A queue in C++ is a container adapter that follows the First-In-First-Out (FIFO) principle, allowing you to efficiently add elements to the back and remove them from the front.
#include <iostream>
#include <queue>
int main() {
std::queue<int> q; // Create a queue of integers
q.push(10); // Add 10 to the queue
q.push(20); // Add 20 to the queue
std::cout << q.front() << std::endl; // Access the front element (10)
q.pop(); // Remove the front element
std::cout << q.front() << std::endl; // Access the next front element (20)
return 0;
}
Understanding C++ Queue
A queue in C++ is a data structure that follows the FIFO (First In, First Out) principle. This means that the first element added to the queue will be the first one to be removed. Queues are essential for various programming tasks, such as managing tasks that need to be processed in the order they arrive, making queues an invaluable asset in software development.
Compared to other data structures, like stacks, which operate on a LIFO (Last In, First Out) basis, queues allow operations that are more aligned with real-world scenarios such as line management in customer service or task scheduling in operating systems.
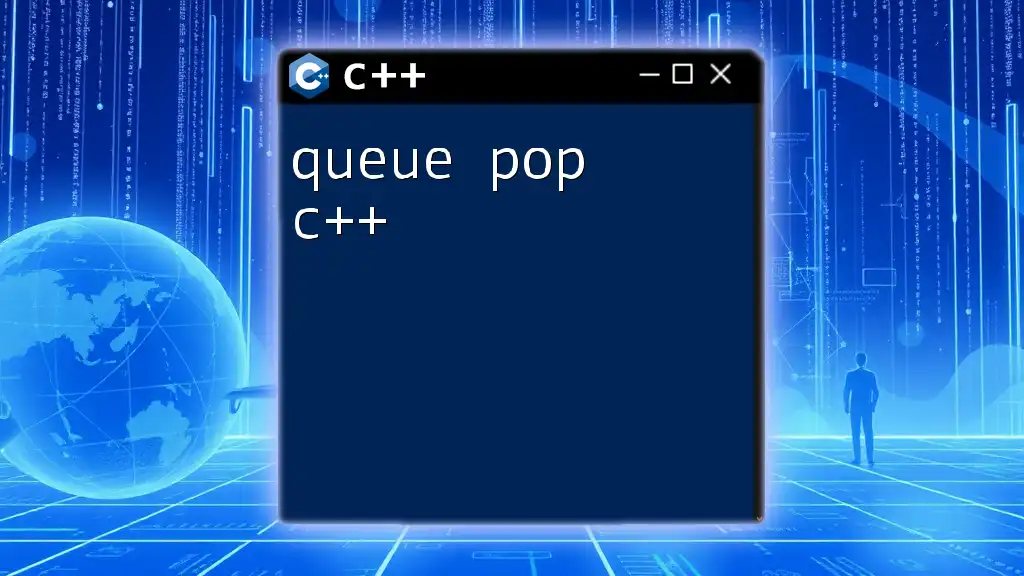
C++ Queue Implementations
The Standard Template Library (STL) Queue
The Standard Template Library (STL) in C++ provides built-in support for queues, making it easier for developers to implement and manage them. To begin using queues from the STL, you need to include the necessary header:
#include <queue>
Defining a Queue in C++
To define a queue in C++, you can use the `std::queue` class template. It can store elements of any data type. Here’s how you can declare and define a queue that holds integers:
std::queue<int> myQueue;
Basic Operations on C++ Queues
Queues support several fundamental operations, which allow you to manage elements effectively.
Enqueue Operation
The enqueue operation refers to adding elements to the back of the queue. This can be accomplished using the `push()` method:
myQueue.push(10); // Adds 10 to the queue
Dequeue Operation
The dequeue operation removes the front element from the queue. You can achieve this by using the `pop()` method:
myQueue.pop(); // Removes the front element
It is important to note that `pop()` does not return the value of the removed element; it merely removes it. To access the value, use the `front()` method before popping.
