An array of arrays in C++ is essentially a two-dimensional array that allows you to store data in a grid-like format, where each element can be accessed using two indices.
Here's a code snippet demonstrating a simple array of arrays:
#include <iostream>
using namespace std;
int main() {
// Declare a 2D array (array of arrays)
int arr[2][3] = {
{1, 2, 3},
{4, 5, 6}
};
// Access and print the elements
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of elements that are stored in a contiguous block of memory. Each element in an array can be accessed using an index. C++ supports several types of arrays including single-dimensional and multidimensional arrays.
Key Characteristics of Arrays:
- Contiguous Memory Allocation: All elements of an array are stored next to each other in memory.
- Data Type Uniformity: Every element in an array must be of the same data type, providing consistency and type safety.
Syntax of Arrays in C++
Declaring and initializing an array in C++ is straightforward. Here’s an example of a single-dimensional array declaration:
int arr[5] = {1, 2, 3, 4, 5};
In this example, `arr` is declared as an array of integers that can hold five values. Note that the size of the array must be known at compile-time.
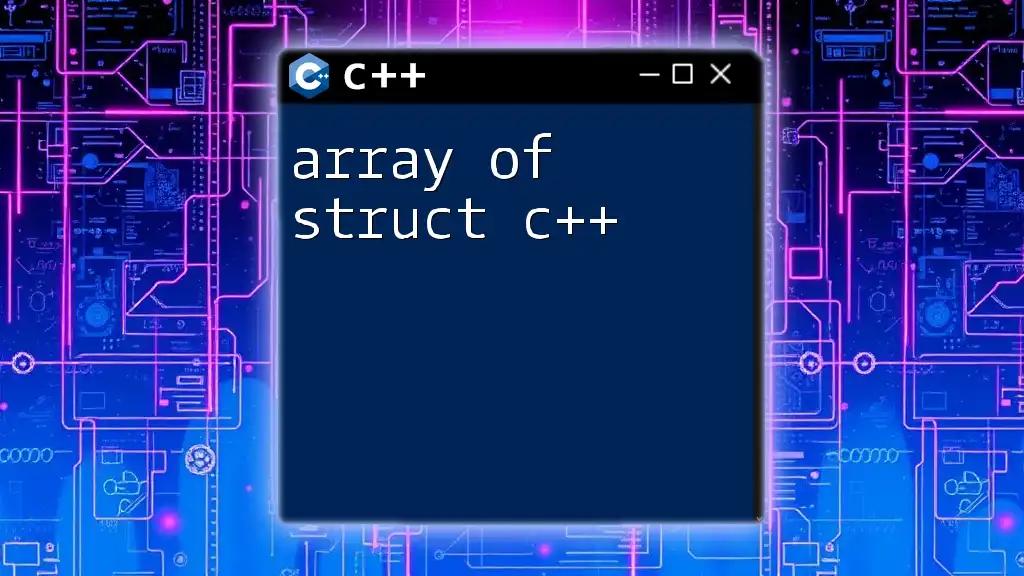
Introduction to Arrays of Arrays
What is an Array of Arrays?
An array of arrays is essentially a multidimensional array. This concept allows programmers to create a structure where each element of an array is itself another array, enabling the representation of matrices or grids.
For example, a two-dimensional array can be visualized as a grid with rows and columns, where each intersection represents a specific element. Understanding this structure is crucial for leveraging more complex data sets within C++.
Syntax of 2D Arrays
To declare and initialize a two-dimensional array, the syntax involves specifying both dimensions. Here’s how to do it:
int arr[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
This example creates a 2D array with 3 rows and 4 columns. Each sub-array (row) contains 4 elements.
