A C++ virtual lab allows users to experiment with and execute C++ commands in a simulated environment, enhancing their programming skills and understanding of concepts.
Here’s a simple example of a C++ command that demonstrates a virtual function:
#include <iostream>
using namespace std;
class Base {
public:
virtual void show() { // virtual function
cout << "Base class show function called." << endl;
}
};
class Derived : public Base {
public:
void show() override { // overriding the base class function
cout << "Derived class show function called." << endl;
}
};
int main() {
Base* b; // Base class pointer
Derived d; // Derived class object
b = &d; // Base class pointer assigned to derived class object
b->show(); // Calls derived class show function
return 0;
}
What is a CPP Virtual Lab?
A CPP Virtual Lab is an interactive environment designed to facilitate learning and experimentation with C++ programming. It allows users to write, compile, and run C++ code in a safe, isolated setting. This environment is particularly important for beginners who are just getting started, as it provides an opportunity for hands-on practice without the fear of corrupting a main system or project. The importance of real-time coding practice cannot be understated; it bridges the gap between theoretical knowledge and practical application.
Benefits of Using a Virtual Lab
Using a CPP virtual lab comes with numerous benefits:
- Safe experimentation: Users can experiment with different C++ commands without the risk of affecting their local machine.
- Immediate feedback: Virtual labs typically provide instant results, which helps learners quickly understand the consequences of their code.
- Enhanced understanding: Engaging with code during a live session promotes deeper comprehension through active participation.
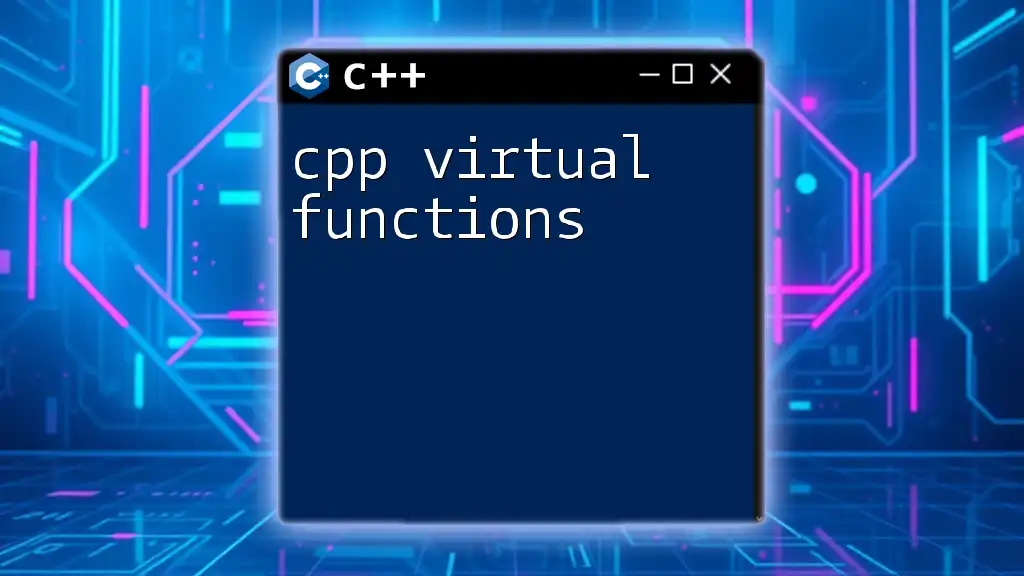
Setting Up Your CPP Virtual Lab
Choosing a Virtual Lab Environment
Selecting the right platform can greatly enhance your learning experience. Popular C++ virtual lab platforms include:
- Replit: A collaborative platform with an intuitive interface.
- CodeChef: Great for competitive programming and offers code challenges.
- OnlineGDB: Supports multiple programming languages, including C++, and is useful for debugging.
Each platform has its own advantages, so it’s beneficial to explore a few options to find the one that fits your style best.
Installation and Setup Instructions
To get started with your CPP virtual lab, follow these simple setup instructions. For this example, we'll use Replit:
- Sign Up: Visit the Replit website and create an account.
- Create a New Project: After logging in, select the option to create a new repl and choose C++ from the list of languages.
- Write Your First Program: Use the provided code editor to write your first C++ program. Here’s a classic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Click the Run button, and you should see the output: `Hello, World!` You've now completed your first step into the world of C++ programming!
