The `double` data type in C++ is used to represent floating-point numbers with double precision, allowing for more accurate representation of decimal values compared to the `float` type.
double pi = 3.14159;
Understanding the C++ Double Type
What is the C++ Double Type?
The double keyword in C++ represents a double-precision floating-point data type. It can store decimal values and is commonly used for calculations that require a high degree of accuracy. A double variable generally provides more precision than the float type, making it suitable for situations where small differences in decimal values matter, such as in scientific computations or financial applications.
The key differences between float and double include:
- Precision: A float typically has 7 decimal digits of precision, while a double provides around 15 decimal digits.
- Storage Size: A float usually takes up 4 bytes, whereas a double occupies 8 bytes in memory.
- Range: Doubles can represent a wider range of numbers than floats, making them more versatile in calculations that require very large or very small values.
Characteristics of C++ Double Variable
A double variable in C++ is defined as a variable specifically designated to store double-precision floating-point numbers. These variables are instrumental in applications that demand precision, such as mathematical modeling or simulations.
When you declare a double variable, the C++ compiler allocates 8 bytes of memory to store the variable's value. The necessity for using a double primarily arises due to the requirements for more significant decimal precision, especially in calculations that might involve fractions.
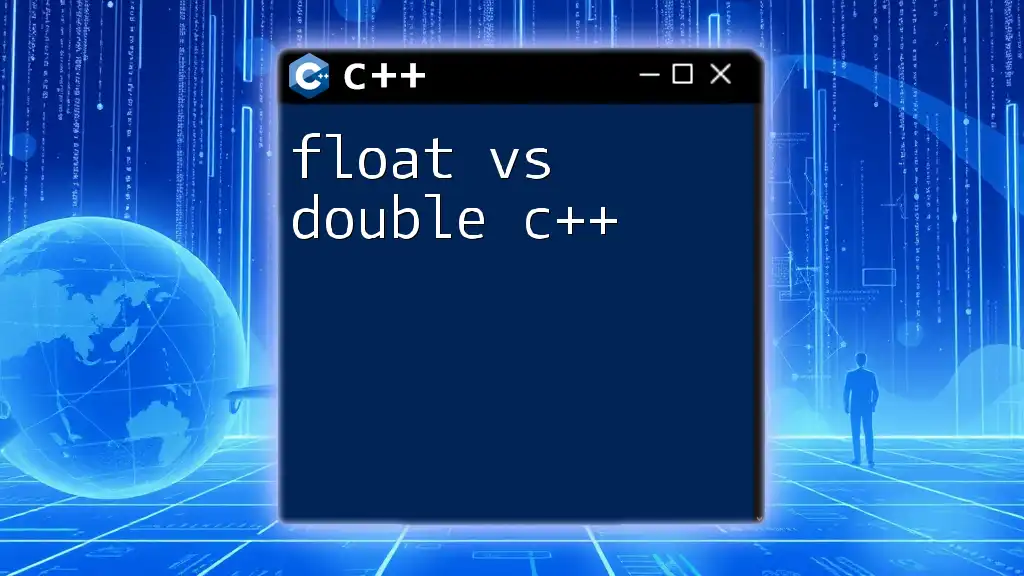
Declaring and Initializing Double Variables
Syntax for Declaring a Double Variable
Declaring a double variable in C++ is straightforward. You use the `double` keyword followed by the variable's name. For example:
double myDouble;
This command creates a double variable named `myDouble`, which can later be assigned with a decimal value.
Initializing a Double Variable
Initialization is another crucial step in working with double variables. There are multiple ways to initialize a double variable:
double myDouble = 5.89; // Direct initialization
double myDouble(3.14); // Initialization using parentheses
You can opt for implicit initialization, where the value is directly assigned upon declaration, or explicit initialization, instantiating the variable with parentheses.
