In C++, a `const pointer` (e.g., `int* const ptr`) means the pointer's address cannot change, while a `pointer const` (e.g., `const int* ptr`) means the value pointed to cannot be modified.
int value = 42;
int* const constPtr = &value; // const pointer: address can't change
const int* ptrConst = &value; // pointer const: value can't change
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. Pointers are essential for dynamic memory management, data structure manipulation, and efficient programming. Understanding how pointers work is crucial to mastering C++.
When you declare a pointer, you specify the type of data it points to. The basic syntax for declaring a pointer is:
int* ptr; // Pointer to an integer
This line does not allocate any memory for an integer itself but indicates that `ptr` will hold the address of an integer.
How Pointers Work
Pointers operate by referencing memory addresses directly. This allows for flexible memory management during runtime. When dereferencing a pointer, you access or modify the data located at the memory address it holds.
For instance:
int value = 5;
int* ptr = &value; // Store the address of 'value'
*ptr = 10; // Modify 'value' through the pointer
If we print `value` after this operation, it will show `10`.
Furthermore, pointer arithmetic allows you to navigate through memory addresses, which can lead to more efficient data handling in arrays or complex data structures.
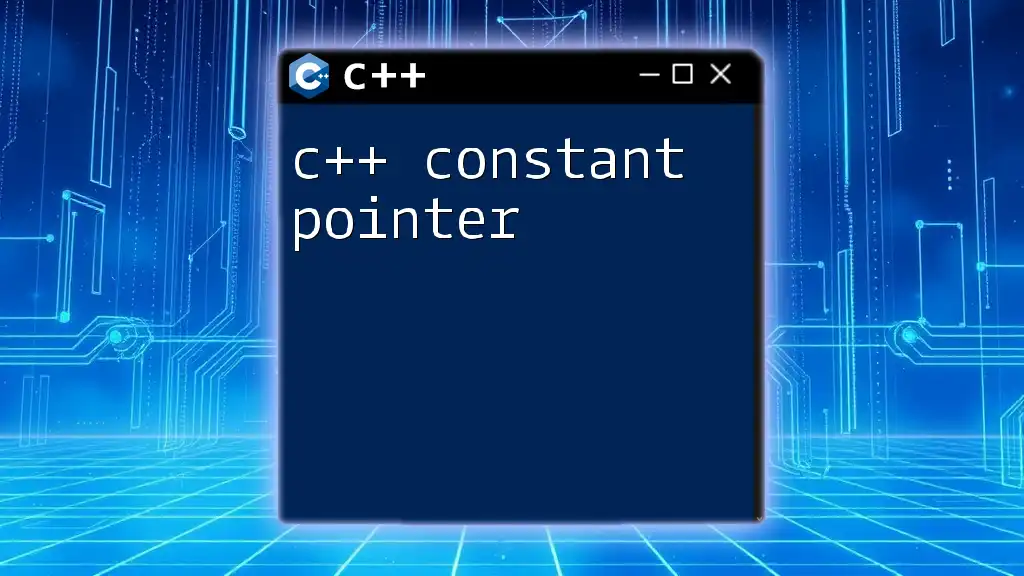
What is a Const Pointer?
Definition of Const Pointer
A const pointer is a pointer that cannot change the address it points to after its initialization. You declare a const pointer with the syntax:
int* const ptr = &value;
In this case, `ptr` can modify the value it points to but cannot point to a different memory address.
Advantages of Using Const Pointers
Const pointers provide a safeguard against pointing to unintended memory locations. By maintaining the address they hold, const pointers ensure that you don’t accidentally change where your pointer is pointing, thereby enhancing the safety and reliability of your code.
Code Example: Using a Const Pointer
void exampleFunc() {
int value = 42;
int* const ptr = &value; // Const pointer to 'value'
*ptr = 100; // Valid: Data can be modified
// ptr = nullptr; // Invalid: Cannot change address
}
In the example above, you can modify `value` through `ptr`, but an attempt to reassign `ptr` to a new address would lead to a compilation error. This reinforces the constancy of the pointer itself.
