To generate random numbers within a specified range in C++, you can use the `<random>` library to create a random number generator and then define the desired range using `std::uniform_int_distribution` for integers or `std::uniform_real_distribution` for floating point numbers. Here's a simple example for generating random integers between 1 and 100:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Seed
std::mt19937 gen(rd()); // Mersenne Twister engine
std::uniform_int_distribution<> distr(1, 100); // Range [1, 100]
// Generate and print a random number
std::cout << distr(gen) << std::endl;
return 0;
}
Understanding Random Number Generation in C++
What are Random Numbers?
Random numbers are essential in programming, often used to introduce unpredictability in applications. They can range from generating patterns in games to simulating real-world phenomena in scientific modeling. The key difference between pseudo-random numbers and true randomness lies in how they are generated. Pseudo-random numbers are created by algorithms that produce a sequence that appears random but are not genuinely random.
Random Number Generation in C++
In C++, random number generation primarily relies on two libraries: `<cstdlib>` for simpler implementations and `<ctime>` for time-seeding the random number generator. The `rand()` function in the `<cstdlib>` library generates pseudo-random numbers. While reliable for basic applications, it may not be sufficient for more advanced needs due to its predictable nature.
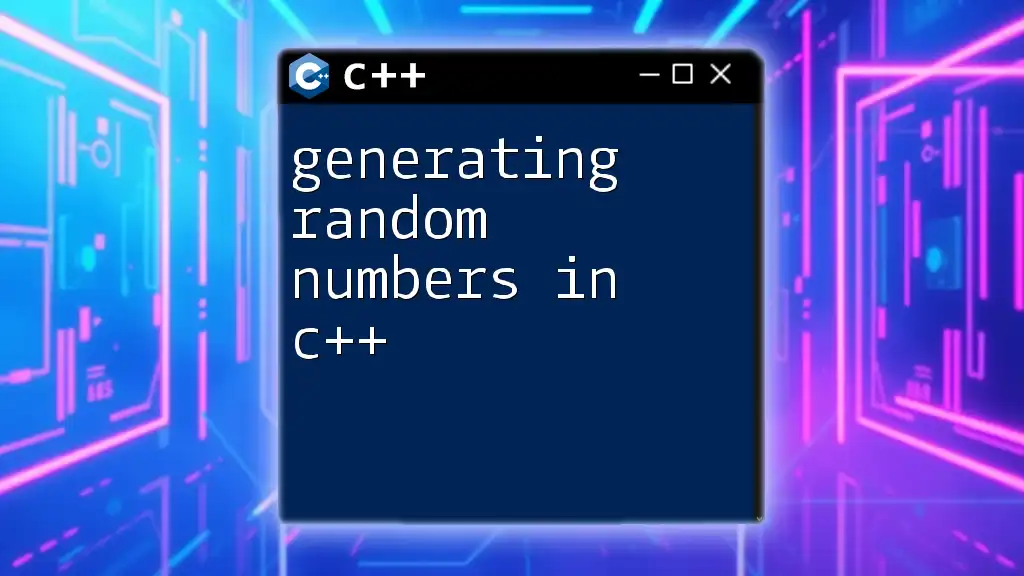
The Basics of Generating Random Numbers Using rand()
Using rand() for Basic Randomness
To begin generating random numbers, the first step is to include the necessary libraries. Here’s a simple example of generating a basic random number:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed the random number generator with the current time
int randomValue = std::rand(); // Generate a random number
std::cout << "Random Value: " << randomValue << std::endl;
return 0;
}
This code snippet uses `std::srand(std::time(0))` to seed the random number generator, making the output different each time the program runs.
