The `<cctype>` header in C++ provides functions for character classification and conversion, allowing you to easily determine the type of characters and manipulate them as needed.
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (std::isupper(ch)) {
std::cout << ch << " is uppercase." << std::endl;
}
std::cout << "Lowercase: " << static_cast<char>(std::tolower(ch)) << std::endl;
return 0;
}
What is `<ctype.h>`?
`<ctype.h>` is a header file in C++ that provides functions for classifying and transforming characters. These functions can distinguish between various types of characters, such as letters, digits, and whitespaces, making it an essential tool for handling character data efficiently.
Understanding how to leverage the functionality of `ctype.h` can greatly enhance your programming, especially when working with user input, parsing text data, or performing operations that require character manipulation.
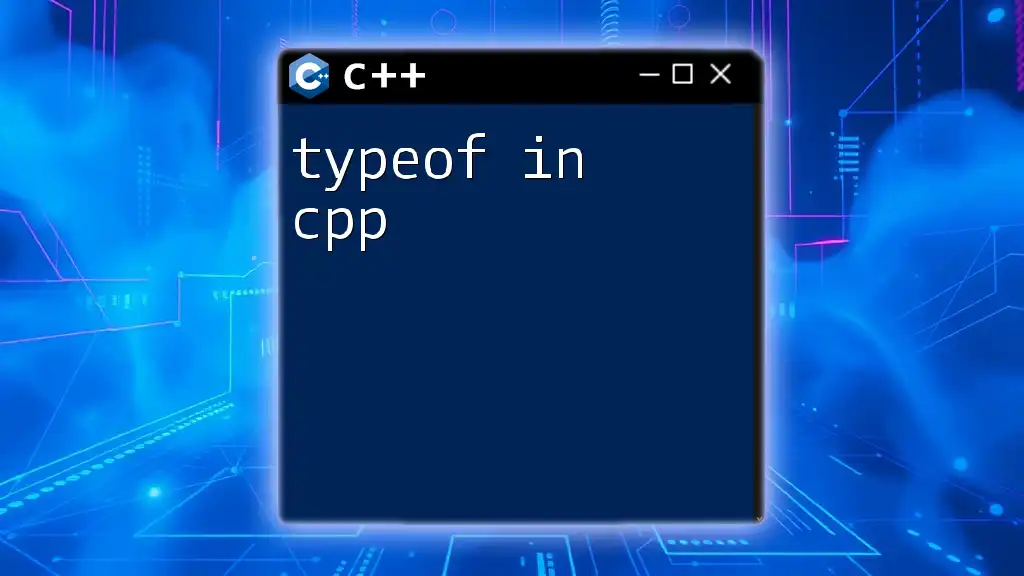
The Functions in `<ctype.h>`
Overview of Character Classification Functions
Character classification functions categorize characters into different classes. This classification can be instrumental when you need to validate user input or process text data efficiently. Utilizing these functions can help streamline many coding scenarios, thereby increasing code readability and reducing errors.
Common Character Classification Functions
Here's a closer look at some of the most commonly used character classification functions found in `<ctype.h>`:
- `isalpha(int c)`: This function checks if the character is an alphabet letter, returning a non-zero value (true) if it is, and zero (false) if it is not.
- `isdigit(int c)`: Similar to `isalpha()`, this function determines if the character is a digit (0-9).
- `islower(int c)`: This function checks if a character is a lowercase letter (a-z).
- `isupper(int c)`: Conversely, this checks if a character is an uppercase letter (A-Z).
- `isspace(int c)`: This function identifies whether a character is a whitespace character (spaces, tabs, newlines).
Code Example: Here’s how these functions can be put to practical use:
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (isalpha(ch)) {
std::cout << ch << " is an alphabet." << std::endl;
}
if (isdigit(ch)) {
std::cout << ch << " is a digit." << std::endl;
}
if (islower(ch)) {
std::cout << ch << " is a lowercase letter." << std::endl;
}
if (isupper(ch)) {
std::cout << ch << " is an uppercase letter." << std::endl;
}
if (isspace(ch)) {
std::cout << ch << " is a whitespace character." << std::endl;
} else {
std::cout << ch << " is not a whitespace character." << std::endl;
}
return 0;
}
Character Transformation Functions
In addition to classification, `<ctype.h>` provides functions that allow you to transform characters:
- `tolower(int c)`: Converts an uppercase letter to its lowercase equivalent.
