A prototype in C++ is a declaration of a function that specifies its name, return type, and parameters, allowing the function to be called before its actual definition.
int add(int a, int b); // Function prototype
int add(int a, int b) { // Function definition
return a + b;
}
Understanding C++ Prototypes
What is a Prototype?
A prototype in C++ is essentially a declaration of a function that provides the function's signature—its return type, name, and parameters—without specifying the body of the function. The primary goal of a prototype is to inform the compiler of the function's existence before it is called.
Importance of Function Prototypes
Using prototypes enhances code organization and readability, especially in larger projects. They help separate interface from implementation, allowing a clearer structure where function declarations can be grouped away from the main logic. This abstract layer of function signatures not only improves the clarity of the code but also aids in debugging and maintaining larger codebases.
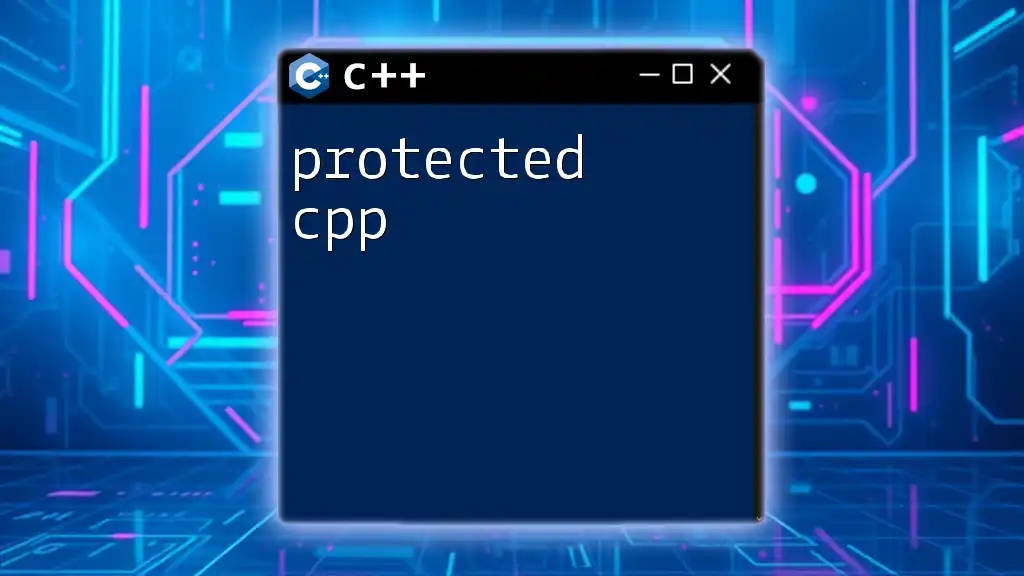
Syntax of Function Prototypes
Basic Structure
The syntax for declaring a function prototype consists of three primary elements: the return type, the function name, and the parameter list. The general form is:
return_type function_name(parameter_list);
For example:
int add(int a, int b);
Components of a Function Prototype
-
Return Type: This indicates the type of value that the function will return. It’s crucial for the compiler to verify that the correct type is returned.
-
Function Name: A meaningful name is vital as it provides context about what the function does, thus making the code easier to understand.
-
Parameter List: This defines what inputs the function expects. It is important to specify not only the type but also the number of parameters. You can even define default parameters here if needed.
