You can obtain the length of a string in C++ using the `length()` method or the `size()` method, which both return the number of characters in the string.
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Length of string: " << myString.length() << std::endl; // or myString.size()
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to represent text. There are two primary types of strings in C++: C-style strings and C++ `std::string`.
- C-style strings are simply arrays of characters terminated by a null character (`\0`). They are part of C programming and require manual handling of memory and string operations.
- `std::string`, introduced in the C++ Standard Library, provides a more robust and user-friendly way to manage strings, automatically handling memory and offering various built-in methods.
Types of Strings in C++
-
C-style Strings: A character array, for example:
char myString[] = "Hello";
This representation relies heavily on null termination to indicate the end of the string.
-
C++ `std::string`: A versatile and more functional approach using the Standard Template Library (STL). Example:
std::string myString = "Hello";
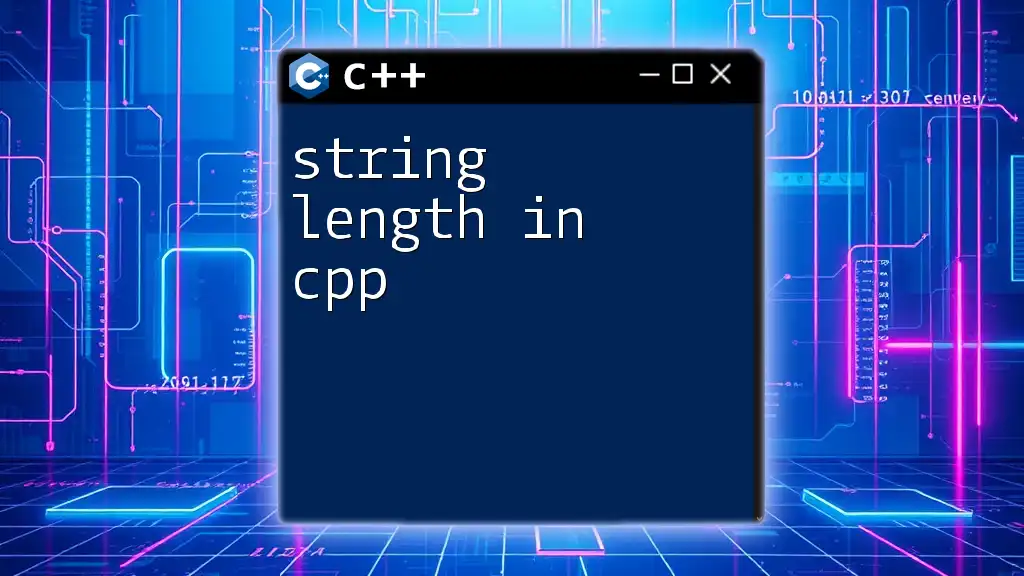
How to Get Length of String in C++
Using `std::string::length()` Method
When you're working with `std::string`, one of the simplest ways to find the length of a string is by using the `length()` method. This method returns the number of characters in the string:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Length of string: " << myString.length() << std::endl;
return 0;
}
Output:
Length of string: 13
The output shows that the string "Hello, World!" contains 13 characters, including punctuation and spaces.
Using `std::string::size()` Method
Another effective method to determine the length of a string is through the `size()` function, which works similarly to `length()`. The choice between these two methods is a matter of personal preference, as they return the same value:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Size of string: " << myString.size() << std::endl;
return 0;
}
Output:
Size of string: 13
Both `length()` and `size()` are part of the `std::string` class and effectively do the same thing—they return the number of characters in the string.