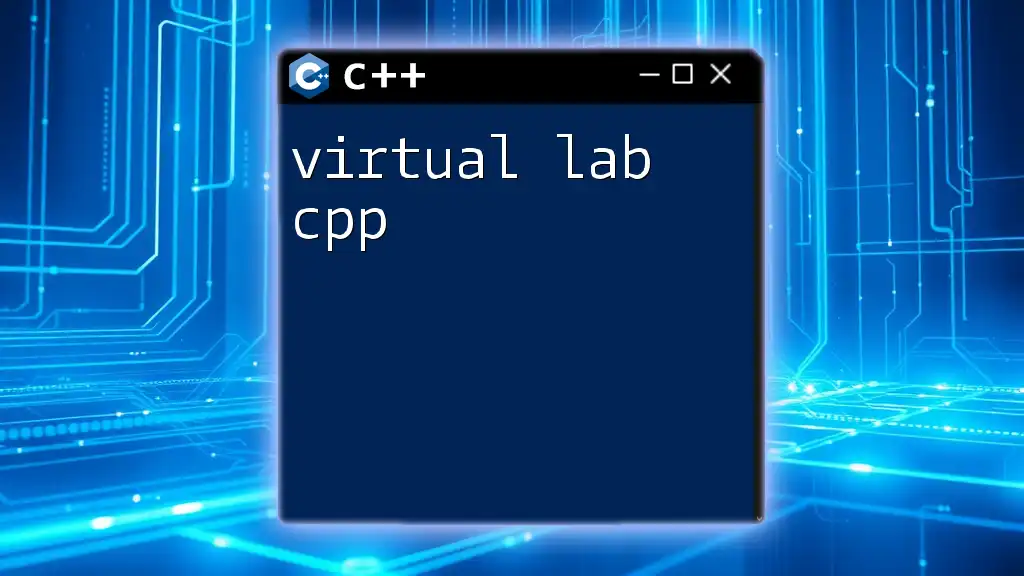
Navigating the CPP Virtual Lab Interface
Main Features to Look Out For
A typical CPP virtual lab comes equipped with various essential features, including:
- Code Editor: This is where you'll spend most of your time writing C++ code. Look for syntax highlighting and auto-completion features, as they can accelerate the coding process.
- Compiler: Understanding how to compile your code is crucial. The compiler transforms your code into an executable file that runs on the computer.
- Output Console: Use the output console to view the results of your code and any debugging messages that can help you track down errors.
Additional Tools Offered
Many CPP virtual labs offer supplementary tools that can enhance your coding experience:
- Collaboration Tools: Features that allow you to share your code with friends or work on projects together.
- Version Control Integration: Tools like Git help maintain a history of changes.
- Enhanced Debugging Features: Visual aids that highlight errors in your code can make troubleshooting more intuitive.
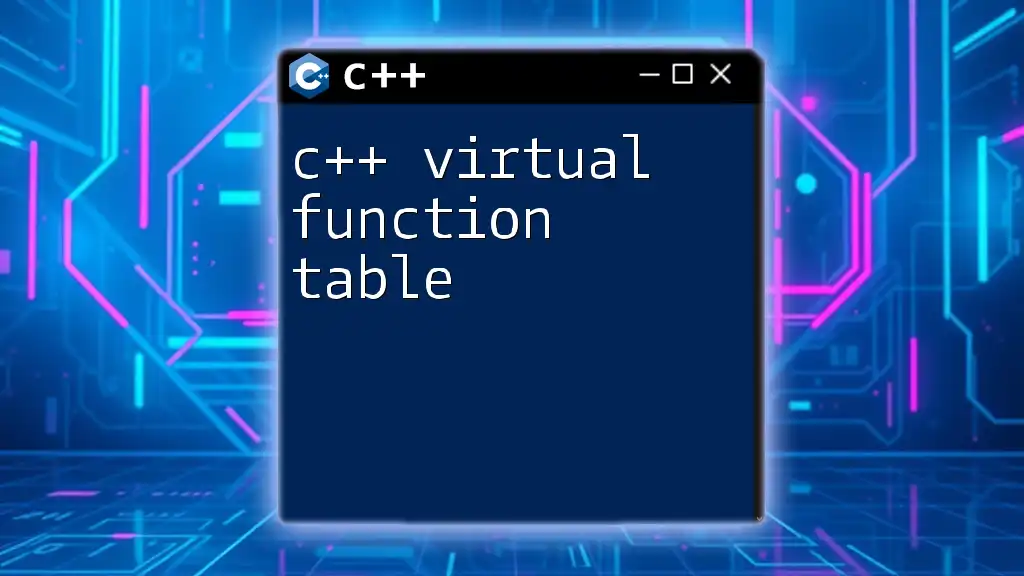
Learning CPP Commands in a Virtual Lab
Basic C++ Commands
Understanding Data Types
In C++, data types play a vital role in defining what kind of values a variable can hold. Here are some fundamental C++ data types:
- int: Integer values
- float: Decimal values
- char: Single characters
You can declare variables of these types as shown in the example below:
int age = 25;
float height = 5.9;
char initial = 'A';
Control Structures
