When you search for "Google C++," you often aim to find resources, libraries, or examples relevant to writing efficient C++ code in a Google context, such as using Google Test for unit testing.
Here's a simple code snippet demonstrating a basic C++ command that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Why Choose Google C++?
When we discuss the keyword "google c++," we're talking about a toolkit that's recognized for its effectiveness in both performance and productivity. The combination of Google's ecosystem and C++, a language designed for speed and efficiency, empowers developers to build scalable and robust applications.
Advantages of Using Google C++
Choosing to harness Google C++ comes with notable advantages:
-
Performance and Efficiency: C++ is known for its high performance. With Google's optimizations and libraries, programmers can achieve better execution speeds, making it ideal for applications that require substantial processing power.
-
Large-scale Application Development: Google has utilized C++ in building massive systems, allowing for complex functionalities while maintaining clarity and speed.
-
Community and Support: As a widely adopted language, combined with Google's influence, you’ll find a vast community providing resources, tools, and support to Java developers.
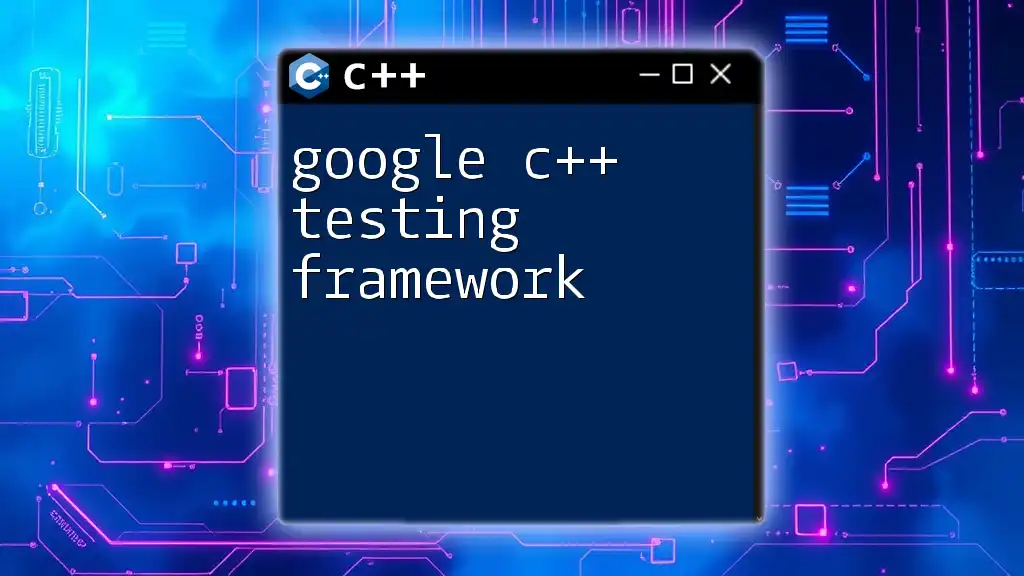
The Foundations of Google C++
Key Concepts in C++
Understanding the foundational concepts of C++ is crucial for anyone venturing into Google C++. This includes grasping the following:
-
Basic Syntax and Structure: Familiarity with standard C++ syntax, including data types, operators, and control statements, is essential.
-
Object-Oriented Programming (OOP): C++ supports OOP principles such as encapsulation, inheritance, and polymorphism. Understanding these will allow you to design flexible and maintainable code.
Google's Contributions to C++
Google has made significant contributions to the C++ landscape:
-
Open-source C++ Libraries: Google has released numerous libraries that enhance C++ capabilities, such as Abseil, which offers reusable C++ components.
-
Google's C++ Style Guide: This guide is invaluable for ensuring code consistency and quality across teams. Adhering to these standards promotes best practices within collaborative environments.
