To delete a character in the terminal using C++, you can utilize the `system()` function to execute a shell command that performs the deletion, as shown in the following example:
#include <iostream>
#include <cstdlib>
int main() {
int position = 5; // Specify the position of the character to delete
std::cout << "\033[1D\033[0K"; // Move cursor left and clear the character
return 0;
}
This snippet moves the cursor one position left and clears the character at that position in the terminal.
Understanding Character Deletion in C++
Character deletion is essential in C++, especially when manipulating strings. Managing strings effectively is crucial as it allows programmers to refine their data handling, streamline output, and prepare data for processing. Learning how to delete characters efficiently can save time and improve the readability of your code.
What are C++ Strings?
C++ provides different ways to handle strings, primarily through `std::string` and C-style character arrays. The `std::string` class is part of the C++ Standard Library and offers a variety of functions that make string manipulation intuitive and straightforward. In contrast, character arrays are traditional and provide a low-level approach, giving programmers fine control but requiring more caution to avoid pitfalls like buffer overflows.
std::string str = "Hello, World!"; // Using std::string
char charArray[] = "Hello, World!"; // Using C-style string
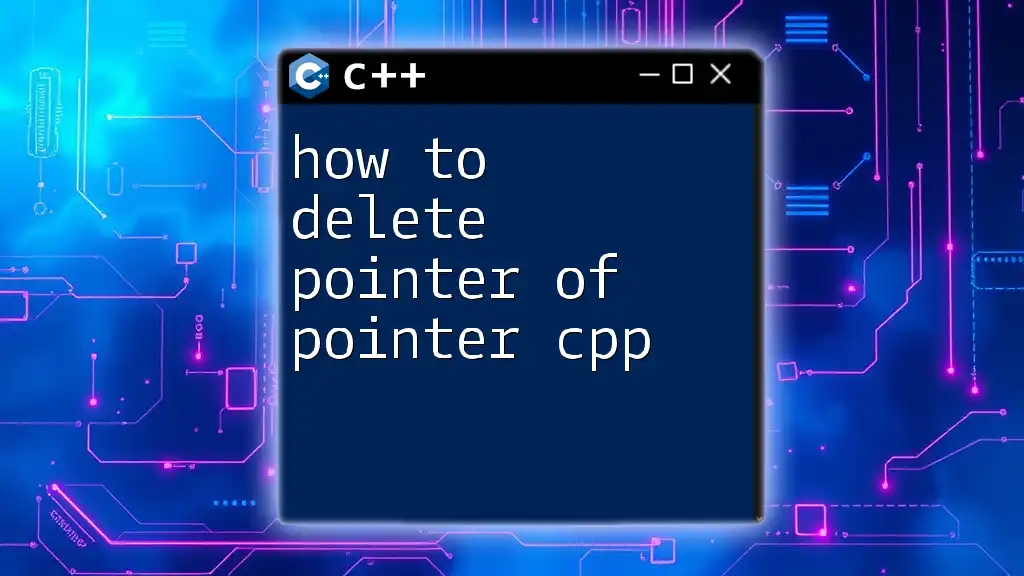
Methods to Delete Characters in C++
There are various techniques to delete characters in C++. Here are the most commonly used methods:
Using `std::string::erase()`
The `erase()` method is a powerful tool for removing specific characters or substrings from a `std::string`. This method can be particularly useful when you know the position of the character you want to delete.
Syntax:
str.erase(position, count);
Where position is the index of the character to delete, and count is the number of characters to remove.
Example:
std::string str = "Hello, World!";
str.erase(5, 1); // Deletes the comma
In this example, the method deletes the comma in the string by specifying its position and the number of characters (which is 1) to be removed.
Using `std::string::replace()`
Another method to delete a character is by using the `replace()` method. This method allows you to replace a specified section of the string. To delete a character, you can replace it with an empty string.
Syntax:
str.replace(position, count, "");
Example:
std::string str = "Hello, World!";
str.replace(5, 1, ""); // Replaces the comma with an empty string
This effectively removes the comma from the original string.
Using C-style Strings
When working with character arrays (C-style strings), deleting characters requires manual manipulation. Unlike `std::string`, which has built-in methods for deletion, character arrays require more hands-on methods.
Example:
char str[] = "Hello, World!";
str[5] = '\0'; // Sets the comma location to null
While this method removes the comma for display purposes, it does not physically resize the array, which is a limitation of C-style strings. Increased care must be taken to avoid memory issues.