Front and Back Operations
Accessing the elements at the front and back of the queue can be done using the `front()` and `back()` methods, respectively:
int frontValue = myQueue.front(); // Get front element without removing it
int backValue = myQueue.back(); // Get back element without removing it
Checking Queue Properties
Understanding the state of a queue is crucial in your applications. The STL provides methods to check if a queue is empty and to get its size:
bool isEmpty = myQueue.empty(); // Check if the queue is empty
int size = myQueue.size(); // Get the current size of the queue
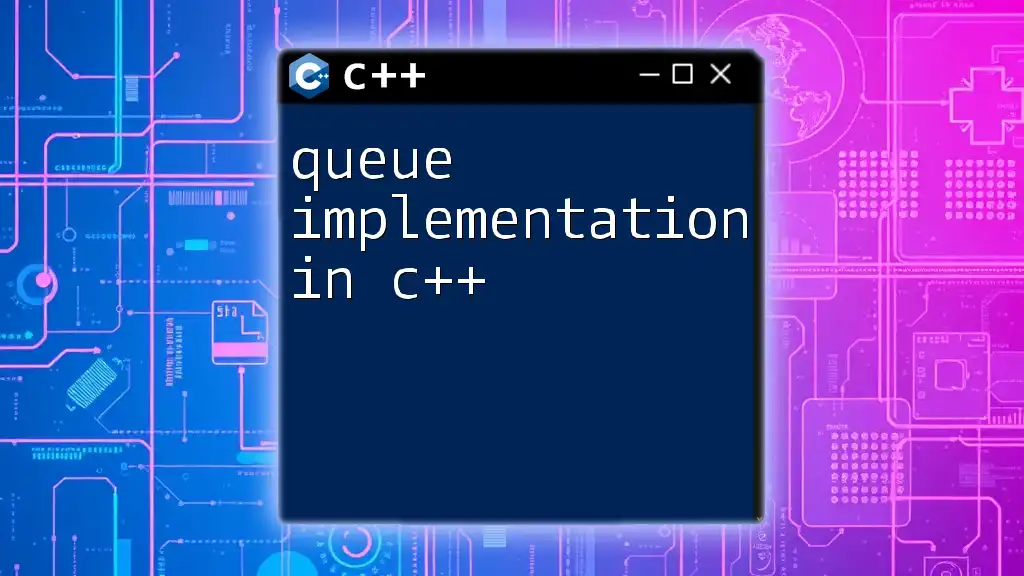
Working with C++ Queues: Practical Examples
Example 1: Simple Queue Operations
Here is a basic example demonstrating some of the operations you can perform on a queue in C++:
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
myQueue.push(1);
myQueue.push(2);
myQueue.push(3);
std::cout << "Front: " << myQueue.front() << std::endl; // Output: 1
myQueue.pop();
std::cout << "Front after pop: " << myQueue.front() << std::endl; // Output: 2
return 0;
}
In this example, we push three integers onto the queue and display the front element. After popping the first element, we display the new front element, illustrating the FIFO behavior.
Example 2: Queue in C++ for Customer Service Simulation
Queues can be particularly useful in simulating real-world scenarios. For example, let’s create a simple program that simulates a customer service management system:
#include <iostream>
#include <queue>
#include <string>
struct Customer {
int id;
std::string name;
};
int main() {
std::queue<Customer> customerQueue;
// Adding customers to the queue
customerQueue.push({1, "Alice"});
customerQueue.push({2, "Bob"});
while(!customerQueue.empty()) {
Customer frontCustomer = customerQueue.front();
std::cout << "Serving customer: " << frontCustomer.name << std::endl; // Serve customer
customerQueue.pop(); // Remove served customer
}
return 0;
}
In this simulation, we define a `Customer` structure and use a queue to manage customer service operations. Each customer is added to the queue, and we serve them in the order they were added until the queue is empty.