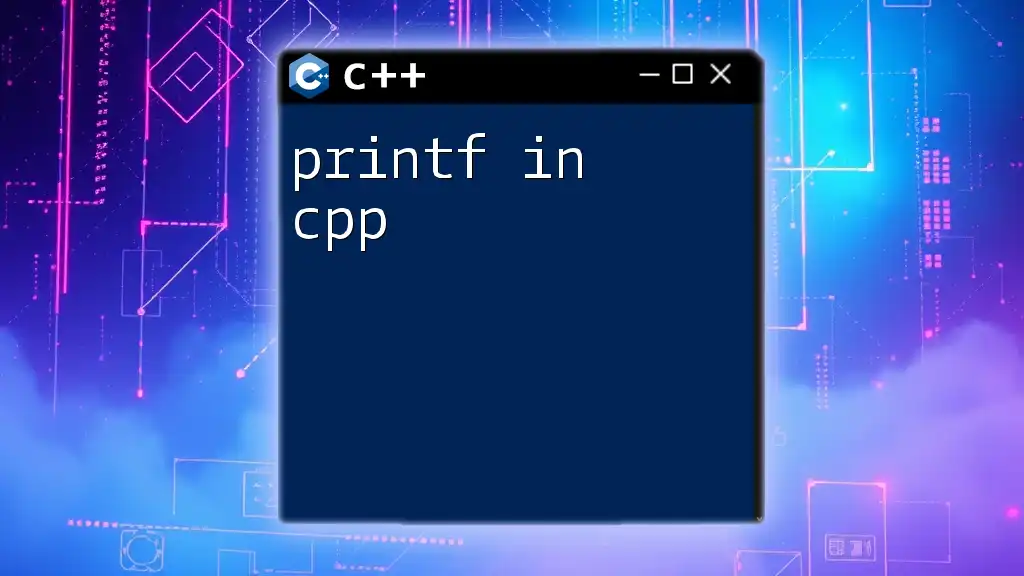
Function Prototypes vs. Function Definitions
Key Differences
Understanding the difference between function prototypes and definitions is essential. A prototype declares a function's interface but doesn’t implement it, while a definition includes the actual code that is executed when the function is called.
Examples of Each
Prototype:
void greet();
Definition:
void greet() {
std::cout << "Hello, World!";
}
By placing prototypes before their usage, you allow for a declaration to preemptively inform the compiler of the function’s structure.
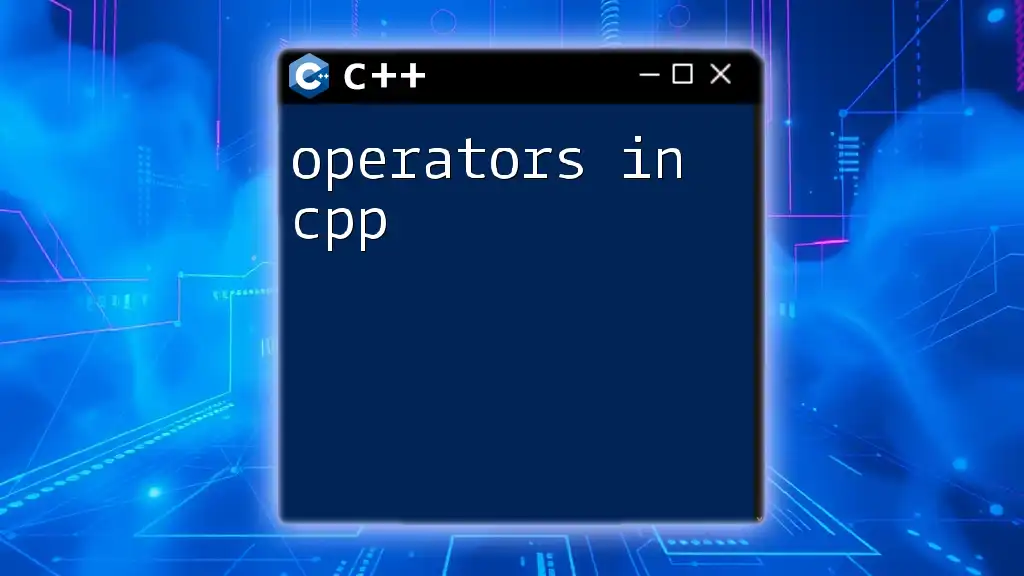
Where to Use Function Prototypes
In Header Files
Typically, prototypes are placed in header files which can be included in multiple implementation files. This practice is beneficial for code management, making it easier to track the function declarations.
For example:
// my_functions.h
int add(int a, int b);
In Implementation Files
While it is common to declare prototypes in header files, you can also use them directly in `.cpp` files, especially for smaller programs where separating files is unnecessary.
Example:
#include "my_functions.h"
int add(int a, int b) {
return a + b;
}
This flexibility allows for a more streamlined approach to using prototypes in various project structures.