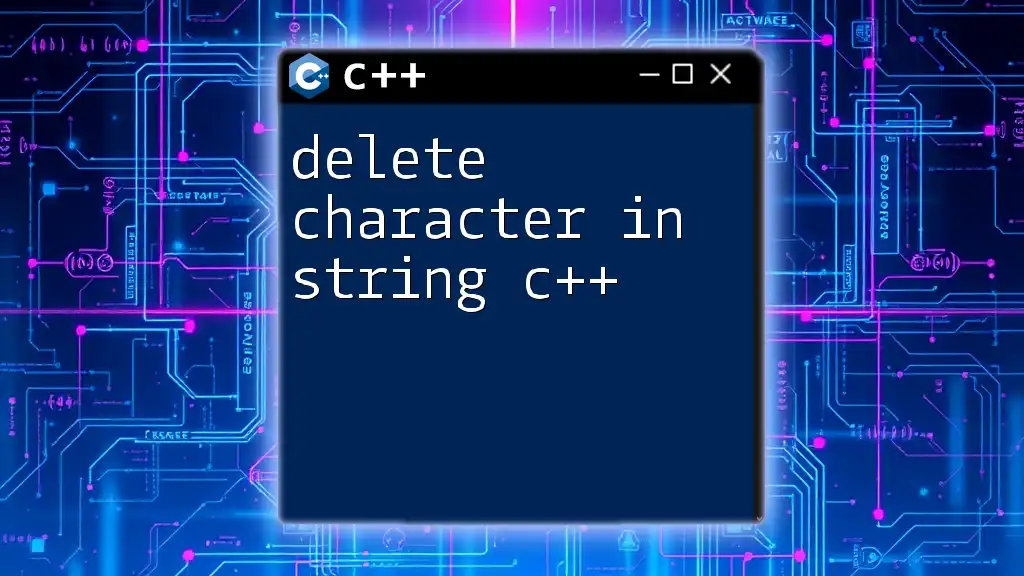
Tips for Deleting Characters in C++
Efficiency and safety are key when deleting characters in C++. Here are some tips to keep in mind:
- Plan for edge cases: Always anticipate scenarios such as deleting characters that do not exist.
- Memory safety: When using C-style strings, ensure you understand memory management concepts to prevent buffer overflows.
Using Loops for Multiple Deletions
If you need to delete multiple characters or target specific characters in a string, using a loop can provide a solution.
Example:
std::string str = "Hello, World!";
for (size_t i = 0; i < str.length(); ++i) {
if (str[i] == ',') {
str.erase(i, 1); // Deletes the comma
break; // Adjust index as necessary
}
}
In this snippet, a loop iterates through the string, checking each character. Upon finding a comma, it deletes it. The `break` statement ensures that the loop stops further unnecessary iterations.
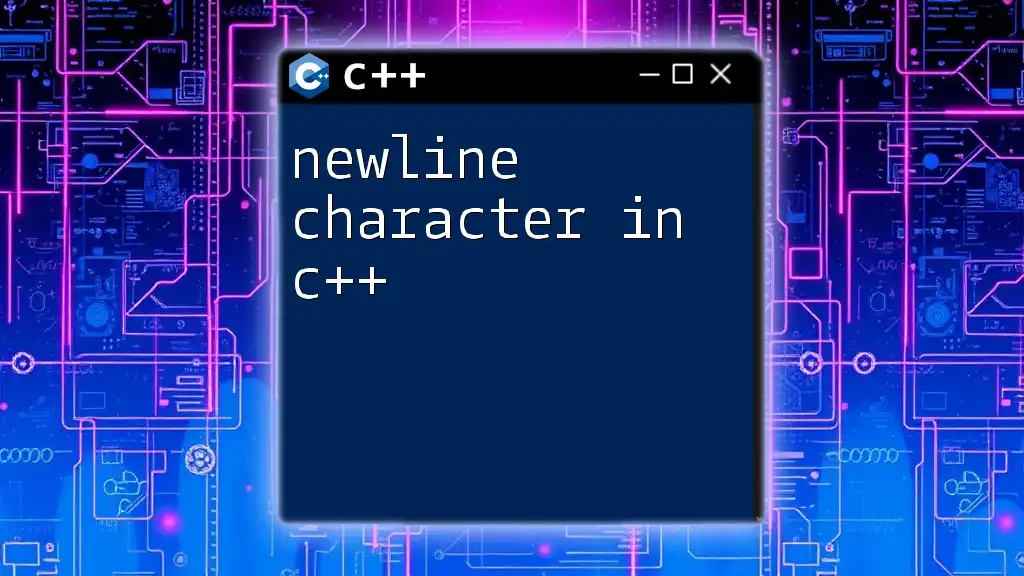
Common Errors and Troubleshooting
While working with string manipulations, errors can occur. Common mistakes include:
- Confusing the index positions: Remember that C++ uses zero-based indexing. Always check the value of the given index.
- Using incorrect parameters in the `erase()` or `replace()` methods, leading to runtime errors.
