A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers, and you can determine if a number is prime in C++ using the following code snippet:
#include <iostream>
using namespace std;
bool isPrime(int n) {
if (n <= 1) return false;
for (int i = 2; i * i <= n; i++) {
if (n % i == 0) return false;
}
return true;
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << (isPrime(num) ? "Prime" : "Not Prime") << endl;
return 0;
}
What is a Prime Number?
A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. In other words, a prime number only has two distinct positive divisors: 1 and itself. This unique property is what makes prime numbers fundamental in the field of mathematics and a critical element in various algorithms.
Importance in Mathematics and Computer Science
Prime numbers play a vital role in numerous mathematical theories and practical applications. They are essential in:
- Cryptography: The security of data encryption relies heavily on the properties of prime numbers.
- Random Number Generation: Prime numbers are often used in algorithms that require randomness due to their unpredictability.

Understanding Prime Numbers in C++
In the realm of programming, particularly in C++, understanding how to efficiently find and verify prime numbers is crucial for tasks ranging from algorithm development to data security. Mastering prime number manipulation can significantly enhance a programmer's problem-solving toolkit.
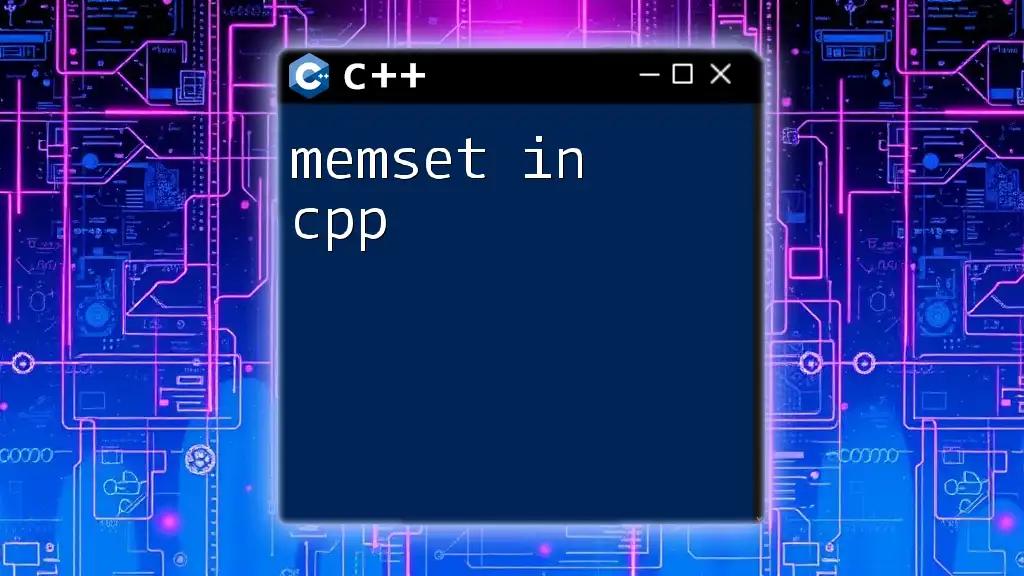
C++: A Brief Overview
Why Use C++ for Mathematical Computations?
C++ is known for its performance optimization and fine control over system resources. This makes it a preferred language for developing mathematical algorithms, enabling developers to implement efficient methods for tasks such as prime number generation. Its rich standard library provides various tools that facilitate mathematical programming and algorithm design.