Control structures dictate how your program flows. Common structures include conditionals and loops. Here’s a simple `for` loop to print numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
Advanced CPP Concepts
Classes and Objects
One of the cornerstones of C++ is Object-Oriented Programming (OOP), which revolves around the concepts of classes and objects. Here’s how you can create a simple class in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this example, the `Dog` class has a single method called `bark`. You can create an object of `Dog` and call this method as follows:
Dog myDog;
myDog.bark();
Inheritance and Polymorphism
Inheritance allows you to create a new class that is based on an existing class, thus promoting code reusability. Here’s an example:
class Animal {
public:
virtual void sound() {
std::cout << "Animal sound" << std::endl;
}
};
class Cat : public Animal {
public:
void sound() override {
std::cout << "Meow!" << std::endl;
}
};
In this example, `Cat` inherits from `Animal`, and you can override the method to provide specific functionality.
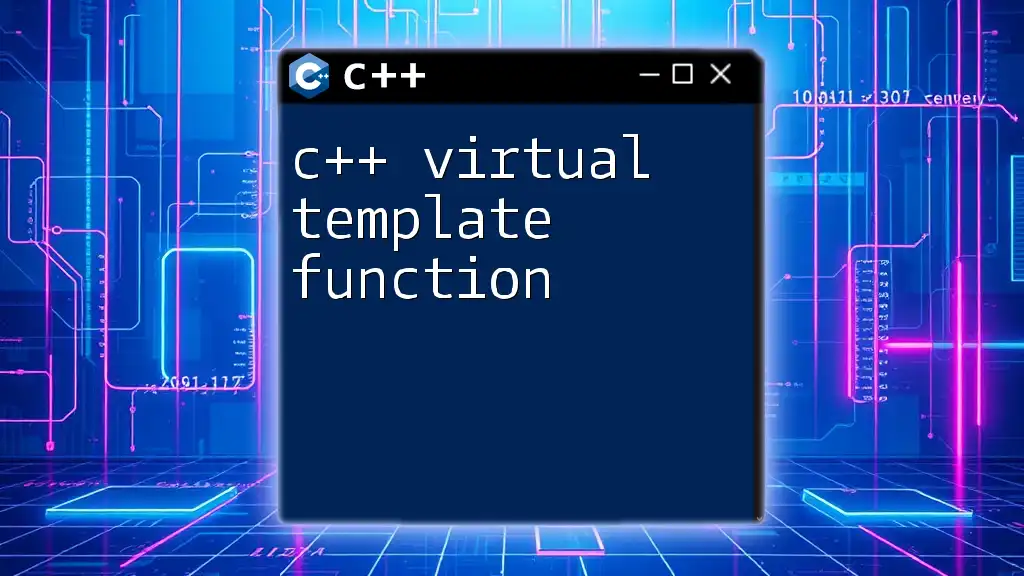
Practical Exercises in the Virtual Lab
Exercise Ideas for Beginners
As a beginner, some useful exercises may include:
- Simple Calculator: Create a program that performs basic arithmetic operations.
- Array Manipulation: Write a program to reverse an array and display the results.
Intermediate and Advanced Exercises
For those with a bit more experience, consider trying:
- Tic-Tac-Toe Game: Build a simple console-based version of this classic game.
- File I/O Application: Create a program that reads from and writes to a text file to understand how data persistence works.