- `toupper(int c)`: Converts a lowercase letter to its uppercase equivalent.
Transformation functions are particularly useful for implementing case-insensitive comparisons or normalizing input data.
Code Example: Here’s how these transformation functions can be applied:
#include <iostream>
#include <cctype>
int main() {
char lower = 'a';
char upper = 'A';
std::cout << "Lowercase to Uppercase: " << toupper(lower) << std::endl;
std::cout << "Uppercase to Lowercase: " << tolower(upper) << std::endl;
return 0;
}
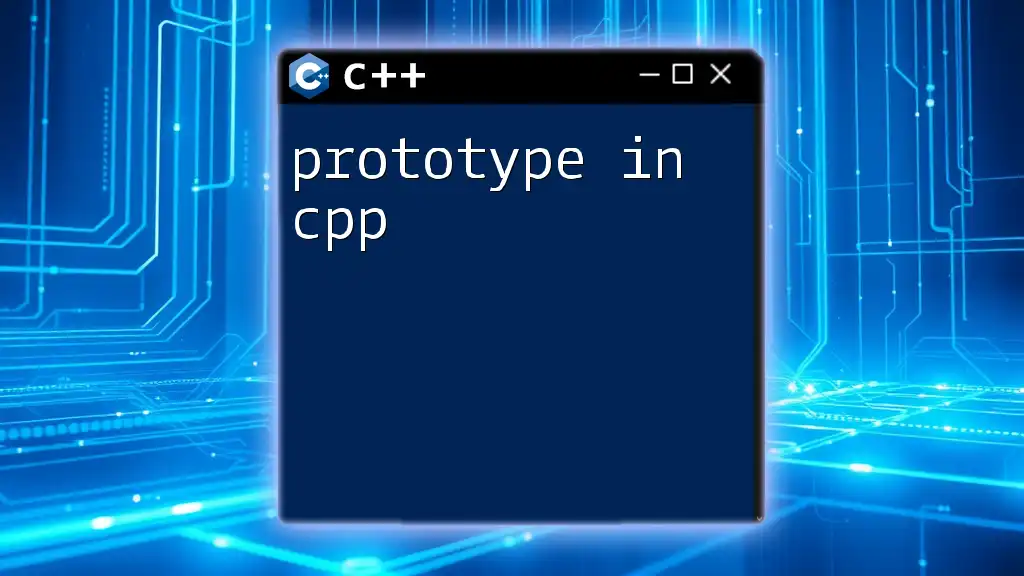
Practical Uses of `<ctype.h>` in Real-world Applications
Input Validation
With user input being a common source of errors, utilizing functions like `isdigit()` can ensure that numerical input is correctly validated. By checking each character in the input string, a programmer can ascertain whether it matches the expected format.
Example Code for Input Validation: Here’s a simple program that validates user input:
#include <iostream>
#include <cctype>
bool isValidInput(const std::string& input) {
for (char ch : input) {
if (!isdigit(ch)) {
return false; // Invalid if a non-digit is found
}
}
return true;
}
int main() {
std::string userInput;
std::cout << "Enter a number: ";
std::cin >> userInput;
if (isValidInput(userInput)) {
std::cout << "Valid input!" << std::endl;
} else {
std::cout << "Invalid input. Please enter digits only." << std::endl;
}
return 0;
}
Text Processing
In text processing scenarios, functions from `ctype.h` can assist in cleaning and preparing data. For instance, using `isspace()` to remove unnecessary spaces or `tolower()` to normalize cases before performing operations like searching or sorting.
