In C++, `return 0;` is used to indicate that the program has executed successfully and is exiting without any errors.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0; // Indicating successful termination
}
The Role of `return 0` in C++
In C++, the `return` statement is critical for every function’s exit process, but it holds particular significance in the `main` function. The command `return 0;` is used to indicate that the program executed successfully. When a program returns a value, it communicates the status of the program to the operating system.
Understanding Status Codes
Exit status codes are an essential aspect of any program written in C++. By convention, `0` signifies that the program completed successfully, while any non-zero value indicates an error or abnormal termination. The specific return codes can vary by application, but generally:
- `0`: Success.
- `1` or other positive numbers: An error occurred during execution.
- Negative values: Typically used in more complex applications to signify various errors.
When you see `return 0;`, it is a way of saying that everything went as anticipated, and the program did what it was supposed to do.

The Main Function: Entry Point of a C++ Program
The `main` function serves as the entry point for any C++ program. It is mandatory and can be defined in two ways:
- Standard signature: `int main()`
- Command-line arguments: `int main(int argc, char* argv[])`
Here's a simple example of a conventional main function:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0; // The program ends here with a return status of 0
}
In the code above, after printing "Hello, World!" to the console, `return 0;` is used to signal that the program has completed successfully.
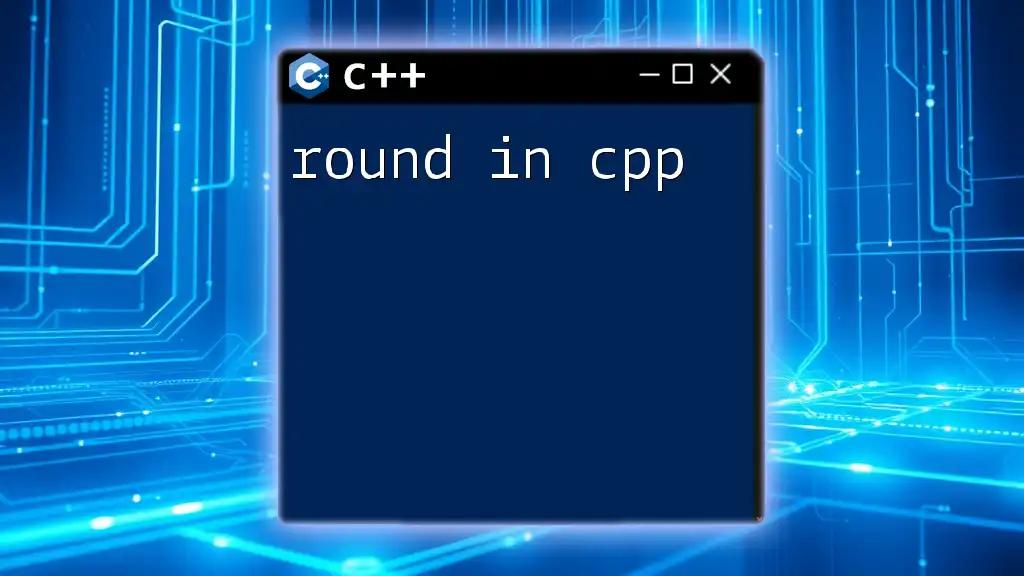
Best Practices for Using `return 0`
When employing `return 0;`, consider the following best practices:
-
Use it for indicating success: It’s widely accepted to use `return 0;` in your programs unless there is a specific reason to signify a different outcome.
-
In scenarios of error handling: You can assert more context-specific feedback with different return values. This makes it easier to debug your program.
-
Omitting `return 0;` in modern C++: With C++11 and later, the return from `main` can be omitted. If there is no return statement, the compiler automatically supplies `return 0;`. However, explicit returns enhance clarity.

Examples: Practical Use Cases of `return 0`
Simple Program Returning `0`
Here is a basic example that demonstrates `return 0;` in a minimalist program:
#include <iostream>
int main() {
return 0; // Successful execution
}
The code above does not perform any operations, but it clearly conveys a successful termination with `return 0;`.
Conditional Returns
A more intricate scenario involves conditionals, where you can return different values based on conditions:
#include <iostream>
int main(int argc, char* argv[]) {
if (argc > 1) {
return 0; // Return success if there are command-line arguments.
}
return 1; // Return failure if no arguments are present.
}
In this example, the return value dynamically reflects the program's state: it returns `0` if command-line arguments were provided, and `1` if they were not.