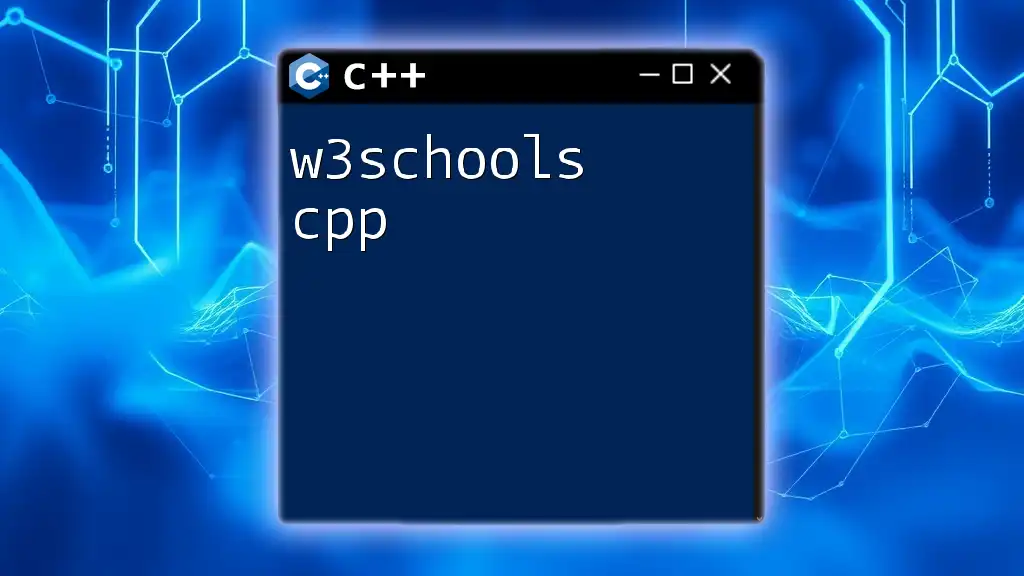
Getting Started with Google C++
Setting Up Your Environment
To dive into Google C++, you'll need to set up your development environment:
-
Tools and IDEs: Popular Integrated Development Environments (IDEs) such as Android Studio and Visual Studio can streamline your C++ development experience. Choose one based on your project requirements.
-
Installing Necessary Libraries and Dependencies: Ensure you have the Google libraries installed for specific functionalities. Libraries like gRPC or TensorFlow can be incorporated depending on your application needs.
Compiling Your First C++ Program
With your environment set up, it's time to write your first C++ program. Here’s a simple example of a “Hello, World!” program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code, the `#include <iostream>` directive lets your program use the input-output stream, while `main()` is the entry point of the program. The `std::cout` command outputs text to the console, demonstrating fundamental C++ operations.
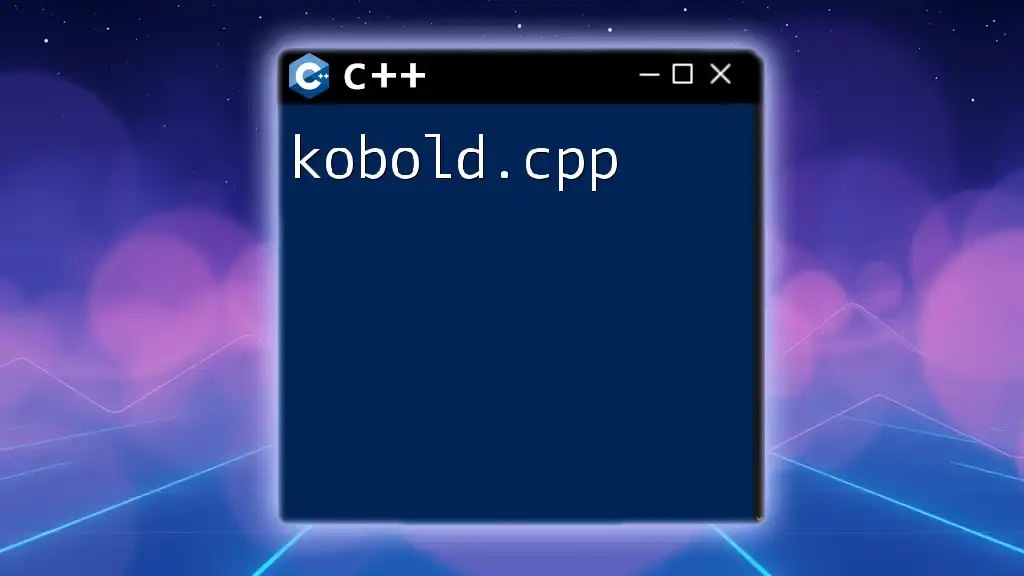
Core C++ Features Emphasized by Google
Memory Management
One of C++’s strengths is its memory management capabilities, which you should fully utilize when coding in a Google C++ environment.