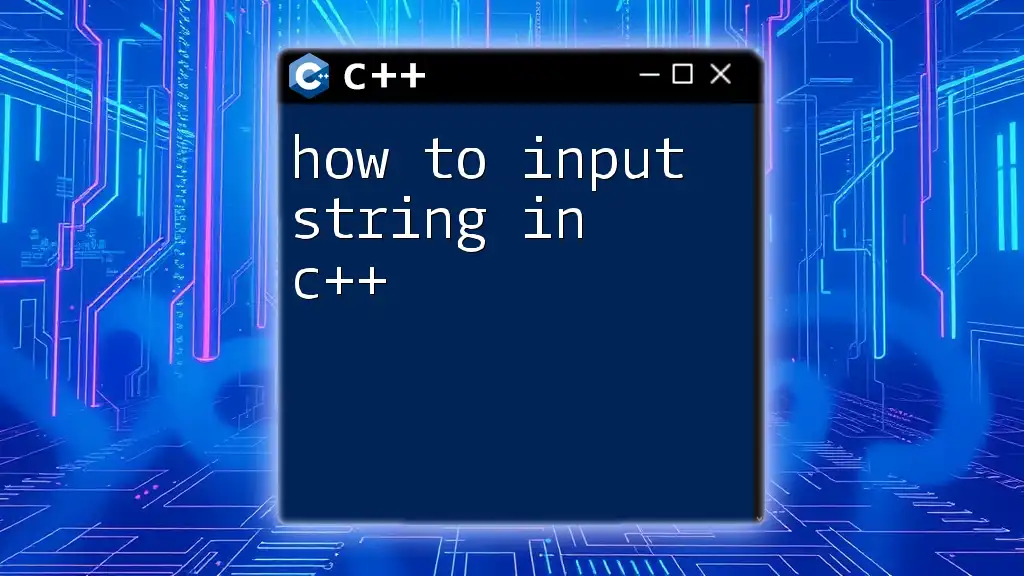
How to Get Length of C-style Strings
Using `strlen()` Function
To find the length of a C-style string, you would use the `strlen()` function, which is defined in the `<cstring>` library. It counts the number of characters until it reaches the null terminator:
#include <iostream>
#include <cstring>
int main() {
const char* myCString = "Hello, World!";
std::cout << "Length of C-style string: " << strlen(myCString) << std::endl;
return 0;
}
Output:
Length of C-style string: 13
It's important to note that `strlen()` does not count the null character itself; it stops counting when it encounters it.
Memory Considerations
C-style strings require careful attention to memory allocation and deallocation. Since they do not automatically manage memory like `std::string`, programmers face challenges such as buffer overflows or memory leaks. It’s crucial to ensure C-style strings are properly null-terminated and that memory is allocated and freed as needed.
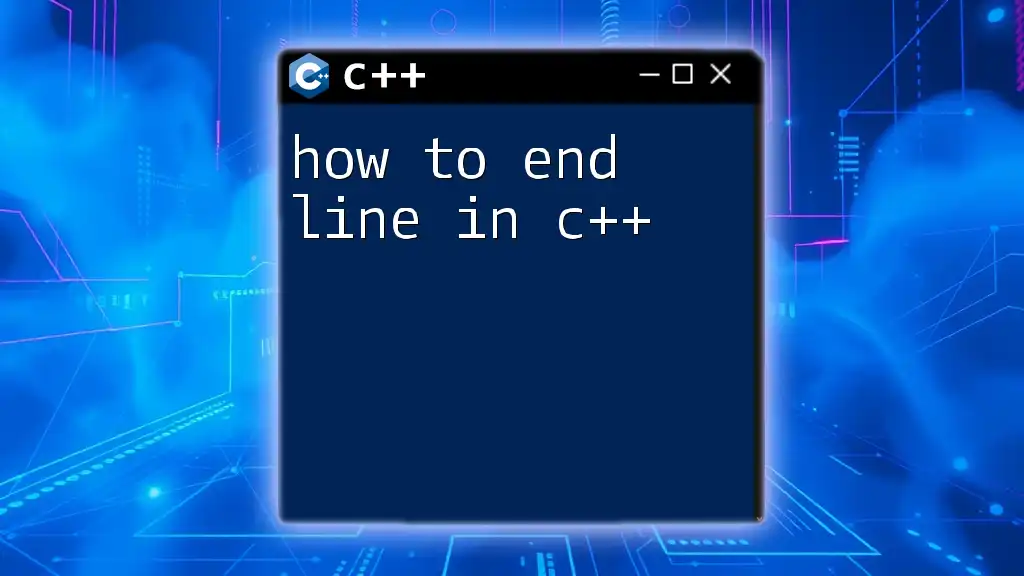
Best Practices for Finding String Length in C++
When to Use `std::string` vs. C-style Strings
The choice between using `std::string` and C-style strings often depends on the application requirements:
- `std::string`: Preferred in most modern C++ applications due to its ease of use, safety, and robust functionality. It automatically manages memory and provides useful methods for string manipulation.
