In C++, you can get user input using the `cin` object from the `<iostream>` library to read data from the standard input stream.
Here's an example:
#include <iostream>
using namespace std;
int main() {
int userInput;
cout << "Enter a number: ";
cin >> userInput;
cout << "You entered: " << userInput << endl;
return 0;
}
Understanding User Input in C++
What is User Input?
User input refers to any information that a user provides to a program during its execution. In programming, particularly in C++, acquiring user input is critical for creating interactive applications that respond dynamically to user actions or data. Whether it's for gathering preferences, processing commands, or validating entries, user input plays an essential role in making software user-friendly.
Why Use C++ for User Input?
C++ is a powerful programming language that offers extensive control over system resources and memory management. It is particularly well-suited for applications that require high-performance operations. When it comes to user input, C++ allows for straightforward handling via its built-in libraries, making it a popular choice among developers creating interactive and console-based applications.
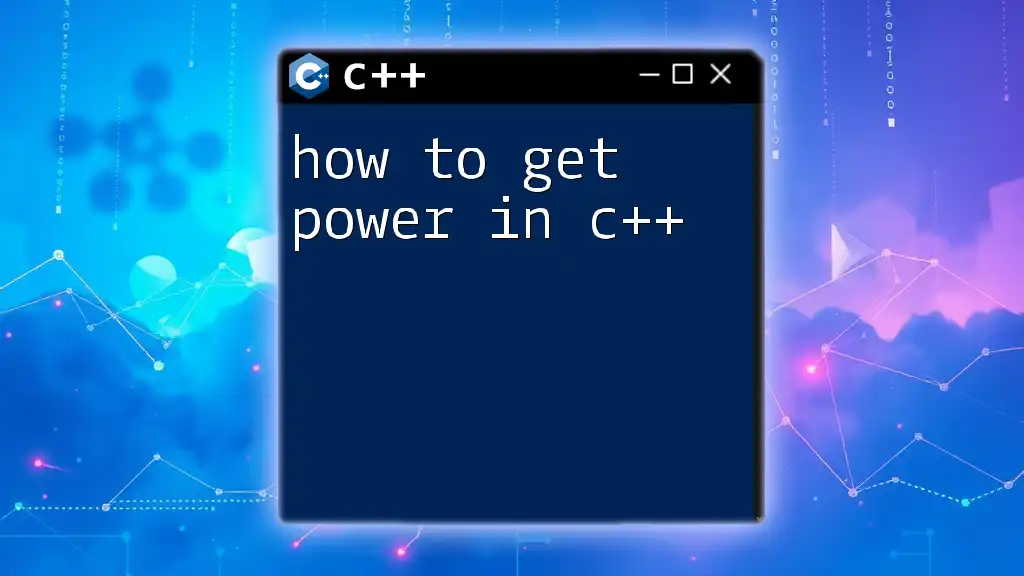
The Basics of Getting User Input in C++
Using `cin` for Standard Input
The primary way to get user input in C++ is through the `cin` object, which is part of the `iostream` library. By using `cin`, developers can easily read data from standard input (typically the keyboard).
Here’s a simple code snippet demonstrating the use of `cin` to capture an integer value from the user:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this example, the program prompts the user for their age and echoes it back. This simple interaction shows how you can get user input using C++.
Understanding Data Types and Input
When capturing user input, it is crucial to match the data type that the variable can hold with the type of data the user provides. Using the right data type ensures that the input is processed correctly. C++ supports various data types, including:
- int: For whole numbers.
- float: For decimal values.
- string: For textual data.
Here’s an example illustrating a mismatch error:
int number;
cout << "Enter a number: ";
cin >> number; // Expecting an integer
If the user inputs a non-integer value, `cin` will fail, optionally requiring additional handling to manage such errors.
