In C++, a pointer to an array of pointers allows you to create a dynamic structure where an array can hold multiple pointers, each pointing to potentially different data types or memory locations.
Here's a simple example demonstrating how to declare and use a pointer to an array of pointers:
#include <iostream>
int main() {
int* arr[3]; // Array of 3 integer pointers
int a = 10, b = 20, c = 30;
arr[0] = &a; // Pointing to a
arr[1] = &b; // Pointing to b
arr[2] = &c; // Pointing to c
for (int i = 0; i < 3; ++i) {
std::cout << *arr[i] << " "; // Dereferencing pointers to print values
}
return 0;
}
Understanding Pointers in C++
What are Pointers?
Pointers are a fundamental concept in C++, representing variables that store the memory address of another variable. They provide crucial control over memory management and allow for efficient handling of structured data. The syntax for declaring a pointer involves placing an asterisk (*) before the pointer's name, which denotes that it points to a data type.
int* ptr; // ptr is a pointer to an integer
How Pointers Work
Pointers allow you to access and manipulate the data directly in memory. Every variable in C++ is stored at a unique memory address, and a pointer helps you refer to that address. By dereferencing a pointer (using the * operator), you can access or modify the value it points to.
Pointer arithmetic enables you to move through memory addresses based on the type of data it points to, making it easier to traverse arrays.
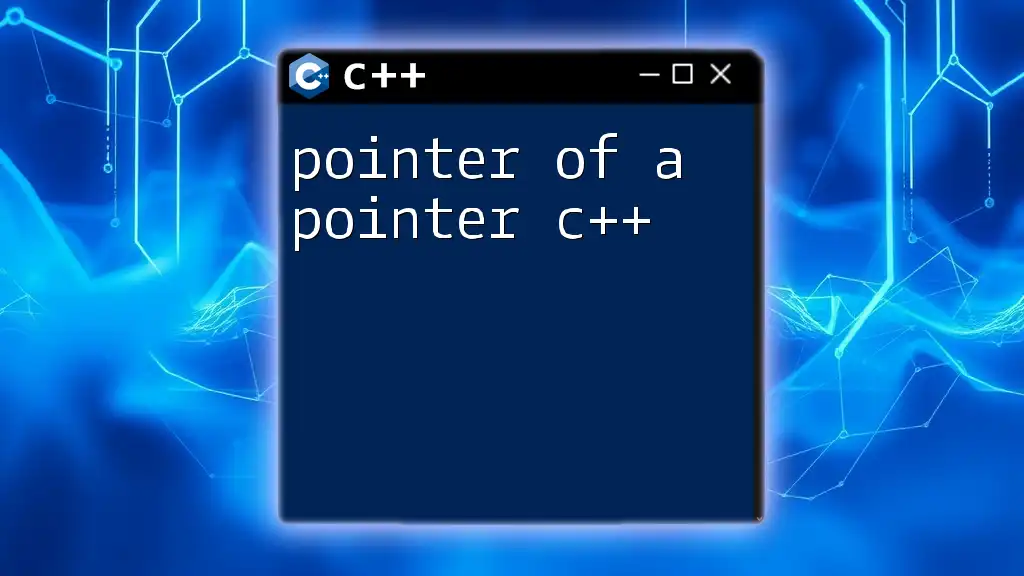
Introduction to Arrays in C++
What is an Array?
An array is a collection of elements of the same data type, stored contiguously in memory. Arrays have a fixed size, determined at compile-time, making them useful for storing a predetermined number of items.
int arr[5]; // An array of 5 integers
Using Arrays with Pointers
There is a close relationship between arrays and pointers in C++. Arrays can be manipulated using pointers, and you can access array elements through pointer notation. For instance, if you define an array `arr`, you can access its elements either through the array index or by using pointers.
