The dot product in C++ can be calculated using the `std::inner_product` function from the `<numeric>` library, which computes the sum of the products of corresponding elements from two sequences.
#include <iostream>
#include <vector>
#include <numeric>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
int dotProduct = std::inner_product(vec1.begin(), vec1.end(), vec2.begin(), 0);
std::cout << "Dot Product: " << dotProduct << std::endl; // Output: 32
return 0;
}
Understanding the Dot Product
What is the Dot Product?
The dot product is one of the fundamental operations in vector mathematics. Mathematically, it is represented as \( A \cdot B = |A| |B| \cos(\theta) \), where \( |A| \) and \( |B| \) are the magnitudes (lengths) of vectors A and B, and \( \theta \) is the angle between them. The result of the dot product is a scalar, providing information about the directional relationship between the two vectors.
Geometric Interpretation: Conceptually, the dot product measures how much of one vector goes in the direction of another. A dot product of zero indicates that the vectors are orthogonal (perpendicular), while higher values suggest a strong directional alignment.
The dot product has significant applications in numerous fields, including physics for calculating work, graphics for lighting computations, and machine learning for operations with data vectors.
The Properties of Dot Product
The dot product exhibits several important properties:
-
Commutative Property: The dot product is commutative, which means \( A \cdot B = B \cdot A \). This property is useful when rearranging terms in equations.
-
Distributive Property: It follows the distributive property, allowing us to expand the product of a vector with a sum. For instance, \( A \cdot (B + C) = A \cdot B + A \cdot C \).
-
Relation to Vector Magnitudes: A deeper understanding of the dot product helps in calculating angles and lengths between vectors, serving as a bridge between algebraic and geometric interpretations.
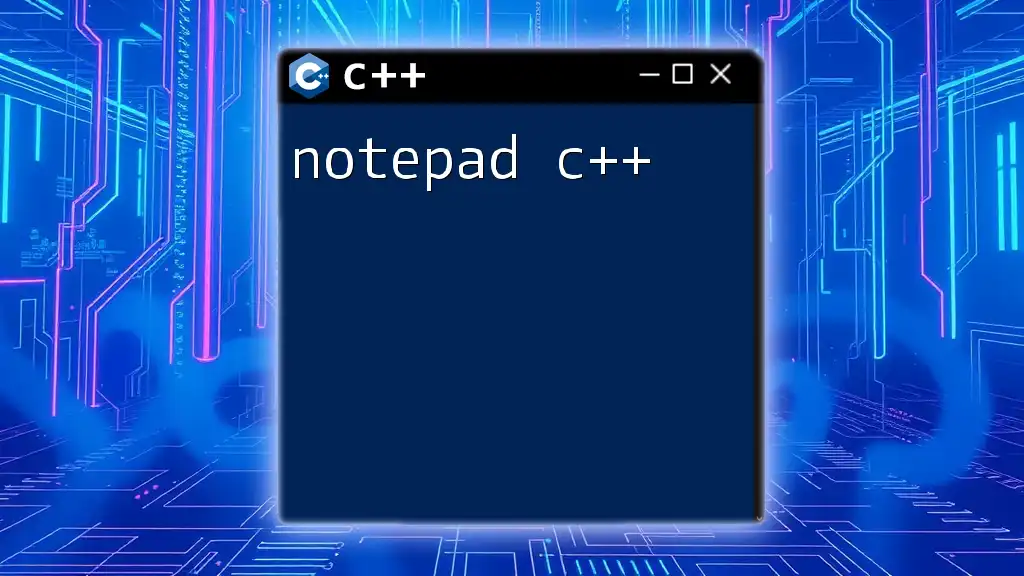
Implementing Dot Product in C++
Basics of Vectors in C++
In C++, vectors can be represented using either the Standard Template Library (STL) vector or a custom vector class. Utilizing a custom class allows for greater flexibility and is beneficial for education purposes, leading to deeper understanding.