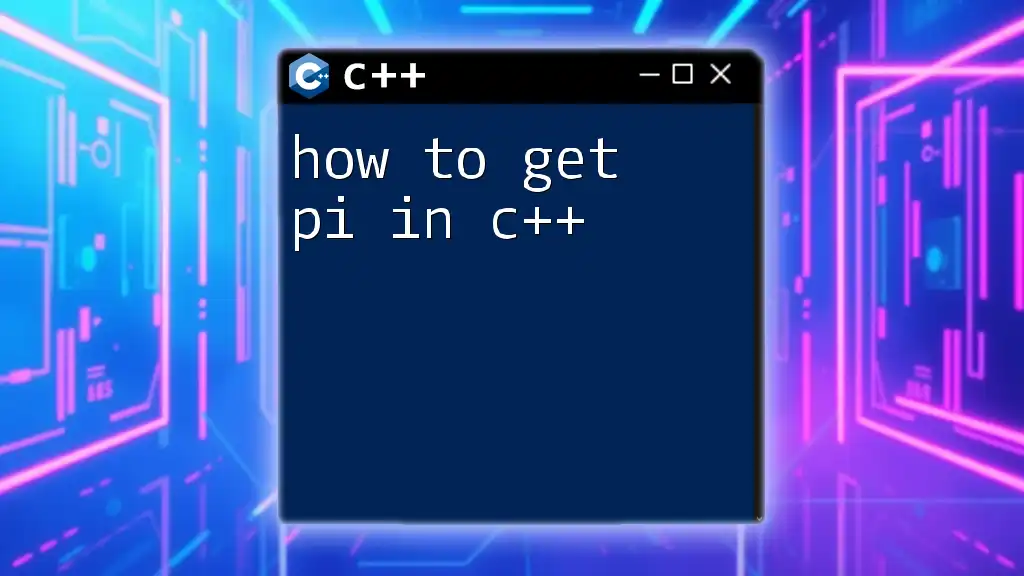
Reading Different Data Types
Getting Integer Input
Capturing integer input is straightforward with `cin`. Here’s how you can ensure that the user provides valid integer data:
int number;
cout << "Enter a number: ";
while (!(cin >> number)) {
cout << "Invalid input. Please enter an integer: ";
cin.clear(); // Clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignore bad input
}
In this code, if the user inputs an invalid type, `cin` will enter a failure state. The error is cleared, and the program prompts the user again.
Getting Float Input
To obtain floating-point numbers, you can use `cin` similarly to how you capture integer inputs. The following code demonstrates this:
float height;
cout << "Enter your height in meters: ";
cin >> height;
cout << "Your height is " << height << " meters." << endl;
This approach works for capturing decimal numbers, allowing users to input their precise height.
Getting String Input
Capturing string input can be done using both `cin` and `getline`. Generally, if you want to get a single word, `cin` suffices. However, for full sentences or strings that may include spaces, `getline` is preferred:
string name;
cout << "Enter your name: ";
getline(cin, name); // for full name input
cout << "Hello, " << name << "!" << endl;
This allows users to write their full names without being interrupted by whitespace characters, offering a more user-friendly experience.
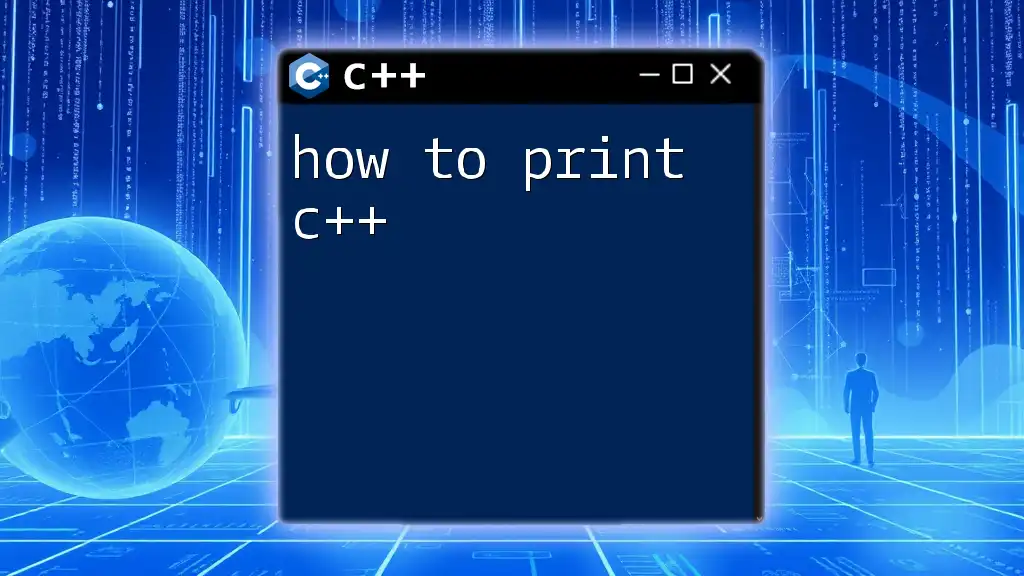
Advanced User Input Techniques
Handling Multiple Inputs
C++ makes it easy to grab multiple inputs in a single line. Here’s how you can capture two integers at once:
int x, y;
cout << "Enter two numbers separated by space: ";
cin >> x >> y; // Accepts two integer inputs
The program will read input until it encounters a whitespace, allowing users to type both values conveniently in one go.
