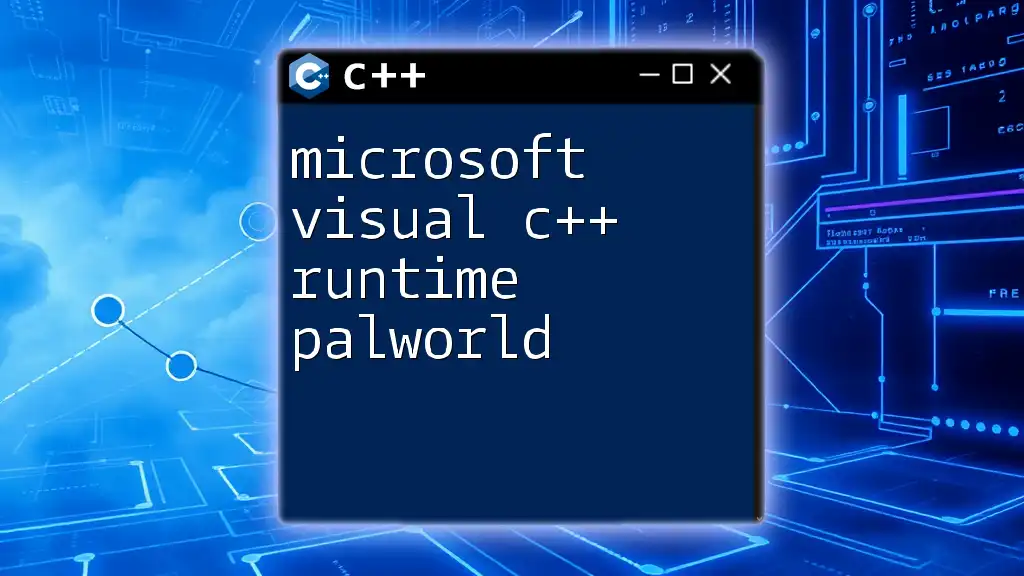
Common Issues and Troubleshooting
Debugging Common Errors
Despite its robust tools, developers may encounter common compilation and runtime errors while using MSVC. Typical issues include unresolved externals, missing include files, or linkage errors.
Example Error and Fix
Suppose you encounter an "unresolved external symbol" error. This may occur if you've declared but not defined a function. Ensuring that every declared function has a corresponding definition will resolve this issue effectively.
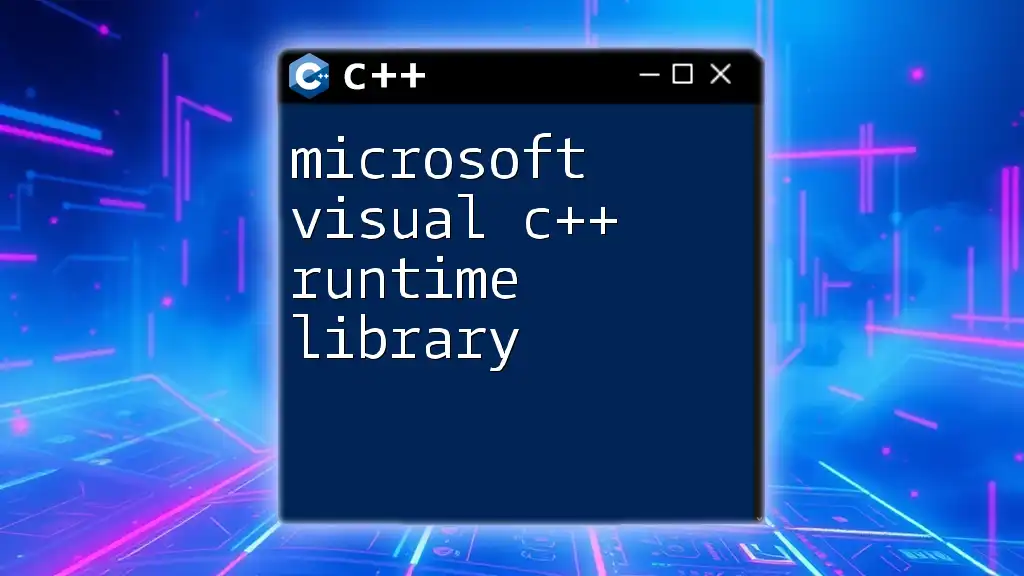
Conclusion
In summary, Microsoft Visual C++ serves as a powerful IDE for C++ development, integrating features that streamline the coding, debugging, and project management process. By leveraging the capabilities of MSVC, developers can enhance their productivity and create efficient, high-quality applications. Exploring this environment offers a significant advantage for anyone diving into C++ programming, making MSVC a preferred choice in the development community.
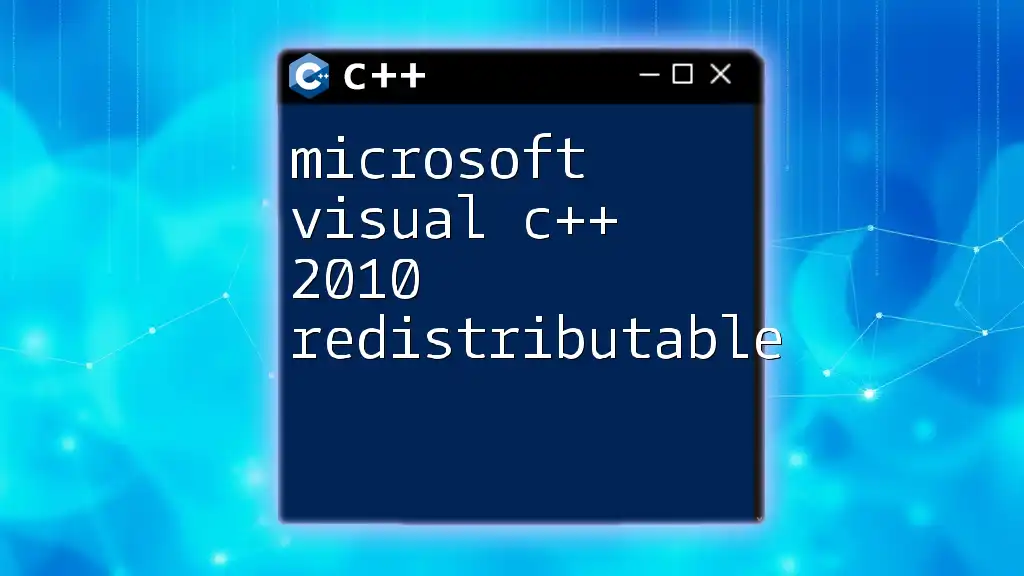
Additional Resources
For further learning and resources, consider exploring the official Microsoft documentation, tutorials, and community forums. These tools will provide invaluable insights and assistance on your programming journey with Microsoft Visual C++.