As an example, a simple vector class for 3D vectors might look like this:
class Vector {
public:
float x, y, z; // 3D vector
Vector(float x, float y, float z) : x(x), y(y), z(z) {}
};
Here, we define a vector that encapsulates its three-dimensional coordinates as member variables. This will allow us to easily access and manipulate the vector components when performing operations, such as calculating the dot product.
Function to Calculate Dot Product
Writing the Dot Product Function
Next, we can implement a function to calculate the dot product. The function takes two vectors as parameters, allowing us to compute the dot product directly.
float dotProduct(const Vector &A, const Vector &B) {
return A.x * B.x + A.y * B.y + A.z * B.z;
}
This function leverages reference parameters for efficiency, preventing unnecessary copies of the vector objects.
Step-by-Step Explanation of the Code
-
Parameter Declaration: Note that we declare parameters as references (`const Vector &A`) to avoid copying the vector data. This is particularly important when working with large vectors, as it improves performance.
-
Dot Product Calculation: The function essentially computes the sum of the products of the corresponding components of the two vectors—this is the essence of how the dot product is mathematically defined.
Example Usage of the Dot Product Function
Here’s how you can use the dot product function in a practical example:
int main() {
Vector A(1.0, 2.0, 3.0);
Vector B(4.0, 5.0, 6.0);
float result = dotProduct(A, B);
std::cout << "Dot Product: " << result << std::endl; // Output: 32
return 0;
}
In this example, we create two vectors, A and B, and calculate their dot product, which yields the output of 32. This result comes from the calculation: \( (14) + (25) + (3*6) = 4 + 10 + 18 = 32 \).
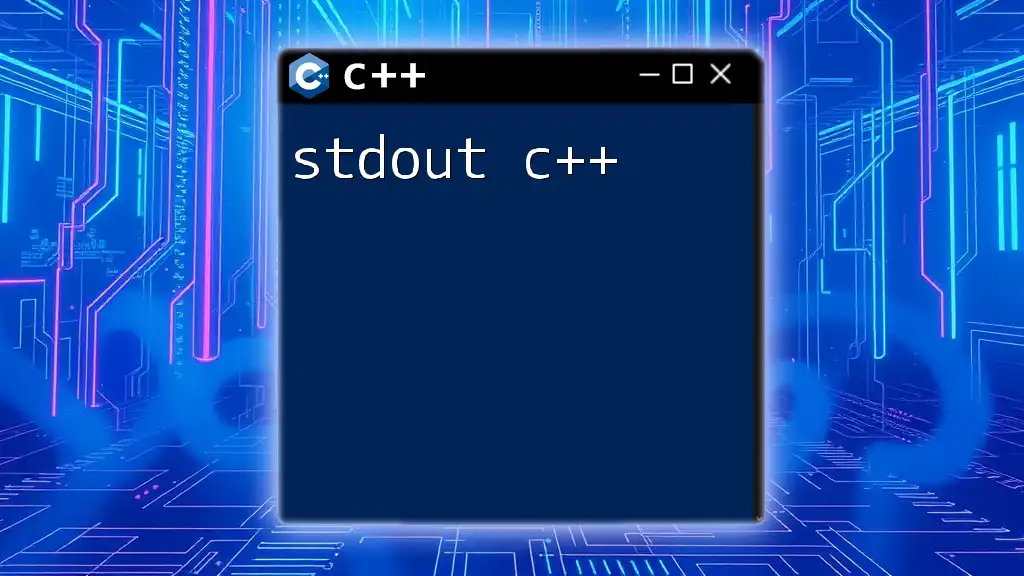
Advanced Techniques and Best Practices
Handling Different Vector Sizes
A significant limitation of fixed-size vectors is that they cannot accommodate vectors of varying dimensions. To build a more adaptable solution, consider using a template-based approach to allow for vectors of any length.
template <typename T>
T dotProduct(const std::vector<T> &A, const std::vector<T> &B) {
if (A.size() != B.size()) throw std::invalid_argument("Vectors must be of same length.");
T result = 0;
for (size_t i = 0; i < A.size(); ++i) {
result += A[i] * B[i];
}
return result;
}
In this implementation, we check for vector size equality before performing the calculation. If they differ, an exception is thrown, ensuring robustness in our function.