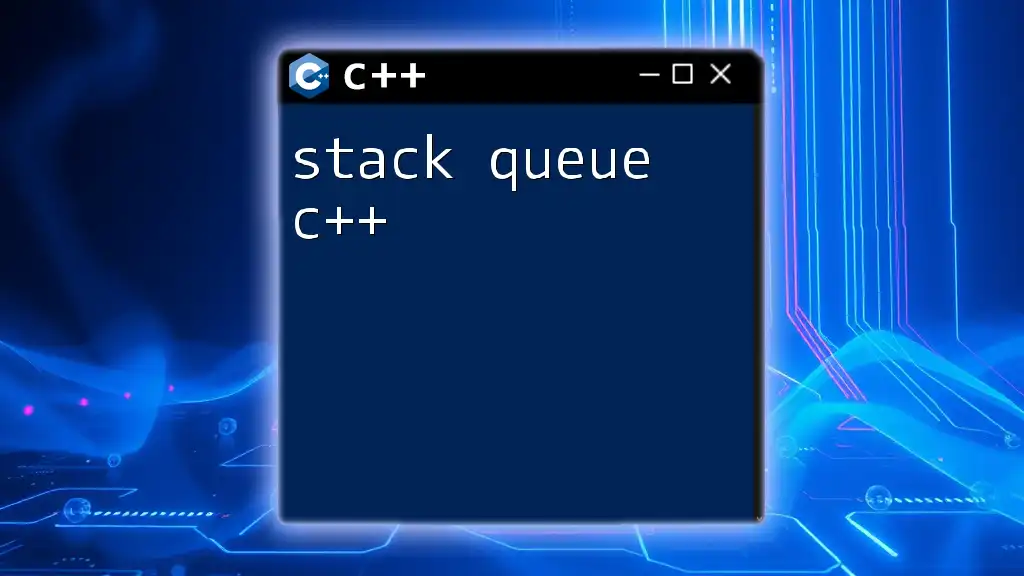
Advanced Queue Features in C++
Using Custom Data Types in C++ Queues
One of the strengths of C++ queues is their ability to handle custom data types. By defining a struct or class, you can create queues that store complex types, which enhances their utility in various applications.
Priority Queue in C++
A priority queue is a more specialized version of a queue where each element has a priority level. Elements with higher priority are processed before those with lower priority, regardless of their order in the queue. STL provides a built-in priority queue, accessible by including the header:
#include <queue>
To declare a priority queue, use:
std::priority_queue<int> pQueue;
You can customize how elements are prioritized by providing a comparison function or using custom data structures as elements.
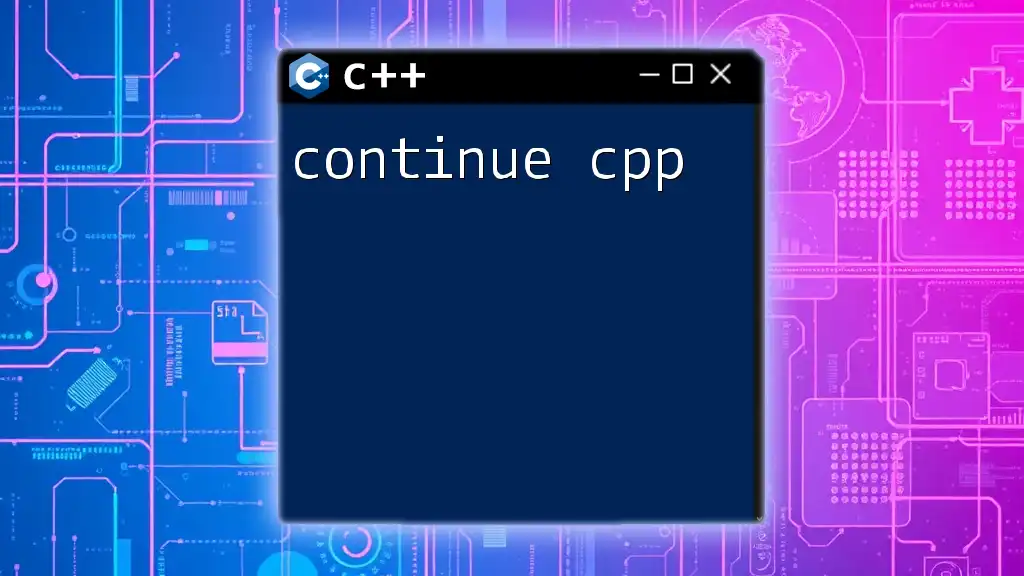
Performance Considerations
Time Complexity of Queue Operations
Understanding the time complexity of various queue operations is essential for optimizing performance. The primary operations include:
- Enqueue (`push`): O(1) – Adding an element to the back of the queue.
