The `sizeof` operator in C++ is used to determine the size, in bytes, of a double data type, which can be crucial for memory allocation and optimization.
Here's an example code snippet demonstrating its usage:
#include <iostream>
int main() {
std::cout << "Size of double: " << sizeof(double) << " bytes" << std::endl;
return 0;
}
What is a Double in C++?
Definition of Double
In C++, `double` is a fundamental data type used to represent floating-point numbers that require double precision. This means that `double` can store significantly large or small numbers and can handle fractions due to its decimal point.
`double` is particularly noted for its higher precision compared to its counterpart `float`, which typically represents single-precision floating point numbers. For cases that demand even more precision, C++ provides the `long double` type, which expands the range and precision beyond `double`, although the implementation can vary by system.
Characteristics of Double
One of the main characteristics of the `double` data type is its ability to represent numbers with approximately 15 to 17 decimal digits of precision. This level of precision makes it suitable for various applications like scientific computations and financial calculations.
To sum up, the `double` data type is generally preferred when:
- High precision is required.
- You are working with very large or very small numeric values.

Understanding Size of Double in C++
Fundamental Concept of Size
In C++, understanding the size of data types is crucial for memory management. The `sizeof` operator helps programmers determine the amount of memory consumed by a specific data type.
The syntax to use `sizeof` is straightforward:
sizeof(type);
For example, to find the size of a `double`, you would use:
sizeof(double);
Size of Double on Different Platforms
The size of the `double` data type is typically 8 bytes (64 bits) on most platforms, but it's important to recognize that this can vary based on architecture and compiler implementations. For instance, when running systems such as:
- Windows: Most compilers adhere to the standard which shows `sizeof(double)` as 8 bytes.
- Linux: Similarly, the common desktop compilers like GCC report 8 bytes for `double`.
- macOS: The situation is consistent with most compilers reporting `double` to also occupy 8 bytes.
It is essential to be aware that while the size might be consistent on many platforms, it is good practice to check this locally, especially when working with cross-platform applications.

Code Example: Finding the Size of Double
Basic Example
A simple way to confirm the size of `double` in C++ is by using the following code snippet:
#include <iostream>
using namespace std;
int main() {
cout << "Size of double: " << sizeof(double) << " bytes" << endl;
return 0;
}
Explanation:
- `#include <iostream>`: This line includes the input/output stream library, which is necessary for using `cout`.
- `cout`: This is used for outputting to the console.
- The expression `sizeof(double)` returns the size of the double data type, which gets printed to the console.
When this program is executed, you will receive an output indicating the size of `double`, which should confirm our previous discussions.
Size in Practice
Understanding the size of `double` is not just an academic exercise; it has practical implications. In fields like scientific computing, where large datasets are common, using `double` can result in considerable memory usage. It's advisable to consider memory limits and efficiency, especially in environments with constrained resources.
By knowing that `double` generally occupies 8 bytes, programmers can make informed decisions on data storage and processing techniques to optimize performance.

Memory Alignment of Double
What is Memory Alignment?
Memory alignment refers to how data is organized and accessed in memory. Proper memory alignment ensures that data types are stored in memory addresses that align well with their sizes, leading to efficient access and manipulation.
Alignment in Relation to Double
When working with `double`, memory alignment typically requires variables to be located at memory addresses that are multiples of 8 bytes. This can enhance the performance since accessing aligned memory is generally faster than unaligned memory.
To illustrate this, consider a structure that includes a `double`:
struct Example {
char a; // 1 byte
double b; // 8 bytes
};
The `Example` struct may require extra padding to ensure `b` is properly aligned. Depending on the compiler, the compiler may introduce padding bytes between `a` and `b` to align `b`. This means that the memory layout could look like:
- 1 byte for `a`
- 7 bytes of padding
- 8 bytes for `b`
This type of memory layout is commonly referred to as a struct alignment issue, and it emphasizes the necessity of understanding both the size of `double` and its alignment.

Conclusion
In summary, comprehending size double C++ is an essential aspect of mastering C++ programming. Knowing what `double` is and the memory it occupies helps in utilizing the language effectively.
By employing the `sizeof` operator and recognizing that `double` typically takes up 8 bytes on most modern platforms, you can make informed decisions when it comes to optimizing memory and processing efficiency.
As you continue your journey in C++, keep pushing the boundaries of your understanding of data types, and leverage that knowledge to create efficient and high-performance applications.

Further Reading and Resources
To deepen your knowledge about C++ data types, consider exploring textbooks and online resources tailored for programmers of various skill levels. Engaging with community forums can also provide valuable insights and support as you venture further into understanding more complex aspects of C++.
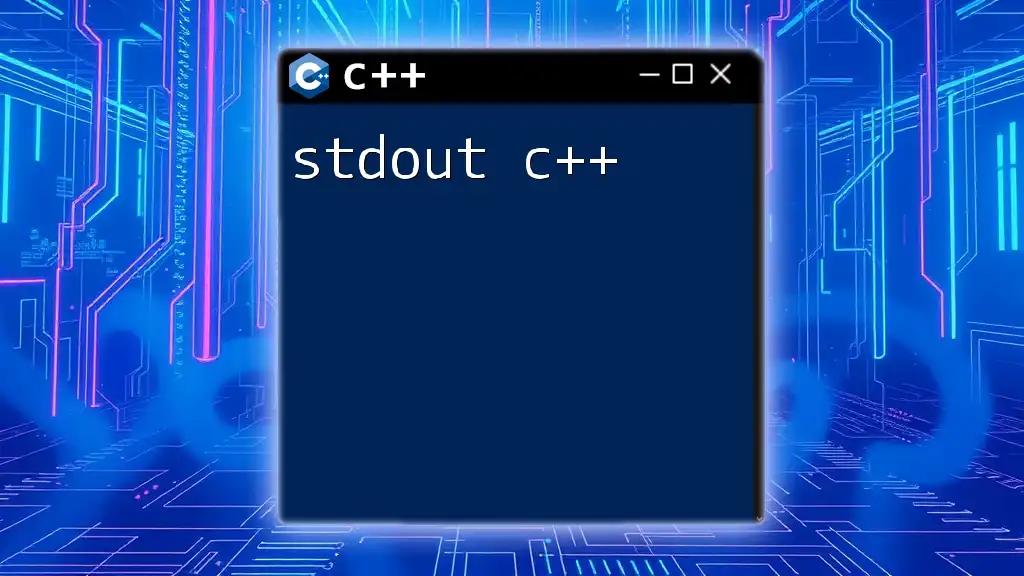
FAQ Section
Common Questions about Double Size in C++
What is the typical size of a double in C++?
The typical size of a `double` in C++ is 8 bytes, although this can vary based on the platform and compiler used.
Why does the size of a double differ across platforms?
Although the C++ standard specifies that `double` must provide at least 15 decimal precision, the actual size can differ due to implementation differences in compilers and hardware.
How can I optimize memory usage with doubles in C++?
You should consider the following strategies:
- Use `float` if less precision is acceptable.
- Evaluate whether `double` is necessary for large arrays; consider alternatives where applicable.
- Avoid unnecessary copies of doubles in your code. Use references or pointers where feasible to minimize memory overhead.