A C++ constant member function is a member function that promises not to modify the object on which it is called, ensuring the integrity of the object’s state.
Here's a code snippet demonstrating a constant member function:
#include <iostream>
class Example {
public:
int value;
Example(int v) : value(v) {}
// Constant member function
int getValue() const {
return value;
}
};
int main() {
Example obj(10);
std::cout << "Value: " << obj.getValue() << std::endl;
return 0;
}
Understanding the Basics of Constant Member Functions
Definition of Constant Member Functions in C++
A c++ constant member function is a member function that is declared with the `const` keyword at the end of its declaration. This indicates that the function will not modify any member variables of the class it belongs to. The primary purpose of constant member functions is to provide a guarantee that the entire object's state remains unchanged when the method is invoked.
Example of a Simple Class with a Constant Member Function
Consider a scenario where you have a class representing a `Vector`:
class Vector {
private:
int x, y;
public:
Vector(int x_val, int y_val) : x(x_val), y(y_val) {}
int getX() const {
return x; // Cannot modify x or y
}
int getY() const {
return y; // Cannot modify x or y
}
};
In this example, `getX()` and `getY()` are constant member functions. They provide read-only access to the private variables `x` and `y`.
The Role of the Keyword `const`
The keyword `const` is crucial as it defines the method's immutability regarding the class's internal state. When you declare a member function as constant, you tell both the compiler and other programmers that this method will not change any member variables.
Difference Between Constant and Regular Member Functions
A regular member function can modify the object's state:
void modifyX(int newX) {
x = newX; // Allowed
}
In contrast, in a constant member function, attempting to modify `x` or `y` would yield a compilation error. This ensures that the object's integrity is preserved.
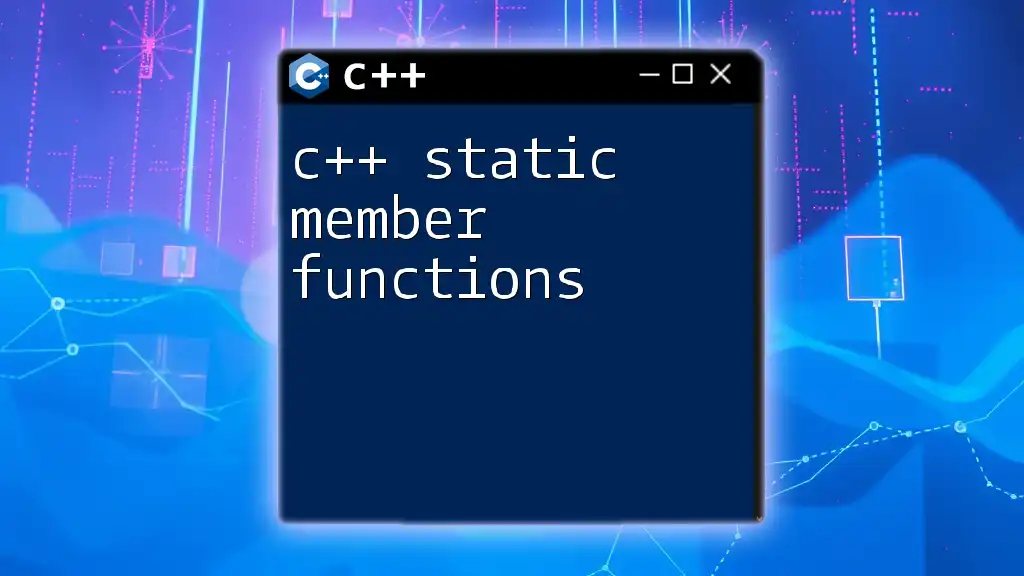
Why Use Constant Member Functions?
Benefits of Constant Member Functions
Constant member functions provide several advantages:
- Preventing Unintended Modifications: They ensure that you do not accidentally change an object's state when you intend to read it.
- Improved Code Readability and Maintenance: Using constant member functions helps clarify your code's intent. Other developers can easily understand which operations modify state and which do not.
- Enabling the Use of Constant Objects: You can invoke constant member functions on constant objects. This is vital for many design patterns where you want to guarantee that the object's state remains unchanged.