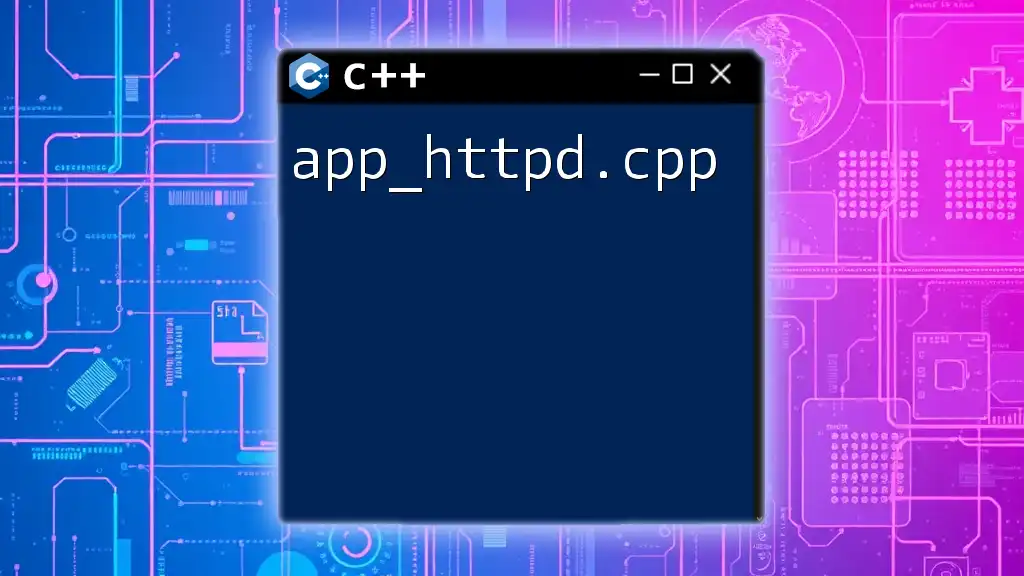
Best Practices for Using Prototypes
Keep Prototypes Simple
A well-structured and uncomplicated prototype aids comprehension. Avoid overly complex prototypes that can cause confusion. Clarity should always be a priority; a simple signature is better than one that complicates the understanding of what a function does.
Consistency in Naming
Using clear and consistent naming conventions for functions helps in maintaining readability throughout the code. Naming should be self-descriptive—e.g., a function that adds two integers can be aptly named `addIntegers()` instead of something vague like `doSomething()`.
Documentation
Adding brief comments next to complex prototypes can significantly help other developers (or even yourself in the future) understand the function's intended purpose.
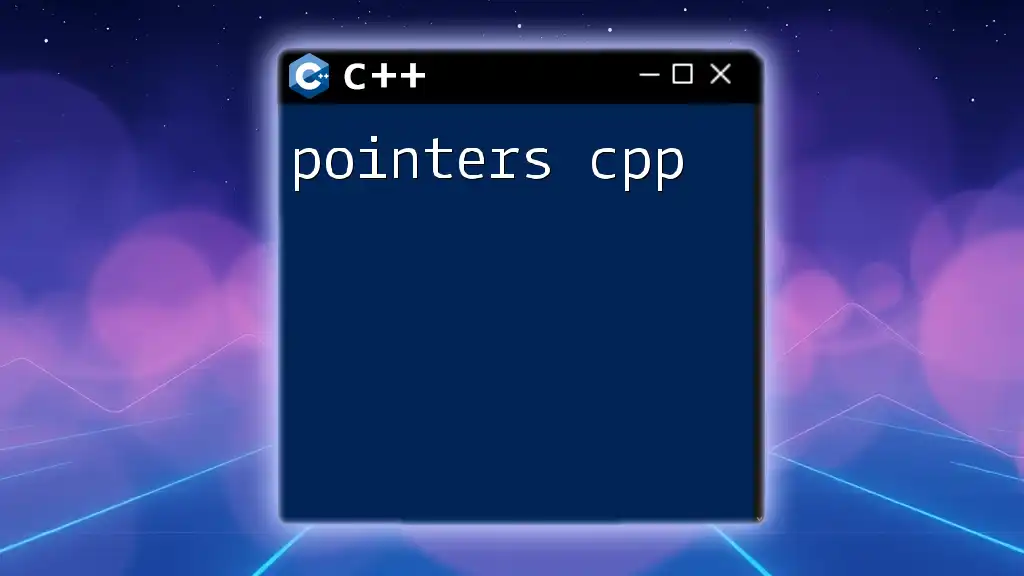
Common Errors Related to Prototypes
Missing Prototypes
One common mistake is failing to declare prototypes before calling them in your code. Not declaring a function before its usage can lead to compilation errors, as the compiler needs to know the function's signature before calling it.
