To delete a pointer to a pointer in C++, you need to first delete the memory allocated for the inner pointer and then delete the outer pointer to prevent memory leaks, as shown in the example below:
int** ptr = new int*[1]; // allocate outer pointer
ptr[0] = new int(10); // allocate inner pointer
delete ptr[0]; // delete the inner pointer
delete[] ptr; // delete the outer pointer
Understanding Pointers and Pointers to Pointers
What is a Pointer?
A pointer in C++ is a variable that stores the address of another variable. Pointers play a crucial role in dynamic memory management, allowing the developer to allocate and deallocate memory as needed. For instance, consider the following example of a basic pointer declaration and initialization:
int* ptr = new int(10);
In this example, `ptr` is a pointer that holds the address of an `int` type variable initialized with the value `10`. This allows us to manipulate memory directly, offering flexibility but also requiring careful management to avoid errors such as memory leaks.
What is a Pointer to a Pointer?
A pointer to a pointer is simply a pointer that points to another pointer, essentially introducing another layer of indirection. This is particularly useful in certain scenarios such as dynamic two-dimensional arrays or when you need to modify a pointer in a function. Here’s how you can declare a pointer to a pointer:
int** pptr;
In this case, `pptr` can ultimately hold the address of a pointer that itself points to an `int`. Managing these pointers effectively is essential for proper memory handling.

Memory Management in C++
Importance of Memory Management
Proper memory management is critical in C++ programming, especially when using dynamic memory allocation. Not doing so can lead to issues such as memory leaks, where memory that is no longer needed isn't released back to the system.
The `new` and `delete` Operators
C++ provides two important operators for memory management: `new` for allocating memory and `delete` for deallocating memory. Here’s a quick look at its usage:
int* ptr = new int(20); // Allocating an integer
delete ptr; // Deallocating that memory
Using `new` allocates memory from the heap, and using `delete` frees it, thereby preventing memory leaks.
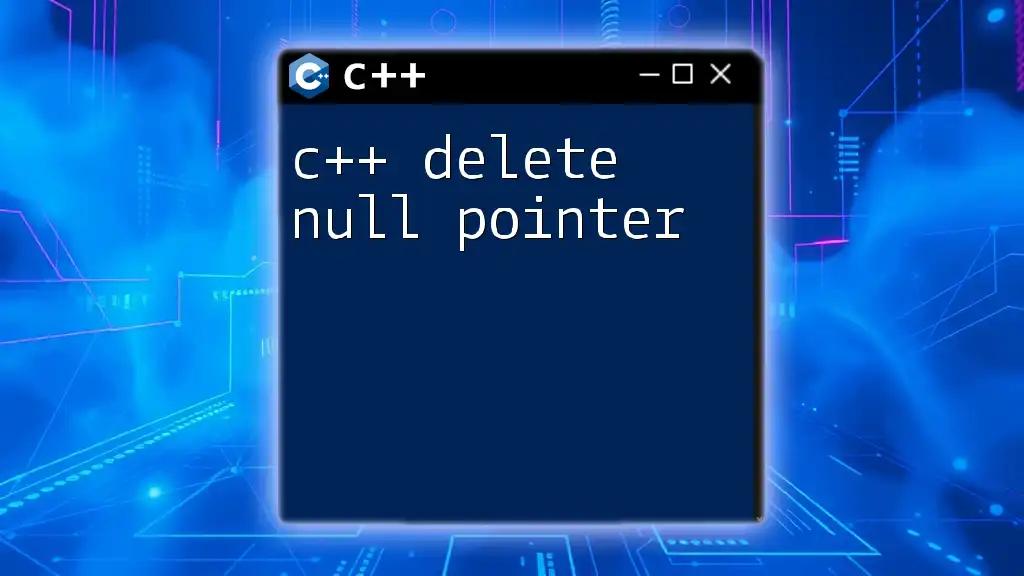
Deleting Pointer to Pointer
Allocating Memory for Pointer to Pointer
When working with a pointer to a pointer, you'll first allocate memory for the inner pointer. Here’s an example of how to allocate a pointer to a pointer:
int** pptr = new int*; // Allocate memory for a pointer
*pptr = new int(30); // Allocate memory for an integer and point to it
In this code, `pptr` is a pointer to a pointer, which is allocated first before pointing to an integer. This layered approach grants you more flexibility in managing complex data structures.
Deleting a Pointer to Pointer: The Concept
Deleting a pointer to a pointer requires a step-by-step approach. You must first delete the memory the inner pointer points to before deleting the outer pointer itself. Failing to do so could lead to memory leaks. Here’s the standard pattern for deletion:
delete *pptr; // Delete the memory allocated for the inner pointer
delete pptr; // Delete the memory allocated for the outer pointer
This ensures that both levels of pointers are properly deallocated, maintaining memory integrity.
Implementing Deletion
Deleting Inner Pointer
To delete the inner pointer you’ve allocated before, use the following code:
delete *pptr; // Free memory allocated for the integer
This line of code frees the memory that the inner pointer points to, which is essential for preventing memory leaks.
Deleting Outer Pointer
After the inner pointer has been deleted, you can now safely delete the outer pointer:
delete pptr; // Free memory allocated for the pointer to pointer
This call eliminates the outer pointer, completing the deallocation operation.