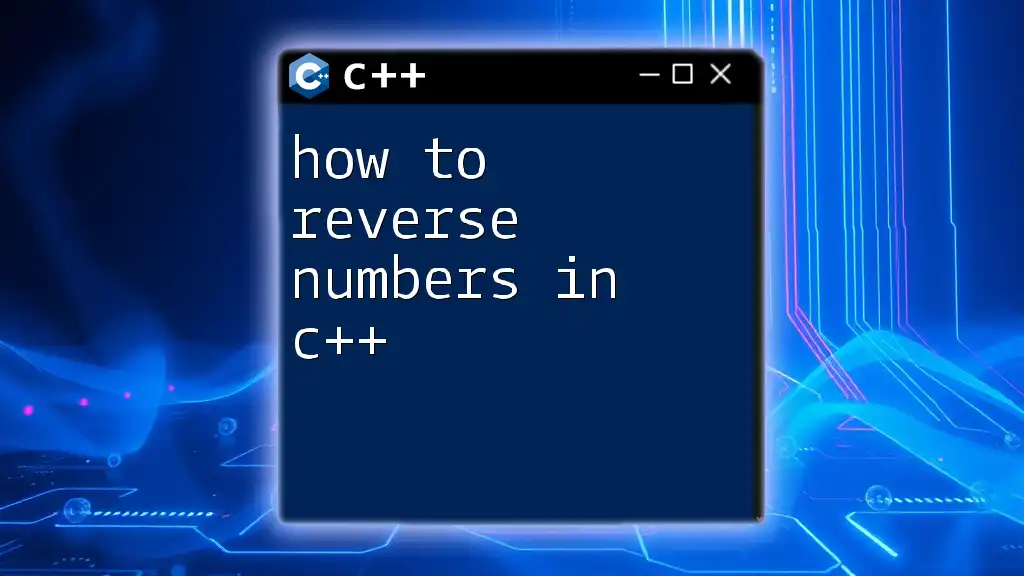
Generating Random Numbers Within a Range
Using the Modulo Operator
To generate random numbers within a specific range, the modulo operator is often used. This operates by taking the result of `rand()` and limiting its output. For instance, to generate a number between 0 and a specified maximum, understand the following code:
int maxValue = 100; // Define the maximum range
int randomInRange = std::rand() % maxValue; // Generate random number from 0 to maxValue
Generating a Random Number in a Specific Range
More commonly, you may want a random number in a certain range `[min, max]`. The formula to achieve this is:
`randomNum = min + (std::rand() % (max - min + 1))`
This allows for inclusive boundaries. Here’s how this looks in C++:
int minValue = 10;
int maxValue = 50;
int randomInSpecificRange = minValue + (std::rand() % (maxValue - minValue + 1));
Example: Get Random Number in Range
Let’s put this knowledge into more comprehensive practice with a function that retrieves a random number within a specified range:
#include <iostream>
#include <cstdlib>
#include <ctime>
int getRandomInRange(int min, int max) {
return min + (std::rand() % (max - min + 1)); // Calculate random number
}
int main() {
std::srand(std::time(0)); // Seed the random number generator
int randomValue = getRandomInRange(1, 100); // Get random number in range 1 to 100
std::cout << "Random Number in Range [1-100]: " << randomValue << std::endl;
return 0;
}
This program defines a function called `getRandomInRange` that encapsulates the logic for generating a random number between `min` and `max`.
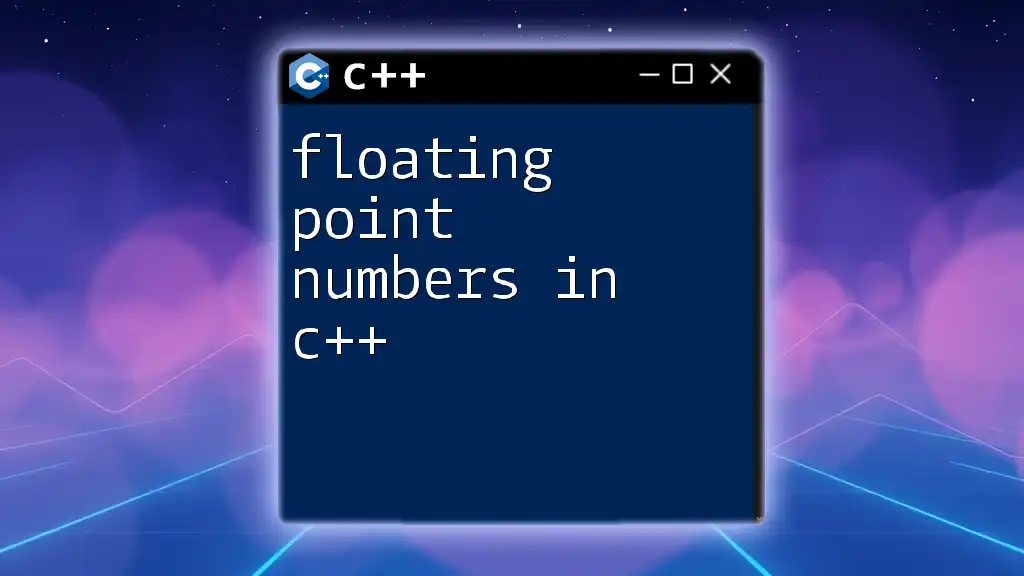
The Limitations of rand() and How to Improve Randomness
Why rand() May Not Be Enough
Despite its utility, the `rand()` function presents limitations, particularly in its predictability. Since it uses a deterministic algorithm based on an initial seed, it can produce sequences that are not random enough for certain applications, such as cryptographic contexts or sophisticated simulations.