Use Cases for Constant Member Functions
Constant member functions are particularly useful in several situations:
- Accessor Methods: When you want to provide read-only access to class properties.
- Operator Overloading: Constant member functions often play a critical role in operations that should not affect object state. For instance, when overloading operators like `==`, the operator should typically be a constant member function.
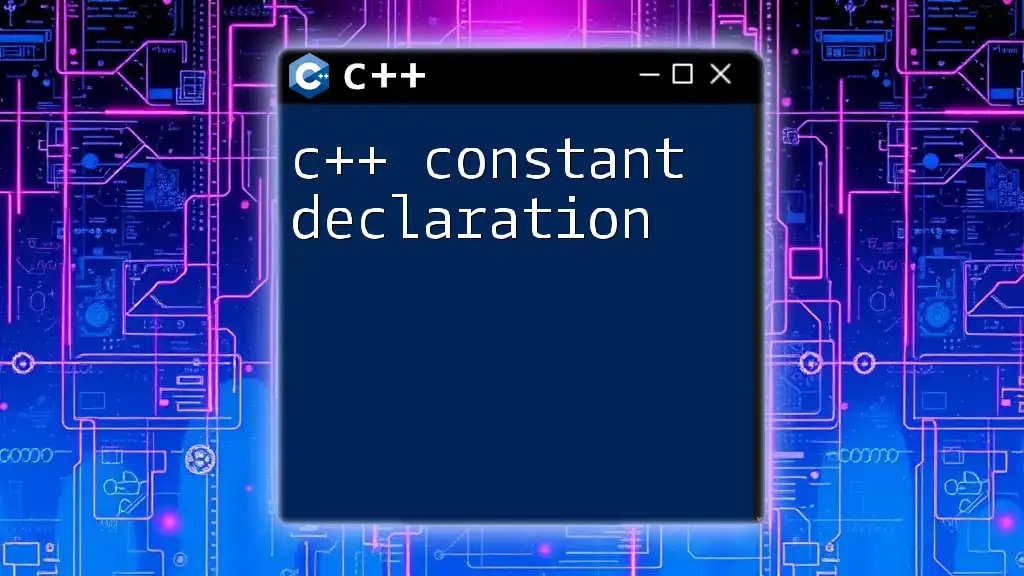
How to Implement Constant Member Functions in C++
Syntax and Structure
The syntax for declaring a constant member function is straightforward. Simply append `const` to the end of the member function declaration, as shown here:
class MyClass {
public:
void display() const; // Constant member function declaration
};
Example of Constant Member Function Implementation
Let’s take a deeper look at the use of c++ constant member functions through a detailed example. Here we have a `Circle` class that calculates the area based on its radius:
class Circle {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getArea() const { // Constant member function
return 3.14159 * radius * radius; // Calculate area without modifying radius
}
};
In this example:
- The `getArea()` function is marked as `const`, ensuring that it does not alter the value of `radius`.
- When we create an instance of `Circle` and call `getArea()`, it guarantees that `radius` remains intact, providing a reliable output.