![How to Delete Char in Terminal C++ [Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fh%2Fhow-to-delete-char-in-terminal-cpp.webp&w=1080&q=75)
Best Practices for Working with Pointers to Pointers
Always Initialize Pointers
Uninitialized pointers can lead to undefined behavior and crashes. Always ensure that pointers are initialized, even if you assign them a `nullptr` initially. For example:
int** pptr = nullptr; // Initialize to nullptr
Avoiding Memory Leaks
Using smart pointers, like `std::unique_ptr` or `std::shared_ptr`, can drastically reduce the chances of memory leaks. C++11 and later versions encourage using these modern constructs over raw pointers:
#include <memory>
std::unique_ptr<int> ptr = std::make_unique<int>(40);
Checking for Null Pointers
Before deleting a pointer, it's crucial to check if it is `nullptr` to avoid attempts to delete an already deleted or unallocated memory. You can implement this easily:
if (pptr) {
delete *pptr;
delete pptr;
}
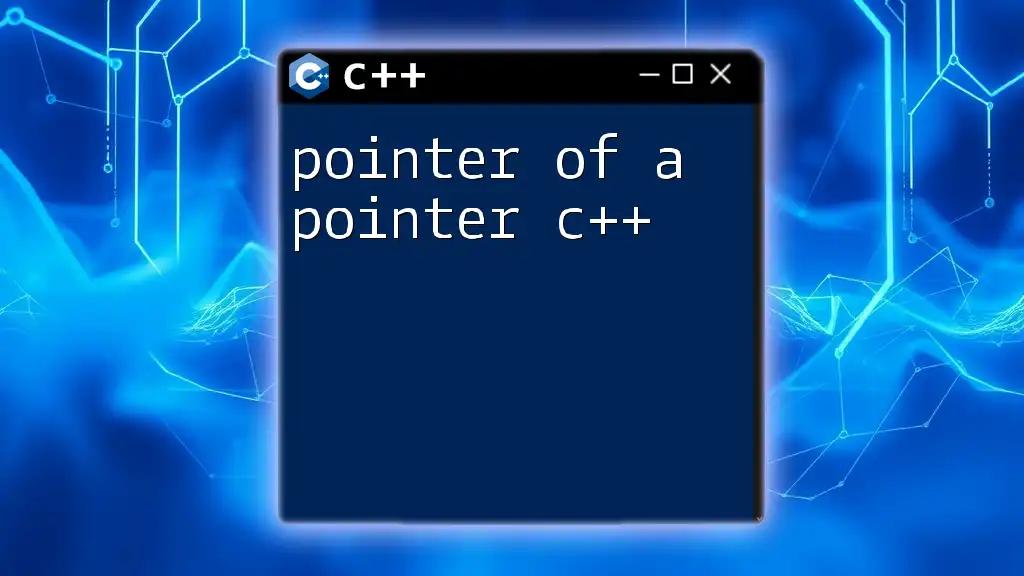
Common Pitfalls and Troubleshooting
Dangling Pointers
A dangling pointer arises when a pointer still holds the address of a memory location that has been deallocated. To prevent this:
- Set pointers to `nullptr` after deletion to signify they no longer point to valid memory:
delete *pptr;
*pptr = nullptr; // Prevent dangling pointer
Double Deletion
Double deletion happens when the same memory is deallocated more than once. This can lead to undefined behavior. Ensure that your pointers are set to `nullptr` after deallocation to avoid this scenario.
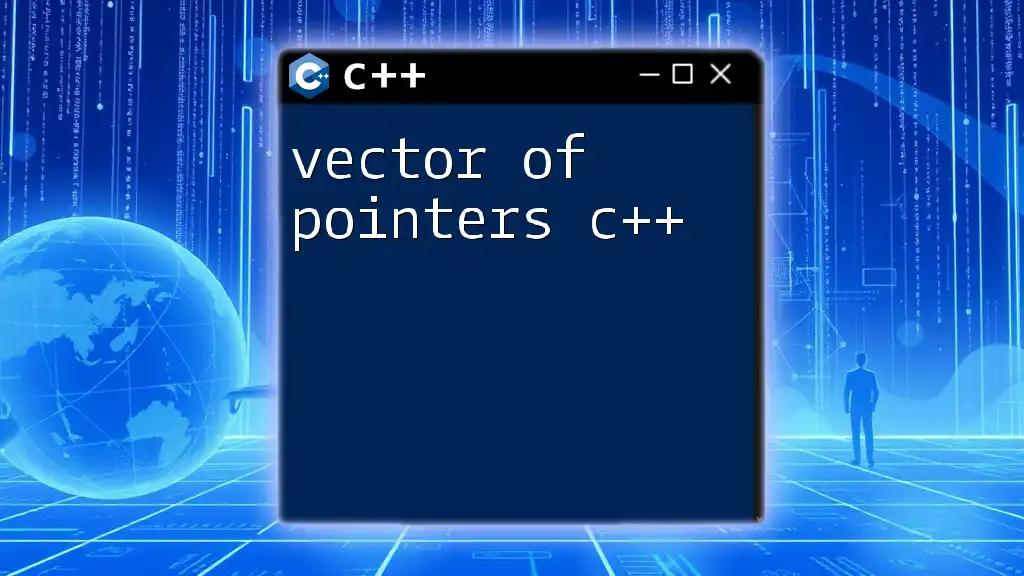
Conclusion
Understanding how to delete pointer of pointer in C++ is vital for effective memory management. By ensuring proper allocation and deallocation of memory, you reduce the risk of memory leaks and maintain the integrity of your application. Always adhere to best practices in managing pointers, such as initializing them, using smart pointers, and performing null checks.
This knowledge will be invaluable as you continue to develop robust, efficient C++ applications. Happy coding!