-
Understanding Dynamic Memory: By using dynamic memory allocation, you can create variables at runtime. However, this requires careful management to prevent memory leaks.
-
Usage of Smart Pointers: Smart pointers like `unique_ptr` and `shared_ptr` are critical for managing memory effectively. Here’s an example using `unique_ptr`:
#include <iostream>
#include <memory>
void useSmartPointer() {
std::unique_ptr<int> p(new int(10));
std::cout << *p << std::endl;
}
In the above code, `unique_ptr` automatically manages memory, ensuring that the allocated memory is freed when the pointer goes out of scope.
Concurrency
With Google C++, managing concurrent processes becomes straightforward. Multithreading allows your application to perform multiple tasks simultaneously.
-
Basics of Multithreading: C++ provides built-in support for multithreading, allowing multiple threads of execution within a program.
-
Using `std::thread`: Here’s how you can create a simple thread:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Thread Function Executed" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Wait for the thread to finish
return 0;
}
This example creates a new thread that runs `threadFunction`, demonstrating a basic use of multithreading.
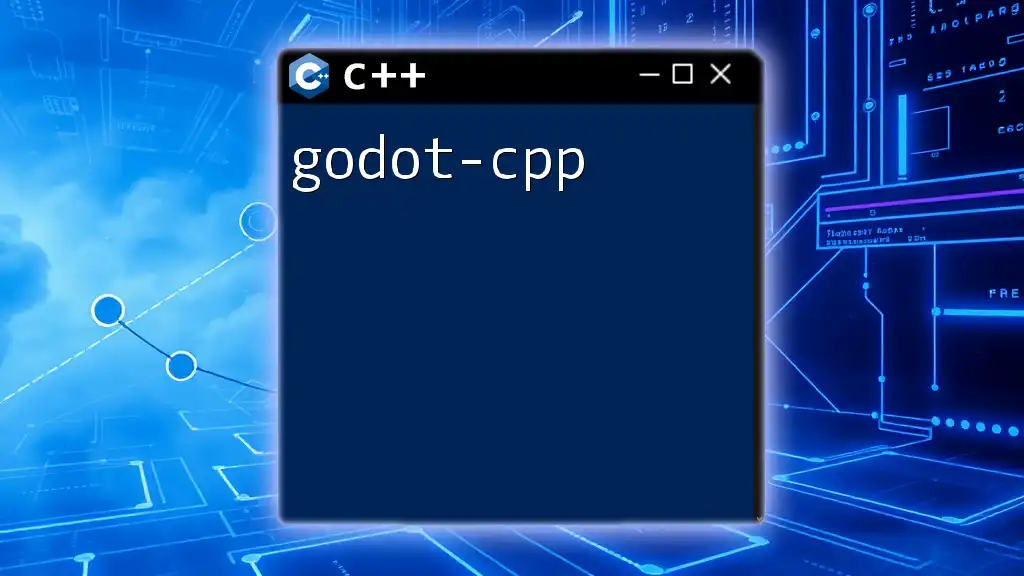
Google's C++ Libraries
Overview of Key Libraries
Google enhances C++ development through a suite of libraries.
-
Google Test Framework: A robust framework that simplifies unit testing in C++. This framework allows you to create tests easily and track your code's correctness.