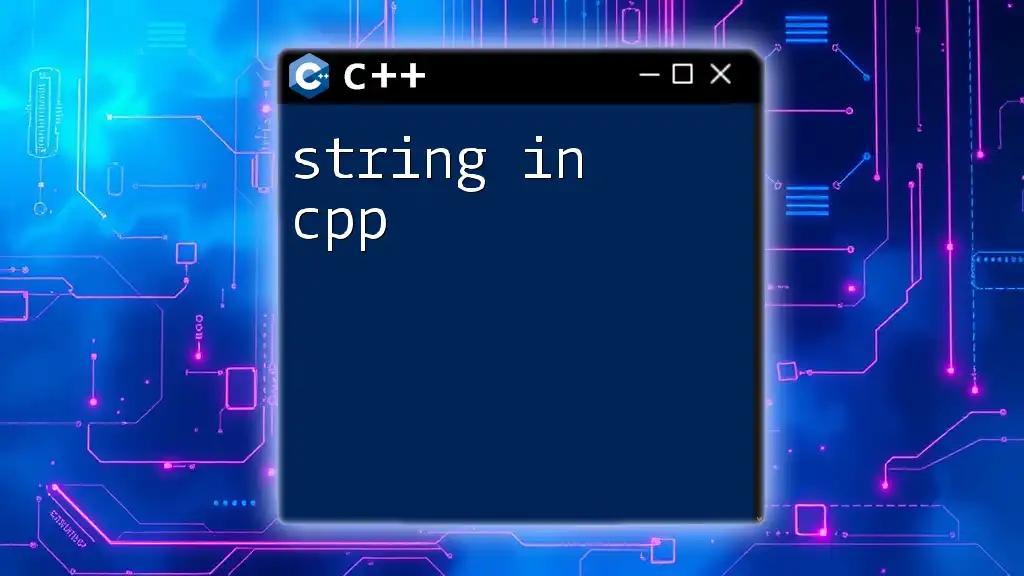
Common Misunderstandings About `return 0`
There are several myths regarding `return 0` that are often misconstrued, such as:
-
`return 0` is mandatory: As earlier mentioned, while it was essential in older C++ versions, modern C++ allows omission of the return statement in `main`.
-
`exit(0)` is identical to `return 0`: Although they achieve a similar outcome, `exit(0);` terminates the entire program, while `return 0;` merely exits the current function context. Using `exit(0);` from within other functions should be handled with caution as it may not execute destructors or cleanup code properly.
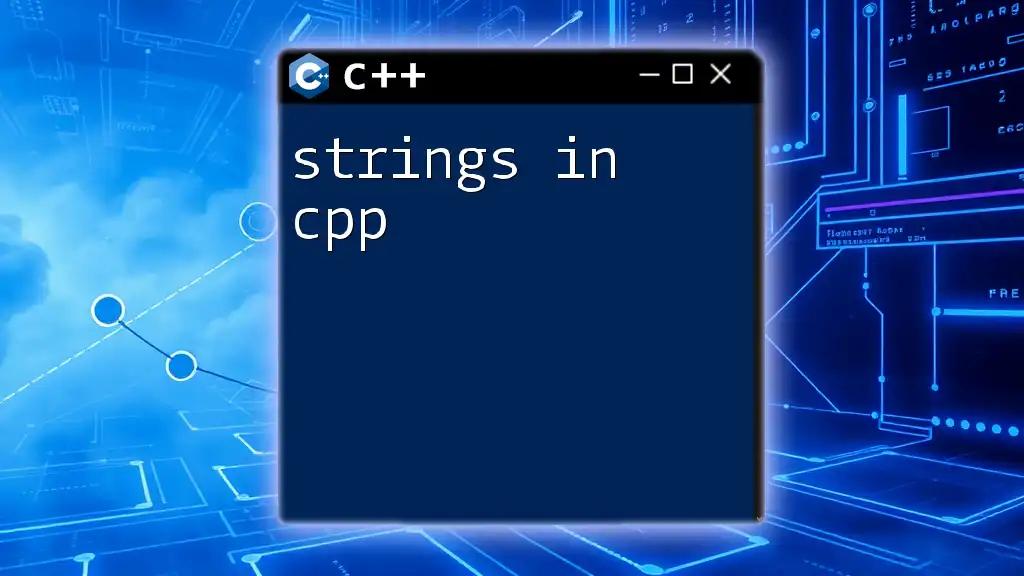
Conclusion
Incorporating `return 0` in C++ programming indicates a successful termination point of your application. Understanding when and how to use it appropriately can enhance your programming skills and prevent errors. As you deepen your knowledge of C++, keep an eye on best practices and remember the significance of status codes in your software development journey.