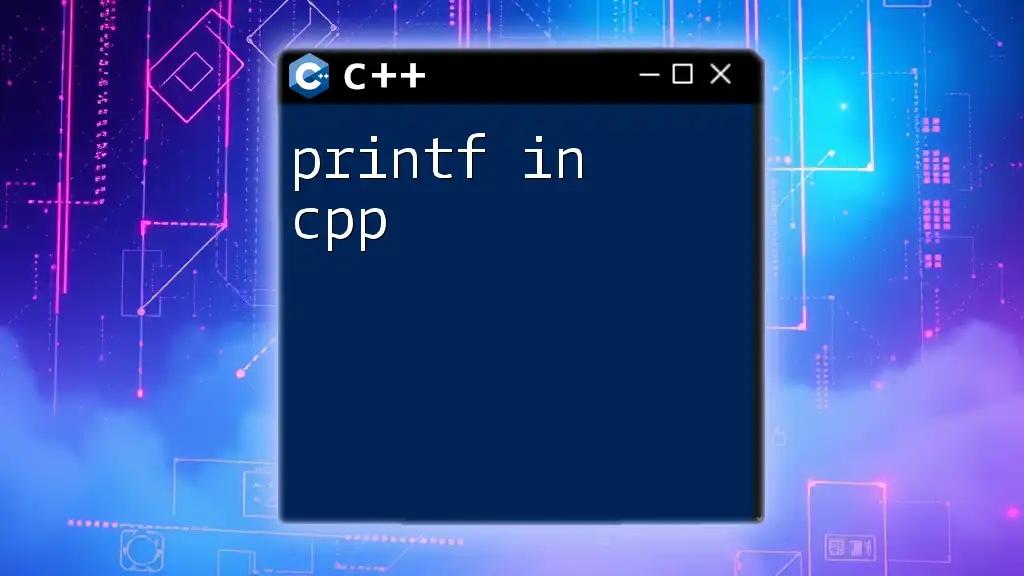
Finding Prime Numbers: The Basics
Understanding the Characteristics of Prime Numbers
To effectively work with prime numbers, a programmer should comprehend their characteristics:
- The smallest prime number is 2, which is also the only even prime.
- All other prime numbers are odd, meaning they cannot be evenly divided by 2.
The Challenge of Finding Prime Numbers
Finding prime numbers can be computationally intensive, especially as numbers grow larger. Efficient algorithms are necessary to avoid excessive processing time and resources when determining the primality of a number.
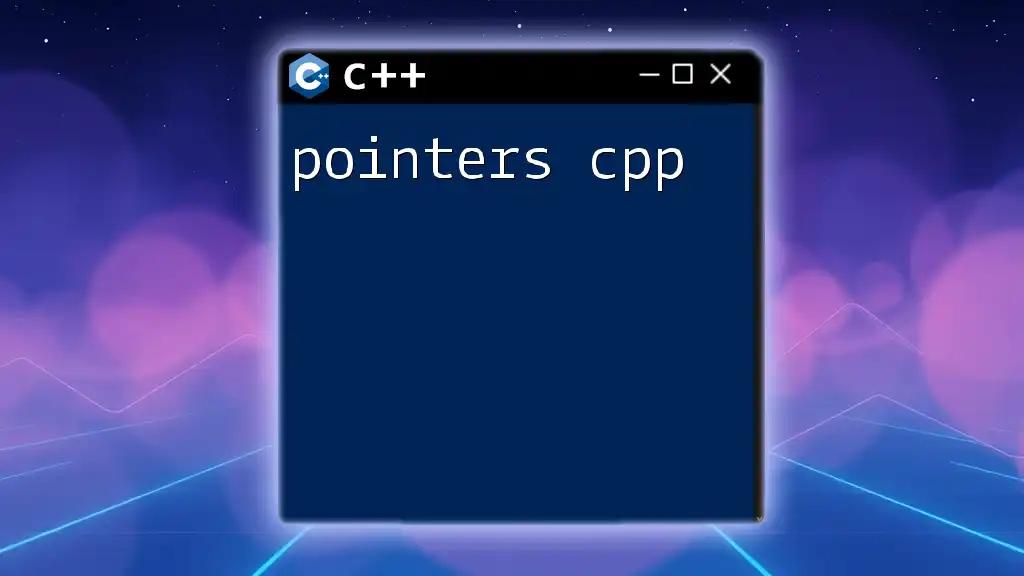
Methods to Determine Prime Numbers in C++
Brute Force Method to Find Prime Numbers
The simplest approach to check if a number is prime is through the brute-force method. This method tests every integer up to the number itself to determine if any divisors exist.