- Dequeue (`pop`): O(1) – Removing the front element.
- Access (`front`/`back`): O(1) – Getting the front or back element.
- Size and Empty Checks: O(1) – Checking if the queue is empty or obtaining its size.
Space Complexity
The space complexity of a queue primarily depends on the number of elements it contains. While a queue may not have any additional space overhead besides its elements, factors such as internal memory overhead of the data structures used can impact memory usage.
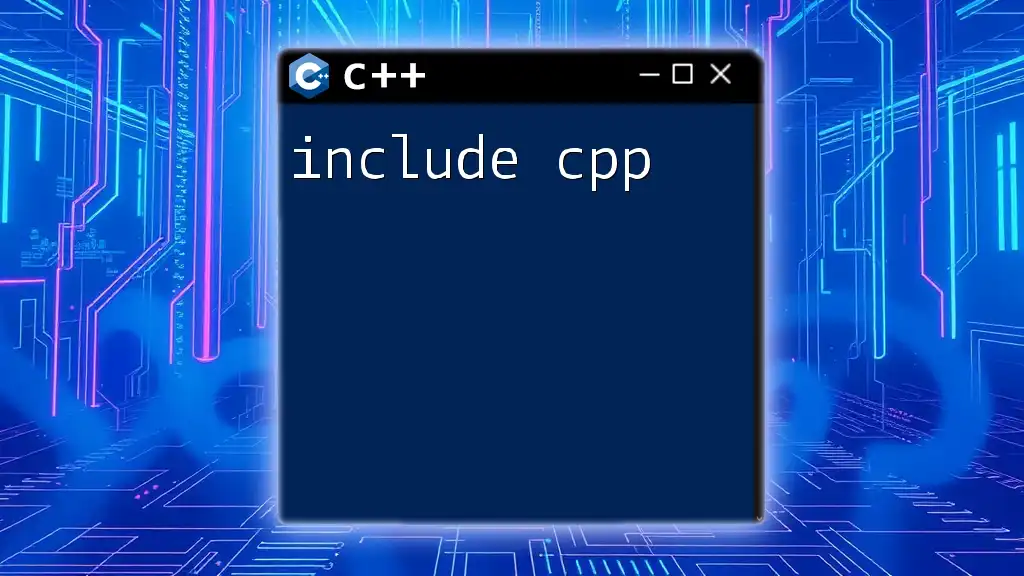
Common Use Cases for Queues in C++
Queues are used in many real-world applications. Here are a few scenarios where queues become vital elements of design:
- Job Scheduling: In operating systems, queues manage scheduling tasks, ensuring that jobs are processed in the order they arrive.
- Customer Service: Queues help manage service requests in help centers, ensuring that customers are served following their arrival times.
- Event-Driven Programming: In games or user interface programming, queues can manage events, ensuring that input handling occurs in the correct order.