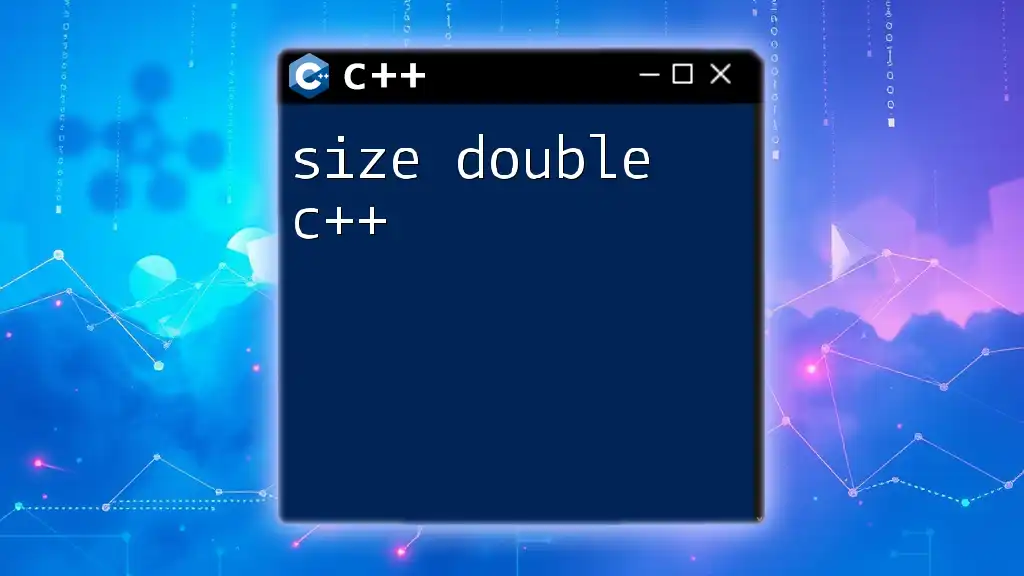
Operations with Double Variables
Arithmetic Operations
When dealing with double variables, you can perform a variety of arithmetic operations. The common operations include addition, subtraction, multiplication, and division. Here’s how you can execute these operations:
double a = 5.5, b = 2.2;
double sum = a + b; // Addition
double difference = a - b; // Subtraction
double product = a * b; // Multiplication
double quotient = a / b; // Division
In these examples, you can see how double variables interact seamlessly with basic arithmetic operators to produce accurate results.
Advanced Mathematical Functions
C++ also allows the use of advanced mathematical functions on double variables through the `<cmath>` library. You can use functions like `sqrt`, `pow`, and `sin` to perform more complex calculations. Here's an example of how to compute the square root:
#include <cmath>
double result = sqrt(myDouble); // Calculates the square root of myDouble
It’s essential to include the necessary header when using these functions to expand the capabilities of your double variables.
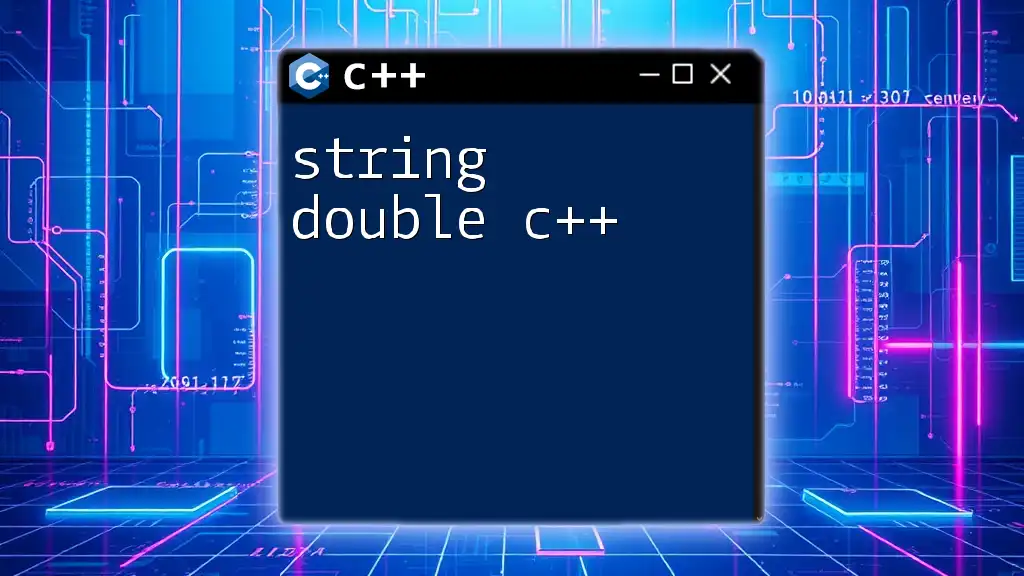
Converting Between Data Types
Type Casting in C++
Type casting is an essential feature in C++. There are two primary types of casting: implicit and explicit. Implicit casting occurs when data is automatically converted by the compiler, while explicit casting requires you to manually change the type.