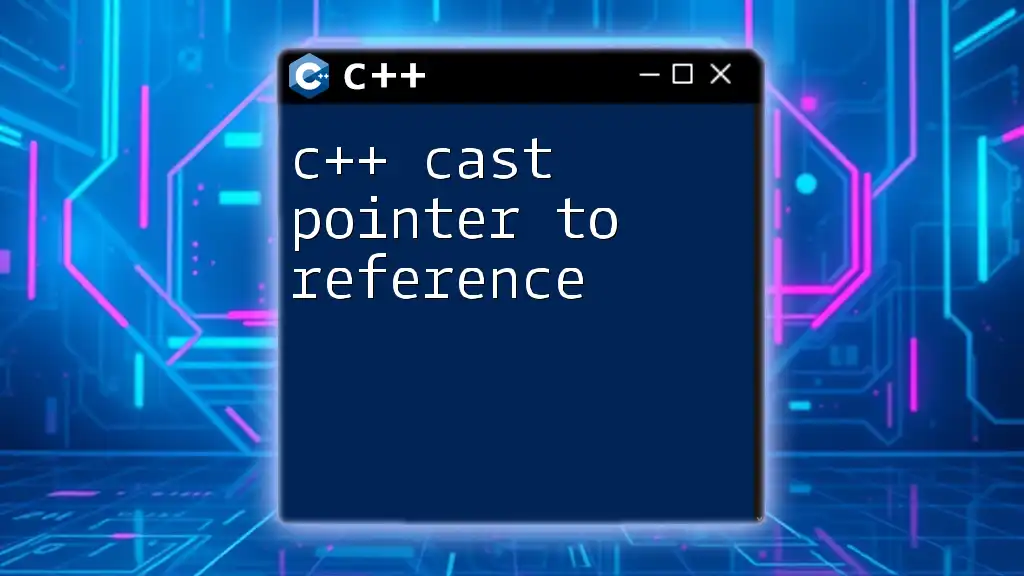
What is a Pointer Constant?
Definition of Pointer Constant
A pointer constant, on the other hand, is a pointer that points to a constant data type. You declare a pointer constant as follows:
const int* ptr;
Here, `ptr` can change its address but cannot be used to modify the value of the data it points to.
Advantages of Using Pointer Constants
Pointer constants allow for greater flexibility since you can point to different locations in memory while ensuring that the data they point to remains unchanged. This can be particularly useful in scenarios where you want to pass variables safely without modifying them.
Code Example: Using a Pointer Constant
void anotherExample() {
const int value = 100;
const int* ptr = &value; // Pointer constant to 'value'
// *ptr = 200; // Invalid: Cannot modify the value
ptr = nullptr; // Valid: Can change address
}
In this code snippet, while we cannot modify `value` through `ptr`, we can freely change what `ptr` is pointing to. This feature is beneficial when dealing with read-only values.
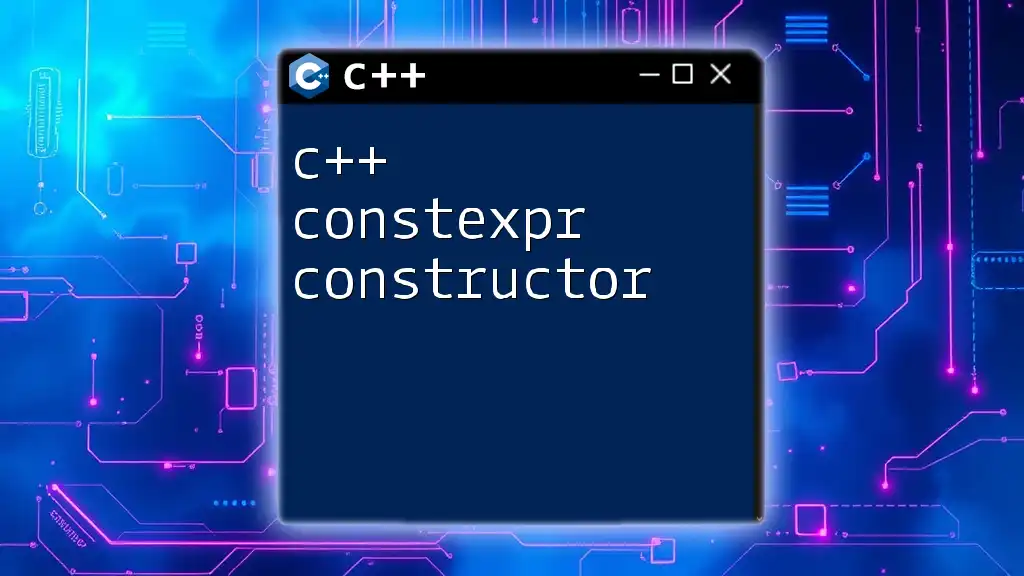
Key Differences: Const Pointer vs Pointer Const
Syntax Comparison
The difference in syntax is fundamental to understanding the distinction between c++ const pointer vs pointer const. A const pointer is declared with `* const` before the pointer name, while a pointer constant uses `const *` before the pointer name.
