A segmentation fault in C++ occurs when a program attempts to access a memory location that it's not permitted to, often due to dereferencing a null or invalid pointer.
Here's a code snippet that demonstrates how a segmentation fault can occur:
#include <iostream>
int main() {
int* ptr = nullptr; // Pointer initialized to null
std::cout << *ptr; // Dereferencing a null pointer causes a segmentation fault
return 0;
}
What is a Segmentation Fault?
Definition
A segmentation fault in C++ is an error that occurs when a program attempts to access a memory region that it's not allowed to. This unintentional memory access typically arises from improper handling of pointers and can lead to program crashes. Understanding segmentation faults is crucial as they are among the most common bugs faced by developers, often resulting from memory access violations.
Why Does it Occur?
Segmentation faults can occur for several reasons, particularly when the program tries to:
- Access out-of-bounds memory: This happens when an array is accessed beyond its defined memory allocation.
- De-reference NULL or uninitialized pointers: If you attempt to access memory through a pointer that hasn’t been assigned a valid memory address, a segmentation fault results.
- Trigger a stack overflow: This condition occurs, for example, in recursive function calls without a proper exit condition.

Common Causes of Segmentation Faults
Accessing Invalid Memory Locations
A program may inadvertently try to access memory locations that have not been allocated to it. Consider this example:
int main() {
int arr[5] = {1, 2, 3, 4, 5};
// Attempting to access the sixth element
int value = arr[5]; // This will cause a segmentation fault
return 0;
}
In this code snippet, accessing `arr[5]` is out-of-bounds since array indices in C++ start at 0 and go up to 4. The attempt to retrieve a value from this invalid memory space can lead to a segmentation fault.
Memory Leaks
Memory leaks occur when a program allocates memory but fails to release it, resulting in wasted resources. Over time, these leaks can accumulate and exhaust available memory, leading to a segmentation fault.
#include <iostream>
int main() {
int* ptr = new int[10]; // Allocates memory for an array of integers
// Forgetting to deallocate memory
// delete[] ptr; // Uncomment this line to avoid a memory leak
return 0; // Memory is not freed, causing a memory leak
}
In the above code, if we allocate memory without freeing it, this may not immediately crash the program but can lead to a segmentation fault later in execution due to running out of memory.
Dereferencing NULL or Uninitialized Pointers
Dereferencing a pointer that was never initialized or set to NULL can lead to dire consequences. Here’s how it looks:
#include <iostream>
int main() {
int* ptr = nullptr; // Pointer is initialized to NULL
int value = *ptr; // Dereferencing NULL leads to a segmentation fault
return 0;
}
In this scenario, `ptr` is intentionally pointing to `NULL`. Attempting to dereference `NULL` causes a segmentation fault since the program is trying to access a memory location that does not exist.
Buffer Overflows
What is Buffer Overflow?
A buffer overflow occurs when a program writes more data to a buffer than it can hold. This can overwrite adjacent memory, leading to undefined behavior, including segmentation faults.
#include <iostream>
#include <cstring>
int main() {
char buffer[10];
strcpy(buffer, "This string is way too long!"); // Buffer overflow
return 0;
}
The above code exceeds the allocated size for `buffer`, which might overwrite critical parts of memory, potentially causing a segmentation fault.
Preventing Buffer Overflows
To mitigate buffer overflows, use safer functions for string and memory handling, such as `strncpy()` or implementing checks on buffer boundaries. Always ensure proper memory allocation and usage practices.
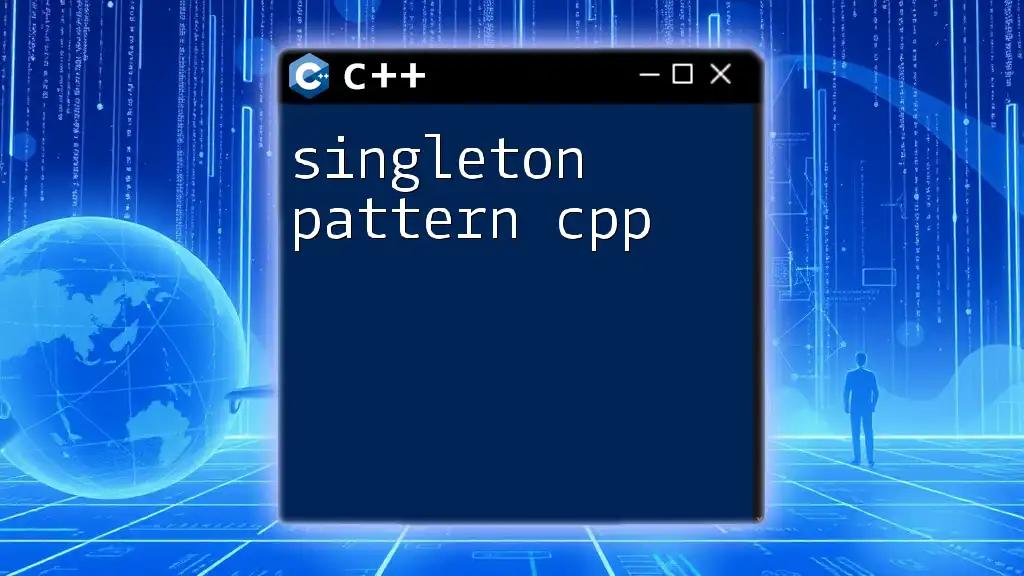
Recognizing Segmentation Faults
Error Messages
When encountering a segmentation fault, common error messages might include phrases like "Segmentation fault (core dumped)." Understanding these messages can significantly help locate and address the issue promptly.
Debugging Techniques
Using tools like gdb (GNU Debugger) allows developers to identify and fix segmentation faults effectively. Here’s a brief on how to use it:
-
Compile your code with debugging symbols enabled:
g++ -g my_program.cpp -o my_program
-
Run the program within gdb:
gdb ./my_program
-
Utilize `run` command to execute the program and `bt` for backtrace if interrupted by a segmentation fault.
Analyzing core dumps provides additional insights into memory usage and allocation at the moment the fault occurred.