-
Protocol Buffers: A language-neutral, platform-neutral extensible mechanism for serializing structured data, making it widely applicable for data exchange between services.
Using Google Test for Effective Testing
Here’s how to leverage Google Test to write a simple test case:
#include <gtest/gtest.h>
TEST(SampleTest, AssertionTrue) {
EXPECT_TRUE(true);
}
In this code, we define a test case that checks if the condition is true. This basic example illustrates how to get started with Google’s powerful testing framework, ensuring that your code remains reliable.
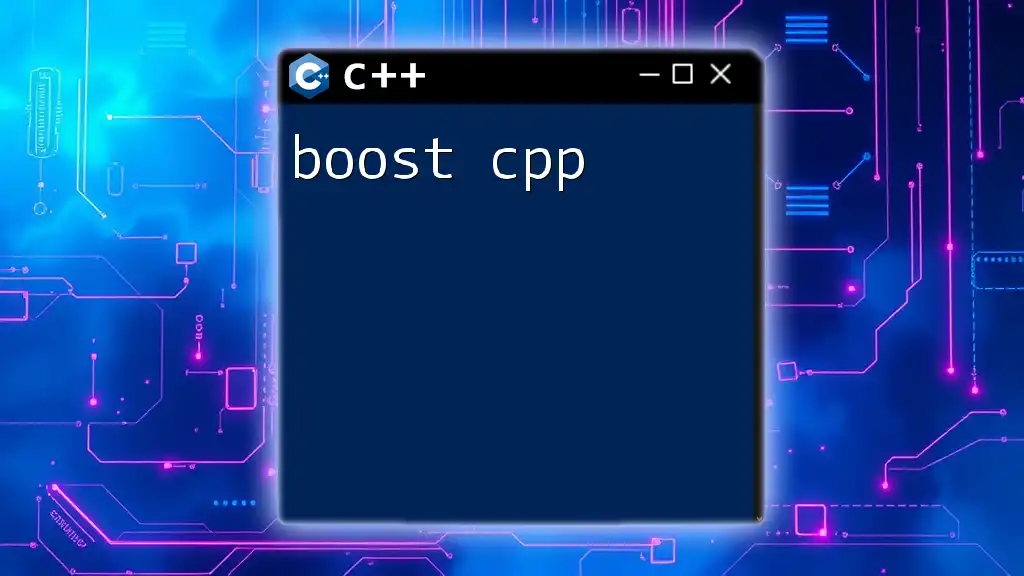
Best Practices in Google C++
Following Google’s C++ Style Guide
Google's C++ Style Guide is a treasure trove of best practices that should not be overlooked. Key guidelines include:
- Code Style: Follow consistent naming conventions (e.g., camelCase for variables, PascalCase for classes).
- Formatting: Maintain clean and readable code through appropriate indentations and spacing.
Common Pitfalls to Avoid
As you embark on your C++ journey, be aware of these common mistakes:
- Memory Leaks: Always ensure proper memory management. Utilize smart pointers to mitigate this risk.
- Undefined Behavior: Be cautious of pointer usage and boundary conditions in arrays to prevent crashes and data corruption.