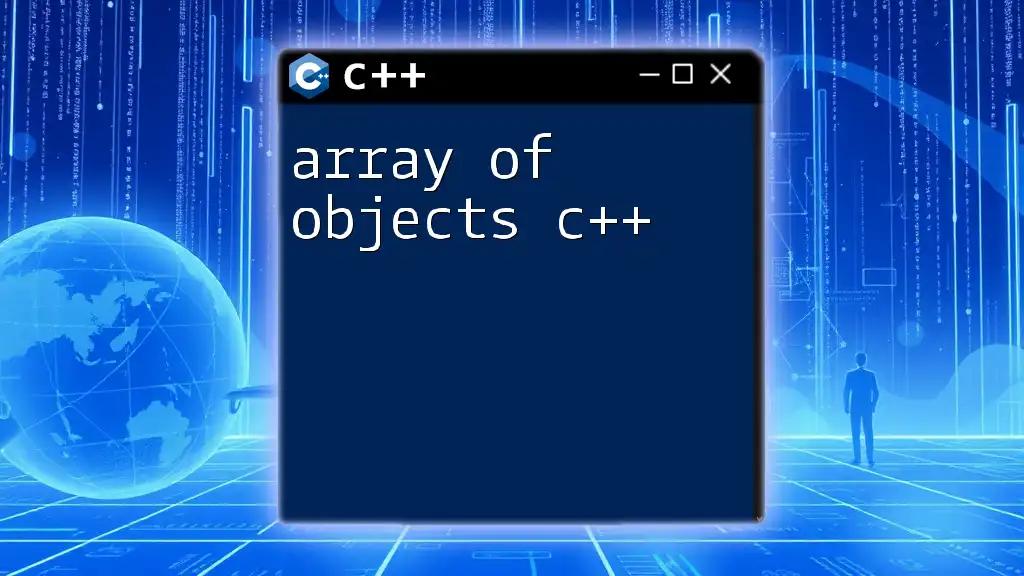
Working with Arrays of Arrays
Accessing Elements
Accessing elements in a 2D array follows a simple syntax where you specify the row index and the column index:
int x = arr[1][2]; // Accesses 7 from the array
In this case, `arr[1][2]` retrieves the third element from the second row (note that indexing starts at zero).
Looping through 2D Arrays
To traverse a 2D array, nested loops are employed. This method allows you to visit and process each element in the grid. Here’s an example of how to print all elements in a 2D array:
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 4; j++) {
cout << arr[i][j] << " ";
}
cout << endl; // New line after each row
}
In the above code, the outer loop iterates over the rows, while the inner loop iterates through the columns. This results in printing each element in a neat grid format.
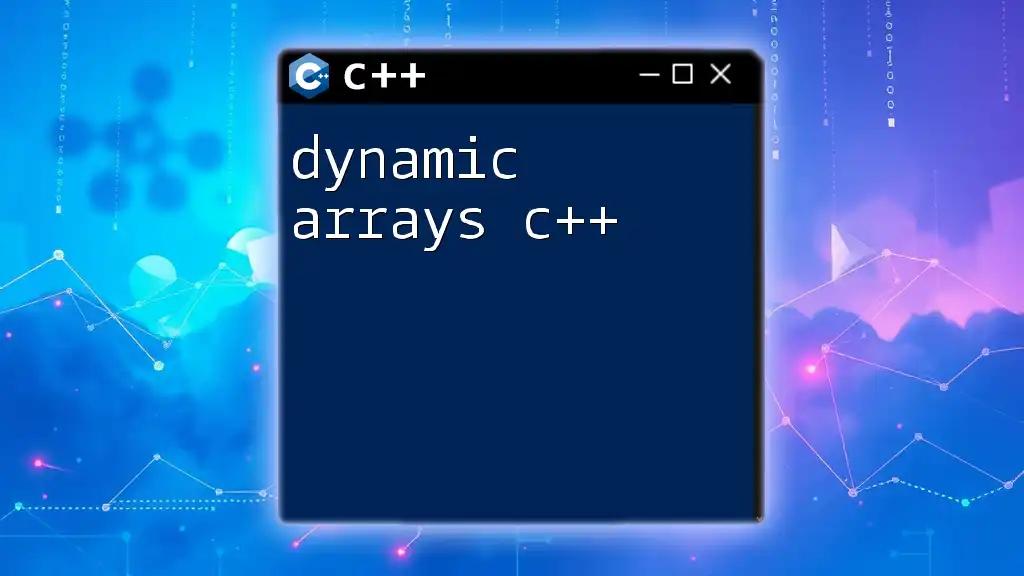
Dynamic Arrays of Arrays
What Are Dynamic Arrays?