arr[0] = 10; // Accessing using array index
int* p = arr; // p points to the first element of the array
*p = 20; // Changing the first element through the pointer
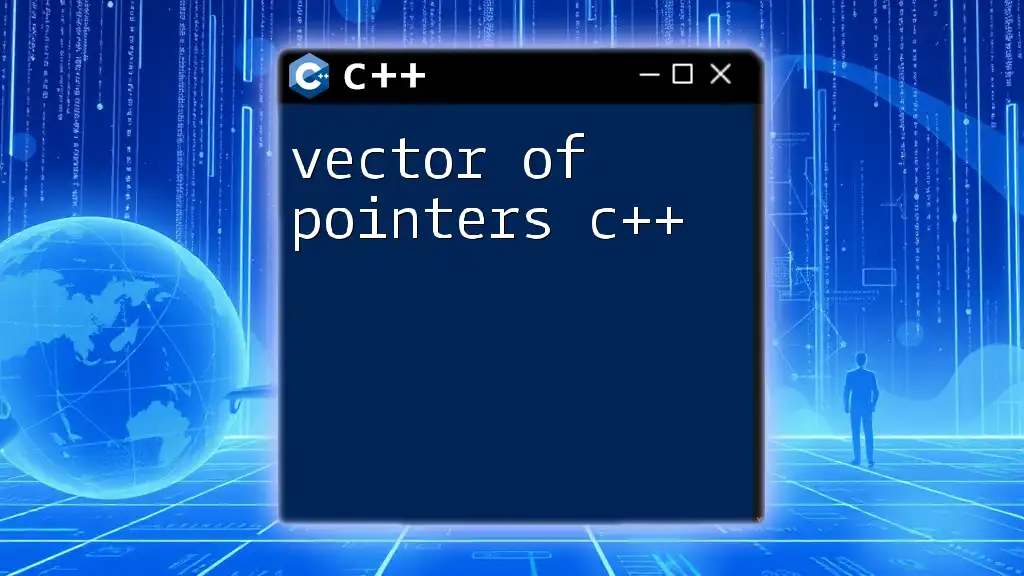
The Concept of an Array of Pointers
What is an Array of Pointers?
An array of pointers is a collection of pointers, where each pointer can point to a distinct piece of data, often of the same type. This structure is particularly beneficial for managing data structures like lists, strings, or multi-dimensional arrays.
Visualizing an Array of Pointers
When visualizing an array of pointers, think of it as an array where each element holds the address of another variable. This allows for more dynamic and flexible data handling.
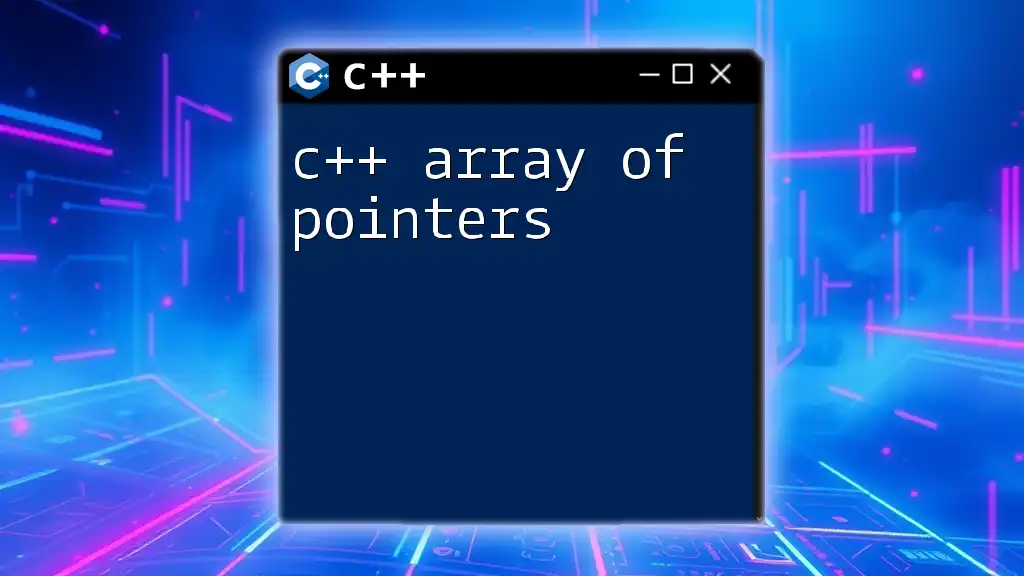
Pointer to an Array of Pointers
Defining a Pointer to an Array of Pointers
A pointer to an array of pointers is simply a pointer that points to the first element of an array containing pointers. The syntax may seem complex, but it follows a straightforward pattern.
int* ptrArray[3]; // Declaration of an array of 3 pointers to int
int** ptrToArray = ptrArray; // ptrToArray is now pointing to the array of pointers
Dynamic Allocation of Pointer Arrays
C++ allows dynamic memory allocation, enabling you to create an array of pointers at runtime. This is done using the `new` keyword, which allocates the required memory.
int n = 5;
int** ptrToArray = new int*[n]; // Allocating an array of n integer pointers
Accessing Elements and Memory Management
Accessing elements within a pointer-to-array scenario is similar to working with regular arrays. You first dereference the pointer to reach the array, then use the desired index.