Optimizing Dot Product Calculations
When working with large datasets, performance becomes a critical factor. To enhance the efficiency of dot product calculations, consider using multithreading. The C++11 standard provides a seamless way to implement threads for parallel calculations.
For instance, divide the vector into segments and calculate partial dot products, combining the results afterward. However, the actual implementation may vary based on specific use cases and environment settings.
Error Handling and Input Validation
Validating vector lengths and implementing robust exception handling is crucial for maintaining program integrity. By checking for input validation before proceeding with calculations, you can prevent runtime errors, ensuring a smoother user experience.
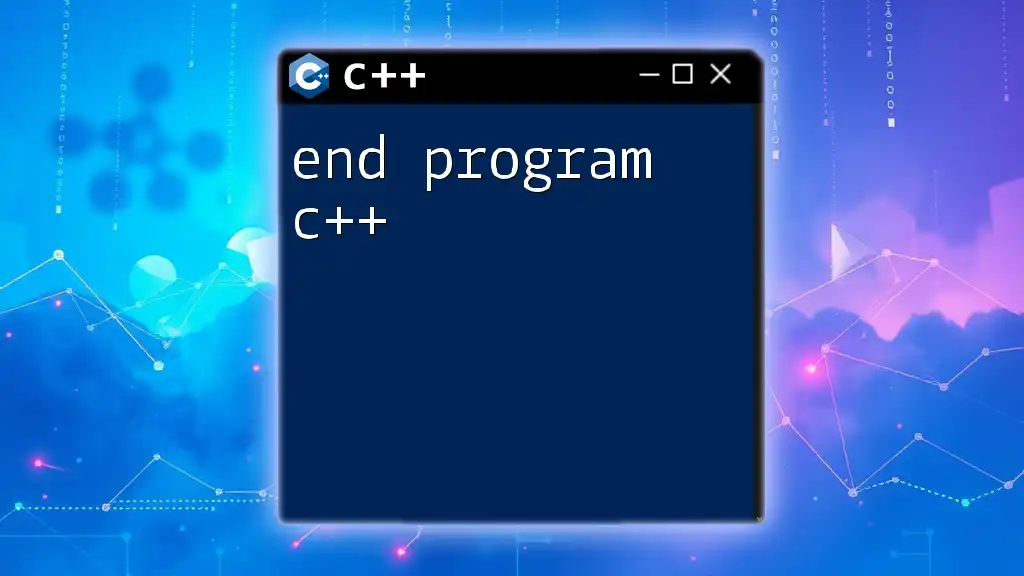
Real-World Applications of Dot Product
In Physics
The dot product is commonly used to calculate work done by a force. Work is defined as the dot product of force and displacement vectors:
\[ W = F \cdot d \]
Using the dot product, you can determine how much of the force efficiently contributes to moving an object along a given path, aiding engineers in designing more efficient systems.
In Computer Graphics
In computer graphics, the dot product is vital for lighting calculations. It helps determine the angle between light sources and surfaces, impacting shading and rendering outputs. Proper implementations of the dot product ensure that graphic applications are visually accurate and appealing.
In Machine Learning
In the world of machine learning, vectors are ubiquitous. The dot product is extensively utilized in data classification algorithms, such as the Support Vector Machine (SVM). It helps determine the relationship between data points, guiding decisions on their classifications and associations.
