In C++, the `sizeof` operator can be used to determine the number of bytes occupied by an array, and dividing this value by the size of one element allows you to find the total number of elements in the array.
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
size_t size = sizeof(arr) / sizeof(arr[0]); // Number of elements in the array
std::cout << "Number of elements in the array: " << size << std::endl;
return 0;
}
Understanding the `sizeof` Operator
What is `sizeof`?
The `sizeof` operator in C++ is a built-in operator that allows you to determine the size in bytes of a data type or variable. Importantly, the size returned by `sizeof` is evaluated at compile time, which can improve performance in certain situations.
It's crucial to know that `sizeof` can be used in two ways:
-
To get the size of a variable:
int a; std::cout << "Size of int: " << sizeof(a) << " bytes." << std::endl;
-
To get the size of a specific type:
std::cout << "Size of int: " << sizeof(int) << " bytes." << std::endl;
How `sizeof` Works with Data Types
Understanding how `sizeof` functions with various data types is essential, especially when dealing with arrays. For instance, common primitive types generally have consistent sizes across platforms:
- `char` typically has a size of 1 byte.
- `int` usually has a size of 4 bytes but may vary based on the platform.
- `float` generally occupies 4 bytes as well.
To illustrate, here’s how to check the size of these data types:
std::cout << "Size of char: " << sizeof(char) << " bytes." << std::endl;
std::cout << "Size of float: " << sizeof(float) << " bytes." << std::endl;
std::cout << "Size of double: " << sizeof(double) << " bytes." << std::endl;
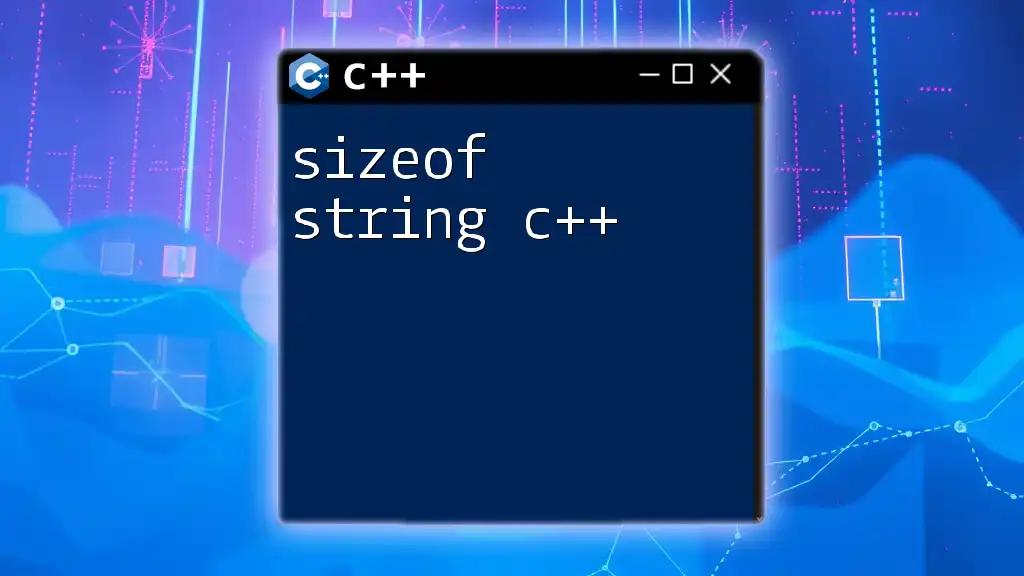
Using `sizeof` with Arrays
Definition of Arrays in C++
In C++, an array is a collection of elements (of the same type) stored in contiguous memory locations. When you declare an array, you essentially create a pointer to the first element of the array, but it's important not to confuse the pointer with the entire array itself.
Syntax for declaring an array:
int myArray[10]; // An array of 10 integers
Calculating Size of an Array
When working with arrays, the `sizeof` operator can provide the total size in bytes of the entire array:
int myArray[10];
std::cout << "Size of array: " << sizeof(myArray) << " bytes." << std::endl; // Outputs 40 bytes for an int array
The output here tells us the total size of the array in memory, which is 10 (elements) * 4 (bytes each) = 40 bytes.
Understanding Array Element Size
To comprehend the size of each individual element within an array, you can utilize `sizeof` on a specific element:
std::cout << "Size of one element: " << sizeof(myArray[0]) << " bytes." << std::endl; // Typically 4 bytes for int
This demonstrates how you can extract the size of the first element, which directly indicates the size for all elements in the array, assuming they are of the same type.

Common Misconceptions About `sizeof` and Arrays
`sizeof` on Pointer Types
A common misconception arises when dealing with arrays and pointers. In C++, arrays decay into pointers when passed to functions, leading to confusion regarding `sizeof`.
For example, consider the following code:
int* ptr = myArray;
std::cout << "Size of pointer: " << sizeof(ptr) << " bytes." << std::endl; // Typically 4 or 8 bytes depending on architecture
Here, `sizeof(ptr)` returns the size of the pointer, not the size of the entire array. This is a critical distinction that can lead to incorrect assumptions and bugs.
The Risk of Using `sizeof` on Function Parameters
When arrays are passed to functions, they do not carry their size information. Instead, they decay to pointers. This commonly leads to confusion:
void printArraySize(int arr[]) {
std::cout << "Size of passed array: " << sizeof(arr) << " bytes." << std::endl; // Will output size of pointer
}
In this example, `sizeof(arr)` will not return the total size of the array but instead the size of the pointer. This can cause problems when you attempt to determine how many elements are in the array.