*ptrToArray[0] = 10; // Assigning value to the first element pointed by the first pointer
It's crucial to manage memory properly by releasing any dynamically allocated memory to avoid memory leaks:
for (int i = 0; i < n; ++i) {
delete ptrToArray[i]; // Freeing each dynamically allocated integer
}
delete[] ptrToArray; // Freeing the array of pointers
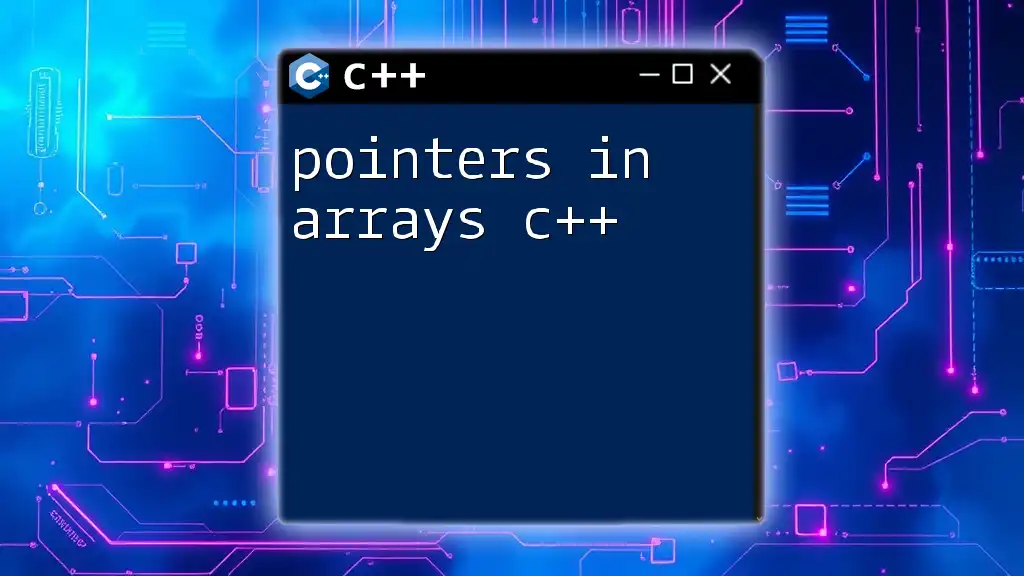
Practical Examples
Example 1: Simple Array of Integer Pointers
Creating an array of pointers to integers and manipulating the data can easily be achieved like this:
int* arr[3]; // Declaring an array of 3 pointers to integers
for (int i = 0; i < 3; ++i) {
arr[i] = new int(i + 1); // Dynamically allocate integers
}
This code snippet allocates memory for three integers and their addresses are stored in `arr`.
Example 2: Pointer to Array of String Pointers
You can also apply the same concept with strings, allowing you to create an array of string pointers efficiently.
std::string* strArray[3]; // Array of three string pointers
strArray[0] = new std::string("Hello");
strArray[1] = new std::string("World");
strArray[2] = new std::string("!");
Once again, don't forget to clean up the allocated memory afterward.
Example 3: Use Case in 2D Arrays
The flexibility of using a pointer to an array of pointers comes into play with 2D arrays. You can create a dynamic 2D array like this:
int rows = 3, columns = 4;
int** array2D = new int*[rows];
for (int i = 0; i < rows; ++i) {
array2D[i] = new int[columns]; // Allocating memory for each row
}
This setup allows you to access `array2D[i][j]` to get to specific elements in a manageable way.
