Generating random numbers in C++ can be efficiently accomplished using the `<random>` library to create a random number generator and produce numbers within a specified range. Here's a simple code snippet demonstrating this:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Obtain a random number from hardware
std::mt19937 eng(rd()); // Seed the generator
std::uniform_int_distribution<> distr(1, 100); // Define the range
// Generate random numbers
std::cout << "Random number: " << distr(eng) << std::endl;
return 0;
}
Understanding Randomness in C++
What Defines a Random Number?
Randomness is a crucial concept in computing, particularly in the field of programming. A random number is often seen as a number generated in such a manner that it cannot be reasonably predicted. Within C++, it's essential to differentiate between pseudorandom and truly random numbers.
- Pseudorandom Numbers: Generated using algorithms, these numbers appear random, but they are actually deterministic. Given the same initial input, or "seed", they will always produce the same sequence of numbers.
- True Random Numbers: These are derived from unpredictable physical phenomena (like atmospheric noise). C++ primarily deals with pseudorandom numbers, which are sufficient for most applications.
Randomness in C++: A Brief History
C++ has evolved over the years, introducing sophisticated ways to handle randomness. In C++11, the introduction of the `<random>` library provided a much more robust framework for generating random numbers compared to previous methods. This update brought along several engines and distributions, greatly enhancing the flexibility and ease of generating random numbers.
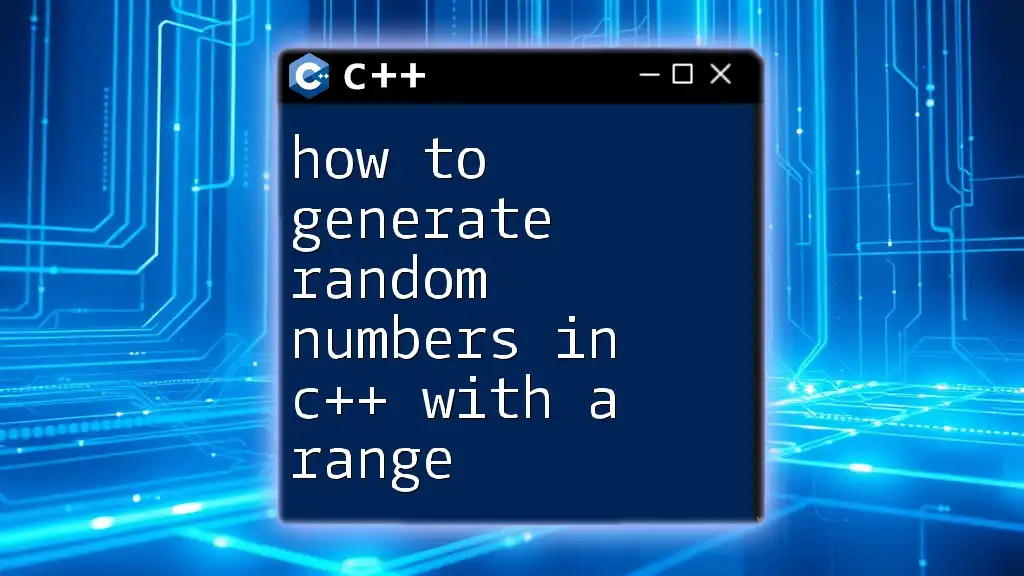
C++ Random Number Generators
How to Generate Random Numbers in C++
To get started with generating random numbers in C++, it is essential to understand the basic steps involved, including the use of the `<random>` library, which greatly simplifies the process.
Key Components of the `<random>` Library
Random Number Engines
At the heart of random number generation in C++ are random number engines. These engines generate sequences of numbers that can mimic randomness. One commonly used engine is `std::default_random_engine`. Here’s how you can set up a generator:
#include <random>
std::default_random_engine generator;
Distribution Types
Once you have a random number engine, you need to decide on the distribution of the random numbers you want to generate. Distributions define how the generated numbers are spread over a range. Common distributions include:
- Uniform Distribution: Returns numbers evenly distributed across a range.
- Normal Distribution: Produces numbers centered around a specified mean.
The choice of distribution is crucial, as it directly influences the randomness and variability of your generated numbers.