Using <random> for Better Randomness
For those seeking a more robust solution, C++11 introduced the `<random>` library. This library provides more powerful random number generation facilities, including various distributions and random number engines.
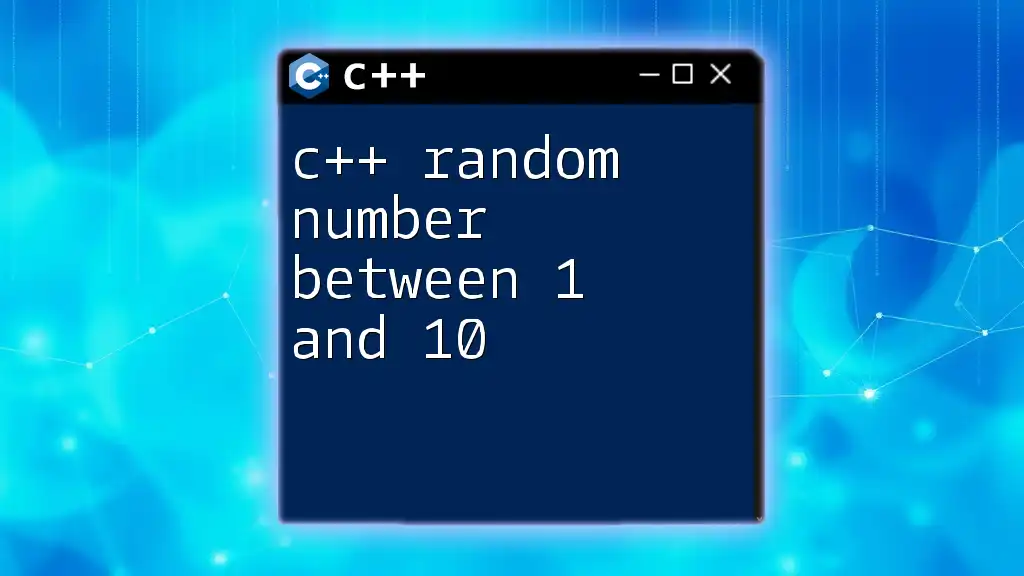
Advanced Random Number Generation Techniques
Generating Random Floats and Doubles
It’s not just integers that can benefit from random number generation. C++ allows for the generation of random floating-point numbers as well. To generate a random float within a specified range, you can use the following approach:
float getRandomFloat(float min, float max) {
float range = max - min; // Calculate the range
return min + static_cast<float>(std::rand()) / (static_cast<float>(RAND_MAX/range)); // Get random float
}
Utilizing Random Engines and Distributions
Employing the `<random>` library unlocks a wealth of randomness options using random engines and distributions. Here’s how to implement a basic uniform distribution:
#include <random>
#include <iostream>
int main() {
std::random_device rd; // Obtain a random number from hardware
std::default_random_engine generator(rd()); // Seed the generator
std::uniform_int_distribution<int> distribution(1, 100); // Define the range
int randomValue = distribution(generator); // Generate random number
std::cout << "Random Value using <random>: " << randomValue << std::endl;
return 0;
}
This approach uses the `std::default_random_engine` to generate a uniformly distributed random number between 1 and 100, offering a better distribution than the traditional `rand()` function.