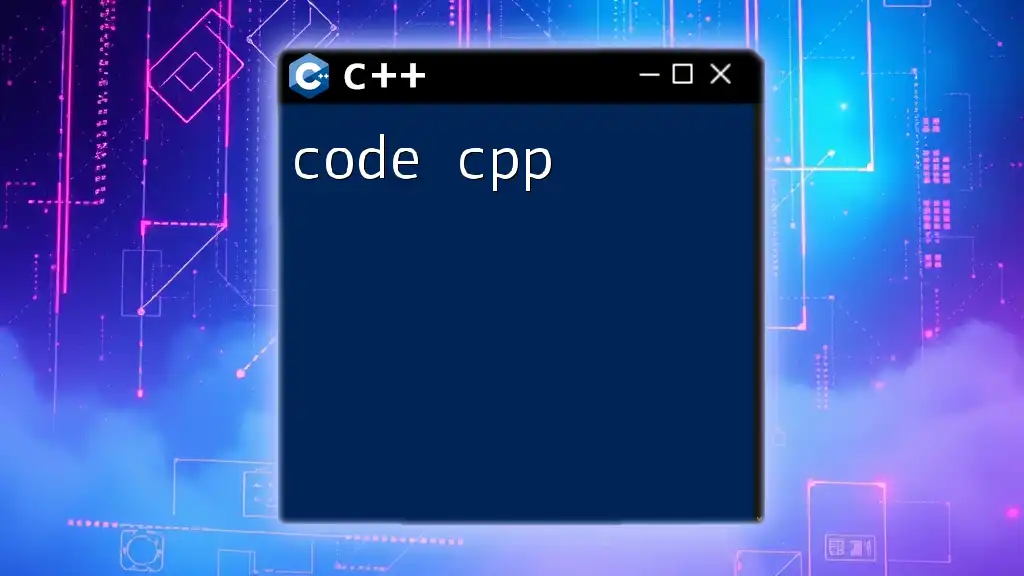
Real-world Applications of Google C++
C++ in Google's Products
C++ is at the core of many Google products. From the backend of Google Search to performance-efficient mobile applications, its applications are vast.
The Future of C++ at Google
As technology evolves, C++ continues to adapt. Keep an eye on the latest trends such as integration with machine learning libraries, IoT applications, and real-time systems expected to grow in the Google ecosystem.
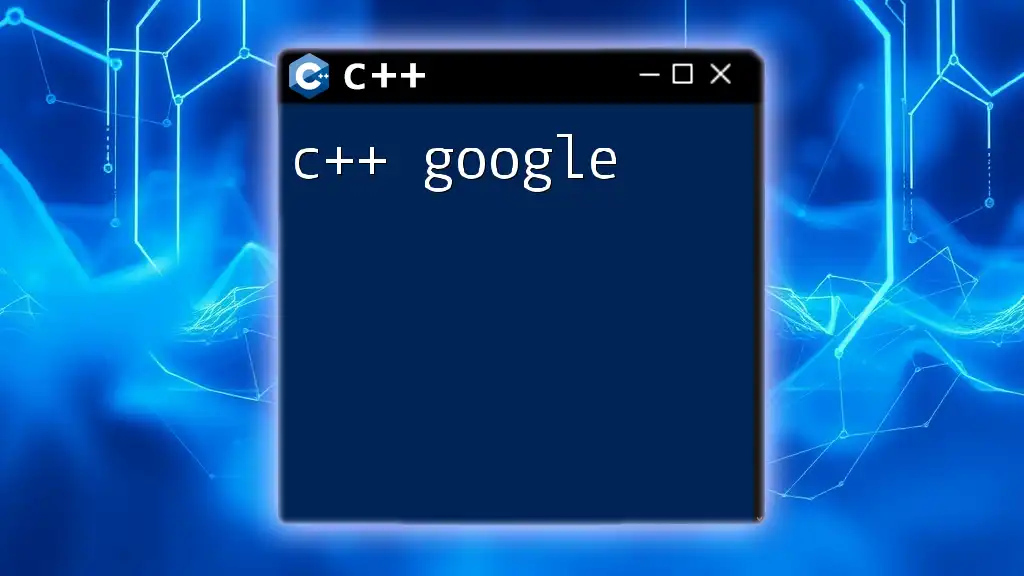
Conclusion
Diving into Google C++ opens a realm of possibilities for developing high-performance applications. Whether you’re interested in building robust systems or exploring cutting-edge technologies, mastering Google C++ will undoubtedly enhance your programming capabilities and broaden your career opportunities.