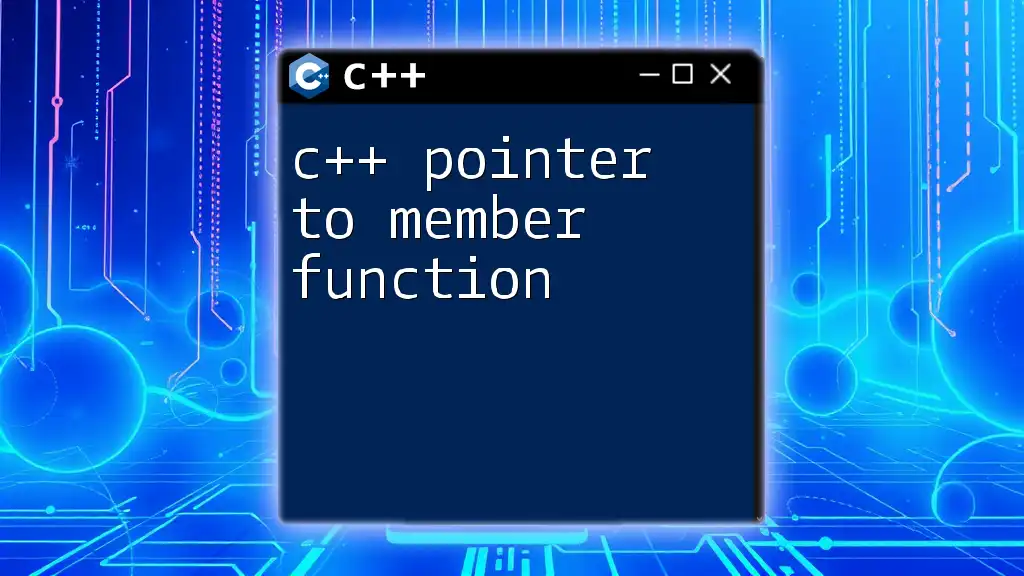
Common Mistakes and Pitfalls
Misunderstanding Const Contexts
One of the common misconceptions about constant member functions is believing that they can modify class members. In reality, any attempt to change a member variable inside a constant member function will lead to a compilation error.
Example of a Mistake:
void increaseRadius() const {
radius++; // Error: cannot modify radius in a const function
}
Best Practices for Constant Member Functions
To maximize the utility of constant member functions, consider the following practices:
- Always declare methods as constant when they only need to read member variables.
- Use constant member functions in conjunction with `const` objects.
- Document the behavior of constant member functions clearly to inform users of your class.
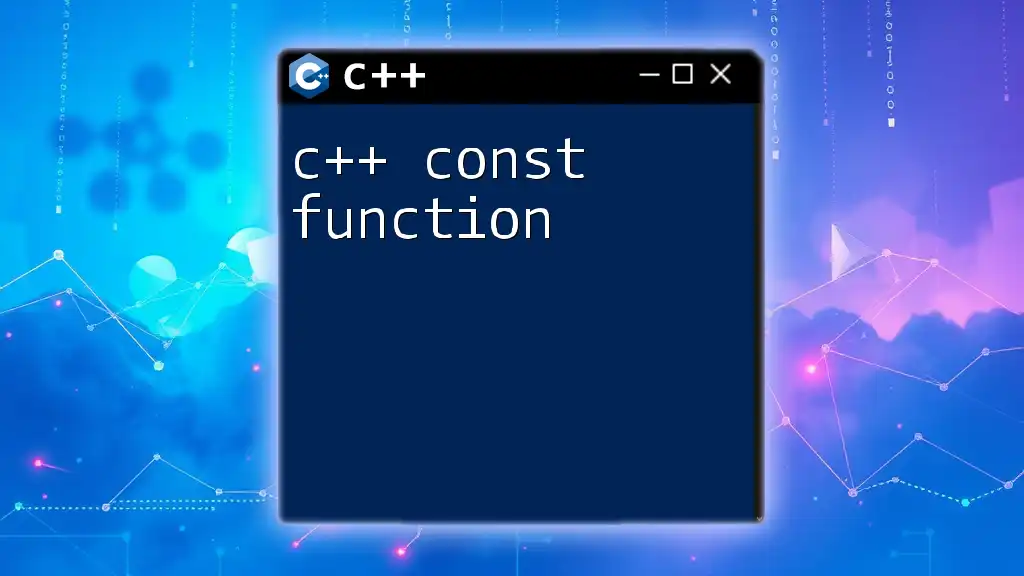
Conclusion
In summary, understanding and using c++ constant member functions is essential for developers aiming to write effective, maintainable, and reliable C++ code. By taking advantage of the immutability that `const` guarantees, you ensure the integrity of your objects and improve the overall quality of your software design. Incorporating these practices into your coding habits will lead to cleaner, more understandable programs.