- C-style strings: Useful in environments where low-level string manipulation is needed, such as performance-critical applications, or when interfacing with legacy C code.
Avoiding Common Pitfalls
- Always ensure that C-style strings are null-terminated to avoid undefined behavior.
- Prefer `std::string` for safety and convenience unless low-level manipulation is specifically required.
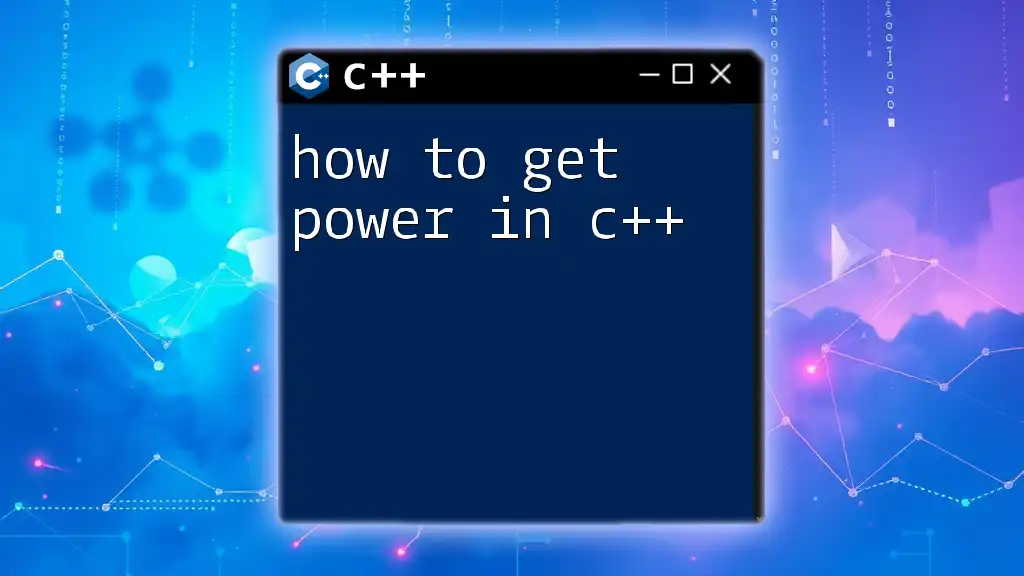
Performance Considerations
Comparing `std::string::length()` and `strlen()`
When measuring performance, it's essential to acknowledge the differences. For small strings and most typical use cases, both `std::string::length()` and `strlen()` perform satisfactorily. However, `std::string` might have a slight overhead because of its dynamic memory management and safety checks.
In performance-critical applications, benchmarks can help assess which method best suits your specific requirements.