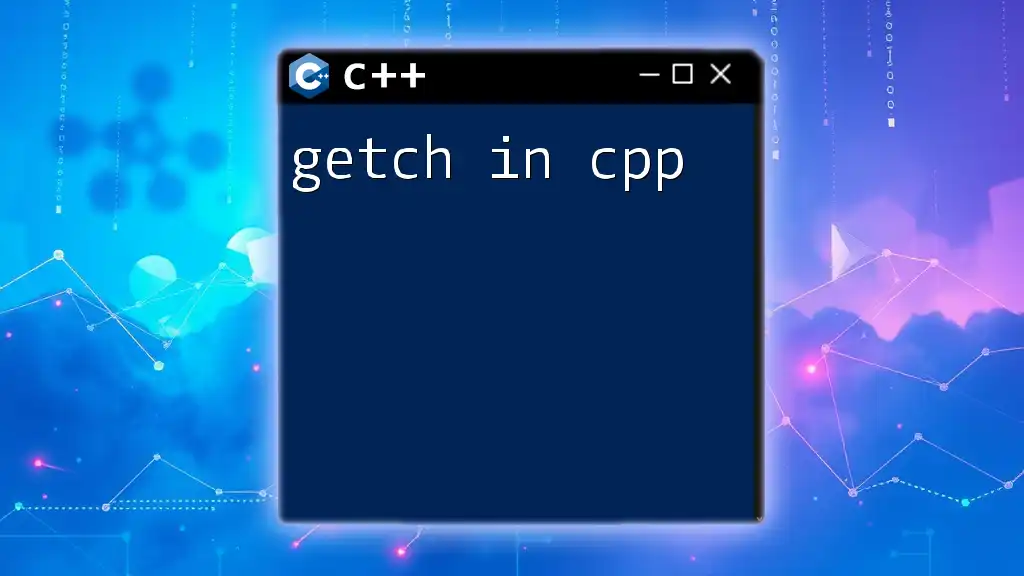
Best Practices When Using `<ctype.h>`
Function Usage Tips
While using the functions in `<ctype.h>`, it is important to consider:
- Character Bounds: Ensure the character being passed to these functions is within valid bounds (i.e., an `unsigned char` or EOF). This will prevent undefined behavior.
- Include the correct header: Remember to include `<cctype>` to avoid compatibility issues.
Performance Considerations
When processing large datasets, consider the implications of using character functions on performance. Always strive for efficiency in loops where these functions are used, and try to minimize unnecessary calls.
Avoiding Common Pitfalls
One common mistake when using `ctype.h` functions is the misconception that they will work properly with non-ASCII characters. When dealing with wide characters or different character encodings, it's advisable to implement checks to ensure you’re processing valid character types.
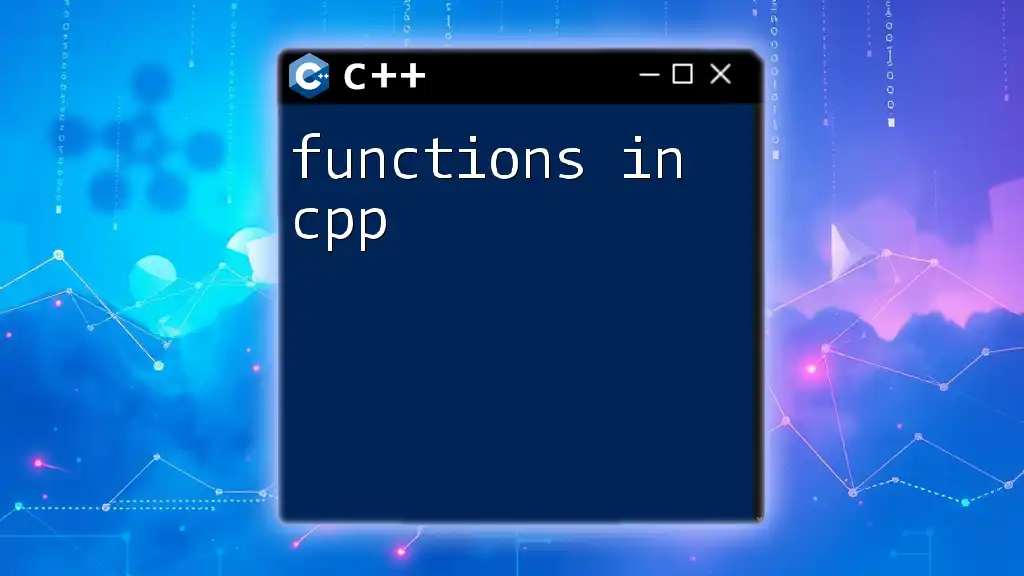
Conclusion
In summary, `<ctype.h>` is a foundational component in C++ that empowers programmers to classify and transform characters efficiently. Understanding how to harness its functionality can be instrumental in character handling, input validation, and text processing. By following best practices and being aware of potential pitfalls, you can utilize the capabilities of `ctype.h` to ensure robust, efficient, and error-free code.
As you delve deeper into C++, explore further resources and documentation to discover advanced techniques and applications of `ctype.h`. Experimenting with the provided examples in your IDE will solidify your understanding and enhance your ability to write effective character manipulation code.