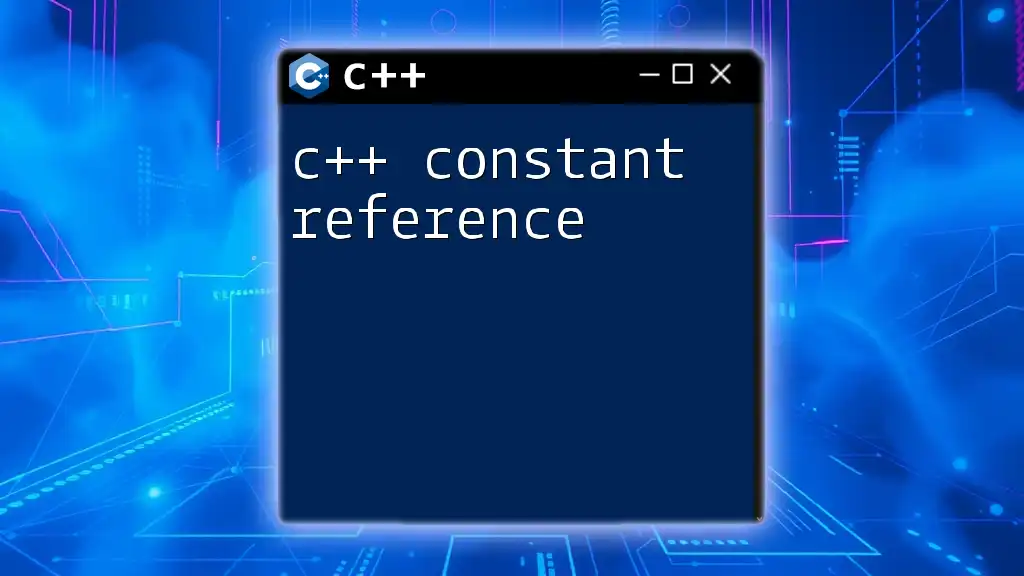
Additional Resources
For those interested in deepening their knowledge of constant member functions and C++ programming:
- Look for tutorials, books, and online platforms focused on advanced C++ topics.
- Participate in community forums or discussion groups where C++ developers share insights and best practices regarding constant member functions and other fundamental concepts.
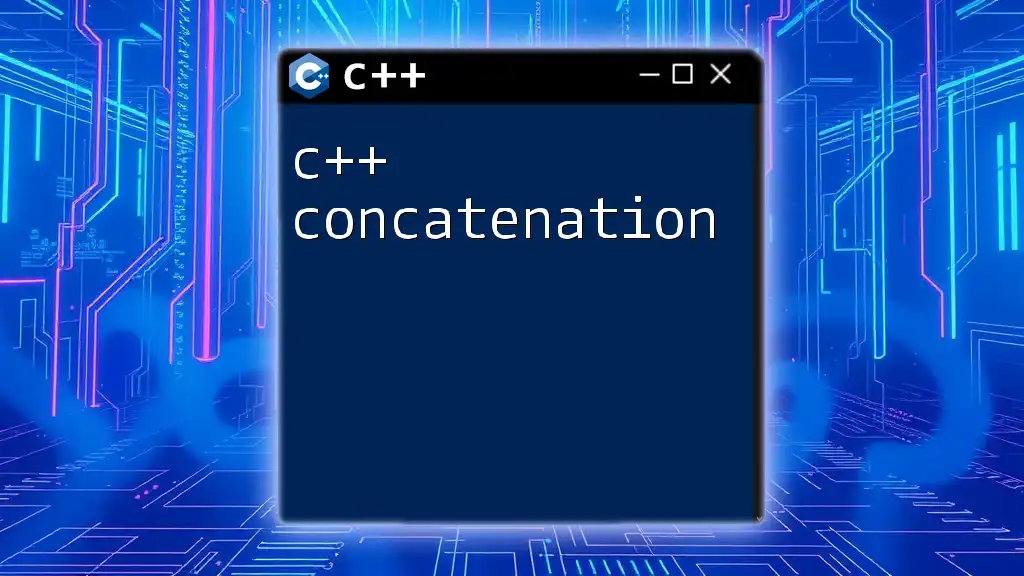
Call to Action
Feel free to share your experiences with constant member functions in the comments section below. If you found this article helpful, consider subscribing for more insightful posts on C++ programming techniques and concepts.