Behavioral Comparison
-
*Const Pointer (int const ptr)**:
- Modification of Data: Yes, you can modify the value pointed to.
- Modification of Pointer: No, you cannot change what the pointer points to.
-
*Pointer Constant (const int ptr)**:
- Modification of Data: No, you cannot modify the value pointed to.
- Modification of Pointer: Yes, you can change what the pointer points to.
Memory Management Considerations
The distinction between these two types of pointers can affect how you manage memory within your programs. Const pointers help ensure that critical memory locations remain unaffected by pointer reassignment, while pointer constants allow for more extensive data manipulation without altering the foundational data structure.
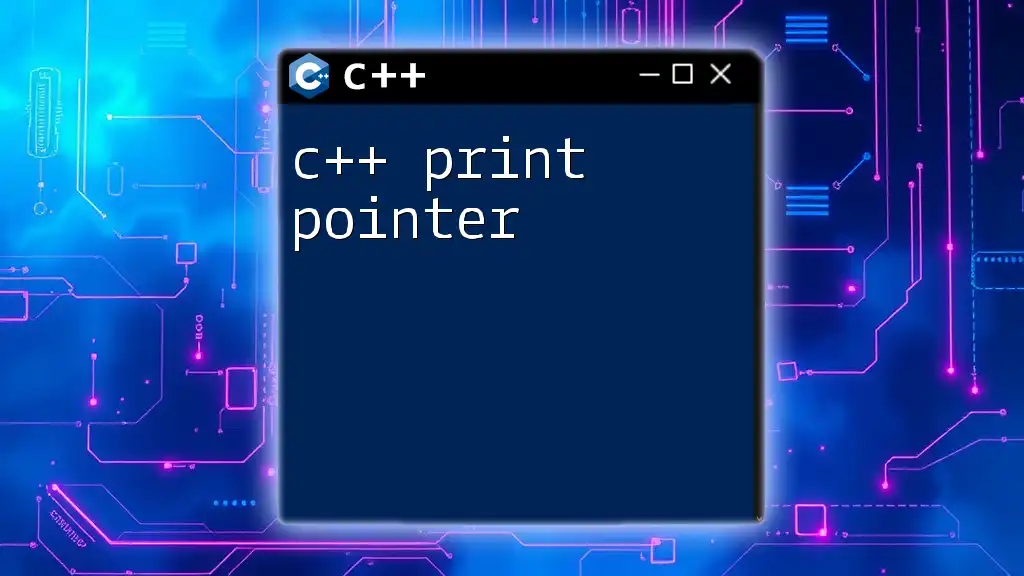
Use Cases and Practical Applications
When to Use Const Pointers
Const pointers are particularly useful when you want to ensure the lifetime of a pointer to an object without the risk of reallocating memory. They are prevalent in function parameters where you wish to modify input data without changing the pointer itself.
void processData(int* const data) {
*data += 10; // Adjust data safely
// data = nullptr; // Invalid
}
When to Use Pointer Constants
Pointer constants are beneficial when passing read-only values to functions or methods. This usage ensures that the values won't be altered while still allowing flexible pointer assignment.