Here is a sample implementation:
#include <iostream>
using namespace std;
bool isPrime(int n) {
if (n <= 1) return false;
for (int i = 2; i <= n / 2; ++i) {
if (n % i == 0) return false;
}
return true;
}
int main() {
for (int num = 1; num <= 100; num++) {
if (isPrime(num))
cout << num << " ";
}
return 0;
}
Explanation of the Code
- Function Declaration: The `isPrime` function checks for primality. If `n` is less than or equal to 1, it returns false.
- Looping through Potential Divisors: The for-loop checks for divisibility from 2 up to n/2. If `n` is divisible by any of these numbers, it returns false.
- Main Function: The main function iterates from 1 to 100, printing out prime numbers by calling `isPrime`.
Optimized Method: Sieve of Eratosthenes
While the brute-force method is straightforward, it can be improved significantly using the Sieve of Eratosthenes algorithm. This ancient algorithm efficiently finds all prime numbers up to a given limit.
Here’s how to implement it in C++:
#include <iostream>
#include <vector>
using namespace std;
void SieveOfEratosthenes(int n) {
vector<bool> prime(n + 1, true);
for (int p = 2; p * p <= n; p++) {
if (prime[p] == true) {
for (int i = p * p; i <= n; i += p)
prime[i] = false;
}
}
for (int p = 2; p <= n; p++) {
if (prime[p])
cout << p << " ";
}
}
int main() {
int n = 100;
SieveOfEratosthenes(n);
return 0;
}
Explanation of the Code
- Vector Initialization: A boolean vector `prime` is initialized to mark all numbers as potentially prime.
- Outer Loop: The outer loop iterates through all numbers starting from 2 to the square root of n to determine non-prime numbers.
- Inner Loop: For each identified prime, the inner loop marks all multiples as non-prime, thus efficiently reducing the amount of checks needed.
- Output: Finally, it prints the numbers still marked as true in the vector, which are the prime numbers.

Practical Applications of Prime Numbers in C++
Prime Numbers in Cryptography
One notable application of prime numbers is in the field of cryptography, especially in algorithms like RSA. Prime factors are used to generate keys that ensure secure communication over the internet. The difficulty of factoring large numbers into their prime constituents is what keeps encrypted data safe.
Finding Prime Numbers in Real-World Applications
Beyond cryptography, prime numbers are essential in various fields. For instance, they help in hash functions for data storage systems and are utilized in random number generation techniques for simulations.

Testing for Prime Numbers in C++
Unit Testing Your Prime Functions
Testing is an essential part of programming that ensures the reliability of your algorithms. Here are some sample test cases to check the correctness of the prime-checking function:
assert(isPrime(5) == true);
assert(isPrime(4) == false);
assert(isPrime(29) == true);
assert(isPrime(1) == false);
assert(isPrime(97) == true);
Testing helps catch potential issues early and guarantees that the functions perform as expected across a range of input values.