Input Validation
Validating input is essential. It helps prevent errors and invalid data from causing issues in the program. For instance, if you want to ensure an age cannot be negative, you can employ the following method:
int age;
cout << "Enter your age: ";
cin >> age;
while (age < 0) {
cout << "Age cannot be negative. Please enter again: ";
cin >> age;
}
This validation loop checks if the entered age is valid and prompts the user until they provide an acceptable input.
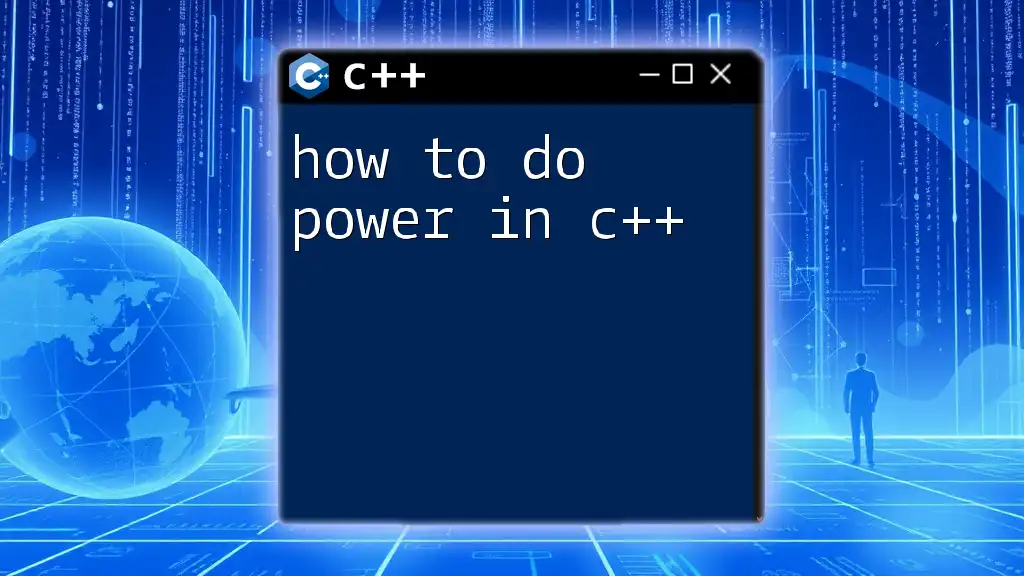
Common Pitfalls When Getting User Input
Issues with `cin` and Input Buffer
One common challenge when using `cin` is managing leftover input in the buffer. If a user enters a value of the wrong type, the input stream enters an error state. Subsequent attempts to read input with `cin` will fail until the error state is cleared, making it crucial to implement error handling as shown above.
Handling Unexpected Input
Unexpected input types can lead to runtime errors or crashes. Implementing validation techniques, such as checks for proper data types before attempting to process them, is vital for building robust applications. Using `try-catch` blocks to catch exceptions can also be useful, though they are less common in simple console applications.