Calculating Number of Elements in an Array
Formula for Element Count
To safely determine the number of elements in an array, you can use the formula:
int numberOfElements = sizeof(myArray) / sizeof(myArray[0]);
std::cout << "Number of elements: " << numberOfElements << std::endl;
This calculation divides the total size of the array by the size of a single element, yielding the correct count of elements in the array.
Practical Example
Putting it all together in a single code snippet demonstrates the calculation of the number of elements in an array:
int myArray[5] = {1, 2, 3, 4, 5};
int numberOfElements = sizeof(myArray) / sizeof(myArray[0]);
std::cout << "Number of elements: " << numberOfElements << std::endl; // Outputs 5
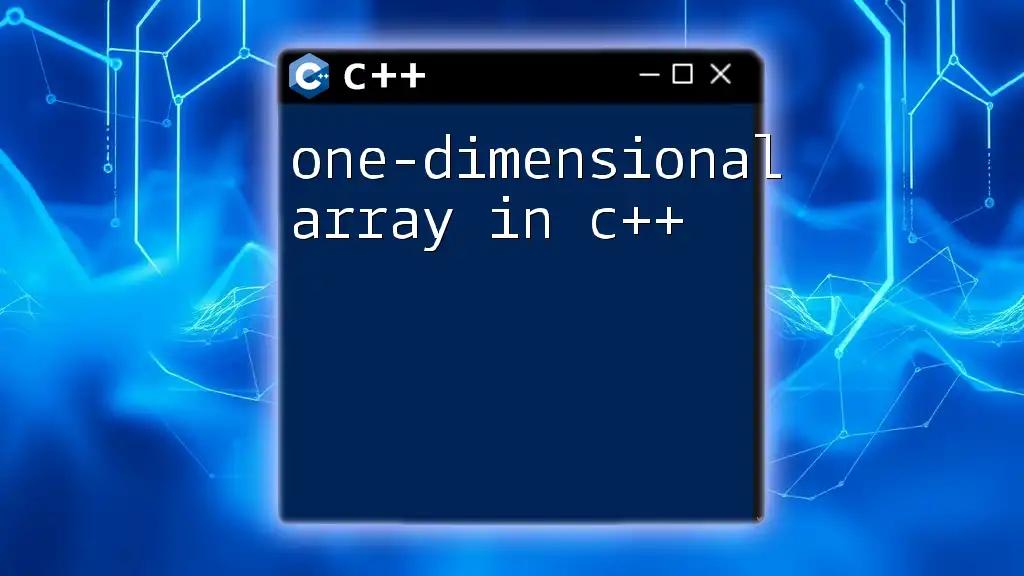
Best Practices When Using `sizeof` with Arrays
When to Use `sizeof`
Using `sizeof` is beneficial in scenarios such as:
- Memory calculations: Knowing how much space an array occupies helps with memory management and optimization.
- Debugging: Ensuring arrays contain the expected number of elements.
When Not to Use `sizeof`
However, there are pitfalls to avoid:
-
Function parameters: As previously discussed, never use `sizeof` on arrays passed into functions; use an additional parameter to communicate the number of elements instead.
-
Pointer misinterpretation: Always remember that `sizeof` on a pointer type returns the size of the pointer itself and not what it points to.

Conclusion
Understanding how to correctly use the `sizeof` operator with arrays in C++ is vital for any programmer. Misapplying `sizeof` can lead to significant bugs and inefficiencies in your code. By grasping these concepts and practicing with code examples, you'll enhance your proficiency with array manipulation and memory management.
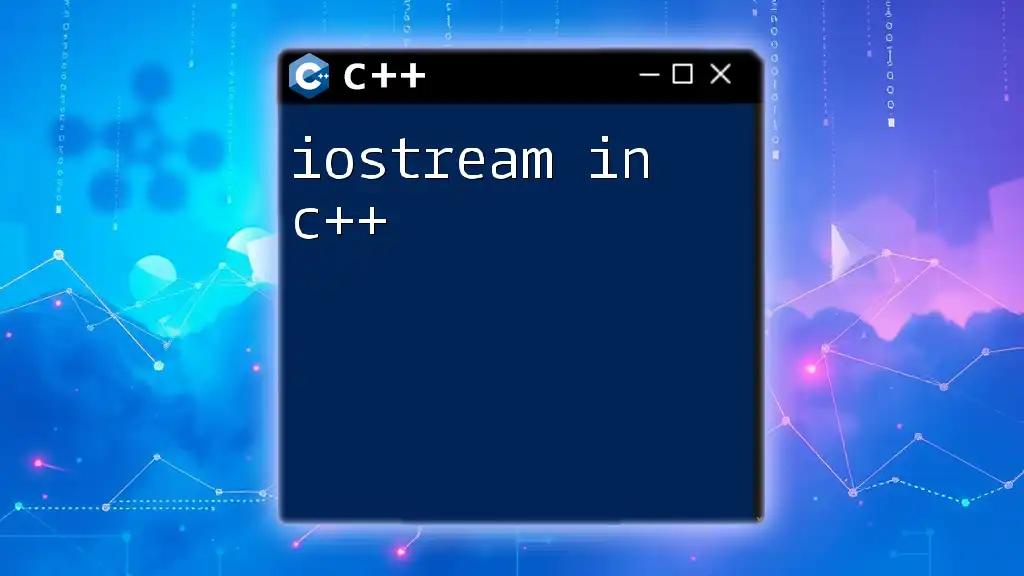
Additional Resources
For further reading and deeper understanding, consider exploring the official C++ documentation, curated tutorials, or educational courses focused on arrays and memory operations. Engaging with community forums can also provide practical insights and aid in developing a solid grasp of these fundamental concepts.