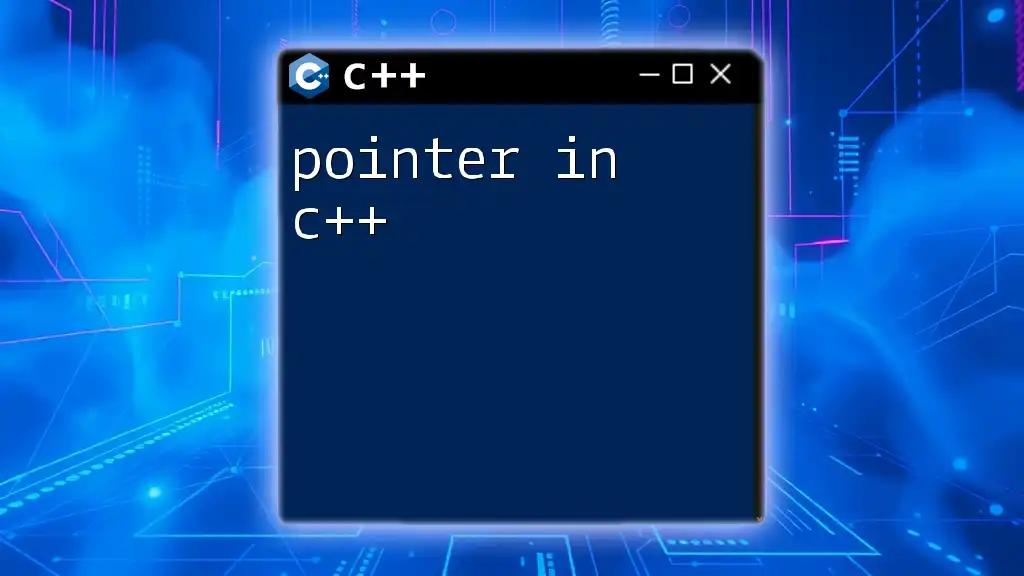
Common Mistakes and Misconceptions
When dealing with prime numbers in C++, programmers may encounter several pitfalls:
- Assuming All Even Numbers Are Non-Prime: While it’s true that all even numbers except 2 are non-prime, failing to account for 2 will lead to inaccurate results.
- Inefficient Algorithms: Relying solely on brute force for large inputs can cause performance bottlenecks. Utilizing methods like the Sieve of Eratosthenes will yield better results.

Conclusion
In summary, understanding how to work with prime numbers in C++ is a vital part of programming. From basic identification methods to advanced applications in cryptography, prime numbers hold immense value. By familiarizing yourself with these concepts and implementing them in C++, you can develop efficient algorithms that solve a variety of computational problems.
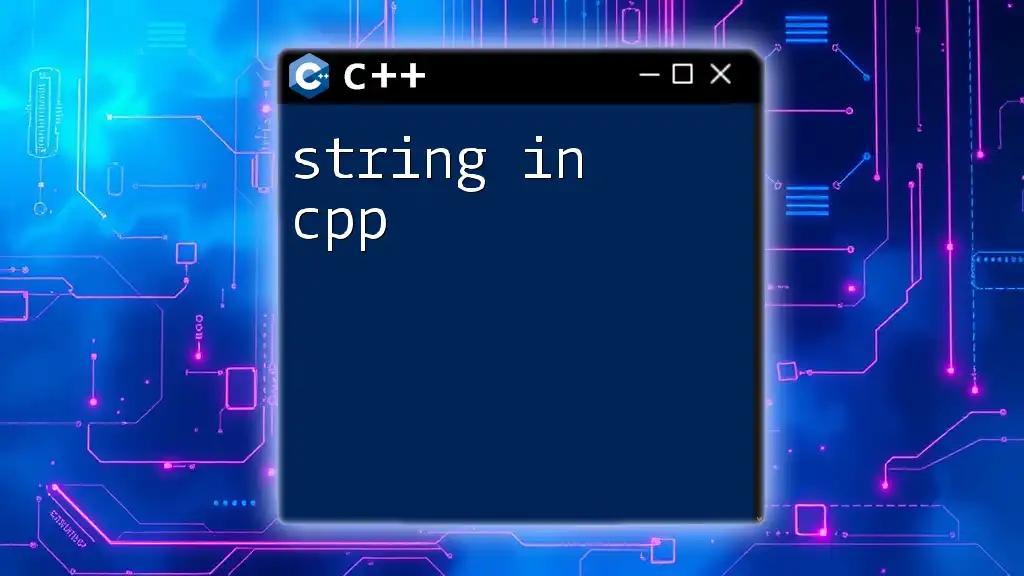
Further Learning Resources
To deepen your understanding of prime numbers and their applications in C++, consider exploring:
- Books on algorithm design
- Online C++ courses focusing on mathematical programming
- Websites and forums dedicated to programming challenges and solutions
The journey of mastering prime numbers in C++ continues beyond this article, paving the way for innovative problem-solving in the world of programming.