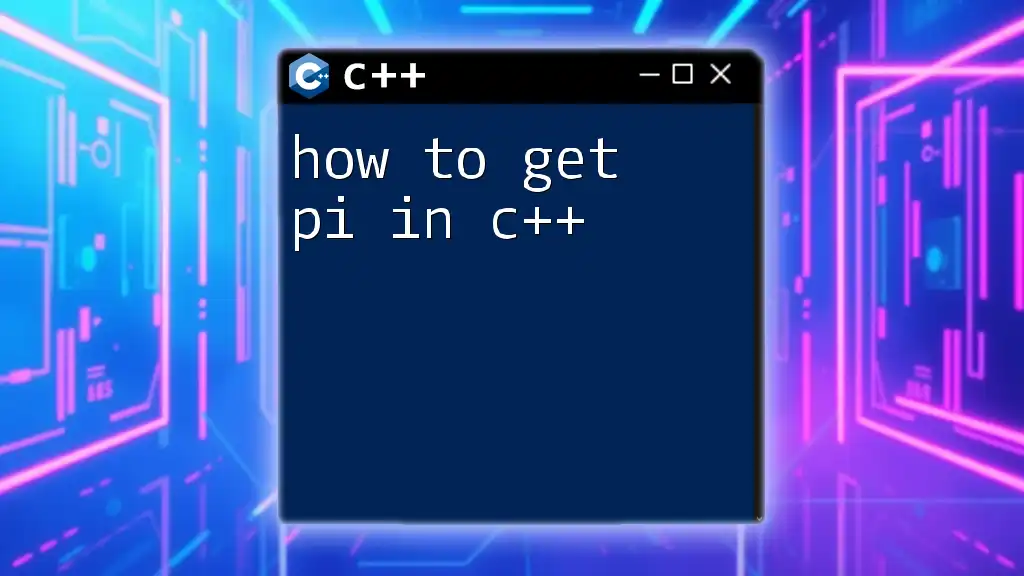
Conclusion
Understanding how to get string length in C++ is essential for effective programming. Whether using `std::string` with methods like `length()` or `size()`, or opting for C-style strings with `strlen()`, it's crucial to choose the right tool for the task while being aware of memory management principles and performance considerations.
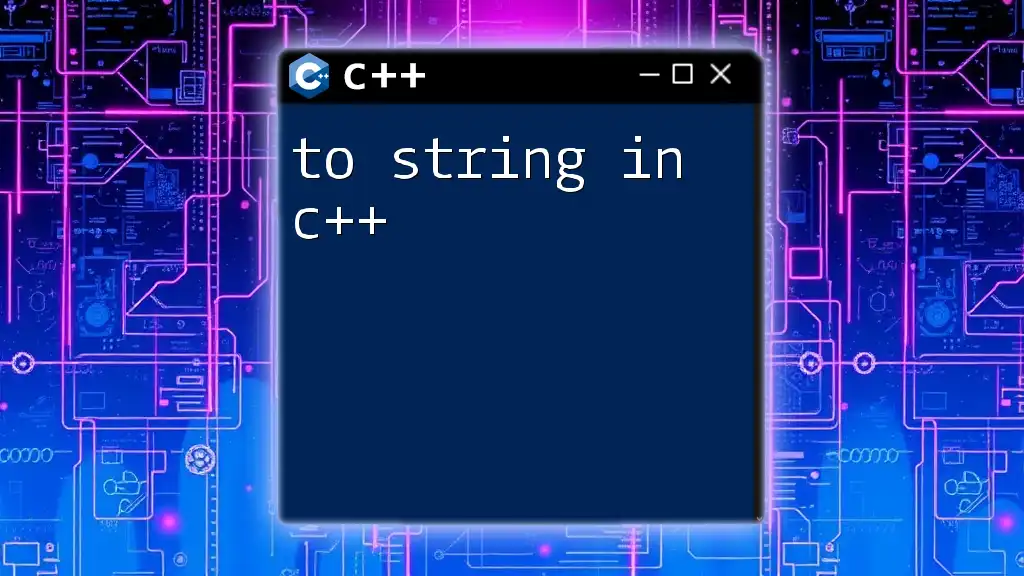
FAQs
How to Get Length of String in C++ Quickly?
The quickest method to retrieve the length of a string in C++ is to utilize `std::string::size()`, which is as efficient as `length()`.
What’s the Difference Between String Length and String Size?
In the context of `std::string`, there is no difference—`length()` and `size()` are interchangeable, both providing the count of characters in the string.
Can I Get Length of a String Without Using Libraries?
While it is possible to handle strings without libraries using basic arrays and pointers, it's generally advised against because of the potential issues related to memory management and safety. Using the Standard Library streamlines these operations significantly.