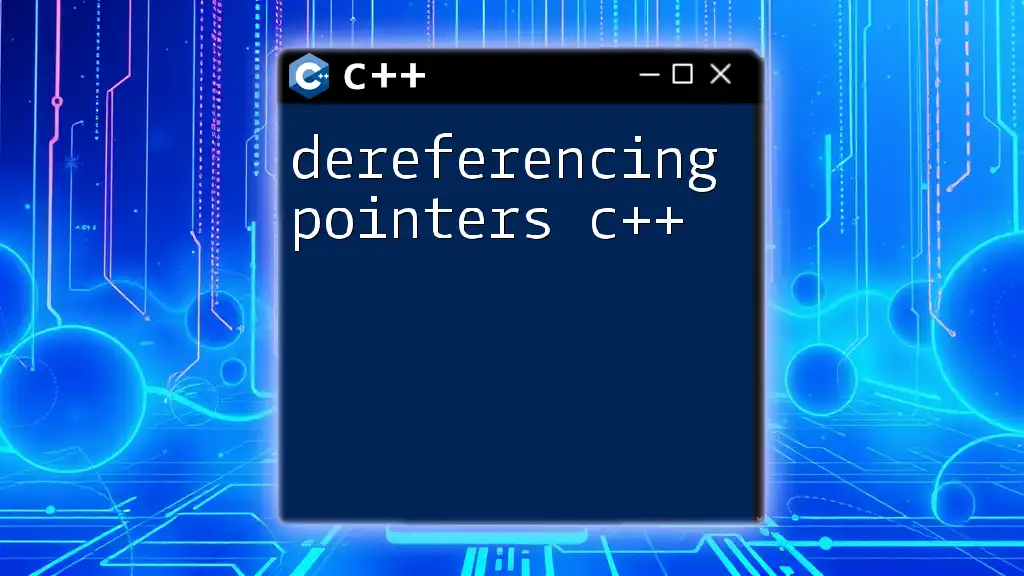
Tips and Best Practices
Important Considerations
While working with pointers, initialization is key. Ensure that pointers are initialized before use to avoid accessing garbage values or encountering segmentation faults.
Common Errors and How to Avoid Them
Be mindful of dangling pointers where pointers point to memory that has been freed. Out-of-bounds access can lead to undefined behavior, so check array bounds when iterating through pointers.
When to Use Pointer-to-Array of Pointers
Use a pointer to an array of pointers when you need a dynamic collection of data structures, especially when the sizes of the arrays may change at runtime.
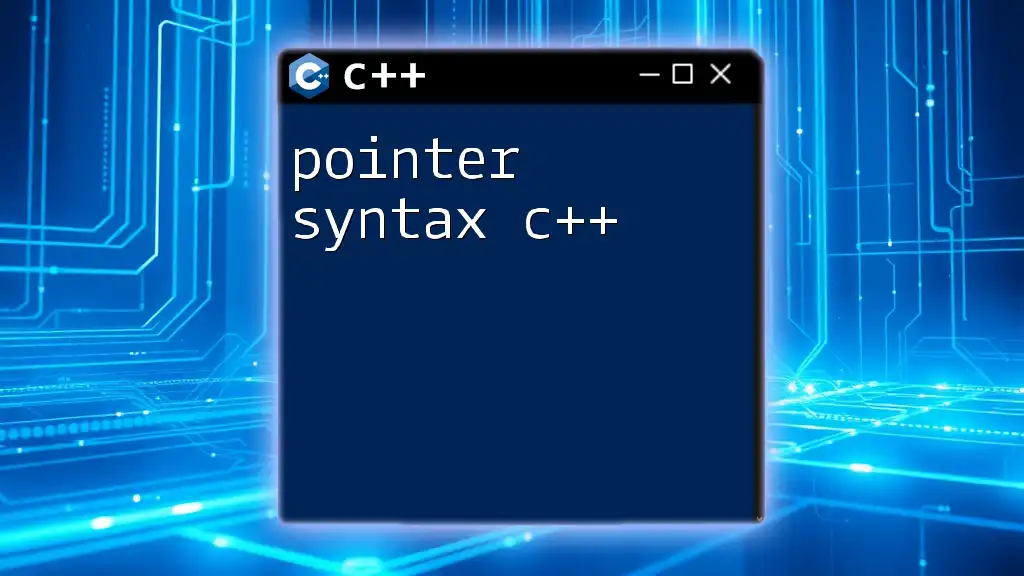
Conclusion
Recap of Key Concepts
Pointers are a powerful feature in C++, offering flexibility and control over memory management. Understanding the concept of a pointer to an array of pointers can enhance your likelihood of creating effective data structures in your applications.
Further Resources
For those looking to deepen their understanding of pointers and arrays in C++, consider exploring reputable online courses or tutorials that emphasize hands-on practice.
Call to Action
Start implementing the examples provided and experiment with creating your own pointer-to-array of pointers scenarios to solidify your understanding and mastery of C++.