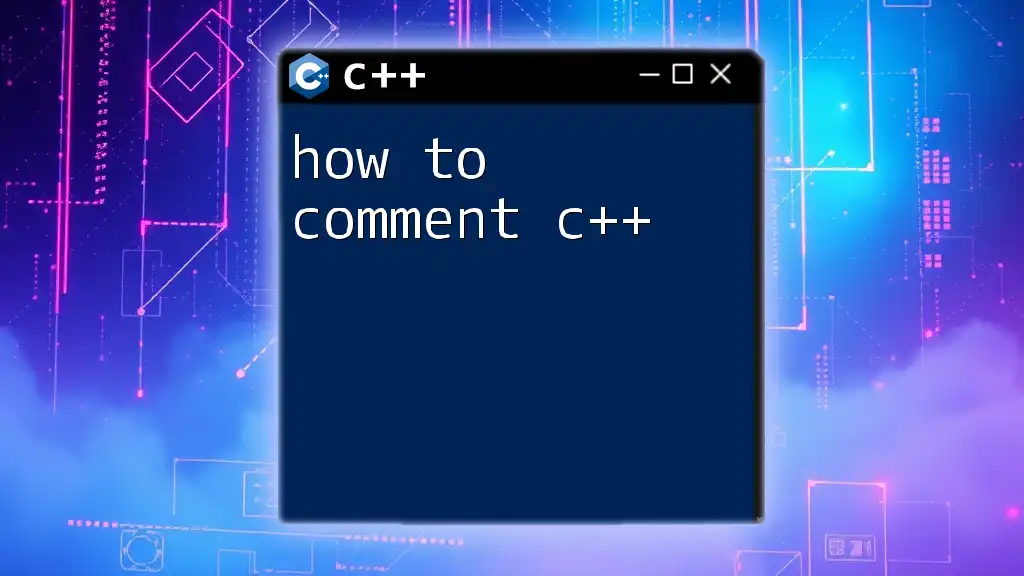
Conclusion
Understanding how to get user input in C++ is essential for creating interactive programs that engage users effectively. By leveraging the principles and techniques described above—such as using `cin`, managing data types, validating input, and handling common pitfalls—you can ensure that your C++ applications are both user-friendly and robust. With practice and application, mastering user input will significantly enhance your programming skill set and the quality of your projects.
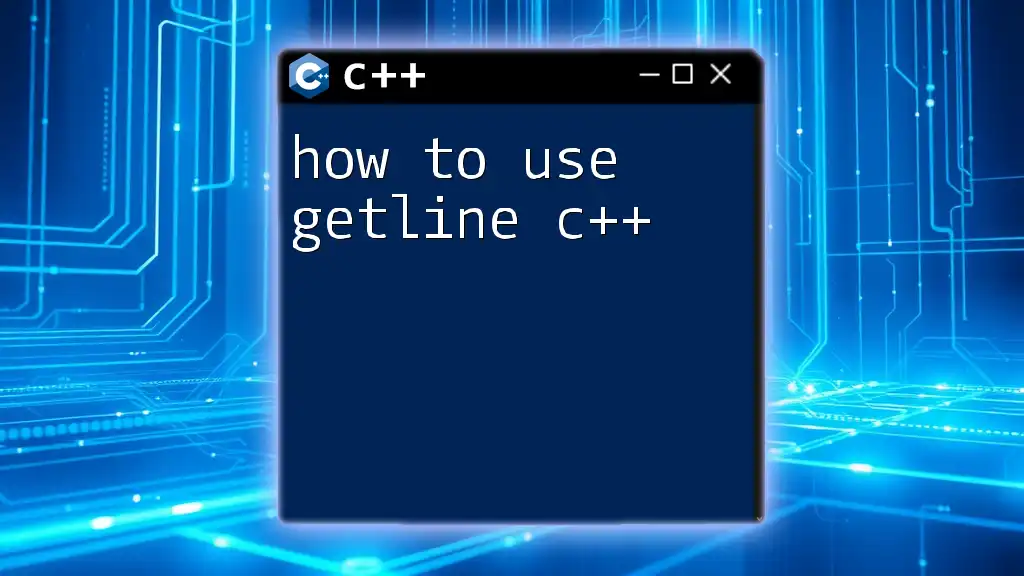
Additional Resources
For further learning, check out the official C++ documentation, which provides comprehensive information about standard libraries and input/output functions. Consider also pursuing C++ programming courses to deepen your understanding and competency in working with user input and other critical programming concepts.