void displayValue(const int* ptr) {
std::cout << *ptr << std::endl; // Safe read
// *ptr = 50; // Invalid: Cannot modify
}
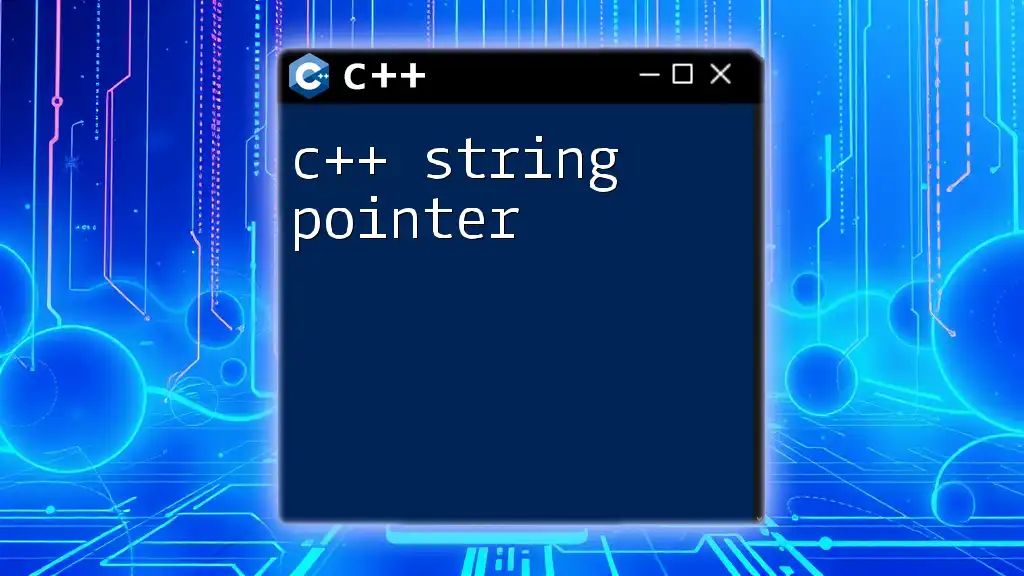
Common Pitfalls and Misunderstandings
Mistakes New Programmers Make
Many new programmers confuse const pointers and pointer constants, leading to errors in their code. One common mistake is attempting to modify the pointer address of a const pointer, which will yield compile-time errors.
Debugging Tips
If you encounter issues related to pointer manipulations, always ensure that you check the const declarations in your code. Using compiler warnings effectively can also aid significantly in identifying potential misuses of pointers.
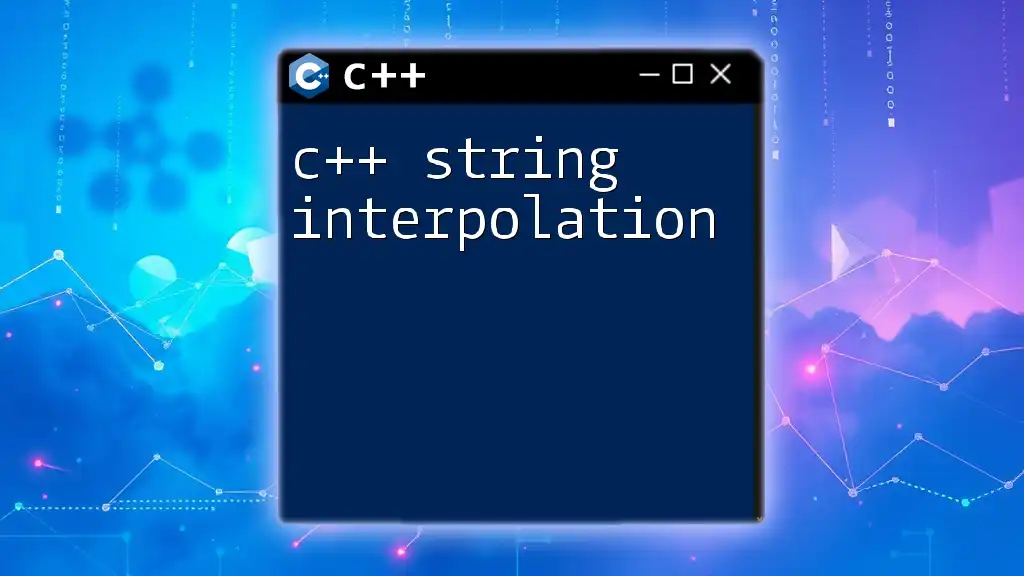
Conclusion
Understanding the differences between c++ const pointer vs pointer const is essential for robust C++ programming. Each type serves distinct purposes that enhance safety and flexibility in your code. Adopting good practices with these tools will lead to more reliable and maintainable code.