Dynamic arrays provide the flexibility to allocate memory at runtime rather than compile-time. This is particularly useful when the size of the array is not known beforehand.
Using `new` and `delete` for Dynamic 2D Arrays
To create a dynamic 2D array, the `new` operator is used. Here’s the syntax:
int** arr = new int*[rows];
for(int i = 0; i < rows; i++) {
arr[i] = new int[cols];
}
In this example:
- An array of integer pointers is created first.
- For each pointer, a new array is created based on the specified number of columns.
Deallocating Memory
Memory management is essential in C++. Failing to deallocate memory can lead to memory leaks. To properly deallocate a dynamic 2D array, use the following:
for(int i = 0; i < rows; i++) {
delete[] arr[i];
}
delete[] arr; // Delete the array of pointers
This code ensures that all allocated memory is returned when it is no longer needed, maintaining optimal memory usage.
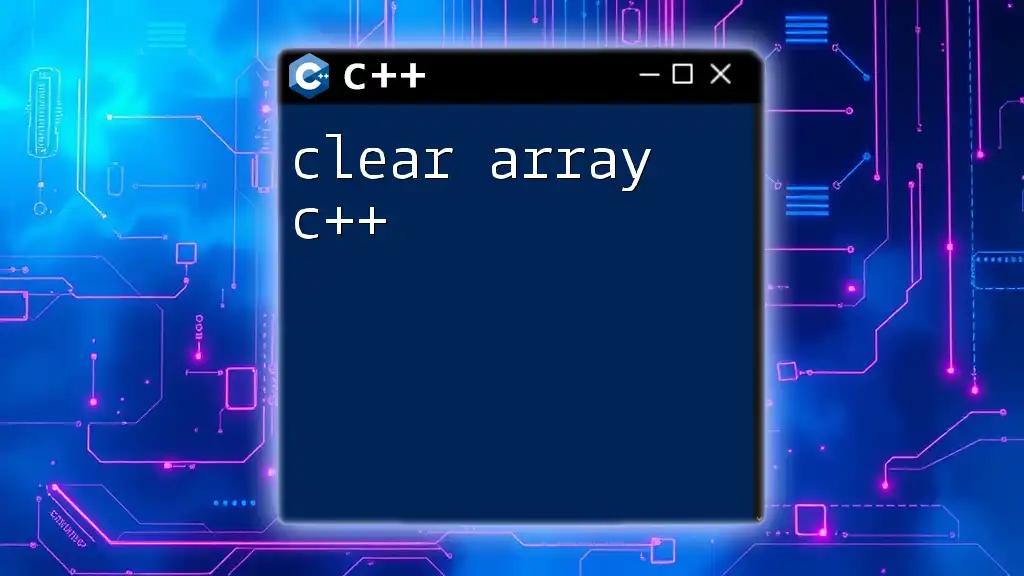
Use Cases of Arrays of Arrays
Arrays of arrays have numerous applications in real-world programming situations. They are ideal for representing grids, such as game boards or pixel maps, and for mathematical operations involving matrices. By utilizing a 2D array, complex data structures can be simplified, making it easier to implement algorithms in various domains including graphics, simulations, and more.