Generating Random Numbers: A Step-by-Step Guide
Step 1: Including Necessary Headers
The first step in generating random numbers in C++ is to ensure you include the necessary libraries. You can include the `<random>` and `<iostream>` headers in your program as follows:
#include <iostream>
#include <random>
Step 2: Creating a Random Number Generator
After including the relevant headers, the next step is to initialize a random number engine. It is advisable to use a random seed for better randomness. Here’s how to do that:
std::random_device rd; // Obtain a random number from hardware
std::default_random_engine eng(rd()); // Seed the generator
Step 3: Choosing a Distribution
Now that you have an engine, you can specify the distribution of the random numbers.
Uniform Distribution
To generate a random integer using a uniform distribution, you would use the `std::uniform_int_distribution`. Here’s an example that demonstrates generating a random integer in the range of 1 to 100:
std::uniform_int_distribution<int> distribution(1, 100); // Range from 1 to 100
int random_number = distribution(eng); // Get a random number
std::cout << "Random number: " << random_number << std::endl;
Normal Distribution
If you prefer to generate numbers that follow a bell curve, utilize normal distribution. For instance, to generate random floating-point numbers following a standard normal distribution with a mean of 0 and standard deviation of 1:
std::normal_distribution<double> normal_distr(0, 1); // Mean 0, Std Dev 1
std::cout << "Random number (normal): " << normal_distr(eng) << std::endl;
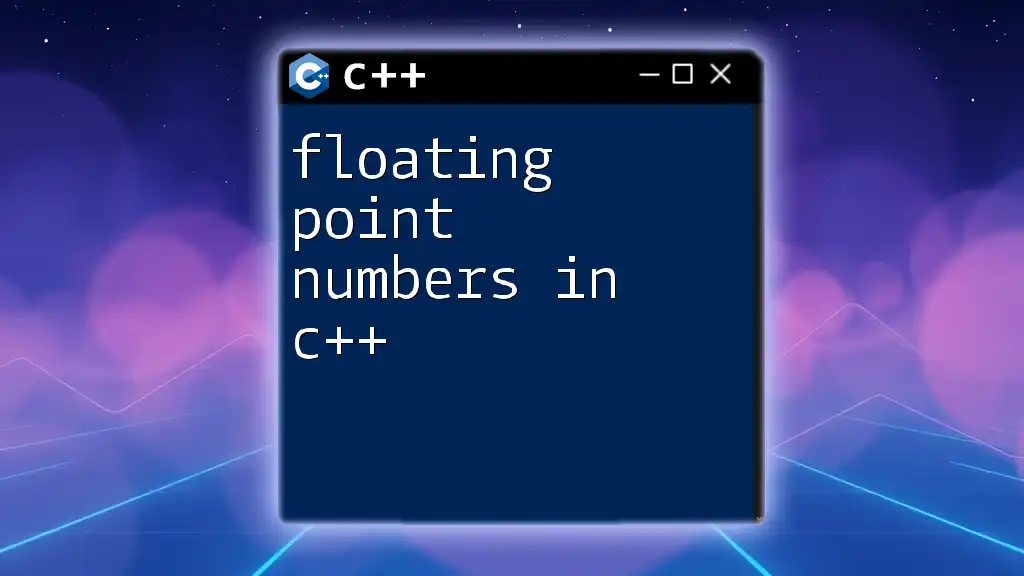
Practical Applications of Random Numbers in C++
Generating random numbers is used in a variety of applications, some of which include:
Games Development
In games, random numbers can enhance gameplay by introducing unpredictability. For example, you might generate random attributes for characters or determine the outcome of in-game events. Here’s a simple example of random health point generation for characters:
int health = distribution(eng); // Random health point between 1 and 100
Simulations and Statistical Models
Random numbers are pivotal in simulations, like Monte Carlo methods, which rely on random sampling to obtain numerical results. For instance, simulating a series of coin tosses to approximate probabilities involves generating random values.
Cryptography
In the realm of cryptography, randomness is key for ensuring data security. Cryptographic systems rely on high-quality random numbers to generate keys that are difficult to predict and replicate.