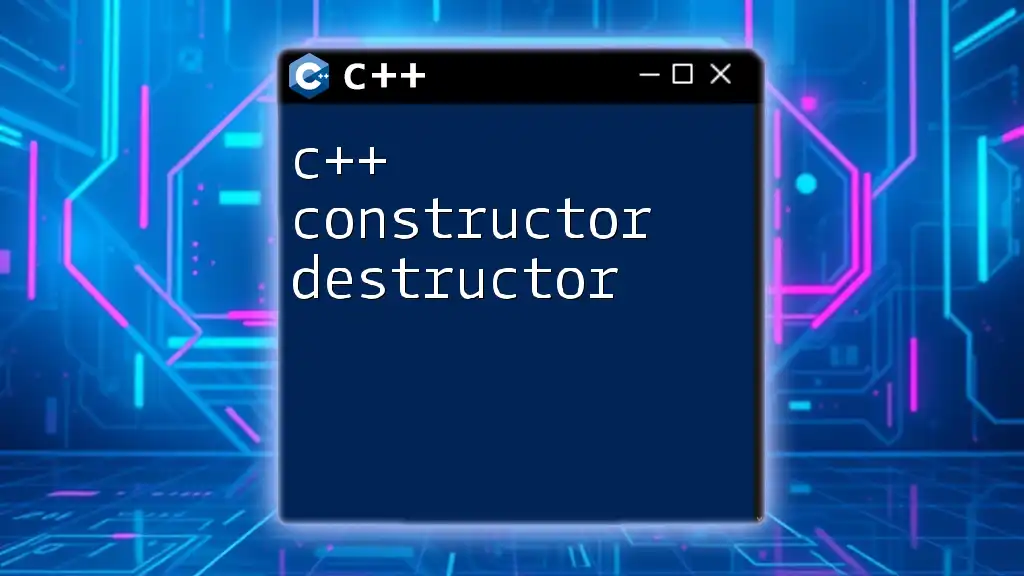
Further Reading and Resources
Exploring additional materials on C++ pointers can vastly improve your programming skills. Look for books and online courses that dive deeper into pointers, smart pointers, and memory management.
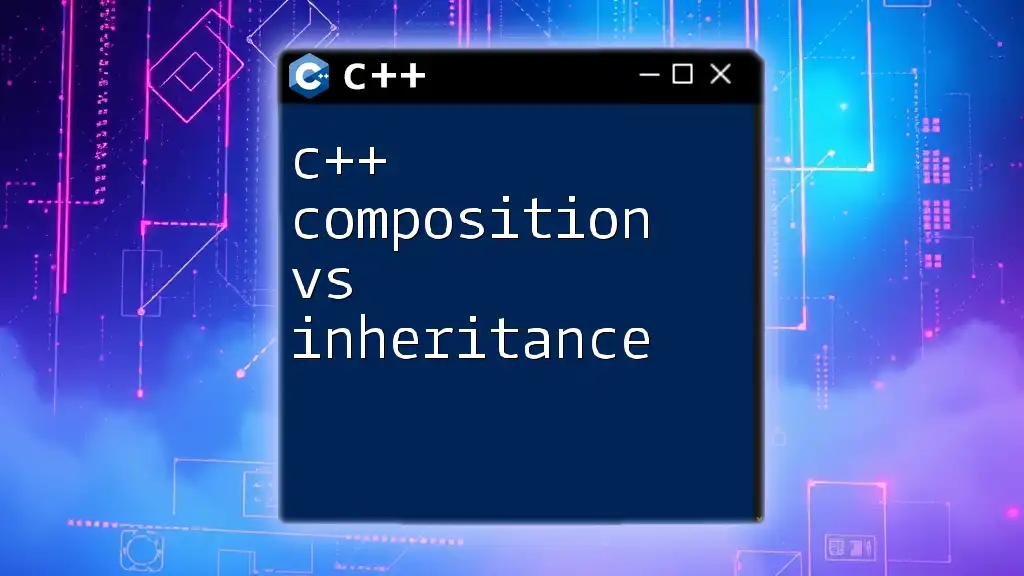
Call to Action
Begin practicing with const pointers and pointer constants in your own projects. For more tutorials and lessons on C++, visit our website for an extensive range of resources committed to enhancing your programming journey.