Validating your approach and leveraging debugging techniques can mitigate these issues.
Debugging Tips
Utilizing `std::cout` for debugging can help visualize the string before and after modifications. For example, you can print the string's content and its length before and after deletions to track changes and ensure operations are performed correctly.
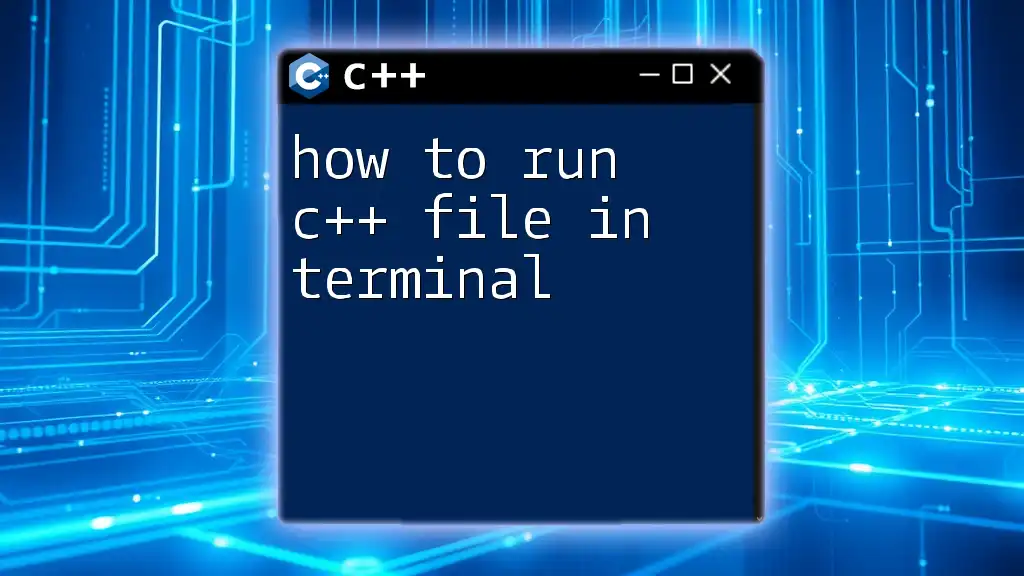
Conclusion
In conclusion, learning how to delete characters in C++ can significantly enhance your string manipulation skills and overall programming efficiency. By mastering functions such as `erase()`, `replace()`, and understanding character arrays, you can handle string data more adeptly. Practice is essential, and trying various examples will build your confidence and proficiency in using these techniques.
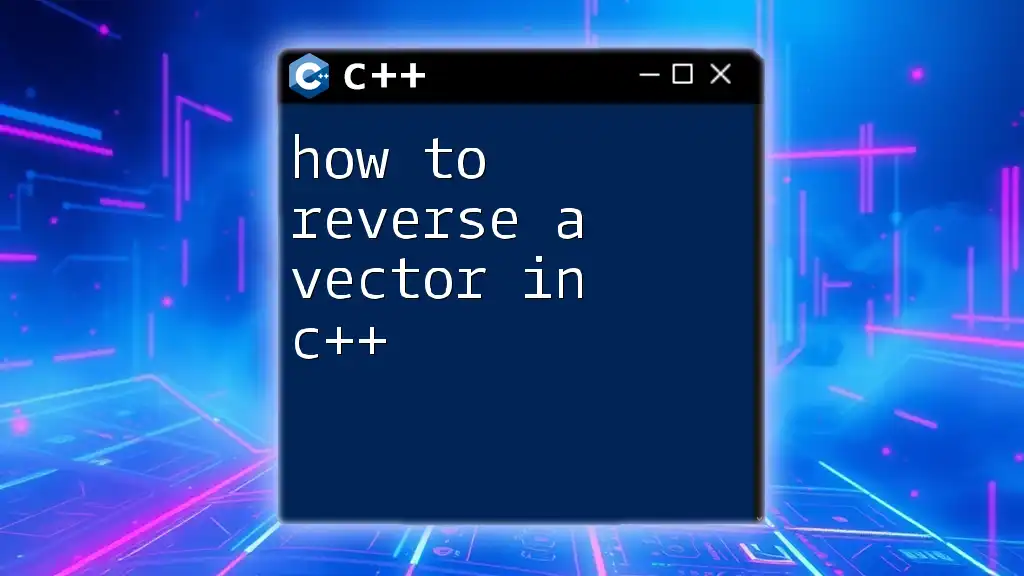
Additional Resources
For those eager to explore further, the official C++ documentation on `std::string` provides comprehensive insights. Additionally, consider engaging with online coding platforms that offer exercises focused on string manipulation, helping you master different approaches to character deletion in C++.