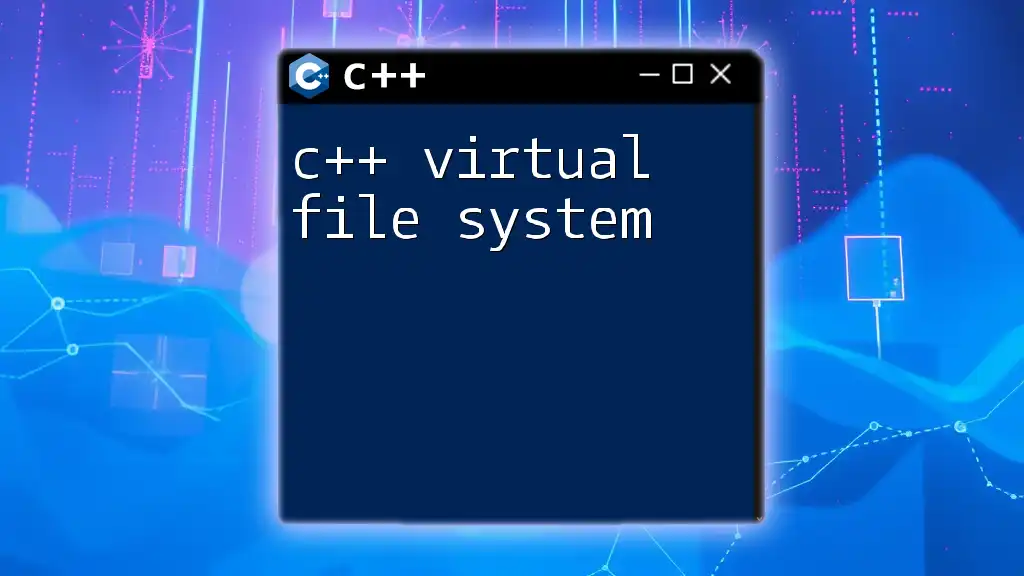
Troubleshooting Common Issues
Compilation Errors
Often, the first hurdle you will encounter while coding in a CPP virtual lab is compilation errors. Common error messages might include syntax errors or missing semicolons. Understanding these messages and what they mean is essential for becoming proficient in CPP.
Runtime Errors
Unlike compilation errors, which stop your code from running, runtime errors occur while your program is executing. Logical errors are particularly challenging, as the program compiles and runs, but does not produce the expected results. Utilize debugging tools provided by your virtual lab to step through your code and identify where things go wrong.
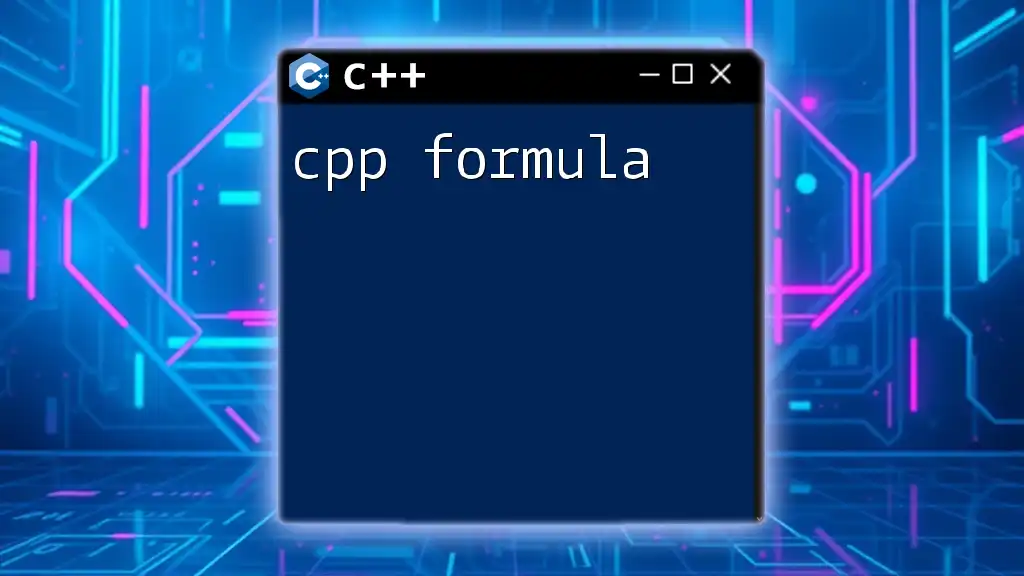
Conclusion
A CPP virtual lab is an invaluable resource for aspiring C++ programmers. It offers a unique platform for hands-on experience, facilitating learning through practice. By leveraging the features available in virtual labs, you can effectively gain a robust understanding of C++ programming concepts.
Call to Action
Ready to dive deeper? Join our C++ course today for structured guidance and additional resources on mastering C++. Whether you're a beginner or looking to refine your skills, there's something for everyone! Feel free to leave any questions or feedback in the comments below!