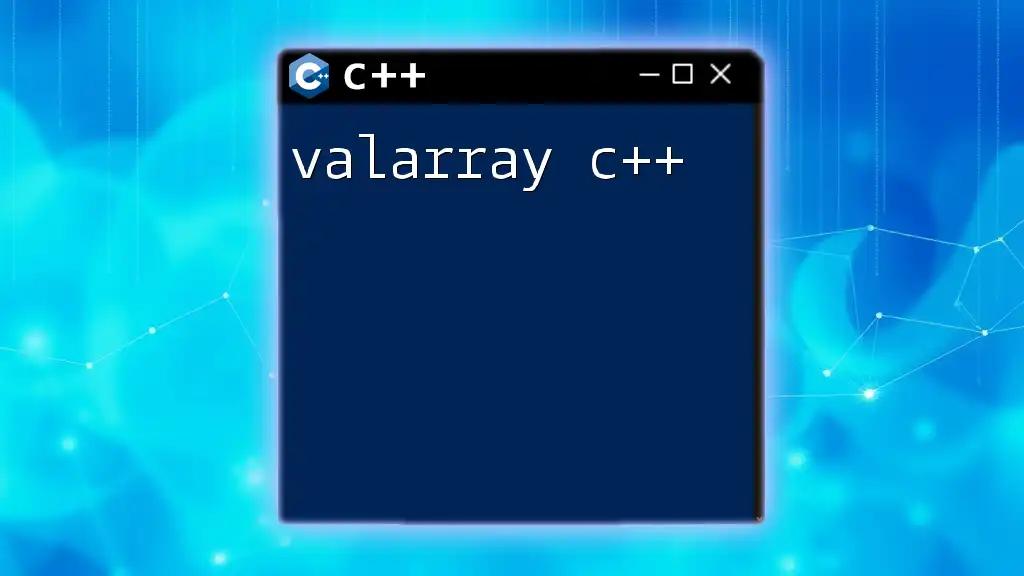
Conclusion
In summary, understanding how to use an array of arrays in C++ is crucial for effective programming, especially when dealing with multidimensional data. The concepts of syntax, memory management, and practical applications provided in this guide serve as a solid foundation. To sharpen your skills, consider practicing with the examples discussed and expanding your understanding by tackling more complex use cases.
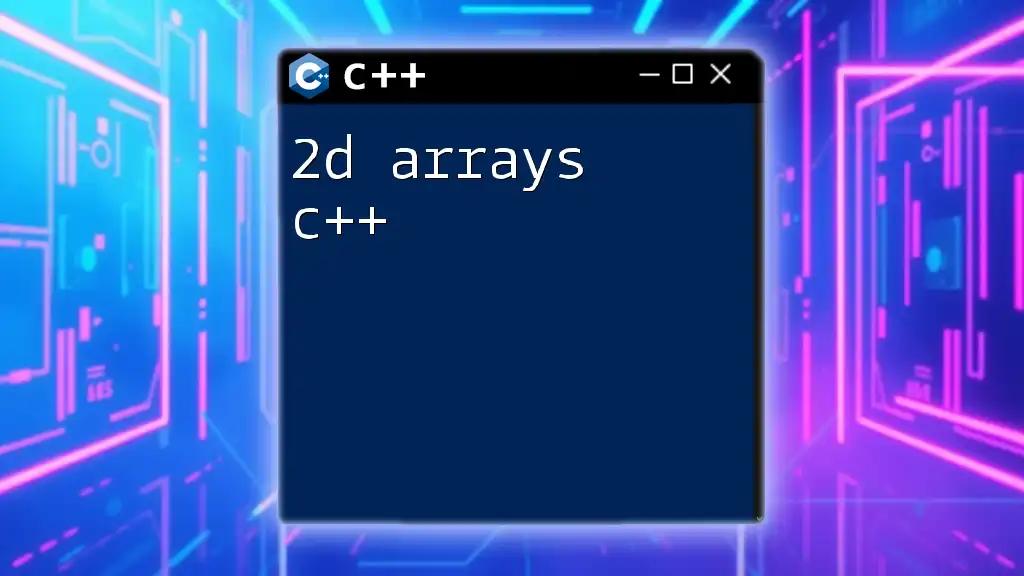
Additional Resources
For further exploration of arrays and dynamic memory in C++, consider checking online tutorials, official documentation, and coding practice platforms that provide hands-on experience with multidimensional arrays and their applications in software development.