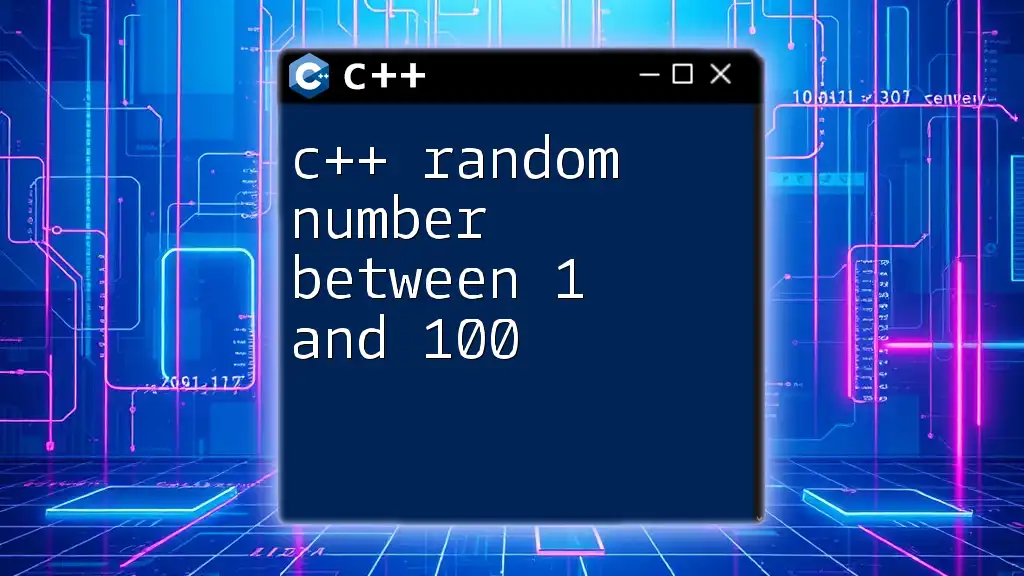
Best Practices for Random Number Generation in C++
Seeding Your Random Number Generator
An often underestimated detail is seeding the random number generator. Properly seeding ensures that the random sequence varies notably across program executions. Using the line `srand(time(0))` is an effective approach, as it seeds the generator with the current time.
Performance Considerations
When it comes to performance, be aware of the implications of various methods of number generation. For applications where performance is crucial, leveraging more advanced methods, such as those found in `<random>`, might be a necessity.
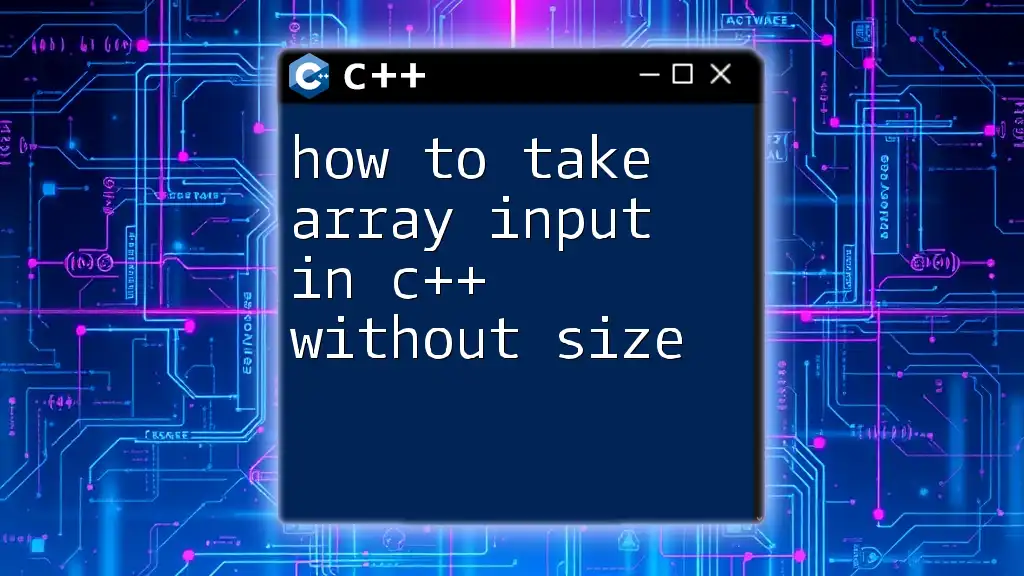
Conclusion
In conclusion, learning how to generate random numbers in C++ with a range involves understanding both basic and advanced techniques. From employing the `rand()` function to harnessing the modern capabilities of the `<random>` library, developers can effectively implement randomness in their applications. As always, the choice of method should depend on the specific needs of the application. With the knowledge and examples provided, you can confidently explore and incorporate random number generation in your C++ programs.