For example, to convert a double to an integer and vice versa, you can use the following code:
int intValue = (int) myDouble; // Explicit conversion from double to int
double anotherDouble = static_cast<double>(intValue); // Conversion back to double
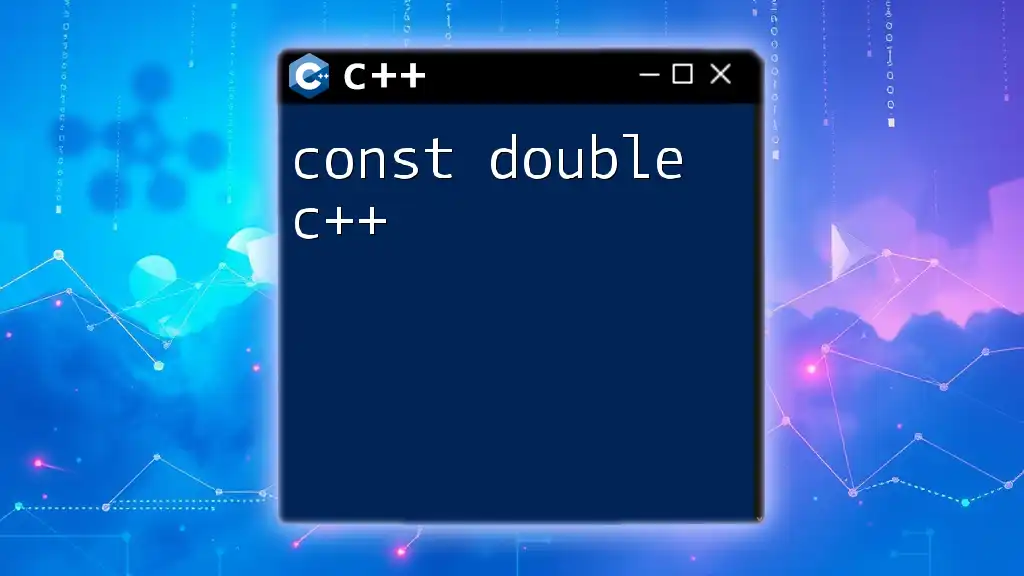
Common Pitfalls with Double Variables
Floating Point Precision Issues
One of the primary concerns when working with the data type double C++ is floating-point precision issues. Due to the nature of binary representation, certain decimal values may not be represented accurately. This can lead to unexpected results in calculations. For instance:
double d1 = 0.1;
double d2 = 0.2;
double sum = d1 + d2; // May not equal 0.3 due to precision issues
Comparing Double Variables
Because of potential precision errors, using the `==` operator for comparing double values can lead to inaccuracies. A better practice involves using a small threshold (epsilon) to evaluate if two double values are close enough to be considered equal. Here's how you can implement this:
const double epsilon = 1e-9; // Define a small tolerance value
if (fabs(d1 - d2) < epsilon) {
// Treat d1 and d2 as equal
}
This method ensures a more reliable comparison of floating-point numbers.
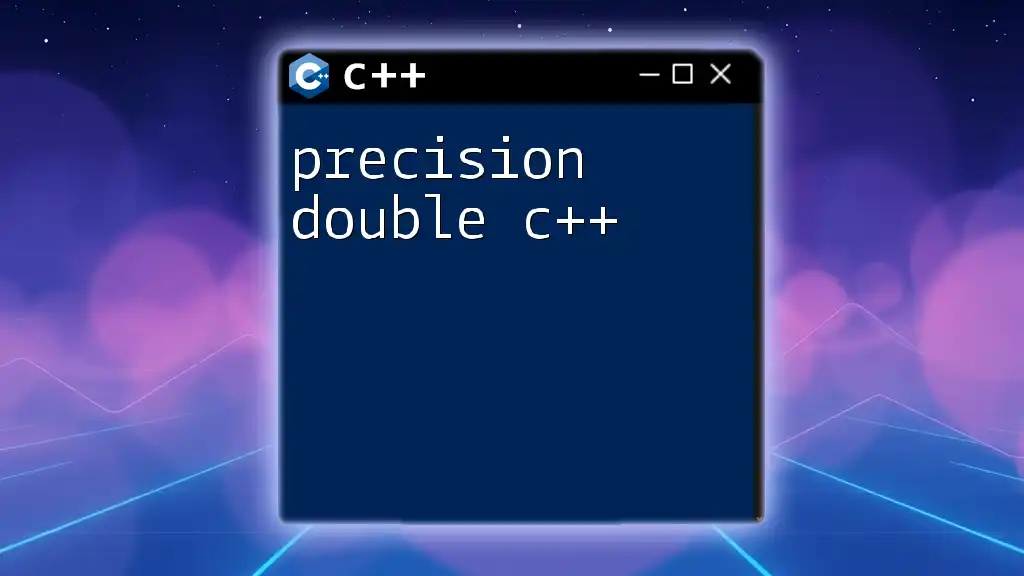
Best Practices for Using Double Variables in C++
When to Use Double Over Other Numeric Types
Knowing when to use the double type is crucial for efficient programming. Opt for double when dealing with calculations that involve fractions, high precision, or a significant range of numbers. However, be mindful that increased precision often comes with a tradeoff in performance, especially in resource-constrained environments.
Code Readability and Maintainability
In coding, clarity often trumps complexity. When naming your double variables, use descriptive names that clearly convey their purpose. Comments and documentation can help maintain readability for others or for yourself in the future, especially in code sections involving complex arithmetic or mathematical functions.