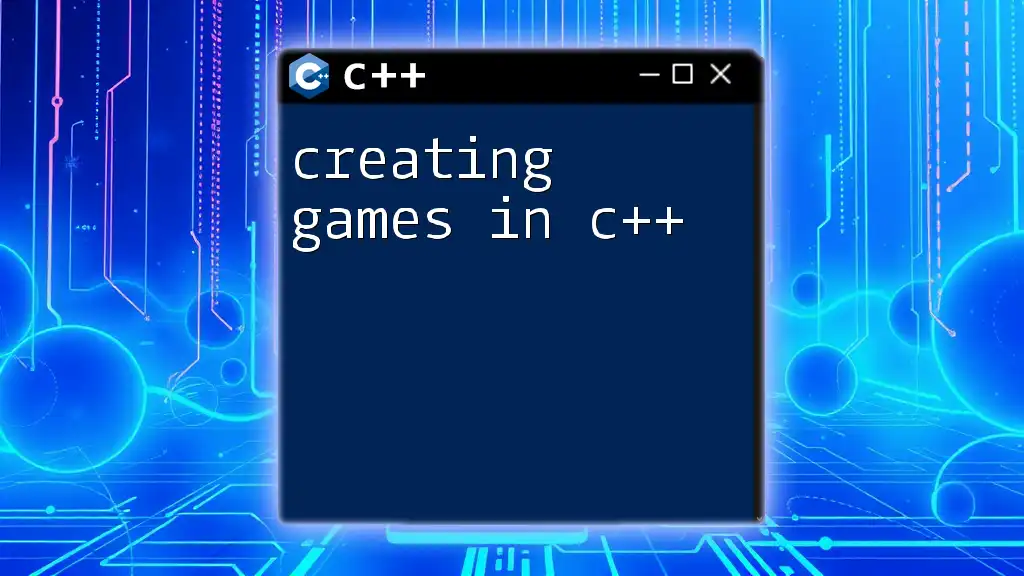
Common Pitfalls and Mistakes
Misunderstanding Randomness
One common misconception is believing that random numbers generated are utterly unique and independent of each other. In reality, pseudorandom numbers generated using the same seed will produce identical sequences, which can lead to issues in applications requiring high entropy.
Performance Considerations
While C++ offers various engines and distributions, understanding the performance implications of different choices is crucial. Some engines may be more efficient than others depending on the context in which they are used.
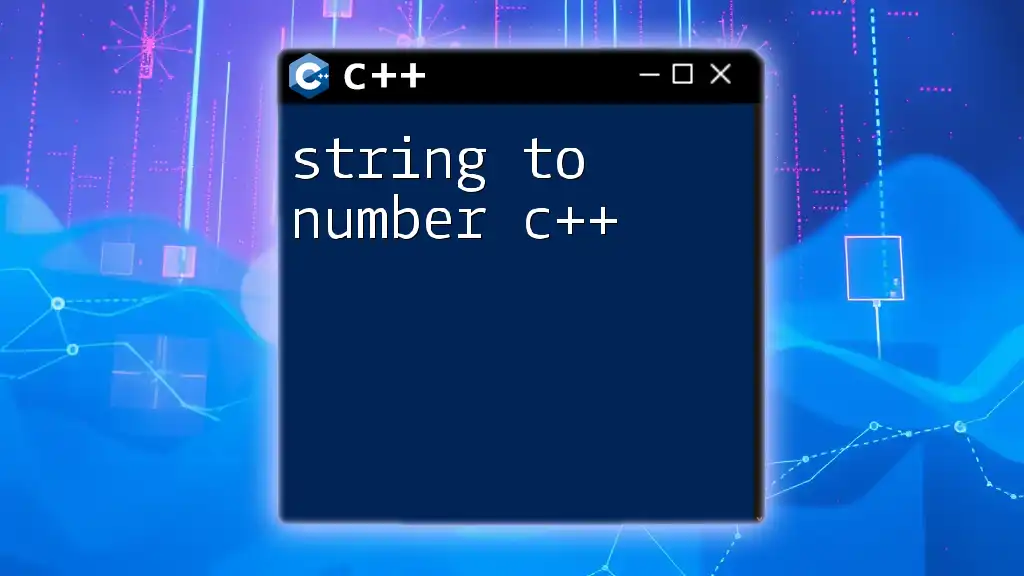
Conclusion
Mastering generating random numbers in C++ is a fundamental skill that opens the door to building complex and unpredictable programs. By leveraging the `<random>` library, you can easily create random numbers tailored to your application needs.
Practice through examples, explore different distributions, and integrate a variety of applications to truly harness the power of randomness in your C++ projects. Don’t hesitate to delve deeper into the available resources and exercises that can further enhance your understanding and expertise in this essential programming concept.