Mismatched Types
Another frequent issue arises from parameter type mismatches. If you declare a prototype with one type and try to call it with another, you’ll receive a compilation error.
Example:
void process(int a);
process(5.0); // Error: mismatch
In this example, calling `process(5.0)` with a double when `process` is expecting an int will lead to a compilation failure. These errors can usually be resolved by ensuring type consistency across function declarations and calls.
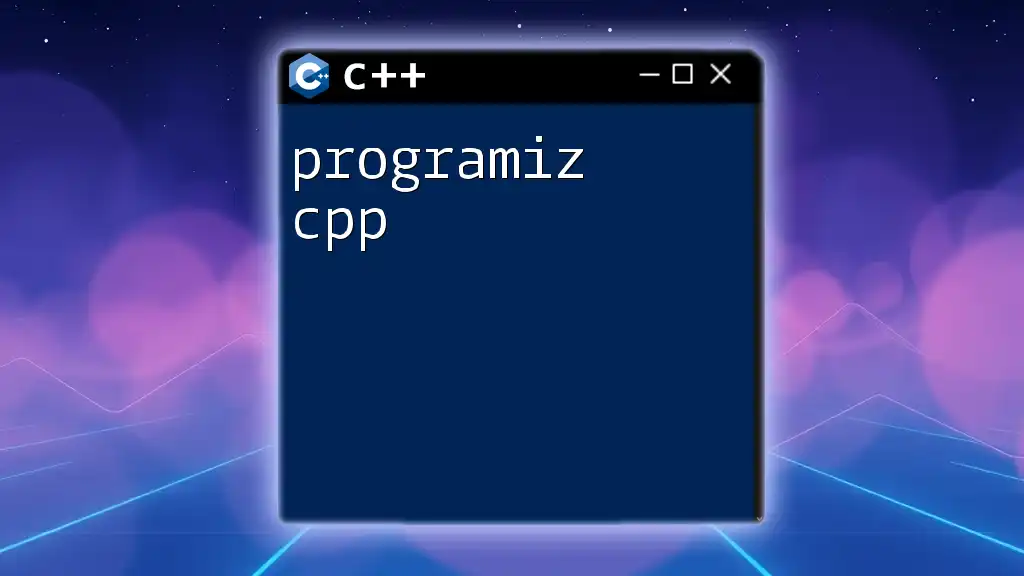
Advanced Topics Regarding Prototypes
Overloading Functions with Prototypes
C++ allows for function overloading, meaning you can have multiple functions with the same name as long as they have different parameter types or counts. When employing function prototypes for overloaded functions, it’s critical to declare each version separately.
For example:
int add(int a, int b);
double add(double a, double b);
This ability to overload provides great flexibility and is a fundamental feature of C++.
Templates as Prototypes
Templates are another powerful feature in C++ that work well with prototypes. You can define a function prototype using templates, allowing for a function definition that can operate on any data type.
Example:
template <typename T>
T add(T a, T b);
This flexibility makes templates extremely useful in creating generic functions that work with any data type, enhancing code reusability and efficiency.