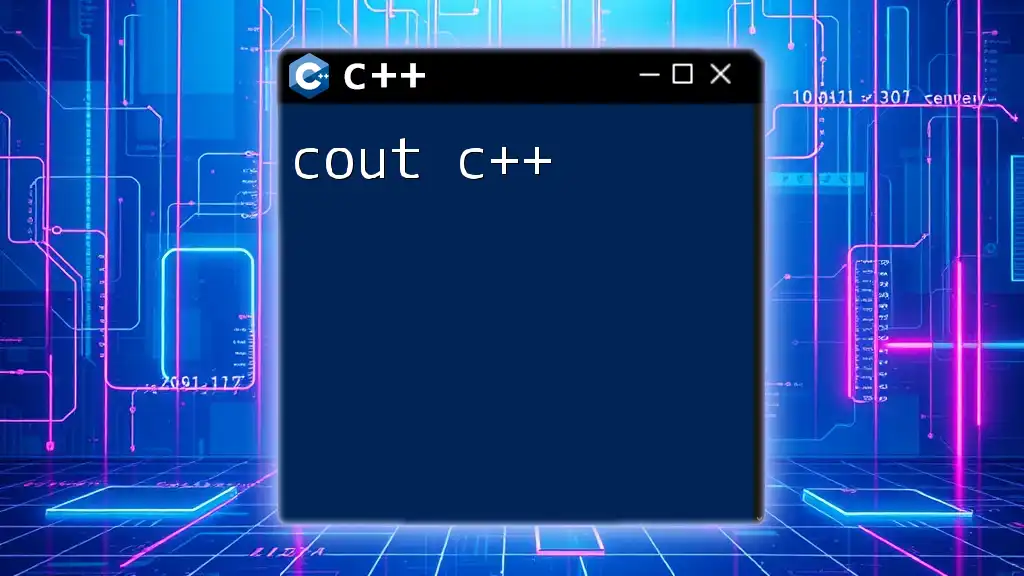
Conclusion
The dot product in C++ is an essential concept that connects various mathematical principles and real-world applications. By understanding its definition, properties, and practical implementations, you can leverage this operation in numerous fields, from physics to computer graphics and machine learning.
Through practice and experimentation, you will deepen your understanding of vector operations, driving your proficiency in C++ programming and mathematical applications alike.
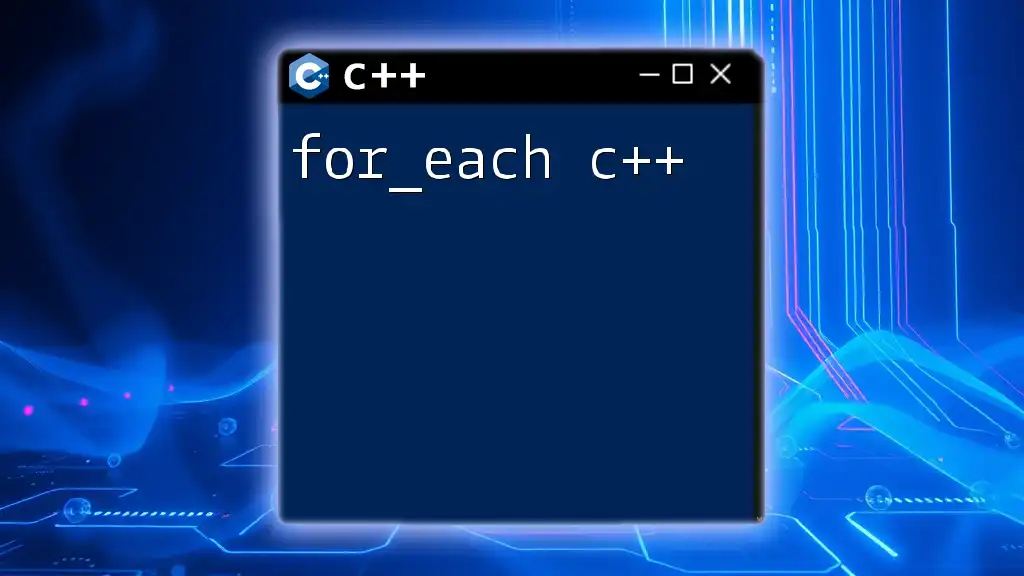
Additional Resources
For those looking to broaden their knowledge, consider exploring:
- C++ vector libraries that offer built-in operations.
- Advanced topics in C++ programming, including template programming and multithreading techniques.
- Code repositories on platforms like GitHub that contain relevant projects and sample implementations.