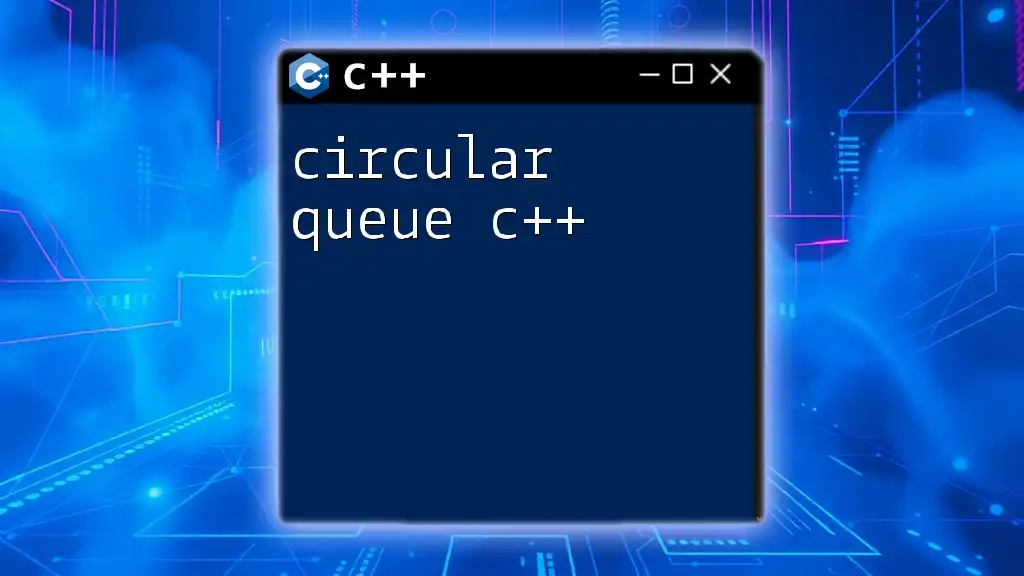
Conclusion
Queues are a fundamental data structure in C++ that can streamline process management and task scheduling. Understanding how to implement and utilize queues effectively is essential for any developer. By mastering queues, you can enhance your programming skills and create more efficient, organized software applications.
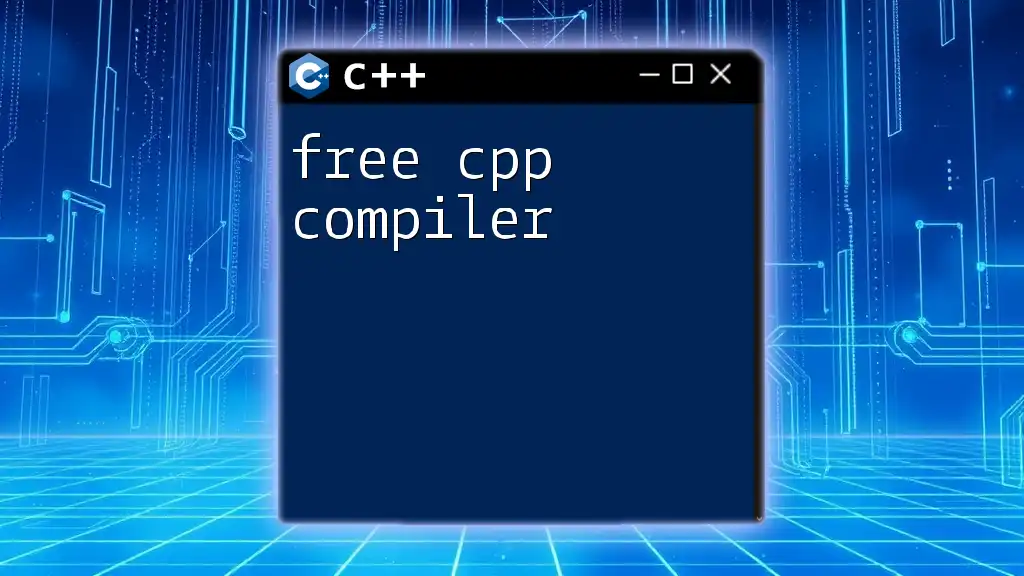
Additional Resources
To further your understanding of queues in C++, explore additional resources such as tutorials, documentation, and community forums where developers share their knowledge and support one another in mastering this essential data structure.