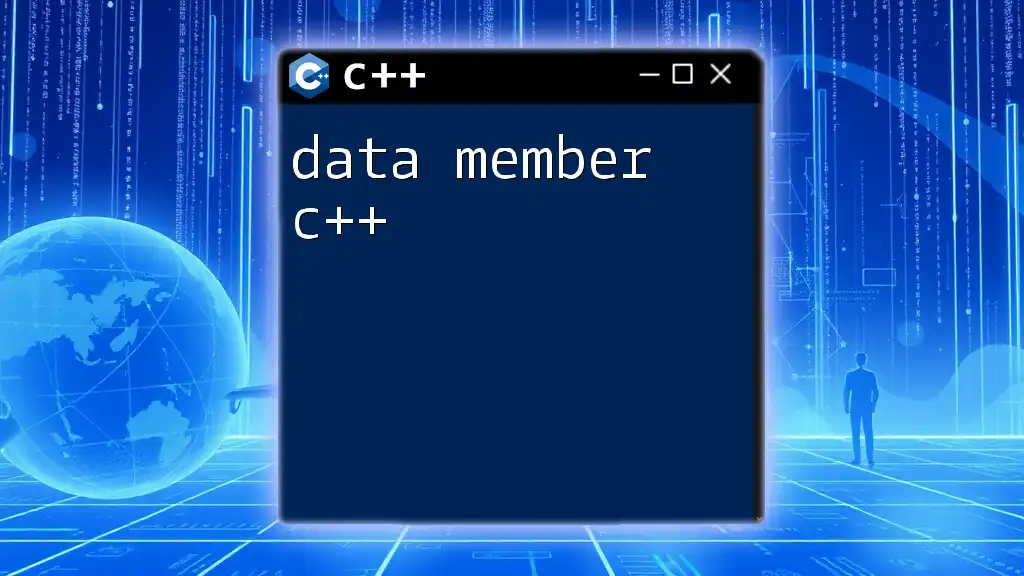
Conclusion
In summary, understanding the data type double C++ is vital for any programmer who needs to perform decimal arithmetic with precision. By grasping the characteristics, operations, and potential pitfalls of double variables, you equip yourself with the tools needed for effective programming practices. As you practice implementing these principles, you'll find yourself able to tackle a wide array of computational challenges with confidence.
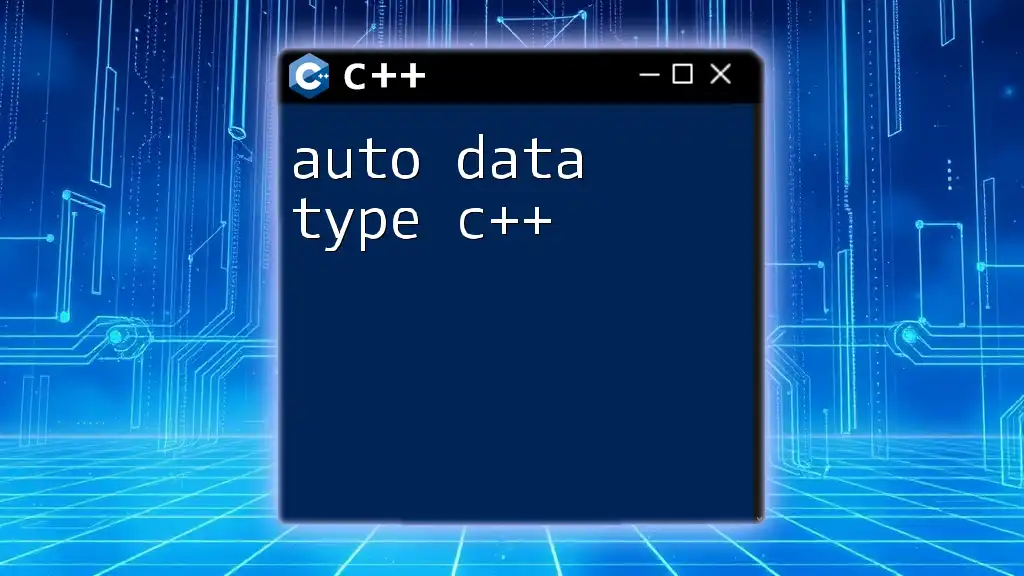
Additional Resources
Useful Links and References
For further learning, consider the following resources to deepen your understanding of C++ data types and the specific workings of double variables:
- [C++ Documentation on Data Types](https://en.cppreference.com/w/cpp/language/data_types)
- [Online Courses for C++ Mastery](https://www.codecademy.com/learn/learn-c-plus-plus)
Frequently Asked Questions
Understanding common queries about double variables can enhance your learning:
- What is the maximum value a double can store?
- Why do many programming languages prefer float over double for performance reasons?
These discussions will enhance your practical understanding and application of the double data type in C++.