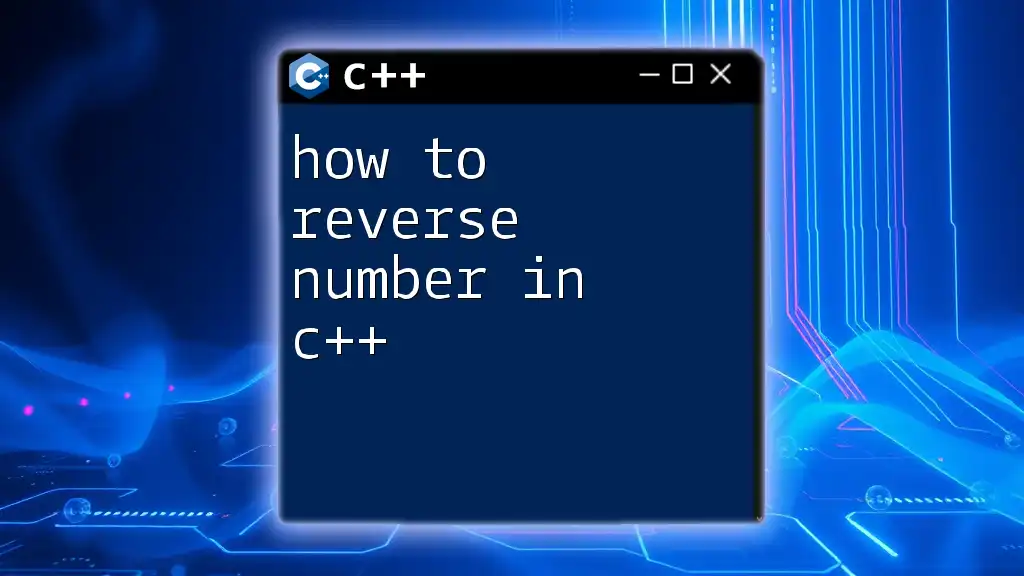
Additional Resources
For deeper understanding and advanced topics in random number generation, consider exploring C++ references, textbooks, or reputable online resources that focus on C++ programming and algorithms.
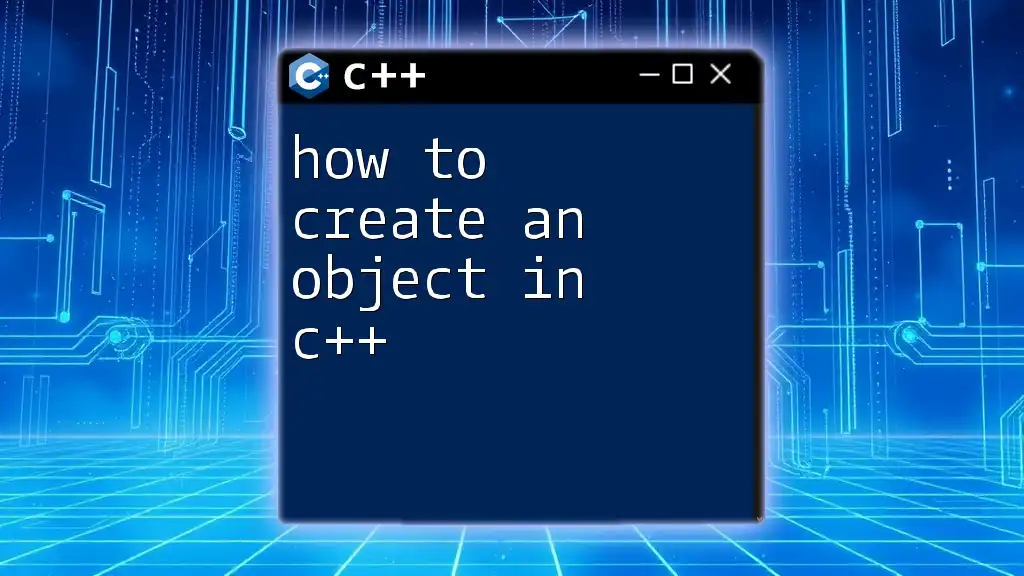
FAQs
What is the difference between rand() and <random>?
`rand()` provides a simple way to generate pseudo-random numbers but lacks flexibility and reliability for advanced applications. The `<random>` library offers a variety of engines and distributions that enrich random number generation, making it a preferred choice for most modern applications.
Can random numbers be truly random in C++?
In computing, random numbers are typically pseudo-random due to their algorithmic nature. Generating true randomness often involves techniques like hardware noise or external environmental inputs.
How can I improve the randomness of my numbers?
To enhance randomness, utilize the `<random>` library for more diverse algorithms and distributions. Additionally, ensure proper seeding to improve the unpredictability of the sequence generated.