Best Practices to Avoid Segmentation Faults
Regularly Initialize Variables
Initializing your pointers and variables is a critical practice. Uninitialized memory can lead to unpredictable program behavior, including experiences with segmentation faults. For example:
int* ptr; // Uninitialized pointer
*ptr = 10; // Undefined behavior leading to a segmentation fault
In contrast, always initialize:
int* ptr = nullptr; // Safe initialization
Use Smart Pointers
Smart pointers such as `unique_ptr` and `shared_ptr` automatically manage memory, reducing the likelihood of memory leaks and segmentation faults. Here’s a brief demonstration:
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr = std::make_unique<int>(5);
std::cout << *ptr; // Safely accesses memory
return 0;
}
Using smart pointers not only enhances code safety but also simplifies memory management.
Implement Boundary Checks
Always implement checks when accessing arrays and memory. Defensive coding practices prevent out-of-bounds access and lead to safer code. Examples include:
if (index >= 0 && index < array_size) {
// Safe to access array
}
Memory Management Techniques
Proper allocation and deallocation of memory are crucial to avoiding segmentation faults. Here’s how you should handle dynamic memory in C++:
int* array = new int[10]; // Dynamically allocate an array
// ... use the array
delete[] array; // Ensure memory is released

Case Studies of Segmentation Faults
Real-World Example
Many large open-source projects face segmentation faults regularly. For instance, the widely used software library libcurl has had instances in its historical versions where improper memory access led to segmentation faults. Analyzing these incidents provides insights into the importance of careful memory management.
Lessons Learned
From these case studies, developers can glean that robust testing, combined with an understanding of memory management, significantly mitigates the risk of segmentation faults. Regular code reviews and employing automated testing tools can catch potential issues early.

Conclusion
In summary, segmentation faults in C++ present significant challenges to developers centered around memory access violations. A comprehensive understanding of their causes, recognition patterns, and preventive measures empowers you to write safer, more efficient code. Always remember to practice good memory management, implement boundary checks, and utilize modern tools like smart pointers to safeguard your applications.

Resources for Further Learning
For those wishing to deepen their understanding, consider exploring resources such as:
- Books on C++ memory management
- Online courses focusing on debugging and C++ best practices
- Tools like Valgrind to analyze memory usage

Call to Action
Join the community and engage with fellow developers through forums and coding groups. Subscribe for more insightful C++ tutorials, tips, and best practices!