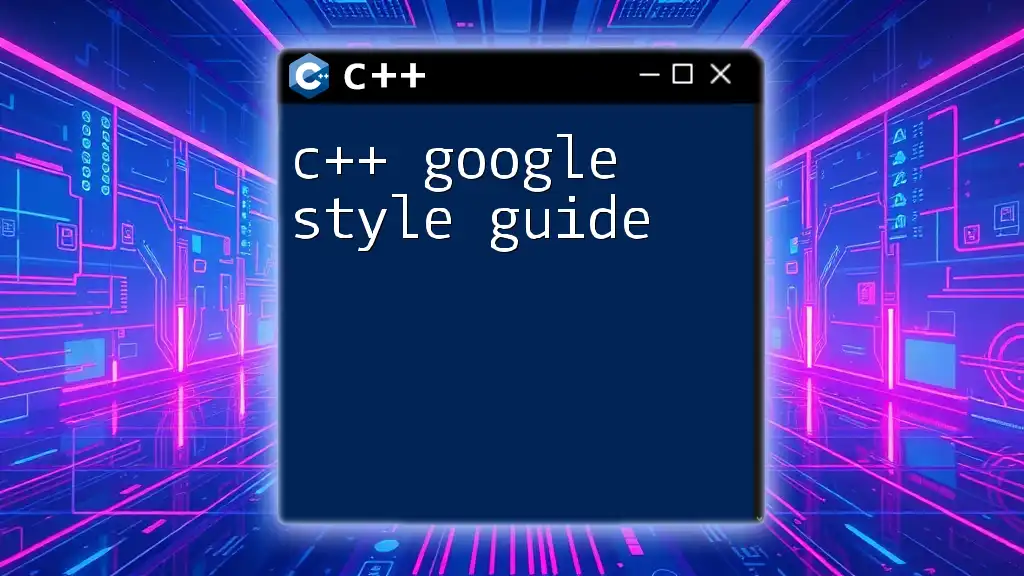
Additional Resources
For further learning, consider exploring the following:
- Comprehensive C++ tutorials.
- Books specifically geared towards C++ development.
- Community forums like Stack Overflow or GitHub to connect with other developers.
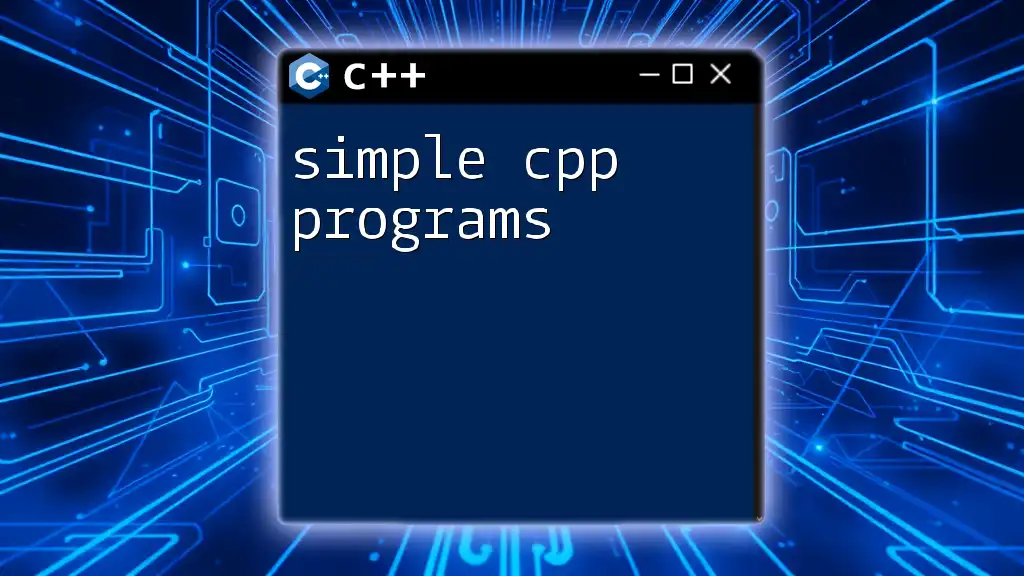
Call to Action
If you found this guide helpful, consider subscribing for more insights. Join our upcoming training sessions to deepen your understanding of Google C++ and elevate your programming skills!