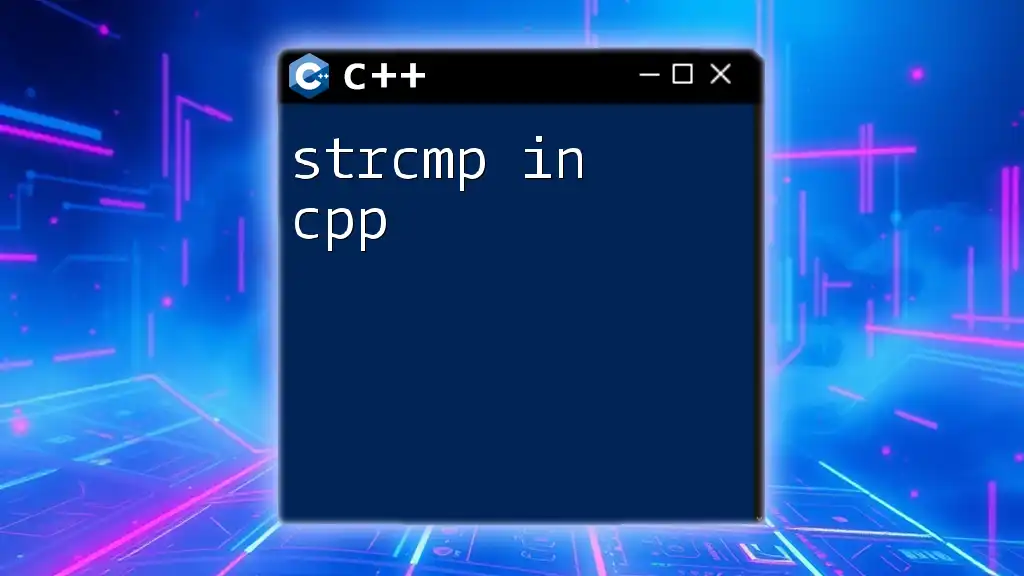
Conclusion
In this guide, we explored the various facets of using a prototype in C++, from basic definitions and syntax to advanced topics like overloading and templates. By understanding and applying the principles behind function prototypes, you can greatly improve the structure and maintainability of your C++ code. Adopting best practices such as simplicity, consistency in naming, and proper documentation will make your coding experience more effective and enjoyable.
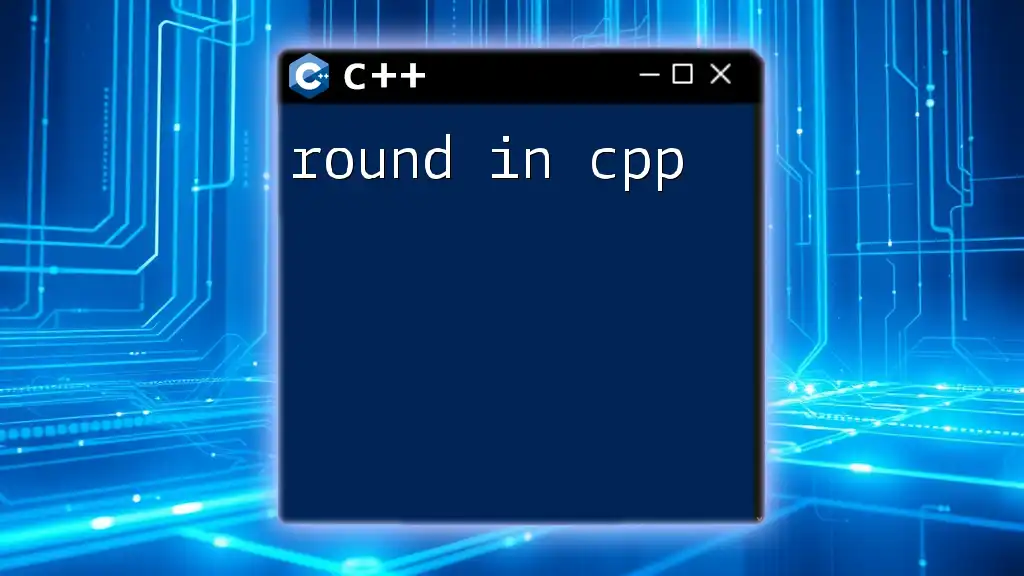
Additional Resources
To further your understanding of C++ prototypes, consider exploring reputable sources, books, and tutorials dedicated to mastering C++. Becoming familiar with these concepts will lay a strong foundation for your programming skills.